C Language Pixel Data Input and Analysis [Image Loading] JPG Image Loading
发布时间: 2024-09-14 19:00:24 阅读量: 16 订阅数: 19 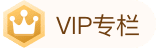
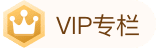
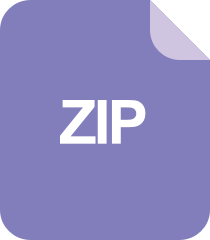
java计算器源码.zip
# 1. Introduction
## 1.1 Introduction to JPG Image File Format
JPEG (Joint Photographic Experts Group) is a common image compression format widely used in digital photography and web image transmission. JPG image files use lossy compression techniques to reduce file size to some extent while maintaining image quality. A JPG file is essentially composed of a series of image pixel data.
## 1.2 The Organization of Image Pixel Data
Images are stored in a computer as pixels, with each pixel containing color information. For JPG image files, pixel data is encoded and stored in the file according to certain rules. Understanding this organization is essential for correctly reading and processing JPG image files. In the subsequent chapters, we will explore how to use the C programming language to read and analyze the pixel data of JPG image files.
# 2. How C Language Reads JPG Image Files
In image processing, reading JPG image files is a basic operation. Below we will introduce how to use the C programming language to read JPG image files.
### 2.1 Using Third-Party Libraries or Native C Language Implementation
In C, we can choose to use third-party libraries (such as libjpeg) to process JPG image files or use native C language implementations to read and process JPG image files. Using third-party libraries is usually more convenient and efficient, but if you want to gain an in-depth understanding of the principles of image processing, native C language implementation is also a good choice.
### 2.2 Basic Steps for Processing JPG Image Files
The basic steps for reading JPG image files include opening the file, reading the file header information, and reading pixel data. In C, we usually implement these operations through file operation functions (such as fopen, fread, etc.). Next, we will delve into how to use the C programming language to read the pixel data of JPG image files.
# 3. Storage and Parsing of Image Pixel Data
Understanding how image pixel data is stored and parsed is very important in image processing. This chapter will introduce the memory model of image pixel data and how to read and parse pixel data.
#### 3.1 Memory Model of Image Pixel Data
Images are represented in a computer as pixels, each containing color information. Generally, images are stored in memory as a two-dimensional array, ***mon image pixel representations include RGB format (red, green, blue), RGBA format (red, green, blue, transparency), and grayscale images.
#### 3.2 Reading Pixel Data and Parsing
In image processing, we need to read image pixel data, and then perform various image processing tasks. Pixel data can be read by accessing the binary data of the image and parsing it into specific pixel information.
```java
// Java example: Reading image pixel data and parsing
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class PixelDataParser {
public static void main(String[] args) {
try {
// Reading image file
File file = new File("image.jpg");
BufferedImage image = ImageIO.read(file);
// Getting the width and height of the image
int width = image.getWidth();
int height = image.getHeight();
// Traversing image pixels and getting pixel information
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int pixel = image.getRGB(x, y);
// Parsing RGB information from pixel values
int red = (pixel >> 16) & 0xff;
int green = (pixel >> 8) & 0xff;
int blue = pixel & 0xff;
System.out.println("Pixel at (" + x + "," + y + "): RGB(" + red + "," + green + "," + blue + ")");
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
With the above code, we can read the pixel data from the image file and parse out the RGB color information for each pixel point. This is very helpful for subsequent image processing and analysis.
# 4. Application of Image Data Analysis Tools
Image data analysis is a very important part of the image processing field. Through in-depth analysis of image data, hidden information and features in the image can be revealed. In C, we can write programs to read image pixel data and analyze it.
#### 4.1 Using C Language for Pixel Data Analysis
In C, we can use file operation functions to read JPG image files and load their pixel data into memory. Then we can write analysis functions to count the number of pixels with different color values, calculate the brightness of the image, etc.
Here is a simple example code for counting the number of pixels in each color channel of an image:
```c
#include <stdio.h>
#include <stdlib.h>
#define IMG_WIDTH 640
#define IMG_HEIGHT 480
// Structure definition representing a pixel
typedef struct {
unsigned char red;
unsigned char green;
unsigned char blue;
} Pixel;
int main() {
FILE *file = fopen("image.jpg", "rb");
if (file == NULL) {
printf("Error opening file\n");
return 1;
}
Pixel image[IMG_HEIGHT][IMG_WIDTH];
fread(image, sizeof(Pixel), IMG_WIDTH * IMG_HEIGHT, file);
int redCount = 0;
int greenCount = 0;
int blueCount = 0;
for (int i = 0; i < IMG_HEIGHT; i++) {
for (int j = 0; j < IMG_WIDTH; j++) {
redCount += image[i][j].red;
greenCount += image[i][j].green;
blueCount += image[i][j].blue;
}
}
printf("Red pixel count: %d\n", redCount);
printf("Green pixel count: %d\n", greenCount);
printf("Blue pixel count: %d\n", blueCount);
fclose(file);
return 0;
}
```
#### 4.2 Analyzing the Color Distribution in Pixel Data
In the above example code, by traversing the image pixel data, we counted the number of pixels in the red, green, and blue channels, and can analyze the color distribution in the image based on these counts. With further algorithms and analysis, we can implement more complex image processing functions, such as color recognition, edge detection, etc.
The application of image data analysis tools can help us better understand the content of images and provide important references for subsequent image processing and recognition.
# 5. Examples and Practice
In this section, we will demonstrate how to use the C programming language to read JPG image files and analyze pixel data. Through example code, we can more intuitively understand the entire process.
#### 5.1 Example Code Display: Reading JPG Image Files and Analyzing Pixel Data
```c
#include <stdio.h>
#include <stdlib.h>
#include "jpeglib.h"
int main() {
FILE *file = fopen("sample.jpg", "rb");
if (!file) {
perror("Error opening file");
return 1;
}
struct jpeg_decompress_struct cinfo;
struct jpeg_error_mgr jerr;
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_decompress(&cinfo);
jpeg_stdio_src(&cinfo, file);
jpeg_read_header(&cinfo, TRUE);
jpeg_start_decompress(&cinfo);
int width = cinfo.output_width;
int height = cinfo.output_height;
int num_components = cinfo.output_components;
JDIMENSION image_width = cinfo.output_width;
JDIMENSION image_height = cinfo.output_height;
JSAMPARRAY buffer = (*cinfo.mem->alloc_sarray)((j_common_ptr) &cinfo, JPOOL_IMAGE,
image_width * image_height, 1);
printf("Image width: %d\n", width);
printf("Image height: %d\n", height);
printf("Number of components: %d\n", num_components);
int pixel_count = width * height;
while (cinfo.output_scanline < height) {
JDIMENSION rows = jpeg_read_scanlines(&cinfo, buffer, cinfo.output_height);
for (int i = 0; i < rows; i++) {
JSAMPROW row = buffer[i];
for (int j = 0; j < width; j++) {
// Pixel processing
}
}
}
jpeg_finish_decompress(&cinfo);
jpeg_destroy_decompress(&cinfo);
fclose(file);
return 0;
}
```
##### Code Explanation:
- Reads JPG image files and obtains pixel data information through the `jpeglib.h` library.
- Uses the `jpeg_read_scanlines` function to read image pixel data line by line and store it in `buffer`.
- Pixel data processing can be performed in the loop, such as color analysis and other operations.
#### 5.2 Practice: Extracting Key Information from JPG Images
Based on the example code, we can expand the functionality in practice to implement the extraction and statistical analysis of the number of specific color pixels from JPG images. This can help us understand the composition and characteristics of image data more deeply.
# 6. Conclusion and Outlook
In this article, we have in-depth discussed the methods of using C language to read JPEG image files and analyze pixel data. Through the introduction of the basic structure of JPEG image files, the storage method of pixel data, and the reading and parsing process, readers can better understand the internal mechanisms of JPEG image files.
Through actual example code demonstrations and practical operations, we have shown how to use the C programming language to process JPEG image files, read pixel data, and perform basic data analysis. In the practical process, we can extract useful information from the image data, such as color distribution, which provides a foundation for further image processing and analysis.
Looking to the future, with the continuous development of image processing technology and the optimization of the C programming language, C language still holds an important position in the field of image processing. Future development directions may include the implementation of more efficient image processing algorithms, the development of more intelligent image data analysis tools, and the involvement of broader application scenarios. Through continuous learning and exploration, the possibilities in the C language image processing field will be even broader.
0
0