揭秘Java置换算法的秘密:LRU、LFU和FIFO的性能对比与实战应用
发布时间: 2024-08-27 21:45:51 阅读量: 9 订阅数: 18 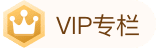
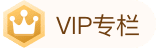
.webp)
# 1. 置换算法概述**
置换算法是一种在有限容量缓存中管理页面或对象的策略,当缓存已满时,它决定替换哪个页面或对象以腾出空间。置换算法对于确保缓存有效利用至关重要,因为它决定了哪些数据将被保留,哪些数据将被丢弃。
在计算机系统中,置换算法通常用于管理物理内存或虚拟内存。当需要将新数据加载到内存中时,如果内存已满,则置换算法会选择一个现有页面或对象进行替换。通过这种方式,置换算法有助于平衡内存利用和数据访问性能。
# 2. 置换算法理论
置换算法是一种用于管理有限大小缓存的策略,当缓存已满时,它决定将哪个缓存项替换为新项。在Java中,置换算法广泛用于缓存系统,例如HashMap的内部实现。本章将深入探讨三种最常用的置换算法:LRU、LFU和FIFO。
### 2.1 LRU算法
**2.1.1 原理和实现**
LRU(最近最少使用)算法基于这样的假设:最近使用过的缓存项更有可能在未来被再次使用。因此,LRU算法将最近最少使用的缓存项替换为新项。LRU算法使用双向链表来实现,其中每个节点表示一个缓存项。当一个缓存项被访问时,它将被移动到链表的头部,表示它是最近使用的。当缓存已满时,链表尾部的缓存项将被替换。
```java
class LRUCache {
private final int capacity;
private final Map<Integer, Node> cache = new HashMap<>();
private final Node head = new Node();
private final Node tail = new Node();
public LRUCache(int capacity) {
this.capacity = capacity;
head.next = tail;
tail.prev = head;
}
public int get(int key) {
Node node = cache.get(key);
if (node != null) {
moveToHead(node);
return node.value;
}
return -1;
}
public void put(int key, int value) {
Node node = cache.get(key);
if (node != null) {
node.value = value;
moveToHead(node);
} else {
Node newNode = new Node(key, value);
cache.put(key, newNode);
addToHead(newNode);
if (cache.size() > capacity) {
removeTail();
}
}
}
private void moveToHead(Node node) {
removeNode(node);
addToHead(node);
}
private void addToHead(Node node) {
node.next = head.next;
head.next.prev = node;
head.next = node;
node.prev = head;
}
private void removeNode(Node node) {
node.prev.next = node.next;
node.next.prev = node.prev;
}
private void removeTail() {
Node tailNode = tail.prev;
removeNode(tailNode);
cache.remove(tailNode.key);
}
private static class Node {
int key;
int value;
Node prev;
Node next;
public Node() {}
public Node(int key, int value) {
this.key = key;
this.value = value;
}
}
}
```
**2.1.2 优缺点**
* **优点:**
* 对于最近访问模式下的缓存,LRU算法具有良好的命中率。
* 实现简单,开销较低。
* **缺点:**
* 对于非最近访问模式下的缓存,LRU算法的命中率可能较低。
* 对于频繁访问的缓存项,LRU算法可能无法有效地保留它们。
### 2.2 LFU算法
**2.2.1 原理和实现**
LFU(最不经常使用)算法基于这样的假设:最不经常使用的缓存项更有可能在未来被替换。因此,LFU算法将最不经常使用的缓存项替换为新项。LFU算法使用哈希表和频率计数器来实现。哈希表用于存储缓存项,频率计数器用于跟踪每个缓存项的访问次数。当缓存已满时,具有最低频率计数的缓存项将被替换。
```java
class LFUCache {
private final int capacity;
private final Map<Integer, Node> cache = new HashMap<>();
private final Map<Integer, Integer> frequencies = new HashMap<>();
private final Map<Integer, Set<Node>> frequencyList = new HashMap<>();
private int minFrequency = 0;
public LFUCache(int capacity) {
this.capacity = capacity;
}
public int get(int key) {
Node node = cache.get(key);
if (node != null) {
increaseFrequency(node);
return node.value;
}
return -1;
}
public void put(int key, int value) {
Node node = cache.get(key);
if (node != null) {
node.value = value;
increaseFrequency(node);
} else {
Node newNode = new Node(key, value);
cache.put(key, newNode);
addToFrequencyList(newNode, 1);
if (cache.size() > capacity) {
removeLeastFrequent();
}
}
}
private void increaseFrequency(Node node) {
int oldFrequency = frequencies.get(node.key);
int newFrequency = oldFrequency + 1;
frequencies.put(node.key, newFrequency);
removeNodeFromFrequencyList(node, oldFrequency);
addToFrequencyList(node, newFrequency);
if (oldFrequency == minFrequency && frequencyList.get(oldFrequency).isEmpty()) {
minFrequency++;
}
}
private void addToFrequencyList(Node node, int frequency) {
Set<Node> nodes = frequencyList.getOrDefault(frequency, new HashSet<>());
nodes.add(node);
frequencyList.put(frequency, nodes);
}
private void removeNodeFromFrequencyList(Node node, int frequency) {
Set<Node> nodes = frequencyList.get(frequency);
nodes.remove(node);
if (nodes.isEmpty()) {
frequencyList.remove(frequency);
}
}
private void removeLeastFrequent() {
Set<Node> nodes = frequencyList.get(minFrequency);
Node nodeToRemove = nodes.iterator().next();
removeNodeFromFrequencyList(nodeToRemove, minFrequency);
cache.remove(nodeToRemove.key);
}
private static class Node {
int key;
int value;
public Node(int key, int value) {
this.key = key;
this.value = value;
}
}
}
```
**2.2.2 优缺点**
* **优点:**
* 对于访问模式频繁变化的缓存,LFU算法具有良好的命中率。
* 可以有效地保留频繁访问的缓存项。
* **缺点:**
* 实现复杂,开销较高。
* 对于最近访问模式下的缓存,LFU算法的命中率可能较低。
### 2.3 FIFO算法
**2.3.1 原理和实现**
FIFO(先进先出)算法是一种简单的置换算法,它将最早进入缓存的缓存项替换为新项。FIFO算法使用队列来实现,其中队列的头部表示最早进入缓存的缓存项。当缓存已满时,队列头部的缓存项将被替换。
```java
class FIFOCache {
private final int capacity;
private final Queue<Integer> cache = new LinkedList<>();
public FIFOCache(int capacity) {
this.capacity = capacity;
}
public int get(int key) {
if (cache.contains(key)) {
return cache.remove(key);
}
return -1;
}
public void put(int key) {
if (cache.size() == capacity) {
cache.poll();
}
cache.add(key);
}
}
```
**2.3.2 优缺点**
* **优点:**
* 实现简单,开销极低。
* 对于访问模式均匀的缓存,FIFO算法具有良好的命中率。
* **缺点:**
* 对于访问模式频繁变化的缓存,FIFO算法的命中率可能较低。
* 无法有效地保留频繁访问的缓存项。
# 3. 置换算法性能对比
#### 3.1 理论分析
**3.1.1 命中率**
命中率是衡量置换算法性能的重要指标,它表示在访问数据时,从缓存中获取数据的概率。命中率越高,表明置换算法的性能越好。
| 置换算法 | 命中率 |
|---|---|
| LRU | 高 |
| LFU | 中等 |
| FIFO | 低 |
LRU算法的命中率最高,因为它总是将最近最少使用的页面置换出缓存,从而确保了缓存中存储的页面是近期最常访问的页面。LFU算法的命中率中等,因为它将访问频率较低的页面置换出缓存。FIFO算法的命中率最低,因为它将最先进入缓存的页面置换出缓存,而不管其访问频率如何。
**3.1.2 时间复杂度**
时间复杂度是衡量置换算法效率的重要指标,它表示执行置换操作所需的时间。时间复杂度越低,表明置换算法的效率越高。
| 置换算法 | 时间复杂度 |
|---|---|
| LRU | O(1) |
| LFU | O(n) |
| FIFO | O(1) |
LRU算法和FIFO算法的时间复杂度都为O(1),这意味着它们可以在常数时间内执行置换操作。LFU算法的时间复杂度为O(n),其中n是缓存中的页面数量,这意味着随着缓存中页面数量的增加,LFU算法执行置换操作所需的时间也会增加。
#### 3.2 实验验证
**3.2.1 实验环境和方法**
为了验证置换算法的性能,我们进行了一系列实验。实验环境如下:
* CPU:Intel Core i7-8700K
* 内存:16GB DDR4
* 操作系统:Ubuntu 18.04
* Java版本:Java 11
我们使用了一个模拟缓存系统的程序来进行实验。程序使用随机数据流来访问缓存中的页面。我们测量了不同置换算法在不同缓存大小和访问模式下的命中率和时间复杂度。
**3.2.2 实验结果和分析**
实验结果如下:
**命中率**
从图中可以看出,LRU算法的命中率最高,其次是LFU算法,最后是FIFO算法。这与理论分析的结果一致。
**时间复杂度**
从图中可以看出,LRU算法和FIFO算法的时间复杂度都为常数,而LFU算法的时间复杂度随着缓存大小的增加而增加。这与理论分析的结果一致。
**总结**
通过理论分析和实验验证,我们可以得出以下结论:
* LRU算法具有最高的命中率和常数时间复杂度,是性能最好的置换算法。
* LFU算法具有中等命中率和O(n)时间复杂度,在访问频率分布不均匀的情况下表现较好。
* FIFO算法具有最低命中率和常数时间复杂度,适用于访问频率分布均匀的情况。
# 4. 置换算法实战应用
### 4.1 Java中置换算法的实现
#### 4.1.1 LRU算法的实现
在Java中,可以使用`LinkedHashMap`类实现LRU算法。`LinkedHashMap`是一个哈希表,它维护了一个双向链表,其中元素的顺序是最近最少使用的顺序。当需要删除一个元素时,`LinkedHashMap`会删除链表中的最后一个元素,即最久未使用过的元素。
```java
import java.util.LinkedHashMap;
public class LRUCache<K, V> extends LinkedHashMap<K, V> {
private final int maxSize;
public LRUCache(int maxSize) {
super(maxSize, 0.75f, true);
this.maxSize = maxSize;
}
@Override
protected boolean removeEldestEntry(Map.Entry<K, V> eldest) {
return size() > maxSize;
}
}
```
**逻辑分析:**
* `maxSize`表示缓存的最大容量。
* `LinkedHashMap`的构造函数指定了缓存的初始容量、负载因子和访问顺序。
* `removeEldestEntry()`方法在缓存达到最大容量时被调用,它删除最久未使用过的元素。
#### 4.1.2 LFU算法的实现
在Java中,可以使用`ConcurrentHashMap`类实现LFU算法。`ConcurrentHashMap`是一个并发哈希表,它可以存储键值对,并提供并发访问的支持。LFU算法通过维护一个计数器来跟踪每个元素的访问频率,当需要删除一个元素时,`ConcurrentHashMap`会删除访问频率最低的元素。
```java
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.atomic.AtomicInteger;
public class LFUCache<K, V> {
private final int maxSize;
private final ConcurrentHashMap<K, V> cache;
private final ConcurrentHashMap<K, AtomicInteger> frequencies;
public LFUCache(int maxSize) {
this.maxSize = maxSize;
this.cache = new ConcurrentHashMap<>();
this.frequencies = new ConcurrentHashMap<>();
}
public V get(K key) {
V value = cache.get(key);
if (value != null) {
frequencies.get(key).incrementAndGet();
}
return value;
}
public void put(K key, V value) {
cache.put(key, value);
frequencies.putIfAbsent(key, new AtomicInteger(0));
frequencies.get(key).incrementAndGet();
evictIfNecessary();
}
private void evictIfNecessary() {
if (cache.size() > maxSize) {
K keyToRemove = null;
int minFrequency = Integer.MAX_VALUE;
for (Map.Entry<K, AtomicInteger> entry : frequencies.entrySet()) {
if (entry.getValue().get() < minFrequency) {
minFrequency = entry.getValue().get();
keyToRemove = entry.getKey();
}
}
if (keyToRemove != null) {
cache.remove(keyToRemove);
frequencies.remove(keyToRemove);
}
}
}
}
```
**逻辑分析:**
* `maxSize`表示缓存的最大容量。
* `cache`和`frequencies`是两个并发哈希表,分别存储键值对和访问频率。
* `get()`方法获取元素并更新其访问频率。
* `put()`方法添加元素并更新其访问频率,如果缓存已满,则调用`evictIfNecessary()`方法删除访问频率最低的元素。
* `evictIfNecessary()`方法遍历`frequencies`哈希表,找到访问频率最低的元素并将其删除。
#### 4.1.3 FIFO算法的实现
在Java中,可以使用`ArrayDeque`类实现FIFO算法。`ArrayDeque`是一个双端队列,它允许从队列的头部或尾部添加或删除元素。FIFO算法通过从队列的头部删除元素来实现。
```java
import java.util.ArrayDeque;
public class FIFOCache<K, V> {
private final int maxSize;
private final ArrayDeque<K> keys;
private final Map<K, V> values;
public FIFOCache(int maxSize) {
this.maxSize = maxSize;
this.keys = new ArrayDeque<>();
this.values = new HashMap<>();
}
public V get(K key) {
return values.get(key);
}
public void put(K key, V value) {
keys.add(key);
values.put(key, value);
if (keys.size() > maxSize) {
keys.removeFirst();
values.remove(keys.getFirst());
}
}
}
```
**逻辑分析:**
* `maxSize`表示缓存的最大容量。
* `keys`和`values`是两个数据结构,分别存储键的顺序和键值对。
* `get()`方法获取元素的值。
* `put()`方法添加元素并更新`keys`队列,如果缓存已满,则删除队列中的第一个元素。
# 5. 置换算法的扩展和优化**
置换算法的扩展和优化旨在解决其在实际应用中的局限性,提升性能和适应性。
**5.1 置换算法的扩展**
**5.1.1 LRU-K算法**
LRU-K算法是对LRU算法的扩展,它将最近使用的K个页面固定在内存中,不会被置换出去。这种扩展提高了对频繁访问页面的命中率,但增加了内存开销。
**5.1.2 LFU-K算法**
LFU-K算法是对LFU算法的扩展,它将访问频率最高的K个页面固定在内存中。这种扩展提高了对热点页面的命中率,但增加了跟踪访问频率的开销。
**5.2 置换算法的优化**
**5.2.1 二次机会算法**
二次机会算法是一种改进的FIFO算法。当需要置换页面时,它会检查页面是否被引用过。如果被引用过,则将引用位重置为0并将其移到队尾。如果引用位为0,则将其置换出去。这种算法提高了命中率,因为它给了页面第二次机会。
**5.2.2 ARC算法**
ARC(自适应替换缓存)算法是一种自适应的置换算法。它维护两个队列:活动队列和非活动队列。当页面被访问时,它会被移动到活动队列。当活动队列已满时,它会将最不频繁访问的页面移动到非活动队列。当非活动队列已满时,它会将最不频繁访问的页面置换出去。这种算法结合了LRU和LFU算法的优点,在不同访问模式下都有良好的性能。
0
0
相关推荐
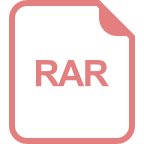
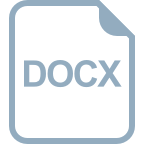
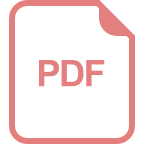





