Java置换算法的实战指南:LRU、LFU和FIFO的应用与优化技巧
发布时间: 2024-08-27 21:47:58 阅读量: 7 订阅数: 18 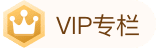
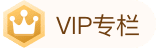
.webp)
# 1. Java置换算法概述
置换算法是一种用于管理有限资源(例如内存或缓存)的算法,当新数据需要存储时,它决定哪些现有数据应该被替换。置换算法对于优化系统性能至关重要,因为它们可以防止资源耗尽,从而导致系统崩溃或性能下降。
Java中常见的置换算法包括LRU(最近最少使用)、LFU(最近最少使用)和FIFO(先进先出)。这些算法使用不同的策略来确定哪些数据应该被替换,例如跟踪最近使用时间或使用频率。选择合适的置换算法取决于应用程序的特定需求和资源约束。
# 2. LRU置换算法
### 2.1 LRU算法原理和实现
#### 2.1.1 LRU算法的工作原理
LRU(最近最少使用)算法是一种页面置换算法,它通过跟踪每个页面的使用情况来决定替换哪个页面。LRU算法的基本原理是,最近最少使用的页面是最有可能被替换的。
LRU算法使用一个队列来跟踪页面的使用情况。当一个页面被访问时,它会被移动到队列的头部。当需要替换一个页面时,队列尾部的页面将被替换。
#### 2.1.2 LRU算法的Java实现
```java
import java.util.HashMap;
import java.util.LinkedList;
public class LRUCache<K, V> {
private final int capacity;
private final HashMap<K, Node<K, V>> cache;
private final LinkedList<Node<K, V>> queue;
public LRUCache(int capacity) {
this.capacity = capacity;
this.cache = new HashMap<>();
this.queue = new LinkedList<>();
}
public V get(K key) {
Node<K, V> node = cache.get(key);
if (node == null) {
return null;
}
queue.remove(node);
queue.addFirst(node);
return node.value;
}
public void put(K key, V value) {
Node<K, V> node = cache.get(key);
if (node == null) {
node = new Node<>(key, value);
cache.put(key, node);
queue.addFirst(node);
if (cache.size() > capacity) {
Node<K, V> lastNode = queue.removeLast();
cache.remove(lastNode.key);
}
} else {
node.value = value;
queue.remove(node);
queue.addFirst(node);
}
}
private static class Node<K, V> {
K key;
V value;
Node<K, V> prev;
Node<K, V> next;
public Node(K key, V value) {
this.key = key;
this.value = value;
}
}
}
```
**代码逻辑分析:**
* `get`方法:如果缓存中存在该键,则返回该值并将其移动到队列的头部。如果缓存中不存在该键,则返回`null`。
* `put`方法:如果缓存中存在该键,则更新该值并将其移动到队列的头部。如果缓存中不存在该键,则创建新节点并将其添加到队列的头部。如果缓存已满,则删除队列尾部的节点并从缓存中删除该键。
### 2.2 LRU算法的优化技巧
#### 2.2.1 LRU算法的变种
* **LFU(最近最不经常使用)算法:**LFU算法跟踪每个页面的访问频率,而不是最近使用时间。当需要替换一个页面时,访问频率最低的页面将被替换。
* **LRU-K算法:**LRU-K算法将页面分为多个组,每个组使用自己的LRU队列。当需要替换一个页面时,从访问频率最低的组中选择一个页面进行替换。
#### 2.2.2 LRU算法的性能优化
* **使用哈希表:**使用哈希表来快速查找页面,可以减少搜索时间。
* **使用双向链表:**使用双向链表来存储页面,可以快速移动页面到队列的头部或尾部。
* **调整容量:**根据实际使用情况调整LRU缓存的容量,可以提高性能。
# 3.1 LFU算法原理和实现
#### 3.1.1 LFU算法的工作原理
LFU(Least Frequently Used)算法是一种基于频率的置换算法,它会跟踪每个页面被访问的频率,并淘汰访问频率最低的页面。LFU算法认为,访问频率较低的页面将来被访问的可能性也较低,因此可以优先淘汰它们。
LFU算法使用一个计数器来记录每个页面的访问频率。当页面被访问时,其计数器就会增加。当需要淘汰页面时,LFU算法会选择计数器值最小的页面进行淘汰。
#### 3.1.2 LFU算法的Java实现
```java
import java.util.HashMap;
import java.util.Map;
public class LFUCache {
private final int capacity;
private Map<Integer, Integer> keyToValue;
private Map<Integer, Integer> keyToCount;
private Map<Integer, LinkedHashSet<Integer>> countToKeys;
private int minCount;
public LFUCache(int capacity) {
this.capacity = capacity;
keyToValue = new HashMap<>();
keyToCount = new HashMap<>();
countToKeys = new HashMap<>();
minCount = 0;
}
public int get(int key) {
if (!keyToValue.containsKey(key)) {
return -1;
}
int count = keyToCount.get(key);
keyToCount.put(key, count + 1);
countToKeys.get(count).remove(key);
if (countToKeys.get(count).isEmpty()) {
countToKeys.remove(count);
}
if (count + 1 > minCount) {
minCount = count + 1;
}
countToKeys.computeIfAbsent(count + 1, k -> new LinkedHashSet<>()).add(key);
return keyToValue.get(key);
}
public void put(int key, int value) {
if (capacity <= 0) {
return;
}
if (keyToValue.containsKey(key)) {
keyToValue.put(key, value);
get(key);
return;
}
if (keyToValue.size() >= capacity) {
int keyToRemove = countToKeys.get(minCount).iterator().next();
countToKeys.get(minCount).remove(keyToRemove);
if (countToKeys.get(minCount).isEmpty()) {
countToKeys.remove(minCount);
}
keyToValue.remove(keyToRemove);
keyToCount.remove(keyToRemove);
}
keyToValue.put(key, value);
keyToCount.put(key, 1);
countToKeys.computeIfAbsent(1, k -> new LinkedHashSet<>()).add(key);
minCount = 1;
}
}
```
**代码逻辑逐行解读:**
1. 构造函数初始化缓存容量、键值对映射、键计数映射和计数键映射。
2. `get` 方法:获取键对应的值,并更新其访问频率。
3. `put` 方法:如果键已存在,更新其值和访问频率;如果不存在,则添加键值对,并淘汰访问频率最低的键值对。
**参数说明:**
* `capacity`:缓存容量。
* `keyToValue`:键值对映射。
* `keyToCount`:键计数映射。
* `countToKeys`:计数键映射。
* `minCount`:最小访问频率。
# 4. FIFO置换算法
### 4.1 FIFO算法原理和实现
#### 4.1.1 FIFO算法的工作原理
FIFO(First In First Out)算法是一种页面置换算法,它遵循先进先出的原则。当需要置换一个页面时,FIFO算法会选择最先进入内存的页面进行置换。
#### 4.1.2 FIFO算法的Java实现
```java
import java.util.LinkedList;
import java.util.Queue;
public class FIFOPageReplacementAlgorithm {
private Queue<Integer> pageQueue;
private int pageSize;
public FIFOPageReplacementAlgorithm(int pageSize) {
this.pageQueue = new LinkedList<>();
this.pageSize = pageSize;
}
public void addPage(int page) {
if (pageQueue.size() == pageSize) {
pageQueue.poll();
}
pageQueue.offer(page);
}
public int getPageToReplace() {
return pageQueue.poll();
}
}
```
### 4.2 FIFO算法的优化技巧
#### 4.2.1 FIFO算法的变种
* **LFU-FIFO算法:**结合LFU算法,在FIFO算法的基础上,将最近最少使用的页面置换出去。
* **Clock算法:**使用一个指针扫描页面,当需要置换页面时,指针指向的页面会被置换出去,但如果该页面被引用过,则指针会向前移动,继续扫描。
#### 4.2.2 FIFO算法的性能优化
* **增加页面大小:**增加页面大小可以减少页面置换的频率,从而提高性能。
* **使用二级缓存:**在内存中使用二级缓存,将最近使用的页面存储在二级缓存中,可以减少对主内存的访问,从而提高性能。
* **使用预取技术:**预取技术可以提前将即将被访问的页面加载到内存中,从而减少页面置换的开销,提高性能。
# 5. 置换算法的应用场景
置换算法在计算机系统中有着广泛的应用,主要应用于以下几个方面:
### 5.1 缓存系统
缓存系统是计算机系统中用于存储经常访问的数据,以提高系统性能。置换算法用于决定当缓存已满时,需要替换哪些数据块。常用的置换算法包括 LRU、LFU 和 FIFO。
### 5.2 内存管理
在内存管理中,置换算法用于决定当物理内存已满时,需要替换哪些页面到磁盘。常用的置换算法包括 LRU、LFU 和 FIFO。
### 5.3 操作系统调度
在操作系统调度中,置换算法用于决定当 CPU 已满时,需要暂停哪些进程。常用的置换算法包括 LRU、LFU 和 FIFO。
#### 5.3.1 LRU 在操作系统调度中的应用
在操作系统调度中,LRU 算法可以用于决定当 CPU 已满时,需要暂停哪些进程。LRU 算法会跟踪每个进程最近一次被使用的信息,并优先暂停最近最少使用的进程。
```java
import java.util.HashMap;
import java.util.LinkedList;
public class LRUScheduler {
private HashMap<Integer, Integer> processUsage;
private LinkedList<Integer> processQueue;
private int maxProcesses;
public LRUScheduler(int maxProcesses) {
this.processUsage = new HashMap<>();
this.processQueue = new LinkedList<>();
this.maxProcesses = maxProcesses;
}
public void addProcess(int processId) {
if (processUsage.containsKey(processId)) {
processUsage.put(processId, processUsage.get(processId) + 1);
} else {
processUsage.put(processId, 1);
processQueue.addFirst(processId);
}
if (processQueue.size() > maxProcesses) {
int leastUsedProcess = processQueue.removeLast();
processUsage.remove(leastUsedProcess);
}
}
public int getLeastUsedProcess() {
return processQueue.getLast();
}
}
```
**代码逻辑分析:**
* `addProcess` 方法用于添加一个进程到调度器中。
* 如果进程已经在调度器中,则更新其使用次数。
* 如果进程不在调度器中,则将其添加到调度器中,并将其添加到队列的头部。
* 如果队列中的进程数量超过最大进程数量,则从队列的尾部移除使用次数最少的进程。
* `getLeastUsedProcess` 方法返回队列中使用次数最少的进程。
**参数说明:**
* `maxProcesses`:调度器可以同时处理的最大进程数量。
# 6. 置换算法的性能评估
### 6.1 性能指标
评估置换算法的性能通常使用以下指标:
- **命中率:**命中率是指在查找数据时,在缓存中找到所需数据的概率。命中率越高,表明算法的性能越好。
- **缺失率:**缺失率是命中率的相反,表示在查找数据时,在缓存中找不到所需数据的概率。
- **平均访问时间:**平均访问时间是指查找数据所需的平均时间。它包括命中和缺失两种情况下的时间。
- **空间开销:**空间开销是指算法所需的内存空间大小。
### 6.2 性能测试方法
测试置换算法的性能可以使用以下方法:
1. **合成基准测试:**使用人工生成的数据集来测试算法的性能。
2. **真实基准测试:**使用真实世界的应用程序数据来测试算法的性能。
3. **模拟:**使用模拟器来模拟算法在不同场景下的性能。
### 6.3 不同算法的性能对比
不同置换算法的性能表现取决于具体应用场景和数据特征。一般来说:
- **LRU算法:**LRU算法在命中率方面表现较好,但在空间开销方面较高。
- **LFU算法:**LFU算法在空间开销方面较低,但在命中率方面不如LRU算法。
- **FIFO算法:**FIFO算法的命中率和空间开销都较低,但实现简单。
在实际应用中,需要根据具体场景选择合适的置换算法。例如,在缓存系统中,命中率是关键指标,因此通常使用LRU算法。而在内存管理中,空间开销是关键指标,因此通常使用LFU算法。
0
0
相关推荐
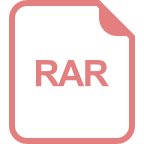
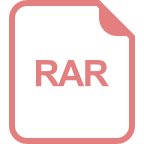
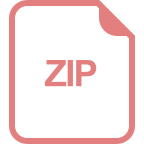





