Python Decorators与性能监控:监控函数性能的5个装饰器设计
发布时间: 2024-10-16 20:21:07 阅读量: 49 订阅数: 36 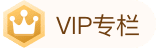
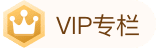
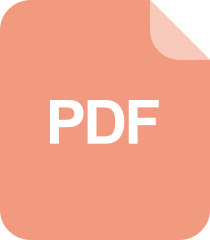
python-装饰器Decorators.pdf
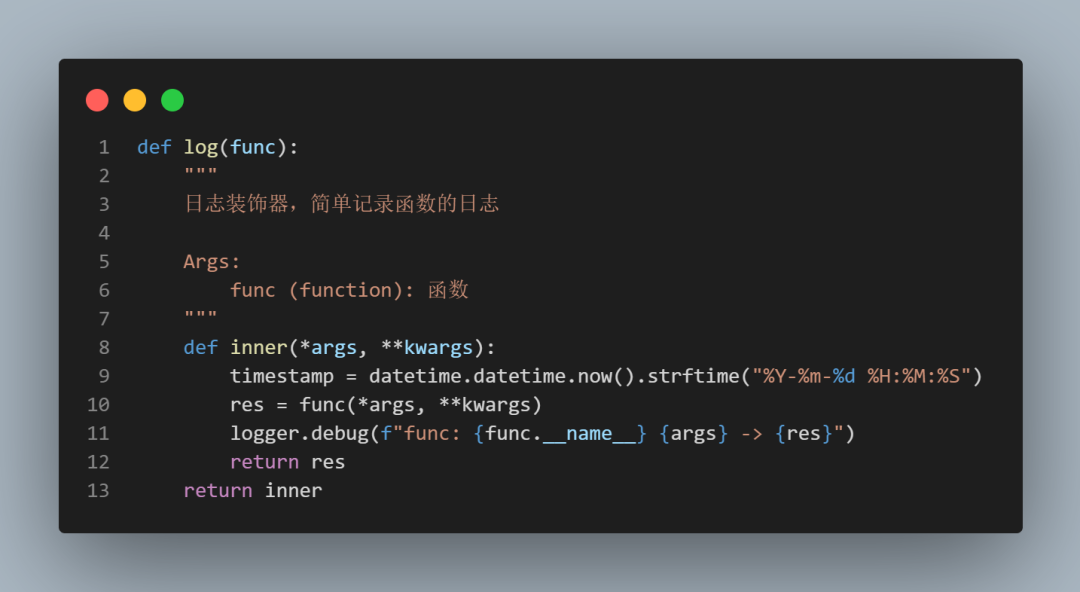
# 1. Python Decorators 简介与原理
Python Decorators 是一种设计模式,它允许程序员在不修改原有函数定义的情况下,增加额外的功能。本质上,装饰器是一种函数,它接受另一个函数作为参数,并返回一个新的函数,这个新函数通常会在原始函数执行前后添加一些功能。
## 装饰器的基本概念和语法
### 函数装饰器的定义
装饰器通过使用 `@` 符号来应用,这是一个语法糖,使得装饰器的使用更加直观。下面是一个简单的装饰器示例:
```python
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
```
### 使用装饰器简化函数代码
装饰器可以在不修改原始函数代码的情况下,添加额外的功能。例如,可以添加日志记录、验证输入参数、缓存函数结果等。
```python
# 日志记录装饰器
def log_decorator(func):
def wrapper(*args, **kwargs):
print(f"Calling {func.__name__} with {args}, {kwargs}")
result = func(*args, **kwargs)
print(f"{func.__name__} returned {result}")
return result
return wrapper
@log_decorator
def multiply(x, y):
return x * y
multiply(2, 3)
```
通过使用装饰器,我们可以避免在每个函数中重复编写相同的代码,从而使代码更加简洁和易于维护。在后续的章节中,我们将深入探讨如何设计和应用更高级的装饰器,以及如何利用装饰器来优化性能和监控代码执行情况。
# 2. 基础装饰器的设计与应用
### 2.1 装饰器的基本概念和语法
#### 2.1.1 函数装饰器的定义
在Python中,装饰器(Decorator)是一种设计模式,它允许用户在不改变函数或方法定义的情况下增加其功能。装饰器本质上是一个接受函数作为参数并返回一个新函数的函数。这种模式在很多情况下都非常有用,尤其是在你需要对函数进行扩展或者修改其行为时。
装饰器通常用于日志记录、性能测量、权限验证、缓存、事务处理等场景。例如,你可以使用装饰器来记录每次函数调用的时间,或者检查用户是否有足够的权限来访问某个函数。
装饰器的基本语法如下:
```python
def decorator(func):
def wrapper():
# 执行一些操作
return func()
return wrapper
@decorator
def my_function():
print("Hello, World!")
my_function()
```
在这个例子中,`decorator` 是一个装饰器,它将 `my_function` 作为参数,并返回一个新的函数 `wrapper`。`my_function` 被装饰后,每次调用 `my_function()` 时,实际上是在调用 `wrapper()` 函数。
#### 2.1.2 使用装饰器简化函数代码
装饰器可以用来简化函数代码,减少重复,提高代码的可读性和可维护性。例如,你可以使用装饰器来自动记录函数的调用时间和参数,而不需要在每个函数中手动添加日志记录代码。
下面是一个简单的装饰器示例,用于记录函数调用的时间:
```python
import time
def log_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time} seconds to execute.")
return result
return wrapper
@log_decorator
def my_function(x):
time.sleep(1) # 模拟耗时操作
return x ** 2
print(my_function(5))
```
在这个例子中,`log_decorator` 是一个装饰器,它记录了被装饰函数的执行时间。当你调用 `my_function(5)` 时,它会输出函数的名称和执行时间。
### 2.2 第一个装饰器实例:记录函数调用
#### 2.2.1 实现基本的日志记录功能
为了实现基本的日志记录功能,我们可以创建一个装饰器,它会在函数执行前后记录时间戳和函数名。这样,我们就可以轻松地追踪函数的调用情况。
下面是一个简单的日志记录装饰器的实现:
```python
import datetime
def log_decorator(func):
def wrapper(*args, **kwargs):
print(f"[{datetime.datetime.now()}] Function '{func.__name__}' is called with arguments: {args} and keyword arguments: {kwargs}")
result = func(*args, **kwargs)
print(f"[{datetime.datetime.now()}] Function '{func.__name__}' returned: {result}")
return result
return wrapper
@log_decorator
def my_function(x, y):
return x + y
my_function(5, 3)
```
在这个例子中,`log_decorator` 装饰器会在 `my_function` 被调用前后记录时间戳和函数名。这样,我们可以清楚地看到函数的调用时间和返回值。
#### 2.2.2 装饰器的参数化和高级特性
装饰器也可以被参数化,这意味着你可以创建一个装饰器工厂,它接受参数并返回一个装饰器。这样,你就可以在装饰器内部使用这些参数来定制装饰器的行为。
下面是一个参数化装饰器的例子:
```python
import datetime
def log_decorator(log_level):
def decorator(func):
def wrapper(*args, **kwargs):
if log_level == "info":
print(f"[{datetime.datetime.now()}] Function '{func.__name__}' is called with arguments: {args} and keyword arguments: {kwargs}")
result = func(*args, **kwargs)
if log_level == "info":
print(f"[{datetime.datetime.now()}] Function '{func.__name__}' returned: {result}")
return result
return wrapper
return decorator
@log_decorator(log_level="info")
def my_function(x, y):
return x + y
my_function(5, 3)
```
在这个例子中,`log_decorator` 是一个装饰器工厂,它接受一个参数 `log_level` 并返回一个装饰器。装饰器 `decorator` 接受一个函数 `func` 并返回一个新的函数 `wrapper`。当 `log_level` 等于 "info" 时,它会在函数调用前后打印日志信息。
### 2.3 第二个装饰器实例:时间测量
#### 2.3.1 测量函数执行时间的方法
为了测量函数执行时间,我们可以创建一个装饰器,它记录函数开始执行的时间和结束执行的时间,然后计算两者之间的差值。这样,我们就可以知道函数执行所需的时间。
下面是一个测量函数执行时间的装饰器的实现:
```python
import time
def time_measure(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time} seconds to execute.")
return result
return wrapper
@time_measure
def my_function(x):
time.sleep(1) # 模拟耗时操作
return x ** 2
print(my_function(5))
```
在这个例子中,`time_measure` 装饰器会在 `my_function` 被调用前后记录时间戳。这样,我们可以清楚地看到函数的执行时间。
#### 2.3.2 如何优化装饰器以减少性能开销
装饰器本身也会带来一定的性能开销,因为它们需要在每次函数调用时执行额外的代码。为了优化性能,我们应该尽量减少装饰器内部的计算量,并且避免在装饰器内部进行不必要的操作。
下面是一个优化后的 `time_measure` 装饰器的例子:
```python
import time
def time_measure(func):
def wrapper(*args, **kwargs):
start_time = time.perf_counter()
result = func(*args, **kwargs)
end_time = time.perf_counter()
print(f"{func.__name__} took {end_time - start_time} seconds to execute.")
return result
return wrapper
@time_measure
def my_function(x):
time.sleep(1) # 模拟耗时操作
return x ** 2
print(my_function(5))
```
在这个例子中,我们使用了 `time.perf_counter()` 而不是 `time.time()` 来测量时间。`time.perf_counter()` 提供了一个更高精度的时间测量方法,这可以进一步减少装饰器的性能开销。
### 2.4 代码块、表格、mermaid流程图的使用
#### 2.4.1 代码块的使用
在本章节中,我们已经多次使用了代码块来展示装饰器的实现。代码块不仅可以清晰地展示代码,还可以通过注释来解释代码的逻辑。
例如,下面是一个带有注释的装饰器代码块:
```python
def decorator(func):
def wrapper(*args, **kwargs):
# 执行一些操作
return func(*args, **kwargs)
return wrapper
```
在这个代码块中,`decorator` 是一个装饰器,它接受一个函数 `func` 并返回一个新的函数 `wrapper`。`wrapper` 函数执行一些操作,然后调用原始函数 `func`。
#### 2.4.2 表格的使用
在本章节中,我们使用了表格来展示装饰器的参数化和高级特性。表格可以帮助读者更好地理解装饰器的行为。
例如,下面是一个表格,展示了不同 `log_level` 参数下的日志记录行为:
| Log Level | Behavior |
|-----------|----------|
| info | Print function call and return information |
| error | Print only error information |
#### 2.4.3 mermaid流程图的使用
在本章节中,我们没有使用 mermaid 流程图。但是,如果你想要展示装饰器的工作流程,mermaid 流程图是一个很好的工具。
例如,下面是一个 mermaid 流程图,展示了装饰器的工作流程:
```mermaid
graph TD
A[Start] --> B[Decorate Function]
B --> C[Call Wrapper Function]
C --> D[Execute Original Function]
D --> E[R
```
0
0
相关推荐







