Elasticsearch数据模型与集群架构介绍
发布时间: 2024-01-13 03:57:27 阅读量: 34 订阅数: 21 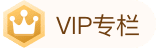
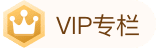
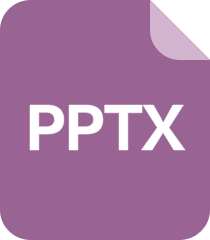
Elasticsearch介绍
# 1. 引言
## 1.1 什么是Elasticsearch
Elasticsearch是一个开源的分布式搜索和分析引擎,基于Apache Lucene构建。它提供了一个高效、强大且易于扩展的全文搜索引擎,能够快速地对大数据集进行搜索和分析。
## 1.2 Elasticsearch的应用场景
Elasticsearch广泛应用于日志分析、实时监控、搜索引擎、地理位置搜索、文本搜索与相关性排序等领域。其分布式特性使得它能够处理海量数据,并通过聚合框架进行实时分析。
## 1.3 为什么要学习Elasticsearch的数据模型与集群架构
Elasticsearch作为目前最流行的开源搜索引擎之一,具有广泛的应用场景和强大的搜索与分析能力。了解其数据模型与集群架构,不仅有助于构建高效的搜索系统,还能够提升数据处理和分析的能力,对于提升工作效率和解决实际问题具有重要意义。
以上是第一章的内容,接下来是第二章的内容。
# 2. Elasticsearch基础知识
Elasticsearch作为一个开源的分布式搜索引擎,具有强大的实时搜索和分析能力。在深入学习Elasticsearch的数据模型与集群架构之前,我们有必要先了解一些Elasticsearch的基础知识,包括核心概念、基本架构和数据流程。
### 2.1 Elasticsearch的核心概念
在使用Elasticsearch之前,需要了解一些核心概念:
- **文档(Document)**:Elasticsearch存储的最小数据单元,以JSON格式表示。
- **索引(Index)**:一组有相似结构的文档的集合,类似于关系型数据库中的表。
- **分片(Shard)**:每个索引可以被分成多个分片,用于提高数据的并行性能以及容错性。
- **复制(Replica)**:每个分片可以有零个或多个副本,副本用于提高系统的容错性和可用性。
- **节点(Node)**:一个运行着Elasticsearch实例的服务器,是集群的一部分。
### 2.2 Elasticsearch的基本架构
Elasticsearch采用分布式架构,主要由以下组件构成:
- **节点(Node)**:每个节点是集群的一部分,可以存储数据、执行数据操作和参与集群的行为。
- **分片(Shard)**:数据的基本单元,每个文档都存储在一个分片中。
- **复制(Replica)**:每个分片可以有零个或多个副本,用于提高系统的容错性和可用性。
### 2.3 Elasticsearch的数据流程
当数据被索引到Elasticsearch时,会经历以下流程:
1. **Indexing**:客户端将文档索引到Elasticsearch中。
2. **Analysis**:文档被分析器处理,分解成适合索引的单词或词项。
3. **Indexing**:分析后的词项被索引,以便后续搜索。
4. **Search**:用户可以执行搜索请求,Elasticsearch在索引中查找匹配的文档并返回结果。
以上是Elasticsearch基础知识的简要介绍,这为后续深入学习Elasticsearch的数据模型和集群架构打下了基础。
接下来,我们将深入探讨Elasticsearch的数据模型,包括索引的创建与管理、文档的插入与更新、数据的查询与搜索以及数据的分析与聚合。
# 3. Elasticsearch数据模型
### 3.1 索引的创建与管理
在Elasticsearch中,数据被组织成一个或多个索引。索引是具有相似特征的文档的集合。本节将介绍如何创建和管理索引。
#### 3.1.1 创建索引
要创建一个新的索引,可以使用Elasticsearch的REST API发送一个PUT请求。
```python
import requests
def create_index(index_name):
url = f"http://localhost:9200/{index_name}"
headers = {
"Content-Type": "application/json"
}
response = requests.put(url, headers=headers)
if response.status_code == 200:
print(f"Index '{index_name}' created successfully.")
else:
print(f"Failed to create index '{index_name}'. Error: {response.text}")
index_name = "my_index"
create_index(index_name)
```
该代码使用Python的requests库向Elasticsearch发送PUT请求来创建索引。其中,index_name是待创建的索引名称。如果成功创建索引,将返回状态码200,并打印"Index 'my_index' created successfully."。如果创建失败,将返回相应的错误信息。
#### 3.1.2 索引的映射
在创建索引时,可以指定索引的映射,即定义字段的数据类型和属性。Elasticsearch支持多种数据类型,如字符串、整数、浮点数、日期等。
```python
import requests
def create_index_with_mapping(index_name):
url = f"http://localhost:9200/{index_name}"
headers = {
"Content-Type": "application/json"
}
data = {
"mappings": {
"properties": {
"title": {
"type": "text"
},
"content": {
"type": "text"
},
"publish_date": {
"type": "date"
}
}
}
}
response = requests.put(url, headers=headers, json=data)
if response.status_code == 200:
print(f"Index '{index_name}' created with mapping successfully.")
else:
print(f"Failed to create index '{index_name}' with mapping. Error: {response.text}")
index_name = "my_index"
create_index_with_mapping(index_name)
```
在上述代码中, 我们通过在`mappings`字段下定义每个字段的数据类型和属性来创建索引的映射。如果成功创建索引和映射,将返回状态码200,并打印"Index 'my_index' created with mapping successfully."。如果创建失败,将返回相应的错误信息。
#### 3.1.3 删除索引
要删除一个索引,可以使用Elasticsearch的REST API发送一个DELETE请求。
```python
import requests
def delete_index(index_name):
url = f"http://localhost:9200/{index_name}"
response = requests.delete(url)
if response.status_code == 200:
print(f"Index '{index_name}' deleted successfully.")
else:
print(f"Failed to delete index '{index_name}'. Error: {response.text}")
index_name = "my_index"
delete_index(index_name)
```
该代码使用Python的requests库向Elasticsearch发送DELETE请求来删除索引。如果成功删除索引,将返回状态码200,并打印"Index 'my_index' deleted successfully."。如果删除失败,将返回相应的错误信息。
### 3.2 文档的插入与更新
在Elasticsearch中,文档是最基本的数据单元。每个文档都有一个唯一的ID,并且属于某个索引。本节将介绍如何插入和更新文档。
#### 3.2.1 插入文档
要插入一个文档,可以使用Elasticsearch的REST API发送一个POST请求。
```python
import requests
def insert_document(index_name, document):
url = f"http://localhost:9200/{index_name}/_doc"
headers = {
"Content-Type": "application/json"
}
response = requests.post(url, headers=headers, json=document)
if response.status_code == 201:
print(f"Document inserted successfully.")
else:
print(f"Failed to
```
0
0
相关推荐
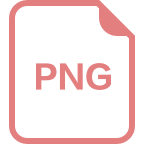
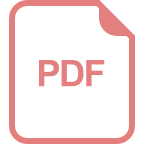
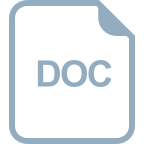
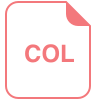
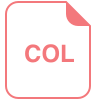
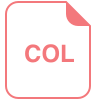
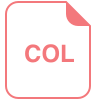
