【邻接图最短路径算法】:Java中的Dijkstra与Bellman-Ford详解
发布时间: 2024-09-10 21:40:24 阅读量: 68 订阅数: 26 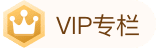
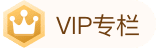
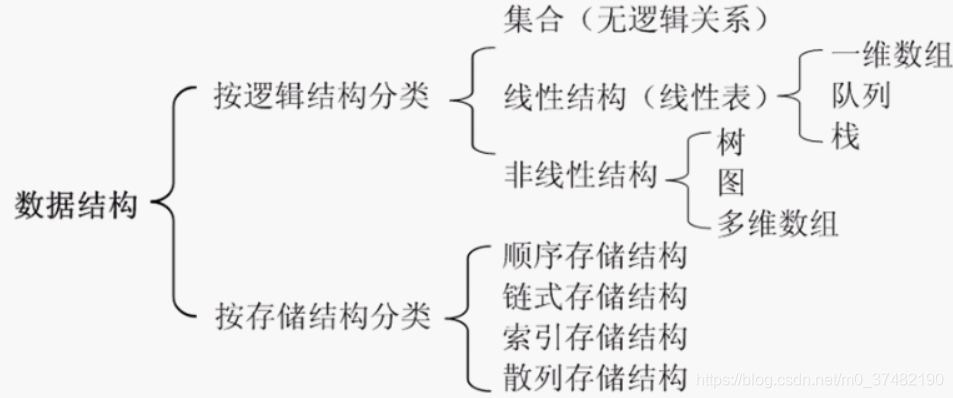
# 1. 图论基础与最短路径问题
## 1.1 图论的基本概念
在计算机科学中,图论是研究图的数学理论和应用的领域。图由节点(顶点)和连接节点的边组成。根据边是否有方向,图可以分为无向图和有向图。在最短路径问题中,通常关心的是图的权重,即边上的数值表示两个顶点之间的距离、成本或其他度量。解决这类问题有助于优化网络数据传输、物流配送、交通导航等多种实际场景。
## 1.2 最短路径问题的定义
最短路径问题(Shortest Path Problem)旨在找到在加权图中两个节点之间的最短路径。这里的“最短”通常指的是路径权重之和最小,但也可以是其他度量,如跳数最少等。这一问题在道路导航、网络通信、社交网络分析等领域具有广泛的应用价值。
## 1.3 最短路径问题的分类
根据不同的条件和约束,最短路径问题可以分为几类:
- 单源最短路径问题(Single-Source Shortest Path Problem):从一个顶点到其他所有顶点的最短路径。
- 全对最短路径问题(All-Pairs Shortest Path Problem):任意两个顶点之间的最短路径。
- 单一最短路径问题(Single Shortest Path Problem):找到两个顶点之间的一个最短路径,不需要考虑所有可能的路径。
这些分类有助于为特定场景选择或开发合适的算法。
# 2. Dijkstra算法的理论与实现
## 2.1 Dijkstra算法概述
### 2.1.1 算法思想与适用场景
Dijkstra算法是图论中解决最短路径问题的一种算法,由荷兰计算机科学家艾兹赫尔·戴克斯特拉(Edsger W. Dijkstra)于1956年提出。该算法的核心思想是贪心策略,即每一步都选择当前已知最短路径的节点,并从未处理的节点集合中排除出去。Dijkstra算法适用于带有非负权重边的有向图和无向图,并且能够计算出给定起点到图中所有其他节点的最短路径。
算法适用场景广泛,例如在网络路由、社交网络中寻找两人之间的最短关系链、地图应用中的路径规划等。Dijkstra算法是许多复杂网络问题的基础,尤其是在单源最短路径问题中,它是目前实现效率最高的算法之一。
### 2.1.2 时间复杂度分析
Dijkstra算法的时间复杂度取决于数据结构的实现方式。在使用简单的邻接矩阵和优先队列的情况下,其时间复杂度为O((V+E)logV),其中V为顶点数,E为边数。如果使用稀疏图的邻接表表示法,时间复杂度可以降低到O(E+VlogV)。
## 2.2 Dijkstra算法的Java实现
### 2.2.1 算法核心代码解析
```java
public class DijkstraAlgorithm {
// 用于标记节点是否已处理
private boolean[] marked;
// 存储从起点到各个顶点的最短路径长度
private int[] distTo;
// 用于确定从起点到其他节点的最短路径
private Edge[] edgeTo;
// Dijkstra算法的主要实现
public DijkstraAlgorithm(EdgeWeightedDigraph graph, int src) {
marked = new boolean[graph.V()];
distTo = new int[graph.V()];
edgeTo = new Edge[graph.V()];
validateVertex(src);
for (int v = 0; v < graph.V(); v++)
distTo[v] = Integer.MAX_VALUE;
distTo[src] = 0;
while (!this.hasQueueEmpty()) {
int v = this.pollQueue();
scanVertex(v, graph);
}
}
private void scanVertex(int v, EdgeWeightedDigraph graph) {
marked[v] = true;
for (Edge e : graph.adj(v)) {
int w = e.to();
if (!marked[w]) {
relax(e);
}
}
}
private void relax(Edge e) {
int v = e.from();
int w = e.to();
if (distTo[w] > distTo[v] + e.weight()) {
distTo[w] = distTo[v] + e.weight();
edgeTo[w] = e;
}
}
}
```
该代码段展示了Dijkstra算法的核心实现。算法首先初始化所有顶点为未标记状态,并设置从起点到每个顶点的距离为无穷大,除了起点本身。接着,算法进入一个循环,每次循环中,算法从优先队列中取出距离最小的顶点,并扫描其所有邻接的未处理顶点,通过松弛(relaxation)操作更新距离值。
### 2.2.2 数据结构选择与优化
在Dijkstra算法中,通常需要使用一个优先队列来存放未处理的顶点,并且每次都能快速获取距离最小的顶点。Java的`PriorityQueue`是实现优先队列的一种高效方式。在Dijkstra算法中,优先队列需要能够快速更新顶点的优先级,因此我们通常使用`TreeMap`或`BinaryHeap`。
### 2.2.3 实例演示与代码测试
为了验证Dijkstra算法的实现,我们可以创建一个图的实例,并运行算法来查找特定源顶点到其他所有顶点的最短路径。
```java
public static void main(String[] args) {
EdgeWeightedDigraph graph = new EdgeWeightedDigraph(5);
// 添加边和权重
graph.addEdge(new Edge(0, 1, 10));
graph.addEdge(new Edge(0, 2, 5));
// ... 添加其他边
int source = 0; // 源顶点为0
DijkstraAlgorithm dijkstra = new DijkstraAlgorithm(graph, source);
// 打印从源顶点到其他顶点的最短路径
for (int i = 0; i < graph.V(); i++) {
System.out.println(source + " to " + i + " : " + dijkstra.distTo[i]);
}
}
```
在上述代码中,我们创建了一个包含5个顶点的图,并添加了一些边和权重。然后我们运行Dijkstra算法并打印出从源顶点0到每个顶点的最短路径长度。
## 2.3 Dijkstra算法的复杂性改进
### 2.3.1 使用优先队列优化
在Dijkstra算法中,使用优先队列可以显著提高性能,尤其是在稀疏图中。优先队列使用堆(如二叉堆)来实现,可以保证从队列中提取最小元素的时间复杂度为O(logV)。
### 2.3.2 优化后的算法复杂性分析
通过使用优先队列,Dijkstra算法的性能主要取决于边的数量和优先队列的操作。每次调用`insert`操作的复杂度为O(logV),每次调用`deleteMin`操作的复杂度为O(logV)。因此,算法的总时间复杂度为O((E+V)logV)。对于稠密图,由于边的数量E接近于V²,因此总时间复杂度接近于O(V²logV);而对于稀疏图,边的数量远小于V²,总时间复杂度接近于O(ElogV)。
为了进一步优化性能,也可以使用斐波那契堆来代替二叉堆实现优先队列,这可以在理论上将时间复杂度降低到O(E+VlogV)。然而,斐波那契堆的实现较为复杂,并且在实际应用中二叉堆通常已经足够快,因此斐波那契堆优化更多地应用于理论研究而非实际工程实现。
# 3. Bellman-Ford算法的理论与实践
## 3.1 Bellman-Ford算法概述
### 3.1.1 算法思想与适用场景
Bellman-Ford算法是一种用于计算给定加权图中单源最短路径的算法。它可以处理带有负权重的边,但不能处理图中存在负权重循环的情况,因为在这种情况下,路径的长度是没有意义的。Bellman-Ford算法的主要思想是迭代地进行松弛操作,即逐步地减少源点到其他所有顶点的路径估计值,直到获得最短路径。
该算法适用场景包括:
- 处理具有负权重的图。
- 需要检测图中是否存在负权重循环。
- 当图的权重随时间变化时,Bellman-Ford算法可以较好地适应,因为它能够重新计算已改变权重的路径。
### 3.1.2 时间复杂度与空间复杂度分析
时间复杂度方面,Bellman-Ford算法需要进行|V|-1次遍历(|V|是顶点数),对于每个顶点,算法需要检查所有出边。因此,时间复杂度为O(|V|*|E|),其中|E|是边数。如果图中存在负权重循环,算法在检测到这一情况时会提前终止。
空间复杂度分析相对简单,Bellman-Ford算法仅需要存储每个顶点到源点的最短路径估计值,因此空间复杂度为O(|V|)。
## 3.2 Bellman-Ford算法的Java实现
### 3.2.1 算法核心代码解析
```java
public class BellmanFord {
// 方法:Bellman-Ford算法实现
```
0
0
相关推荐
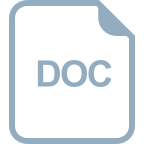
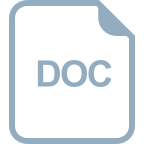
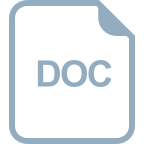
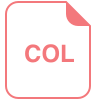
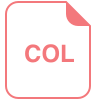
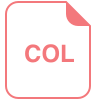
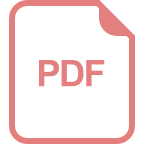
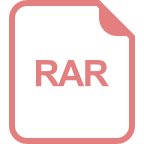
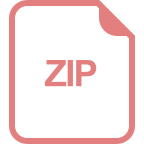