如何将单链表划分为两个部分
发布时间: 2024-04-12 10:03:39 阅读量: 78 订阅数: 45 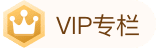
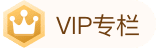
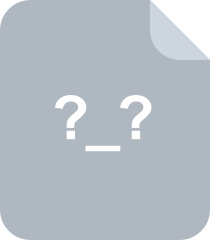
将一个结点值自然数的单链表拆分为两个单链表,原表中保留值为偶数的结点,而值为奇数的结点按它们在原表中的相对次序组成一个新的单链表
# 1. 单链表的基础概念
在计算机科学中,链表是一种常见的线性数据结构。它由一系列节点组成,其中每个节点包含数据项和指向下一个节点的指针。相比于数组,链表的大小在运行时可以动态变化,不需要提前声明大小。然而,链表的查询操作比较耗时,因为需要遍历整个链表找到目标节点。
单链表是最简单的链表形式,每个节点只有一个指向下一个节点的指针。节点的组成通常包括数据和指针两部分。通过指针的连接,多个节点形成一个链表结构,具备顺序存储和动态操作的特性。
总的来说,单链表是一种灵活、简单的数据结构,适合于插入、删除操作频繁的场景,是算法和数据结构中重要的基础知识之一。
# 2. 单链表的常见操作
### 2.1 插入操作
链表的插入操作包括头部插入、尾部插入和指定位置插入。在单链表中,插入操作需要考虑节点指针的重新连接,以确保链表的完整性。
#### 2.1.1 头部插入
头部插入是将新节点插入到链表的开头。首先创建一个新节点,将新节点的指针指向链表的头节点,然后更新链表的头指针指向新节点。
```python
class ListNode:
def __init__(self, value=0, next=None):
self.value = value
self.next = next
def insert_at_head(head, value):
new_node = ListNode(value)
new_node.next = head
return new_node
```
#### 2.1.2 尾部插入
尾部插入是将新节点插入到链表的末尾。遍历链表找到尾节点,然后将尾节点的指针指向新节点,并更新新节点为尾节点。
```python
def insert_at_tail(head, value):
new_node = ListNode(value)
if not head:
return new_node
current = head
while current.next:
current = current.next
current.next = new_node
return head
```
#### 2.1.3 指定位置插入
指定位置插入是将新节点插入到链表的指定位置。遍历链表找到指定位置的前一个节点,然后进行插入操作。
```python
def insert_at_position(head, value, position):
if position == 0:
return ListNode(value, head)
current = head
for _ in range(position - 1):
if current is None:
return head
current = current.next
if current is None:
return head
new_node = ListNode(value, current.next)
current.next = new_node
return head
```
### 2.2 删除操作
链表的删除操作包括删除头部节点、删除尾部节点和删除指定节点。删除操作也需要维护节点指针的连接,确保链表的连接不中断。
#### 2.2.1 删除头部节点
删除头部节点即将链表的头指针指向下一个节点,释放原头节点的内存空间。
```python
def delete_head_node(head):
if head is None:
return None
return head.next
```
#### 2.2.2 删除尾部节点
删除尾部节点需要找到尾节点的前一个节点,将其指针指向空,并释放尾节点的内存空间。
```python
def delete_tail_node(head):
if head is None or head.next is None:
return None
current = head
while current.next.next:
current = current.next
current.next = None
return head
```
#### 2.2.3 删除指定节点
删除指定节点需要找到该节点的前一个节点,将前一个节点的指针绕过指定节点连接到下一个节点,从而删除指定节点。
```python
def delete_node_at_position(head, position):
if position == 0:
return head.next
current = head
for _ in range(position - 1):
if current is None:
return head
current = current.next
if current is None or current.next is None:
return head
current.next = current.next.next
return head
```
以上是单链表的常见操作,包
0
0
相关推荐
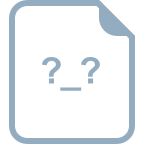





