Delphi连接SQL数据库:常见问题及解决方案,快速解决连接难题
发布时间: 2024-07-30 23:40:51 阅读量: 34 订阅数: 23 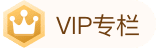
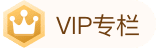

# 1. Delphi连接SQL数据库简介**
**1.1 Delphi与SQL数据库连接概述**
Delphi是一个强大的编程语言,它提供了连接和操作SQL数据库的功能。通过连接SQL数据库,Delphi应用程序可以访问和管理存储在数据库中的数据,从而实现各种业务需求。
**1.2 连接SQL数据库的必要步骤**
连接SQL数据库需要遵循以下步骤:
- 安装SQL数据库服务器软件。
- 创建数据库和用户。
- 在Delphi应用程序中添加数据库连接组件。
- 配置连接组件的参数,包括服务器地址、数据库名称、用户名和密码。
- 打开连接,执行SQL查询和更新操作。
# 2. 连接SQL数据库的理论基础
### 2.1 SQL数据库连接原理
#### 2.1.1 数据库连接的建立与关闭
数据库连接是客户端应用程序与数据库服务器之间建立的通信通道,用于数据交互。在Delphi中,建立数据库连接需要使用`TADOConnection`或`TIBDatabase`组件。
```delphi
// 使用TADOConnection组件建立连接
TADOConnection1.ConnectionString := 'Provider=SQLOLEDB.1;Data Source=localhost;Initial Catalog=AdventureWorks2019;User ID=sa;Password=your_password;';
TADOConnection1.Open();
// 使用TIBDatabase组件建立连接
TIBDatabase1.DatabaseName := 'AdventureWorks2019';
TIBDatabase1.UserName := 'sa';
TIBDatabase1.Password := 'your_password';
TIBDatabase1.Open();
```
数据库连接建立后,需要在使用完毕后关闭,释放资源。
```delphi
TADOConnection1.Close();
TIBDatabase1.Close();
```
#### 2.1.2 连接池的管理与优化
连接池是一种缓存机制,用于存储预先建立的数据库连接,以提高后续数据库操作的性能。连接池可以减少建立新连接的开销,从而提高应用程序的响应速度。
Delphi中使用`TADOConnectionPool`或`TIBDatabasePool`组件来管理连接池。
```delphi
// 使用TADOConnectionPool组件管理连接池
TADOConnectionPool1.ConnectionString := 'Provider=SQLOLEDB.1;Data Source=localhost;Initial Catalog=AdventureWorks2019;User ID=sa;Password=your_password;';
TADOConnectionPool1.MaxPoolSize := 10; // 设置连接池最大连接数
TADOConnectionPool1.Active := True; // 激活连接池
// 使用TIBDatabasePool组件管理连接池
TIBDatabasePool1.DatabaseName := 'AdventureWorks2019';
TIBDatabasePool1.UserName := 'sa';
TIBDatabasePool1.Password := 'your_password';
TIBDatabasePool1.MaxPoolSize := 10; // 设置连接池最大连接数
TIBDatabasePool1.Open(); // 打开连接池
```
### 2.2 Delphi连接SQL数据库的实现
#### 2.2.1 ADO组件的使用
ADO(ActiveX Data Objects)是微软提供的一组COM组件,用于访问和操作数据库。Delphi中可以使用`TADOConnection`、`TADOQuery`等ADO组件来连接和操作SQL数据库。
```delphi
// 使用TADOConnection组件连接数据库
TADOConnection1.ConnectionString := 'Provider=SQLOLEDB.1;Data Source=localhost;Initial Catalog=AdventureWorks2019;User ID=sa;Password=your_password;';
TADOConnection1.Open();
// 使用TADOQuery组件查询数据
TADOQuery1.Connection := TADOConnection1;
TADOQuery1.SQL.Text := 'SELECT * FROM Person.Contact';
TADOQuery1.Open();
```
#### 2.2.2 FireDAC组件的使用
FireDAC是Embarcadero提供的数据库访问组件库,支持多种数据库类型。Delphi中可以使用`TIBDatabase`、`TIBQuery`等FireDAC组件来连接和操作SQL数据库。
```delphi
// 使用TIBDatabase组件连接数据库
TIBDatabase1.DatabaseName := 'AdventureWorks2019';
TIBDatabase1.UserName := 'sa';
TIBDatabase1.Password := 'your_password';
TIBDatabase1.Open();
// 使用TIBQuery组件查询数据
TIBQuery1.Database := TIBDatabase1;
TIBQuery1.SQL.Text := 'SELECT * FROM Person.Contact';
TIBQuery1.Open();
```
# 3. 连接SQL数据库的实践应用
### 3.1 连接不同类型的SQL数据库
#### 3.1.1 MySQL数据库的连接
```delphi
procedure TForm1.btnConnectMySQLClick(Sender: TObject);
var
Conn: TMyConnection;
begin
Conn := TMyConnection.Create(nil);
try
Conn.Host := 'localhost';
Conn.Port := 3306;
Conn.User := 'root';
Conn.Password := '';
Conn.Database := 'test';
Conn.Connected := True;
ShowMessage('连接MySQL数据库成功');
finally
Conn.Free;
end;
end;
```
**代码逻辑分析:**
* 创建一个TMyConnection对象Conn。
* 设置连接参数:主机、端口、用户名、密码、数据库名。
* 调用Connected属性连接数据库。
* 显示连接成功信息。
**参数说明:**
| 参数 | 说明 |
|---|---|
| Host | 数据库服务器主机地址 |
| Port | 数据库服务器端口 |
| User | 数据库用户名 |
| Password | 数据库用户密码 |
| Database | 要连接的数据库名 |
#### 3.1.2 Oracle数据库的连接
```delphi
procedure TForm1.btnConnectOracleClick(Sender: TObject);
var
Conn: TOraConnection;
begin
Conn := TOraConnection.Create(nil);
try
Conn.ConnectionString := 'Data Source=XE;User Id=system;Password=oracle';
Conn.Connected := True;
ShowMessage('连接Oracle数据库成功');
finally
Conn.Free;
end;
end;
```
**代码逻辑分析:**
* 创建一个TOraConnection对象Conn。
* 使用ConnectionString属性设置连接字符串,包括数据源、用户名、密码。
* 调用Connected属性连接数据库。
* 显示连接成功信息。
**参数说明:**
| 参数 | 说明 |
|---|---|
| ConnectionString | 连接字符串,包含连接参数 |
| Data Source | 数据库服务名或SID |
| User Id | 数据库用户名 |
| Password | 数据库用户密码 |
#### 3.1.3 PostgreSQL数据库的连接
```delphi
procedure TForm1.btnConnectPostgreSQLClick(Sender: TObject);
var
Conn: TPostgreSQLConnection;
begin
Conn := TPostgreSQLConnection.Create(nil);
try
Conn.Host := 'localhost';
Conn.Port := 5432;
Conn.User := 'postgres';
Conn.Password := '';
Conn.Database := 'test';
Conn.Connected := True;
ShowMessage('连接PostgreSQL数据库成功');
finally
Conn.Free;
end;
end;
```
**代码逻辑分析:**
* 创建一个TPostgreSQLConnection对象Conn。
* 设置连接参数:主机、端口、用户名、密码、数据库名。
* 调用Connected属性连接数据库。
* 显示连接成功信息。
**参数说明:**
| 参数 | 说明 |
|---|---|
| Host | 数据库服务器主机地址 |
| Port | 数据库服务器端口 |
| User | 数据库用户名 |
| Password | 数据库用户密码 |
| Database | 要连接的数据库名 |
### 3.2 执行SQL查询和更新
#### 3.2.1 查询数据的获取
```delphi
procedure TForm1.btnQueryClick(Sender: TObject);
var
Conn: TMyConnection;
Cmd: TMyCommand;
Reader: TMyDataReader;
begin
Conn := TMyConnection.Create(nil);
try
Conn.Host := 'localhost';
Conn.Port := 3306;
Conn.User := 'root';
Conn.Password := '';
Conn.Database := 'test';
Conn.Connected := True;
Cmd := TMyCommand.Create(nil);
Cmd.Connection := Conn;
Cmd.CommandText := 'SELECT * FROM users';
Reader := Cmd.ExecuteReader;
while Reader.Read do
begin
ShowMessage(Reader.Fields[0].AsString);
end;
finally
Reader.Free;
Cmd.Free;
Conn.Free;
end;
end;
```
**代码逻辑分析:**
* 创建一个TMyConnection对象Conn并连接数据库。
* 创建一个TMyCommand对象Cmd并设置连接和SQL查询语句。
* 执行查询并获取TMyDataReader对象Reader。
* 循环读取Reader中的数据并显示。
**参数说明:**
| 参数 | 说明 |
|---|---|
| Connection | 命令所属的连接 |
| CommandText | 要执行的SQL查询语句 |
#### 3.2.2 数据的插入、更新和删除
```delphi
procedure TForm1.btnInsertClick(Sender: TObject);
var
Conn: TMyConnection;
Cmd: TMyCommand;
begin
Conn := TMyConnection.Create(nil);
try
Conn.Host := 'localhost';
Conn.Port := 3306;
Conn.User := 'root';
Conn.Password := '';
Conn.Database := 'test';
Conn.Connected := True;
Cmd := TMyCommand.Create(nil);
Cmd.Connection := Conn;
Cmd.CommandText := 'INSERT INTO users (name, age) VALUES (@name, @age)';
Cmd.Parameters.Add('name', ftString, 50, 'John');
Cmd.Parameters.Add('age', ftInteger, 0, 20);
Cmd.ExecuteNonQuery;
ShowMessage('插入数据成功');
finally
Cmd.Free;
Conn.Free;
end;
end;
```
**代码逻辑分析:**
* 创建一个TMyConnection对象Conn并连接数据库。
* 创建一个TMyCommand对象Cmd并设置连接和SQL插入语句。
* 添加参数并设置参数值。
* 执行插入操作并显示成功信息。
**参数说明:**
| 参数 | 说明 |
|---|---|
| Connection | 命令所属的连接 |
| CommandText | 要执行的SQL插入、更新或删除语句 |
| Parameters | 命令的参数集合 |
| Name | 参数名称 |
| DataType | 参数数据类型 |
| Size | 参数大小 |
| Value | 参数值 |
# 4. 连接SQL数据库的常见问题及解决方案
### 4.1 连接失败问题
#### 4.1.1 数据库服务器不可用
**问题描述:**
尝试连接数据库时,出现连接失败错误,提示数据库服务器不可用。
**解决方案:**
- 确保数据库服务器正在运行。
- 检查数据库服务器的网络连接是否正常。
- 检查数据库服务器的防火墙设置,确保允许来自客户端的连接。
#### 4.1.2 连接参数错误
**问题描述:**
连接参数配置错误,导致连接失败。
**解决方案:**
- 检查连接字符串是否正确,包括数据库服务器地址、端口、数据库名称、用户名和密码。
- 确保连接字符串中使用的数据库服务器名称或IP地址是正确的。
- 确保连接字符串中使用的端口号是数据库服务器正在监听的端口。
- 确保连接字符串中使用的数据库名称是存在的。
- 确保连接字符串中使用的用户名和密码是正确的。
#### 4.1.3 防火墙或网络限制
**问题描述:**
防火墙或网络限制阻止了客户端与数据库服务器之间的连接。
**解决方案:**
- 检查客户端和数据库服务器之间的防火墙设置,确保允许来自客户端的连接。
- 检查客户端和数据库服务器之间的网络连接是否正常。
- 尝试使用其他网络端口进行连接,以排除端口被阻止的可能性。
### 4.2 查询和更新失败问题
#### 4.2.1 SQL语法错误
**问题描述:**
执行SQL查询或更新语句时,出现语法错误。
**解决方案:**
- 检查SQL语句的语法,确保符合SQL标准。
- 检查SQL语句中使用的表名、字段名和关键字是否正确。
- 检查SQL语句中使用的引号和括号是否成对出现。
#### 4.2.2 数据类型不匹配
**问题描述:**
尝试插入或更新数据时,出现数据类型不匹配错误。
**解决方案:**
- 检查插入或更新的数据类型是否与表中的字段类型相匹配。
- 确保插入或更新的数据符合字段的长度和精度限制。
#### 4.2.3 权限不足
**问题描述:**
尝试执行查询或更新操作时,出现权限不足错误。
**解决方案:**
- 确保当前用户拥有执行该操作所需的权限。
- 检查数据库服务器的权限设置,确保当前用户具有访问该表或执行该操作的权限。
# 5. 连接SQL数据库的性能优化
### 5.1 连接池的优化
#### 5.1.1 连接池大小的设置
连接池大小是连接池中同时可用的连接数。连接池大小的设置需要根据应用程序的并发连接数和数据库服务器的负载情况进行调整。
**参数说明:**
- `MinPoolSize`:连接池的最小连接数。
- `MaxPoolSize`:连接池的最大连接数。
**代码块:**
```delphi
// 设置连接池大小
adoConnection.ConnectionPool.MinPoolSize := 10;
adoConnection.ConnectionPool.MaxPoolSize := 100;
```
**逻辑分析:**
这段代码设置了连接池的最小连接数为10,最大连接数为100。当应用程序需要连接数据库时,如果连接池中没有可用的连接,则会自动创建新的连接。当连接池中的连接数达到MaxPoolSize时,新的连接请求将被放入队列中等待。
#### 5.1.2 连接池的超时管理
连接池超时管理是指设置连接池中连接的超时时间。当连接池中的连接空闲时间超过超时时间后,该连接将被关闭并从连接池中移除。
**参数说明:**
- `ConnectionLifetime`:连接的超时时间,单位为秒。
**代码块:**
```delphi
// 设置连接池超时时间
adoConnection.ConnectionPool.ConnectionLifetime := 600;
```
**逻辑分析:**
这段代码设置了连接池中连接的超时时间为600秒。当连接池中的连接空闲时间超过600秒后,该连接将被关闭并从连接池中移除。
### 5.2 查询和更新的优化
#### 5.2.1 索引的使用
索引是一种数据结构,它可以快速查找数据库中的特定记录。使用索引可以显著提高查询和更新的性能。
**代码块:**
```sql
CREATE INDEX idx_name ON table_name (column_name);
```
**逻辑分析:**
这段SQL语句在`table_name`表上创建了一个名为`idx_name`的索引,该索引基于`column_name`列。当查询或更新操作涉及到`column_name`列时,数据库将使用该索引来快速查找记录。
#### 5.2.2 SQL语句的优化
SQL语句的优化是指通过调整SQL语句的结构和语法来提高其执行效率。以下是一些常见的SQL语句优化技巧:
- 使用适当的连接类型(INNER JOIN、LEFT JOIN、RIGHT JOIN)。
- 避免使用子查询。
- 使用UNION ALL代替UNION。
- 使用LIMIT子句限制返回记录数。
**代码块:**
```sql
SELECT * FROM table_name WHERE column_name = 'value' LIMIT 10;
```
**逻辑分析:**
这段SQL语句从`table_name`表中查询`column_name`列等于`value`的所有记录,并限制返回记录数为10。通过使用LIMIT子句,可以提高查询性能,因为数据库只需要扫描表中的前10条记录即可。
# 6.1 分布式数据库连接
### 6.1.1 分布式数据库的概念
分布式数据库是一种将数据存储在多个物理位置的数据库系统,这些位置可能位于不同的计算机、服务器甚至不同的地理位置。分布式数据库的目的是提高数据可用性、可扩展性和性能。
### 6.1.2 Delphi连接分布式数据库的实现
Delphi可以使用FireDAC组件连接分布式数据库。FireDAC提供了一个统一的接口,允许开发人员使用相同的代码连接到各种数据库,包括分布式数据库。
连接分布式数据库时,需要指定以下信息:
- **连接字符串:**包含连接到数据库所需的信息,例如服务器名称、端口、用户名和密码。
- **分布式连接属性:**指定分布式数据库的特定设置,例如数据源名称(DSN)或连接池大小。
以下代码示例演示如何使用FireDAC连接分布式数据库:
```delphi
// 创建一个新的FireDAC连接
FDConnection1 := TFDConnection.Create(nil);
// 设置连接字符串
FDConnection1.ConnectionString := 'DriverID=Firebird;Database=C:\path\to\database.fdb;Server=192.168.1.100;Port=3050;User=SYSDBA;Password=masterkey';
// 设置分布式连接属性
FDConnection1.DistributedConnectionProperties.DSN := 'MyDSN';
FDConnection1.DistributedConnectionProperties.Pooling := True;
// 打开连接
FDConnection1.Open;
```
连接到分布式数据库后,可以使用相同的FireDAC组件执行查询和更新操作。
0
0
相关推荐
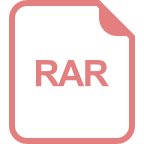
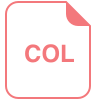
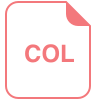
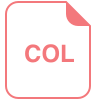
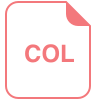

