揭秘HTML5与CSS3的实战利器:打造响应式网页的秘籍
发布时间: 2024-07-19 19:39:10 阅读量: 24 订阅数: 21 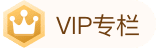
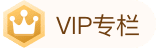
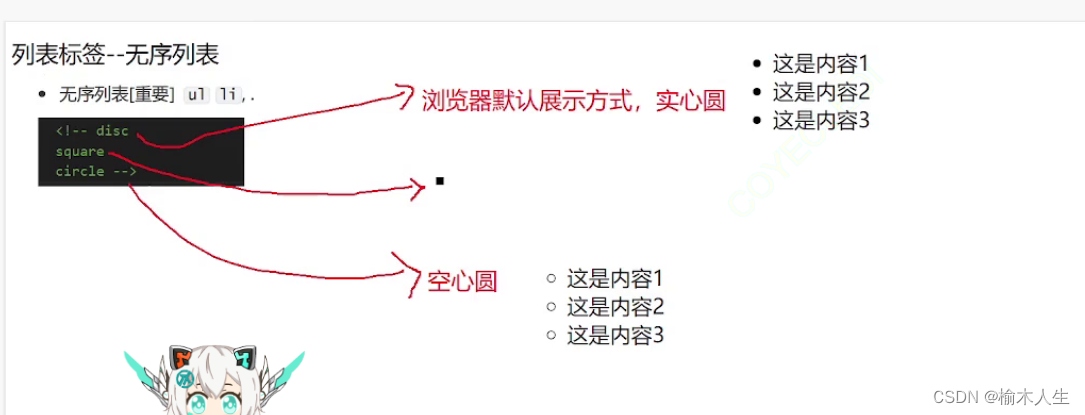
# 1. HTML5与CSS3基础
HTML5和CSS3是现代Web开发的基础技术。HTML5为网页内容提供了结构和语义,而CSS3则用于定义网页的外观和布局。
HTML5引入了许多新元素和属性,使开发人员能够创建更丰富、更交互的网页。例如,`<canvas>`元素允许创建动态图形,而`<video>`元素允许播放视频。
CSS3提供了许多新功能,使开发人员能够创建更复杂和更美观的网页布局。例如,`flexbox`布局允许开发人员创建灵活的布局,可以根据设备屏幕大小进行调整。
# 2. 响应式网页设计原理
### 2.1 流式布局和媒体查询
#### 流式布局
流式布局是一种响应式设计技术,它允许网页元素根据可用空间自动调整大小和位置。流式布局使用百分比或ems等相对单位来定义元素的尺寸,而不是固定的像素值。
#### 媒体查询
媒体查询是一种CSS技术,它允许您根据设备的屏幕尺寸、方向或其他特性来应用不同的样式。媒体查询使用`@media`规则,它可以检测设备的功能并应用特定的样式表。
**代码块:**
```css
@media (max-width: 768px) {
/* 适用于屏幕宽度小于或等于 768px 的设备 */
body {
font-size: 14px;
}
}
@media (min-width: 769px) and (max-width: 1024px) {
/* 适用于屏幕宽度在 769px 到 1024px 之间的设备 */
body {
font-size: 16px;
}
}
@media (min-width: 1025px) {
/* 适用于屏幕宽度大于或等于 1025px 的设备 */
body {
font-size: 18px;
}
}
```
**逻辑分析:**
这段代码定义了三个媒体查询:
* 第一个媒体查询适用于屏幕宽度小于或等于 768px 的设备,它将`body`元素的字体大小设置为 14px。
* 第二个媒体查询适用于屏幕宽度在 769px 到 1024px 之间的设备,它将`body`元素的字体大小设置为 16px。
* 第三个媒体查询适用于屏幕宽度大于或等于 1025px 的设备,它将`body`元素的字体大小设置为 18px。
### 2.2 弹性盒布局和网格系统
#### 弹性盒布局
弹性盒布局是一种CSS布局模型,它允许您控制元素在容器中的位置、大小和对齐方式。弹性盒布局使用`flexbox`属性来定义元素的布局行为。
#### 网格系统
网格系统是一种响应式设计技术,它使用网格来组织网页内容。网格系统定义了列和行的网格,您可以将元素放置在网格中以创建响应式布局。
**代码块:**
```html
<div class="container">
<div class="row">
<div class="col-sm-6">...</div>
<div class="col-sm-6">...</div>
</div>
<div class="row">
<div class="col-sm-4">...</div>
<div class="col-sm-4">...</div>
<div class="col-sm-4">...</div>
</div>
</div>
```
**逻辑分析:**
这段代码使用Bootstrap网格系统创建了一个响应式布局:
* `container`类定义了一个容器,它将限制网格的宽度。
* `row`类定义了一行,它将元素水平排列。
* `col-sm-6`类定义了一个占据一行中 50% 空间的列。
* `col-sm-4`类定义了一个占据一行中 33.33% 空间的列。
**mermaid流程图:**
```mermaid
graph LR
subgraph 流式布局
A[流式布局]
B[百分比单位]
C[ems 单位]
end
subgraph 媒体查询
D[媒体查询]
E[@media 规则]
F[设备功能检测]
end
subgraph 弹性盒布局
G[弹性盒布局]
H[flexbox 属性]
I[元素布局行为]
end
subgraph 网格系统
J[网格系统]
K[网格]
L[列和行]
end
```
# 3. HTML5 实战应用**
### 3.1 表单增强和多媒体支持
HTML5 引入了许多增强表单功能的新特性,使 Web 开发人员能够创建更强大、更用户友好的表单。这些特性包括:
- **placeholder 属性:**为表单字段提供占位符文本,指导用户输入。
- **required 属性:**要求用户在提交表单之前填写特定字段。
- **pattern 属性:**指定输入字段必须匹配的正则表达式。
- **datalist 属性:**提供预定义选项列表,供用户在输入字段中选择。
- **autofocus 属性:**自动将焦点设置到指定的表单字段。
此外,HTML5 还提供了对各种多媒体格式的支持,包括:
- **audio 元素:**用于嵌入音频文件。
- **video 元素:**用于嵌入视频文件。
- **canvas 元素:**用于在网页上绘制图形。
### 3.2 Canvas 和 WebGL 图形编程
**Canvas 元素**
Canvas 元素是一个用于在网页上绘制图形的位图画布。它提供了丰富的 API,允许开发人员创建各种图形,包括:
- 形状(矩形、圆形、线条)
- 文本
- 图像
- 动画
**代码示例:**
```javascript
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
// 绘制一个矩形
ctx.fillStyle = "red";
ctx.fillRect(10, 10, 100, 100);
// 绘制一个圆形
ctx.beginPath();
ctx.arc(150, 150, 50, 0, 2 * Math.PI);
ctx.fillStyle = "blue";
ctx.fill();
```
**WebGL**
WebGL 是一种基于 OpenGL ES 2.0 的 JavaScript API,用于在网页上进行 3D 图形编程。它允许开发人员创建交互式 3D 场景,包括:
- 3D 模型
- 纹理
- 光照
- 阴影
**代码示例:**
```javascript
// 创建 WebGL 上下文
const gl = canvas.getContext("webgl");
// 创建顶点着色器
const vertexShader = gl.createShader(gl.VERTEX_SHADER);
gl.shaderSource(vertexShader, vertexShaderSource);
gl.compileShader(vertexShader);
// 创建片元着色器
const fragmentShader = gl.createShader(gl.FRAGMENT_SHADER);
gl.shaderSource(fragmentShader, fragmentShaderSource);
gl.compileShader(fragmentShader);
// 创建着色器程序
const program = gl.createProgram();
gl.attachShader(program, vertexShader);
gl.attachShader(program, fragmentShader);
gl.linkProgram(program);
// 绑定着色器程序
gl.useProgram(program);
// 创建顶点数据
const vertices = [
-1, -1, 0,
1, -1, 0,
0, 1, 0,
];
// 创建顶点缓冲区对象
const vertexBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, vertexBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(vertices), gl.STATIC_DRAW);
// 设置顶点属性
const positionAttributeLocation = gl.getAttribLocation(program, "a_position");
gl.enableVertexAttribArray(positionAttributeLocation);
gl.vertexAttribPointer(positionAttributeLocation, 3, gl.FLOAT, false, 0, 0);
// 清除颜色缓冲区
gl.clearColor(0, 0, 0, 1);
gl.clear(gl.COLOR_BUFFER_BIT);
// 绘制三角形
gl.drawArrays(gl.TRIANGLES, 0, 3);
```
# 4. CSS3 实战应用
### 4.1 3D 变换和动画效果
#### 3D 变换
CSS3 引入了 3D 变换属性,允许对元素进行三维空间的旋转、平移和缩放。这些属性包括:
- `transform`: 设置元素的整体变换,包括旋转、平移和缩放。
- `translate`: 平移元素。
- `rotate`: 旋转元素。
- `scale`: 缩放元素。
例如,以下代码将元素沿 x 轴旋转 45 度:
```css
transform: rotateX(45deg);
```
#### 动画效果
CSS3 还提供了强大的动画功能,允许对元素的属性进行平滑的过渡。动画效果可以通过 `transition` 和 `animation` 属性实现。
- `transition`: 定义元素在属性更改时如何过渡。
- `animation`: 定义元素的动画效果,包括持续时间、延迟和重复次数。
例如,以下代码将元素的背景颜色在 2 秒内从红色过渡到蓝色:
```css
transition: background-color 2s;
background-color: blue;
```
### 4.2 滤镜和混合模式
#### 滤镜
CSS3 滤镜允许对元素应用各种视觉效果,例如模糊、色调和饱和度调整。滤镜属性包括:
- `filter`: 设置元素的滤镜效果。
- `blur`: 模糊元素。
- `hue-rotate`: 旋转元素的色调。
- `saturate`: 调整元素的饱和度。
例如,以下代码将元素模糊 10 像素:
```css
filter: blur(10px);
```
#### 混合模式
混合模式允许将元素与背景或其他元素混合。混合模式属性包括:
- `mix-blend-mode`: 设置元素的混合模式。
- `multiply`: 将元素与背景相乘。
- `screen`: 将元素与背景相加。
- `overlay`: 将元素与背景叠加。
例如,以下代码将元素与背景混合,使用叠加模式:
```css
mix-blend-mode: overlay;
```
# 5. 响应式网页开发实践
### 5.1 响应式网页布局设计
#### 流式布局和媒体查询
流式布局是一种响应式布局技术,它允许网页元素根据可用空间自动调整大小和位置。媒体查询是一种 CSS 技术,它允许您针对特定屏幕尺寸或设备类型设置不同的样式。
**代码块:**
```css
/* 流式布局 */
.container {
width: 100%;
max-width: 1200px;
margin: 0 auto;
}
/* 媒体查询 */
@media (max-width: 768px) {
.container {
max-width: 768px;
}
}
```
**逻辑分析:**
* `.container` 类定义了一个流式布局容器,其宽度为 100%,最大宽度为 1200px,并水平居中。
* 媒体查询针对最大宽度为 768px 的设备设置了不同的样式,将 `.container` 的最大宽度限制为 768px。
#### 弹性盒布局和网格系统
弹性盒布局是一种 CSS 布局模型,它允许您控制元素在容器内的排列和对齐方式。网格系统是一种 CSS 框架,它提供了预定义的布局结构,简化了响应式网页设计。
**代码块:**
```css
/* 弹性盒布局 */
.flex-container {
display: flex;
justify-content: center;
align-items: center;
}
/* 网格系统 */
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-gap: 10px;
}
```
**逻辑分析:**
* `.flex-container` 类使用弹性盒布局,将元素水平居中并垂直居中。
* `.grid-container` 类使用网格系统,创建了一个三列网格,列间距为 10px。
### 5.2 响应式网页测试和优化
#### 响应式网页测试
响应式网页测试是确保您的网页在不同设备和屏幕尺寸上都能正常显示和运行的过程。您可以使用在线工具(例如 Google Mobile-Friendly Test)或设备模拟器来测试您的网页。
#### 响应式网页优化
响应式网页优化涉及提高网页在不同设备上的性能和用户体验。一些优化技巧包括:
* 使用轻量级图像和视频
* 优化 CSS 和 JavaScript 文件
* 避免使用过多的动画和效果
* 确保网页加载速度快
**表格:响应式网页优化技巧**
| 优化技巧 | 描述 |
|---|---|
| 使用轻量级图像和视频 | 压缩图像并使用适当的文件格式,例如 JPEG、PNG 和 WebP。 |
| 优化 CSS 和 JavaScript 文件 | 缩小、压缩和合并 CSS 和 JavaScript 文件。 |
| 避免使用过多的动画和效果 | 过多的动画和效果会降低网页的性能。 |
| 确保网页加载速度快 | 使用内容分发网络 (CDN) 和缓存技术来加快网页加载速度。 |
# 6.1 HTML5离线存储和Web Workers
### HTML5离线存储
**应用场景:**
* 允许网页在没有网络连接的情况下访问数据,提升用户体验。
* 适用于缓存静态资源(如图像、视频、脚本)或动态数据(如用户偏好设置)。
**实现方式:**
* **Application Cache:** 浏览器内置的缓存机制,用于缓存静态资源。
* **IndexedDB:** 一个NoSQL数据库,用于存储结构化数据。
* **Web Storage:** 包括sessionStorage和localStorage,用于存储键值对数据。
### Web Workers
**应用场景:**
* 在主线程之外创建并行线程,执行耗时的任务,避免阻塞用户界面。
* 适用于图像处理、视频编码、数据分析等任务。
**实现方式:**
* 创建一个JavaScript文件,作为Web Worker。
* 使用`postMessage()`方法与主线程通信。
* 使用`addEventListener()`监听主线程发来的消息。
### 代码示例
**HTML5离线存储(IndexedDB):**
```javascript
// 创建数据库
const request = indexedDB.open('myDatabase', 1);
// 数据库创建成功回调
request.onsuccess = function(event) {
const db = event.target.result;
// 创建对象存储
const objectStore = db.createObjectStore('myObjectStore', { keyPath: 'id' });
// 添加数据
objectStore.add({ id: 1, name: 'John' });
};
```
**Web Workers:**
```javascript
// 创建Web Worker
const worker = new Worker('myWorker.js');
// 发送消息到Web Worker
worker.postMessage({ message: 'Hello from main thread' });
// 监听Web Worker发来的消息
worker.addEventListener('message', function(event) {
console.log(event.data); // 输出Web Worker返回的消息
});
```
0
0
相关推荐
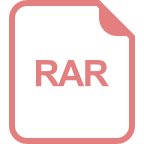







