决策树模型优化大全:参数调整与交叉验证的高级应用
发布时间: 2024-09-08 08:52:13 阅读量: 272 订阅数: 62 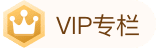
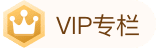
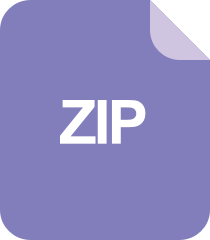
毕设和企业适用springboot企业数据管理平台类及跨境电商管理平台源码+论文+视频.zip
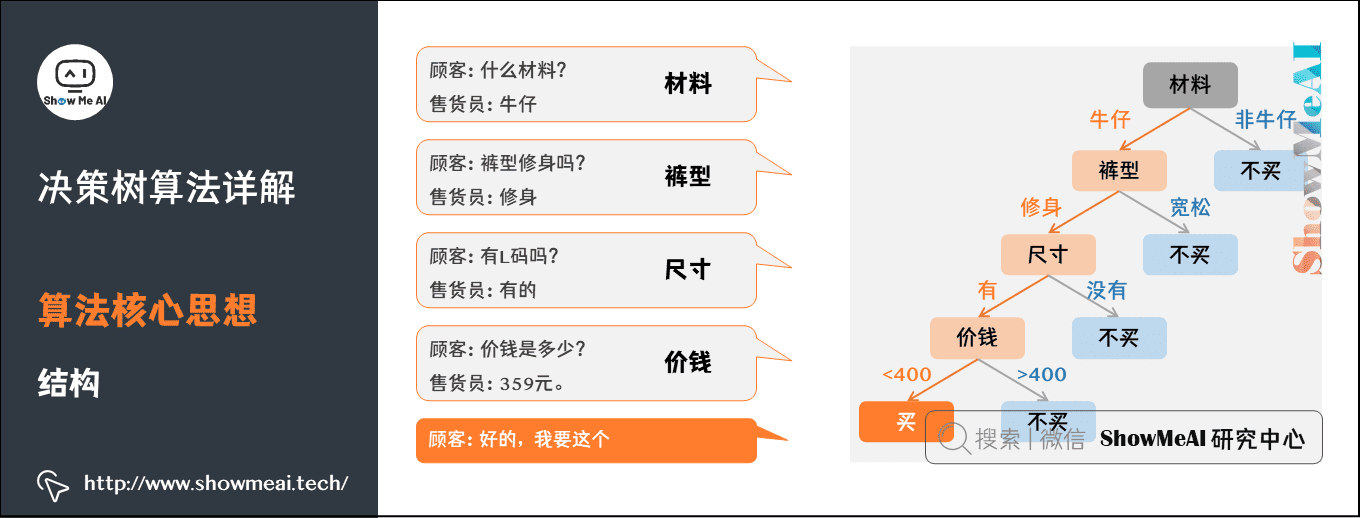
# 1. 决策树模型的理论基础
在探索数据科学的宝库中,决策树模型是一种基础而强大的算法,它通过一系列规则对数据进行划分,以达到预测或分类的目的。决策树的核心在于模拟人类的决策过程,通过“如果-那么”规则,构建出一个树状结构模型,每一个节点代表一个属性或特征的判断,而每个分支代表判断结果的输出。
## 1.1 决策树的构成元素
决策树由内部节点、分支和叶节点组成。内部节点代表特征或属性的选择,分支代表特征选择的结果,叶节点代表最终的决策结果。这一简单的逻辑结构使得决策树容易解释和理解。
## 1.2 决策树的工作原理
构建决策树的过程,本质上是特征选择的过程。从所有可用的特征中,选择对数据集分割最有价值的一个作为当前节点的划分标准。这个选择通常基于信息增益或基尼不纯度等标准。随后,树会递归地为每个子集建立分支和节点,直到满足停止条件。
通过这一章节,读者将能够理解决策树的理论基础,并为进一步掌握决策树模型的高级应用和优化打下坚实的基础。接下来的章节将深入探讨如何通过参数调整、交叉验证和集成方法来提升模型性能。
# 2. 决策树模型的参数调整技巧
决策树模型在实际应用中,模型的性能很大程度上受到其参数的配置影响。本章将深入探讨决策树模型的参数调整技巧,帮助读者掌握如何通过调整参数来优化决策树模型,提升模型的准确性和泛化能力。
## 2.1 决策树参数基础
### 2.1.1 理解决策树的关键参数
在学习如何调整决策树参数之前,我们需要了解一些关键的参数,并且理解它们在模型构建中的作用。
- `criterion`:用于评估切分点的标准,常用的有基尼不纯度(gini)和信息增益(entropy)。
- `max_depth`:决策树的最大深度,控制树的复杂度,防止过拟合。
- `min_samples_split`:内部节点再划分所需的最小样本数。
- `min_samples_leaf`:叶子节点的最小样本数,限制了叶子节点的最小样本量。
- `max_features`:划分时考虑的最大特征数。
### 2.1.2 参数对模型性能的影响
- `criterion`:不同的划分标准对模型的准确度和运行时间有不同的影响。基尼不纯度通常在计算上更快,而信息增益则能提供更纯净的分割。
- `max_depth`:深度越大,模型越复杂,拟合能力越强,但过深可能导致过拟合,同时增加模型训练和预测的时间。
- `min_samples_split`和`min_samples_leaf`:这些参数有助于限制树的增长,减少过拟合的风险,但太高的值可能会导致欠拟合。
### 2.2 高级参数调整方法
#### 2.2.1 使用网格搜索优化参数
网格搜索(Grid Search)是通过枚举所有参数组合来找出最佳模型的一种方法。它将给定的参数范围划分为网格,然后对每个组合进行训练和验证,找到最优的参数组合。
```python
from sklearn.model_selection import GridSearchCV
from sklearn.tree import DecisionTreeClassifier
# 定义参数网格
param_grid = {
'criterion': ['gini', 'entropy'],
'max_depth': [5, 10, 15],
'min_samples_split': [2, 4, 6]
}
# 创建决策树模型
dt = DecisionTreeClassifier()
# 创建GridSearchCV对象
grid_search = GridSearchCV(estimator=dt, param_grid=param_grid, cv=5, verbose=1)
# 执行网格搜索
grid_search.fit(X_train, y_train)
# 输出最佳参数和得分
print("Best parameters found: ", grid_search.best_params_)
print("Best score: ", grid_search.best_score_)
```
通过上述代码,我们使用了五折交叉验证(`cv=5`)来评估不同的参数组合。最终,`GridSearchCV`将输出最佳的参数组合及其对应的得分。
#### 2.2.2 随机搜索与贝叶斯优化
除了网格搜索之外,随机搜索(Randomized Search)和贝叶斯优化(Bayesian Optimization)也是两种常用的参数优化方法。随机搜索在参数空间中随机选择一定数量的参数组合进行测试,而贝叶斯优化则根据已评估点的性能来智能选择下一个测试点。
```python
from sklearn.model_selection import RandomizedSearchCV
from sklearn.tree import DecisionTreeClassifier
from scipy.stats import randint
# 定义参数分布
param_dist = {
'criterion': ['gini', 'entropy'],
'max_depth': randint(1, 20),
'min_samples_split': randint(2, 10)
}
# 创建决策树模型
dt = DecisionTreeClassifier()
# 创建RandomizedSearchCV对象
random_search = RandomizedSearchCV(estimator=dt, param_distributions=param_dist, n_iter=100, cv=5, verbose=1)
# 执行随机搜索
random_search.fit(X_train, y_train)
# 输出最佳参数和得分
print("Best parameters found: ", random_search.best_params_)
print("Best score: ", random_search.best_score_)
```
在实际应用中,通常会先使用随机搜索来缩小参数范围,然后再用网格搜索来精细化调整。
### 2.3 参数调整的实践案例分析
#### 2.3.1 实际数据集上的参数调整流程
接下来,我们将通过一个实际的数据集来演示参数调整的整个流程。
```python
# 假设X_train和y_train为已经准备好的训练数据集
from sklearn.model_selection import train_test_split
# 加载数据集
X, y = load_data() # 假设load_data()是一个用于加载数据的函数
# 分割数据集为训练集和验证集
X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.2, random_state=42)
# 决策树模型实例化
dt = DecisionTreeClassifier(random_state=42)
# 使用GridSearchCV进行参数搜索
param_grid = {
'criterion': ['gini', 'entropy'],
'max_depth': range(1, 11),
'min_samples_split': range(2, 11)
}
grid_search = GridSearchCV(dt, param_grid, cv=5, scoring='accuracy')
grid_search.fit(X_train, y_train)
```
#### 2.3.2 案例结
0
0
相关推荐
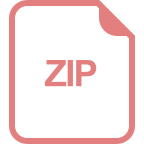
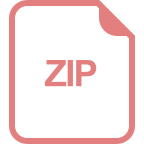