Efficient Text Search in Notepad Using Regular Expressions
发布时间: 2024-09-14 22:03:26 阅读量: 17 订阅数: 23 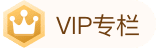
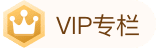
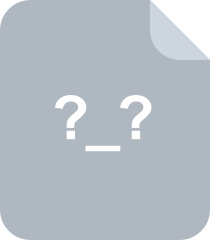
Sublime Text3 可用的Notepad++豆沙绿 软绿色 风格配色方案主题文件
## 1. Efficient Text Searching in Notepad with Regular Expressions
### 1.1 What are Regular Expressions?
A regular expression is a powerful text pattern matching tool that helps users perform flexible and efficient search and replace operations in text.
#### Basic Concepts of Regular Expressions
- Regular expressions consist of literal characters (such as letters, numbers) and special characters (metacharacters) that describe combinations of character patterns.
- Metacharacters include `.` (matches any character), `*` (matches the preceding character 0 or more times), and more, allowing for versatile specification of matching rules.
#### Common Syntax Rules of Regular Expressions
- `[]`: Specifies a character set, such as `[abc]` which matches any single character a, b, or c.
- `()`: Marks a group, combining multiple elements for easier subsequent processing.
- `|`: Represents logical "OR", matching any one of multiple conditions.
Regular expressions are a vital tool in text processing, and mastering their basic concepts and common syntax rules is crucial for efficient searching.
## 2. Introduction to Notepad
Notepad is a simple text editor in the Windows operating system. Although its functions are basic, it is very practical for handling simple text editing tasks. Let's explore Notepad's features and characteristics, and how to use the find function within it.
### 2.1 Features and Characteristics of Notepad
The following table lists some basic features and characteristics of Notepad:
| Feature/Characteristic | Description |
|-----------------------|-----------------------------------------------------------------------------|
| User-friendly interface | Simple interface, intuitive operations |
| Plain text editing | Can edit various text files, does not support complex formatting |
| Automatic line breaks | Automatically adjusts text wrapping based on window size |
| Find and replace | Provides find and replace functionality for convenience and speed |
### 2.2 How to Use the Find Function in Notepad
Using the find function in Notepad is quite simple. Press the shortcut keys `Ctrl + F` or go to the "Edit" option in the menu bar and click "Find" to open the find dialog box. In the dialog box that appears, type the text you want to search for, and click "Find Next" to begin your text search.
Below is an example code snippet demonstrating how to perform find and replace operations automatically in Notepad using a Python script:
```python
import pyautogui
import time
def find_and_replace(target_text, replace_text):
pyautogui.hotkey('ctrl', 'f') # Open the find dialog
pyautogui.typewrite(target_text) # Input the text to find
pyautogui.press('enter') # Start the search
time.sleep(1) # Wait for the search result to appear
pyautogui.write(replace_text) # Input the text to replace
pyautogui.hotkey('ctrl', 'h') # Open the replace dialog
pyautogui.press('enter') # Start the replacement
pyautogui.hotkey('alt', 'f4') # Close the find and replace dialog
find_and_replace("old_text", "new_text") # Example: Replace "old_text" with "new_text" in the document
```
With this code, we can automate find and replace operations in Notepad, enhancing work efficiency. In the next section, we will introduce how to use regular expressions to perform efficient text searching in Notepad.
## 3. Using Regular Expressions for Text Searching in Notepad
Regular expressions are a powerful text matching tool. When combined with Notepad's find function, they enable efficient text searches. This section will show you how to use regular expressions for text searching in Notepad.
### 3.1 How to Enable Regular Expression Search Functionality
Enabling regular expression search functionality in Notepad is straightforward:
1. Open the Notepad software.
2. Use the shortcut keys "Ctrl + H" or click "Edit" -> "Replace" in the menu bar.
3. Check the "Use Regular Expressions" option.
### 3.2 Common Regular Expression Syntax Examples
The syntax and common usage of regular expressions in Notepad are as follows:
| Regular Expression | Description |
|----------
0
0
相关推荐







