MATLAB 2014a 对象导向编程实战:面向对象设计与实现,对象导向编程全攻略
发布时间: 2024-06-14 03:59:31 阅读量: 70 订阅数: 29 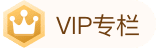
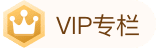
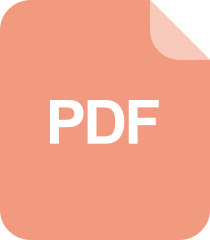
matlab 面向对象编程

# 1. 面向对象编程(OOP)基础**
面向对象编程(OOP)是一种编程范式,它将程序组织成称为对象的抽象数据类型。对象包含数据(称为属性)和操作数据的方法。OOP 的核心概念包括:
* **封装:**将数据和方法捆绑在一起,形成一个单一的实体。
* **继承:**允许子类从父类继承属性和方法。
* **多态性:**允许对象以不同的方式响应相同的消息,具体取决于它们的类型。
# 2. MATLAB OOP 的理论基础**
**2.1 对象、类和继承**
**对象**
对象是现实世界实体的抽象表示。它封装了数据(属性)和操作(方法)的集合。对象具有状态,它可以被查询或修改。
**类**
类是对象的蓝图,它定义了对象的属性和方法。类提供了一种创建具有相同属性和行为的对象的方法。
**继承**
继承允许一个类(派生类)从另一个类(基类)继承属性和方法。派生类可以重写基类的方法,以提供不同的行为。
**代码块:**
```
class Person
properties
name
age
end
methods
function obj = Person(name, age)
obj.name = name;
obj.age = age;
end
function greet(obj)
disp(['Hello, my name is ', obj.name, ' and I am ', num2str(obj.age), ' years old.']);
end
end
end
class Student < Person
properties
studentId
end
methods
function obj = Student(name, age, studentId)
obj = obj@Person(name, age); % Call the constructor of the base class
obj.studentId = studentId;
end
function greet(obj)
disp(['Hello, my name is ', obj.name, ' and I am ', num2str(obj.age), ' years old. My student ID is ', num2str(obj.studentId), '.']);
end
end
end
```
**逻辑分析:**
* `Person` 类定义了一个具有 `name` 和 `age` 属性和一个 `greet` 方法的对象。
* `Student` 类从 `Person` 类继承,并添加了 `studentId` 属性。
* `Student` 类重写了 `greet` 方法,以显示额外的学生 ID 信息。
**参数说明:**
* `Person` 构造函数:`name`(字符串)、`age`(数字)
* `Student` 构造函数:`name`(字符串)、`age`(数字)、`studentId`(数字)
* `greet` 方法:无参数
**2.2 多态性和抽象类**
**多态性**
多态性允许对象以不同的方式响应相同的操作,具体取决于它们的类型。这使得代码更灵活,更易于维护。
**抽象类**
抽象类是不能被实例化的类。它们用于定义公共接口,而派生类实现该接口。抽象类可以包含抽象方法,这些方法必须在派生类中实现。
**代码块:**
```
abstract class Shape
properties
color
end
methods (Abstract)
area()
perimeter()
end
end
class Rectangle < Shape
properties
width
height
end
methods
function obj = Rectangle(color, width, height)
obj = obj@Shape(color);
obj.width = width;
obj.height = height;
end
function a = area(obj)
a = obj.width * obj.height;
end
function p = perimeter(obj)
p = 2 * (obj.width + obj.height);
end
end
class Circle < Shape
properties
radius
end
methods
function obj = Circle(color, radius)
obj = obj@Shape(color);
obj.radius = radius;
end
function a = area(obj)
a = pi * obj.radius^2;
end
function p = perimeter(obj)
p = 2 * pi * obj.radius;
end
end
end
```
**逻辑分析:**
* `Shape` 抽象类定义了公共接口,包括 `color` 属性和 `area` 和 `perimeter` 抽象方法。
* `Rectangle` 和 `Circle` 派生类实现了 `Shape` 抽象类,并提供了 `area`
0
0
相关推荐
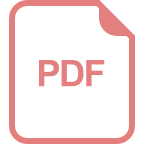
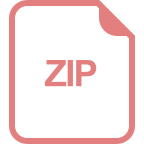
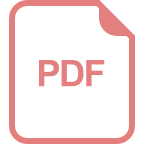
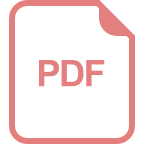
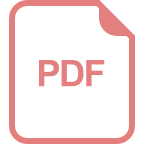
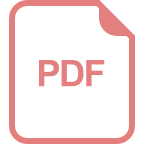