【Python车牌识别实战指南】:从零打造车牌识别系统,轻松上手
发布时间: 2024-08-07 07:21:53 阅读量: 36 订阅数: 24 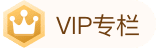
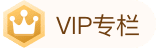
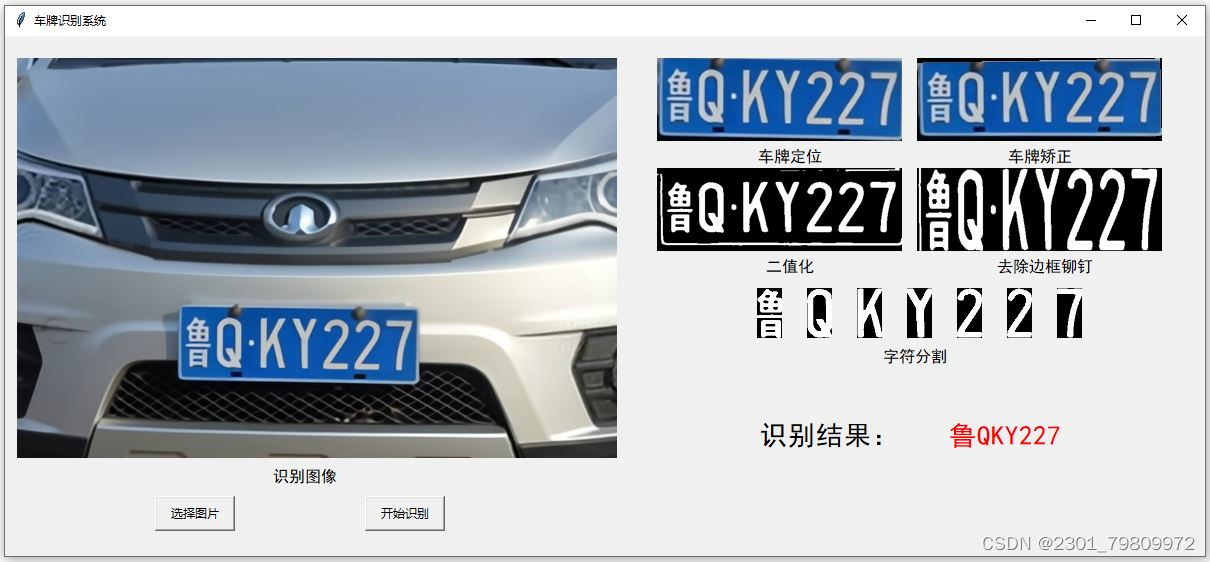
# 1. 车牌识别基础理论
车牌识别是一种利用计算机视觉技术对车牌进行识别和提取的图像处理技术。其核心原理是通过图像预处理、车牌定位和字符识别等步骤,将车牌图像中的字符信息提取出来。
车牌识别技术广泛应用于交通管理、车辆管理和安全监控等领域。它可以实现车辆的自动识别和管理,提高交通效率和安全水平。
# 2. Python车牌识别技术实践
### 2.1 图像预处理
图像预处理是车牌识别系统中至关重要的环节,其目的是将原始图像转换为更适合后续处理的格式。常见的图像预处理技术包括图像灰度化、图像二值化和图像降噪。
#### 2.1.1 图像灰度化
图像灰度化是指将彩色图像转换为灰度图像,即将图像中每个像素的RGB值转换为一个灰度值。灰度值范围为0-255,其中0表示黑色,255表示白色。
```python
import cv2
# 读取彩色图像
image = cv2.imread("car_plate.jpg")
# 转换为灰度图像
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 显示灰度图像
cv2.imshow("Gray Image", gray_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.imread()`函数读取彩色图像。
* `cv2.cvtColor()`函数将彩色图像转换为灰度图像,其中`cv2.COLOR_BGR2GRAY`参数指定转换类型。
* `cv2.imshow()`函数显示灰度图像。
* `cv2.waitKey(0)`函数等待用户按键。
* `cv2.destroyAllWindows()`函数关闭所有窗口。
#### 2.1.2 图像二值化
图像二值化是指将灰度图像转换为二值图像,即将图像中每个像素的值转换为0(黑色)或255(白色)。二值图像通常用于后续的边缘检测和轮廓提取。
```python
import cv2
# 读取灰度图像
gray_image = cv2.imread("car_plate_gray.jpg", cv2.IMREAD_GRAYSCALE)
# 二值化图像
threshold_value = 127
binary_image = cv2.threshold(gray_image, threshold_value, 255, cv2.THRESH_BINARY)[1]
# 显示二值图像
cv2.imshow("Binary Image", binary_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.imread()`函数读取灰度图像。
* `cv2.threshold()`函数进行图像二值化,其中:
* `threshold_value`参数指定二值化阈值。
* `255`参数指定二值化后白色像素的值。
* `cv2.THRESH_BINARY`参数指定二值化类型。
* `cv2.imshow()`函数显示二值图像。
* `cv2.waitKey(0)`函数等待用户按键。
* `cv2.destroyAllWindows()`函数关闭所有窗口。
#### 2.1.3 图像降噪
图像降噪是指去除图像中的噪声,例如椒盐噪声、高斯噪声等。图像降噪可以提高后续处理的准确性。
```python
import cv2
# 读取二值图像
binary_image = cv2.imread("car_plate_binary.jpg", cv2.IMREAD_GRAYSCALE)
# 图像降噪
denoise_image = cv2.fastNlMeansDenoising(binary_image, None, 10, 7, 21)
# 显示降噪后的图像
cv2.imshow("Denoise Image", denoise_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.imread()`函数读取二值图像。
* `cv2.fastNlMeansDenoising()`函数进行图像降噪,其中:
* `None`参数指定使用默认参数。
* `10`参数指定搜索窗口大小。
* `7`参数指定模板窗口大小。
* `21`参数指定搜索半径。
* `cv2.imshow()`函数显示降噪后的图像。
* `cv2.waitKey(0)`函数等待用户按键。
* `cv2.destroyAllWindows()`函数关闭所有窗口。
# 3. Python车牌识别系统实战
### 3.1 系统架构设计
#### 3.1.1 系统模块划分
车牌识别系统主要由以下模块组成:
| 模块 | 功能 |
|---|---|
| 图像采集模块 | 负责采集待识别图像 |
| 车牌识别模块 | 负责对图像进行预处理、定位和字符识别 |
| 结果展示模块 | 负责将识别结果展示给用户 |
#### 3.1.2 数据流处理流程
车牌识别系统的处理流程如下:
```mermaid
graph LR
subgraph 图像采集
A[图像采集] --> B[图像预处理]
end
subgraph 车牌识别
B[图像预处理] --> C[车牌定位] --> D[字符识别]
end
subgraph 结果展示
D[字符识别] --> E[结果展示]
end
```
**1. 图像采集**
图像采集模块负责从摄像头或其他图像源采集图像。
**2. 图像预处理**
图像预处理模块对图像进行一系列处理,包括灰度化、二值化和降噪,以增强图像的质量和提高识别率。
**3. 车牌定位**
车牌定位模块负责在图像中定位车牌区域。它使用边缘检测和轮廓提取等技术来识别车牌的形状和位置。
**4. 字符识别**
字符识别模块负责识别车牌区域内的字符。它使用特征提取和分类器训练等技术来识别每个字符。
**5. 结果展示**
结果展示模块负责将识别结果展示给用户。它可以将结果显示在文本框、图像或其他用户界面元素中。
### 3.2 系统实现
#### 3.2.1 图像采集模块
图像采集模块可以使用 OpenCV 等库来实现。以下代码展示了如何使用 OpenCV 从摄像头采集图像:
```python
import cv2
# 创建VideoCapture对象
cap = cv2.VideoCapture(0)
# 循环读取帧
while True:
# 读取帧
ret, frame = cap.read()
# 如果读取成功,显示帧
if ret:
cv2.imshow('frame', frame)
# 按下'q'键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
else:
break
# 释放摄像头
cap.release()
# 销毁所有窗口
cv2.destroyAllWindows()
```
#### 3.2.2 车牌识别模块
车牌识别模块可以使用 OpenALPR 等库来实现。以下代码展示了如何使用 OpenALPR 识别图像中的车牌:
```python
import alpr
# 创建Alpr对象
alpr = alpr.Alpr("eu", "/path/to/openalpr.conf", "/path/to/openalpr.weights")
# 加载图像
image = cv2.imread("car_plate.jpg")
# 识别车牌
results = alpr.recognize_ndarray(image)
# 打印识别结果
for result in results:
print("车牌:", result['plate'])
print("置信度:", result['confidence'])
print("坐标:", result['coordinates'])
```
#### 3.2.3 结果展示模块
结果展示模块可以使用 Tkinter 等库来实现。以下代码展示了如何使用 Tkinter 创建一个简单的结果展示窗口:
```python
import tkinter as tk
# 创建窗口
window = tk.Tk()
window.title("车牌识别结果")
# 创建文本框
text = tk.Text(window)
text.pack()
# 设置文本框内容
text.insert(tk.END, "车牌:\n置信度:\n坐标:")
# 运行窗口
window.mainloop()
```
# 4. Python车牌识别系统优化
### 4.1 性能优化
#### 4.1.1 并行处理
并行处理是一种通过同时使用多个处理器或内核来执行任务的技术,可以显著提高车牌识别系统的性能。
```python
import multiprocessing
def process_image(image):
# 图像预处理、车牌定位、字符识别
return result
if __name__ == '__main__':
pool = multiprocessing.Pool(processes=4)
images = [image1, image2, image3, image4]
results = pool.map(process_image, images)
```
**代码逻辑:**
1. 创建一个包含指定数量进程的进程池。
2. 将图像列表映射到 `process_image` 函数,该函数执行图像处理和车牌识别任务。
3. `pool.map` 方法并行执行所有任务,并返回结果列表。
#### 4.1.2 算法优化
优化算法可以提高车牌识别系统的速度和准确性。例如,可以使用更快的边缘检测算法或更有效的字符识别模型。
```python
import cv2
def edge_detection(image):
# 使用 Sobel 算子进行边缘检测
return edges
if __name__ == '__main__':
image = cv2.imread('image.jpg')
edges = edge_detection(image)
```
**代码逻辑:**
1. 使用 OpenCV 的 Sobel 算子执行边缘检测。
2. Sobel 算子是一种快速有效的边缘检测算法。
### 4.2 精度优化
#### 4.2.1 数据增强
数据增强是一种通过对现有数据集进行转换和修改来生成新数据的方法,可以提高模型的泛化能力和精度。
```python
import albumentations as A
transform = A.Compose([
A.RandomCrop(width=320, height=160),
A.RandomBrightnessContrast(brightness_limit=0.2, contrast_limit=0.2),
A.HorizontalFlip(p=0.5)
])
if __name__ == '__main__':
dataset = MyDataset(images, labels)
augmented_dataset = dataset.map(transform)
```
**代码逻辑:**
1. 创建一个包含图像增强操作的 Albumentations 转换。
2. 将转换应用于数据集,生成一个包含增强图像的新数据集。
#### 4.2.2 模型调优
模型调优是一种调整模型超参数以提高其性能的过程。可以调整的超参数包括学习率、批大小和正则化参数。
```python
import tensorflow as tf
model = tf.keras.models.Sequential([
# ...
])
optimizer = tf.keras.optimizers.Adam(learning_rate=0.001)
model.compile(optimizer=optimizer, loss='categorical_crossentropy', metrics=['accuracy'])
if __name__ == '__main__':
model.fit(train_data, epochs=10, validation_data=val_data)
```
**代码逻辑:**
1. 创建一个包含指定学习率的 Adam 优化器。
2. 将优化器编译到模型中。
3. 训练模型,并使用验证数据监控其性能。
# 5. Python车牌识别系统应用
车牌识别系统在实际应用中有着广泛的应用场景,主要集中在车辆管理和交通违章管理两个方面。
### 5.1 车辆管理系统
#### 5.1.1 车辆进出管理
在车辆进出管理系统中,车牌识别系统可以自动识别进出车辆的车牌号,并与数据库进行比对,实现车辆的进出管理。具体操作步骤如下:
1. 安装车牌识别摄像头在车辆进出通道处。
2. 摄像头采集车辆图像并进行车牌识别。
3. 将识别的车牌号与数据库中的车辆信息进行比对。
4. 根据比对结果,控制闸门或道闸的开关,允许或拒绝车辆进出。
#### 5.1.2 停车场管理
在停车场管理系统中,车牌识别系统可以自动识别进出停车场的车辆车牌号,并根据停车时间计算停车费用。具体操作步骤如下:
1. 安装车牌识别摄像头在停车场出入口。
2. 摄像头采集车辆图像并进行车牌识别。
3. 将识别的车牌号与数据库中的车辆信息进行比对,获取车辆的进场时间。
4. 当车辆出场时,再次识别车牌号,并计算停车时间。
5. 根据停车时间和停车场收费标准,计算停车费用。
### 5.2 交通违章管理
#### 5.2.1 超速检测
在超速检测系统中,车牌识别系统可以自动识别超速行驶的车辆,并记录其车牌号和超速信息。具体操作步骤如下:
1. 安装车牌识别摄像头在超速检测路段。
2. 摄像头采集车辆图像并进行车牌识别。
3. 将识别的车牌号与数据库中的车辆信息进行比对,获取车辆的限速信息。
4. 根据车辆的实际行驶速度和限速信息,判断车辆是否超速。
5. 如果车辆超速,则记录其车牌号、超速信息和超速时间。
#### 5.2.2 闯红灯检测
在闯红灯检测系统中,车牌识别系统可以自动识别闯红灯的车辆,并记录其车牌号和闯红灯信息。具体操作步骤如下:
1. 安装车牌识别摄像头在红绿灯路口。
2. 摄像头采集车辆图像并进行车牌识别。
3. 将识别的车牌号与数据库中的车辆信息进行比对,获取车辆的闯红灯记录。
4. 根据车辆的实际行驶情况和红绿灯信号,判断车辆是否闯红灯。
5. 如果车辆闯红灯,则记录其车牌号、闯红灯信息和闯红灯时间。
0
0
相关推荐
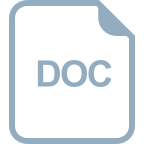
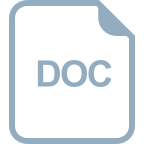
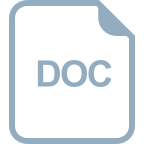
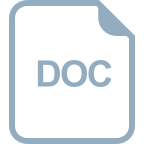
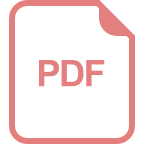
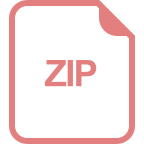
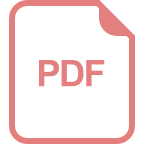
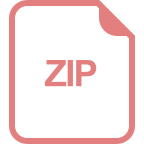
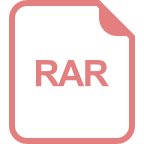