C Language Image Pixel Data Input and Analysis [Image Reading] PNG Image Reading
发布时间: 2024-09-14 18:59:39 阅读量: 28 订阅数: 19 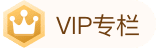
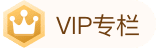
# 1. Introduction
In this chapter, we will introduce the subject and purpose of this article, summarizing the content and focus to be discussed.
# 2. A Brief Introduction to PNG Image Format
PNG (Portable Network Graphics) is a lossless compressed bitmap graphic file format widely used in image processing and transmission. PNG image files employ various color modes, such as indexed color, grayscale, and true color, and support transparency channels. Its features include:
- Utilization of the Deflate compression algorithm for lossless compression of image data, preserving image details.
- Support for Alpha channels, enabling semi-transparent effects in images.
- Use of text labels and metadata for convenient storage of additional information.
- Adoption of Adaptive Filtering to preprocess image data, reducing file size.
The structure of a PNG image file mainly consists of a PNG file header, image data chunks, and a file footer. Pixel data is stored in IDAT data chunks, with each pixel represented by RGB (red, green, blue) or RGBA (red, green, blue, alpha) ***pared to JPEG format, PNG excels in lossless compression and transparent backgrounds but may result in larger file sizes.
When processing PNG images, special attention must be paid to the storage method of pixel data and color channels to accurately read and analyze the image information.
# 3. Image Reading Libraries in C Language
In C language, there are many commonly used image processing libraries to choose from, some of which specialize in reading and processing PNG image files. Below, we will briefly introduce several common image processing libraries, their features, and applicable scenarios.
1. **libpng**
- **Features:** `libpng` is an open-source PNG image processing library that offers a rich set of functions to read, write, and manipulate PNG image files.
- **Applicable Scenarios:** Suitable for projects that require detailed processing and analysis of PNG images, offering high flexibility and customizability.
2. **stb_image**
- **Features:** `stb_image` is a lightweight image processing library that comes in a single header file, is easy to use, and is easily integrated into projects.
- **Applicable Scenarios:** Suitable for simple PNG image reading needs, offering excellent results for quickly retrieving image pixel data.
3. **OpenCV**
- **Features:** `OpenCV` is a set of open-source cross-platform computer vision libraries that support not only PNG format but also the reading and processing of various image formats.
- **Applicable Scenarios:** Suitable for projects that require complex image processing and computer vision tasks, powerful and supports operations with various image formats.
The choice of the appropriate image processing library depends on the complexity of project requirements and the desired functionality. If you only need to read PNG image files and obtain pixel data, a lightweight library like `stb_image` can be chosen; for more image processing and analysis, `libpng` or `OpenCV` might be more suitable. In the following chapters, we will discuss in detail how to use these libraries to read and analyze pixel data in PNG image files.
# 4. Reading PNG Images and Obtaining Pixel Data
In this section, we will discuss in detail how to use C language image reading libraries to open and read PNG image files and how to obtain pixel data from PNG files. We will explore the arrangement of pixel data, storage formats, and other details to help readers better understand the image data processing.
First, let's use an example to demonstrate how to read PNG image files using the libpng library in C:
```c
#include <stdio.h>
#include <stdlib.h>
#include <png.h>
void read_png_file(char *filename) {
FILE *fp = fopen(filename, "rb");
png_structp png = png_create_read_struct(PNG_LIBPNG_VER_STRING, NULL, NULL, NULL);
if (!png) {
fclose(fp);
return;
}
png_infop info = png_create_info_struct(png);
if (!info) {
png_destroy_read_struct(&png, NULL, NULL);
fclose(fp);
return;
}
png_init_io(png, fp);
png_read_info(png, info);
int width = png_get_image_width(png, info);
int height = png_get_image_height(png, info);
int color_type = png_get_color_type(png, info);
int bit_depth = png_get_bit_depth(png, info);
// Reading pixel data
png_bytep *row_pointers = (png_bytep*)malloc(sizeof(png_bytep) * height);
for (int y = 0; y < height; y++) {
row_pointers[y] = (png_byte*)malloc(png_get_rowbytes(png, info));
}
png_read_image(png, row_pointers);
// Processing pixel data
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
// Process each pixel data
png_byte* ptr = &(row_pointers[y][x * 4]); // 4 represents RGBA channels
// Perform pixel value processing, can get RGBA values for operation
}
}
// Free memory and resources
for (int y = 0; y < height; y++) {
free(row_pointers[y]);
}
free(row_pointers);
png_destroy_read_struct(&png, &info, NULL);
fclose(fp);
}
int main() {
char *filename = "example.png";
read_png_file(filename);
return 0;
}
```
In the code above, we use the libpng library to read PNG image files and obtain pixel data. First, we open the file and create the appropriate png_struct and png_info structures to read image information. Then, we use functions like `png_get_image_width` and `png_get_image_height` to get the image's width, height, color type, and bit depth. Next, we allocate memory and use the `png_read_image` function to read pixel data, and finally, we process each pixel.
With this code example, we can clearly understand how to read PNG image files and obtain pixel data in C language, laying the foundation for subsequent image processing and analysis work.
# 5. Image Pixel Data Analysis and Processing
During the image processing process, the obtained pixel data is crucial. Once we successfully read the PNG image file and obtain the pixel data, we can begin various operations and processing on the image. The following will discuss how to analyze and process image pixel data:
1. **Pixel Value Parsing**:
In image processing, understanding the value of each pixel in the image is essential. By reading the pixel data, you can obtain the numerical values of each pixel point, usually representing color values. These values can be grayscale values (for grayscale images), RGB values (for color images), etc., depending on the color representation method.
2. **Number of Channels and Color Formats**:
Pixel data typically contains different channels to represent the color information of the image. Depending on the type of image (grayscale image, color image), pixel data may include one channel, three channels (red, green, blue), or four channels (red, green, blue + transparency). You need to parse pixel data according to the image's color format and the number of channels.
3. **Pixel Data Processing Methods**:
Once pixel data is obtained, various processing can be performed based on actual needs, such as image filtering, edge detection, color adjustment, etc. Depending on different processing requirements, appropriate algorithms and methods can be selected to process pixel data, thereby achieving image improvement and optimization.
4. **Sample Code**:
Below is a simple sample code demonstrating how to read PNG image files and obtain pixel data:
```python
import png
def read_png_image(file_path):
with open(file_path, 'rb') as f:
image = png.Reader(file=f)
width, height, pixels, metadata = image.read_flat()
return width, height, pixels
# Read PNG image file
file_path = 'example.png'
width, height, pixels = read_png_image(file_path)
# Print image width, height, and pixel data
print("Image width:", width)
print("Image height:", height)
print("Pixels data:", pixels)
```
With the above code examples, you can read PNG image files and obtain their pixel data, providing basic data support for subsequent image processing operations.
When processing image pixel data, you need to choose appropriate processing methods based on specific requirements and goals to ensure the effective implementation of image processing tasks.
# 6. Application Examples and Conclusion
In this section, we will demonstrate how to read and analyze pixel data in PNG image files through a simple example. First, we need to use the C language image reading library (such as libpng) to open and read PNG image files, and then obtain pixel data. Next, we can parse the pixel data, including pixel values, channel numbers, and color formats. Finally, we will provide some common pixel data processing methods and techniques.
```c
#include <stdio.h>
#include <stdlib.h>
#include <png.h>
void read_png_image(const char *file_name) {
FILE *fp = fopen(file_name, "rb");
if (!fp) {
fprintf(stderr, "Error: Unable to open file %s\n", file_name);
return;
}
png_structp png_ptr = png_create_read_struct(PNG_LIBPNG_VER_STRING, NULL, NULL, NULL);
if (!png_ptr) {
fclose(fp);
fprintf(stderr, "Error: Unable to create read struct\n");
return;
}
png_infop info_ptr = png_create_info_struct(png_ptr);
if (!info_ptr) {
png_destroy_read_struct(&png_ptr, NULL, NULL);
fclose(fp);
fprintf(stderr, "Error: Unable to create info struct\n");
return;
}
png_init_io(png_ptr, fp);
png_read_info(png_ptr, info_ptr);
int width = png_get_image_width(png_ptr, info_ptr);
int height = png_get_image_height(png_ptr, info_ptr);
int bit_depth = png_get_bit_depth(png_ptr, info_ptr);
int color_type = png_get_color_type(png_ptr, info_ptr);
printf("PNG image details:\n");
printf("Width: %d\nHeight: %d\nBit Depth: %d\nColor Type: %d\n", width, height, bit_depth, color_type);
// Read pixel data and process
png_destroy_read_struct(&png_ptr, &info_ptr, NULL);
fclose(fp);
}
int main() {
const char *file_name = "image.png";
read_png_image(file_name);
return 0;
}
```
**Code Explanation:**
- First, we define a `read_png_image` function to open and read PNG image files.
- In the `main` function, we specify the PNG image file to be read as `image.png`, and then call the `read_png_image` function.
- In the `read_png_image` function, we use libpng library-related functions to open and read PNG image files and output basic information such as width, height, bit depth, and color type.
**Result Description:**
- After running the program, it will output the relevant information of the PNG image file `image.png`, including width, height, bit depth, and color type.
- With this simple example, we can implement the functionality of reading PNG image files and obtaining basic information.
Through the above example, we have a more intuitive understanding of how to read and analyze pixel data in PNG image files. In practical applications, we can perform further processing and analysis based on the read pixel data, thereby realizing more complex image processing functions.
0
0
相关推荐








