Python Decorators在Web开发中的应用:Flask和Django的10个实践案例
发布时间: 2024-10-16 19:21:54 阅读量: 16 订阅数: 26 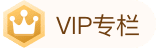
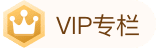

# 1. Python Decorators简介
## 1.1 Decorators的基本概念
在Python中,Decorators是一种设计模式,它允许用户在不修改原有函数或类定义的情况下,增加额外的功能。Decorator本质上是一个函数,它接受另一个函数作为参数,返回一个新的函数作为结果。这个新函数通常会在原始函数执行前后添加一些逻辑。
```python
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
```
在上面的例子中,`my_decorator`是一个Decorator,它在原始的`say_hello`函数执行前后打印了一些信息。
## 1.2 Decorators的使用场景
Decorators在Python编程中非常有用,特别是在以下几个场景:
- **日志记录**:在函数执行前后记录日志信息,方便跟踪程序运行情况。
- **权限验证**:在执行特定函数前进行用户权限检查。
- **性能分析**:在函数执行前后记录时间,用于性能分析。
- **缓存**:将函数的结果缓存起来,下次调用时直接返回缓存结果,减少重复计算。
## 1.3 Decorators的进阶用法
随着对Decorators理解的深入,我们可以编写更复杂的Decorator,例如:
- **装饰器接收参数**:让Decorator本身也能够接收额外的参数,以便更加灵活地控制装饰行为。
- **装饰器链式调用**:一个函数可以被多个Decorator装饰,形成一层层的装饰链。
```python
def repeat(times):
def decorator_repeat(func):
def wrapper(*args, **kwargs):
for _ in range(times):
result = func(*args, **kwargs)
return result
return wrapper
return decorator_repeat
@repeat(times=3)
def greet(name):
print(f"Hello {name}!")
greet("Alice")
```
在上述代码中,`repeat`是一个接收参数的Decorator,它可以让被装饰的函数重复执行指定次数。通过这种方式,我们可以创建更加强大和灵活的Decorator。
# 2. Flask框架中的Decorators应用
## 2.1 Flask路由和视图的装饰
在Flask框架中,Decorators是用于修改或增强函数行为的强大工具。在这一节中,我们将深入探讨如何使用Decorators来定义路由以及在路由参数中应用Decorators。
### 2.1.1 使用@装饰器定义路由
在Flask应用中,路由是将URL映射到视图函数的过程。使用`@app.route()`装饰器可以非常方便地完成这一任务。这个装饰器不仅定义了路由的URL,还可以指定HTTP方法(如GET、POST等)。
```python
from flask import Flask
app = Flask(__name__)
@app.route('/')
def index():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
```
在上述代码中,`@app.route('/')`装饰器将`index`函数映射到根URL。当用户访问主页时,将调用`index`函数并返回响应“Hello, World!”。
#### 路由参数的应用
路由参数允许我们在URL中传递参数给视图函数。例如,如果我们想创建一个用户个人资料页面,我们可以这样做:
```python
@app.route('/user/<username>')
def show_user_profile(username):
return f'User {username}'
@app.route('/post/<int:post_id>')
def show_post(post_id):
return f'Post {post_id}'
```
在这里,`<username>`和`<int:post_id>`是路由参数。`<username>`匹配任何字符串,而`<int:post_id>`匹配整数。
### 2.1.2 装饰器在路由参数中的应用
Decorators还可以与其他装饰器结合使用,例如`@app.login_required`,这需要用户登录才能访问特定的路由。
```python
from flask import Flask, redirect, url_for
@app.route('/admin')
@app.login_required
def admin_page():
return 'Admin Panel'
@app.login_required
def login():
return redirect(url_for('index'))
```
在这个例子中,`@app.login_required`装饰器确保只有经过验证的用户才能访问`/admin`路由。`login`函数重定向未登录的用户到首页。
#### 路由参数的深入分析
路由参数不仅可以是静态的,还可以动态地从URL中提取值。这为创建动态Web应用提供了极大的灵活性。例如,如果我们要为每个用户创建一个个人资料页面,我们可以这样做:
```python
@app.route('/user/<username>')
def user_profile(username):
# 假设我们有一个函数来获取用户数据
user_data = get_user_data(username)
return render_template('user_profile.html', user=user_data)
```
在这个例子中,`<username>`是一个动态路由参数,它将匹配URL中的任何用户名称,并将其作为参数传递给`user_profile`函数。
#### 路由参数的进一步分析
为了进一步理解路由参数的工作原理,我们可以使用Flask内置的`url_map`来查看路由配置。
```python
from flask import current_app
@app.route('/user/<username>')
def user_profile(username):
print(current_app.url_map)
return 'User Profile'
```
运行上述代码,我们将在终端看到所有路由的详细信息,包括动态路由参数。
通过本章节的介绍,我们已经了解了如何使用Flask中的`@app.route()`装饰器来定义路由和路由参数。这些基础是构建动态Web应用的关键。接下来,我们将探讨如何在Flask中使用Decorators
0
0
相关推荐
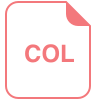
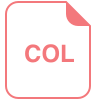
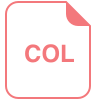
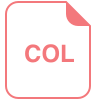
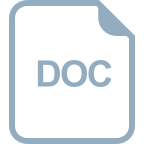
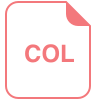
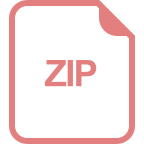
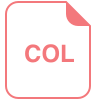
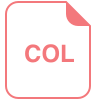