【Java数据结构高级应用】:BlockingQueue使用场景优化分析
发布时间: 2024-09-11 11:31:53 阅读量: 111 订阅数: 43 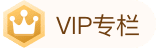
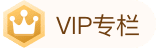
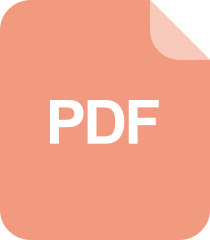
基于java中BlockingQueue的使用介绍
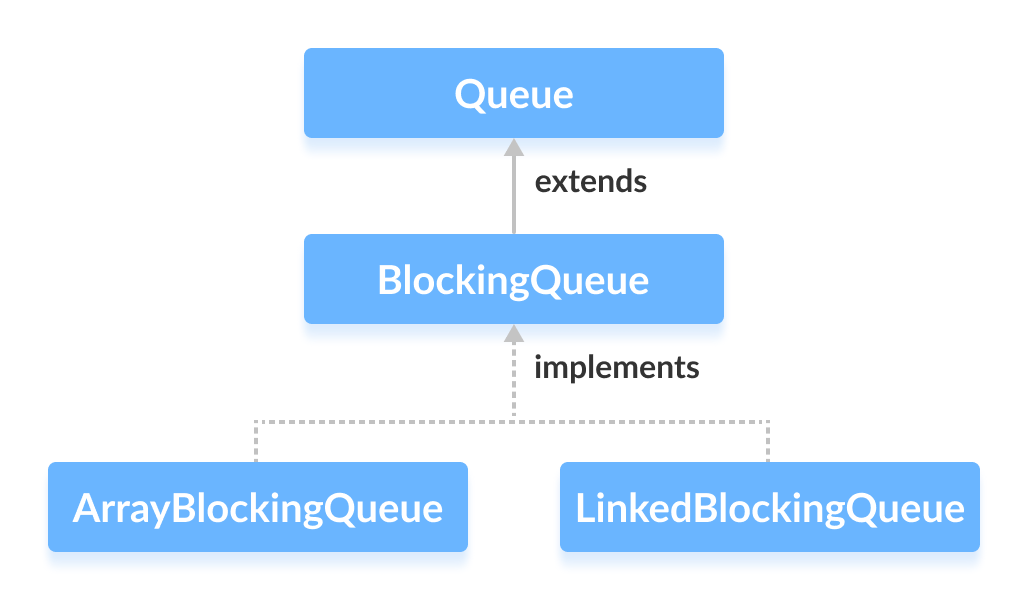
# 1. Java BlockingQueue 概述
Java的`BlockingQueue`是一个接口,它是Java集合框架的一部分,专为多线程设计。其核心功能在于,能够在生产者线程和消费者线程之间提供阻塞和等待机制,以确保对队列进行线程安全的操作。当队列满时,向其中添加元素的操作会阻塞生产者线程直到有空间为止;当队列空时,消费者线程取元素的操作会被阻塞直到队列中有元素可取。这种同步机制是多线程环境下实现线程间协作与资源控制的有效手段。
从功能上来说,`BlockingQueue`不仅保证了元素的添加和移除操作的原子性,也提供了多种队列操作方式,例如,阻塞式插入元素、阻塞式移除元素、带有超时的非阻塞操作等。这使得`BlockingQueue`成为实现生产者-消费者模式及其他并发算法的理想选择。
在接下来的章节中,我们将深入探讨`BlockingQueue`的内部实现机制,以及在不同场景中的具体应用和性能优化策略。了解`BlockingQueue`的工作原理,对于设计高效且线程安全的多线程应用程序至关重要。
# 2. BlockingQueue 的内部实现机制
### 2.1 基于锁的线程同步
#### 2.1.1 ReentrantLock 的使用和原理
ReentrantLock是Java中提供的一个可重入的互斥锁,它提供了比synchronized关键字更高级的线程同步功能。ReentrantLock允许尝试获取锁失败的线程等待指定时间后再次尝试获取锁,并且提供了公平和非公平的锁实现。它通常通过try-finally结构来确保锁的释放,避免了锁无法释放的风险。
在Java并发编程中,ReentrantLock内部使用了AbstractQueuedSynchronizer(AQS)来管理同步状态。AQS内部维护了一个整型的状态变量和一个线程的引用,这个线程引用在特定状态下会被设置为当前占有锁的线程。当一个线程尝试获取锁时,会调用AQS的acquire方法,该方法会检查当前状态是否允许获取锁,若不允许则将当前线程加入到同步队列中等待。
**代码示例:**
```java
import java.util.concurrent.locks.ReentrantLock;
public class ReentrantLockExample {
private final ReentrantLock lock = new ReentrantLock();
public void performTask() {
lock.lock();
try {
// Critical section of code
} finally {
lock.unlock();
}
}
}
```
在上述示例中,`lock()`方法尝试获取锁,如果锁已被其他线程占用,则调用线程将阻塞直到锁被释放。`unlock()`方法释放锁,无论当前线程是否持有锁,都必须在`try-finally`块的`finally`部分进行释放,以确保锁的正确释放。
#### 2.1.2 Condition 条件变量的协作机制
在多线程环境下,Condition提供了比Object类的wait/notify更强大的线程协调功能。一个Condition关联到一个Lock上,可以用来实现多个等待/通知场景。一个线程可以在等待条件变量时释放锁,这样其他线程就可以进入临界区修改条件,并通过`signal()`或`signalAll()`方法唤醒等待的线程。
**代码示例:**
```java
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class ConditionExample {
private final Lock lock = new ReentrantLock();
private final Condition condition = lock.newCondition();
private boolean ready = false;
public void await() {
lock.lock();
try {
while (!ready) {
condition.await(); // 暂停线程,释放锁
}
} finally {
lock.unlock();
}
}
public void signal() {
lock.lock();
try {
ready = true;
condition.signalAll(); // 唤醒所有等待线程
} finally {
lock.unlock();
}
}
}
```
在这个示例中,`await()`方法使得调用它的线程在`ready`变为`true`之前阻塞,释放了锁。一旦另一个线程调用了`signal()`方法,`await()`方法中的线程将被唤醒。被唤醒的线程在返回前需要重新获取锁。
### 2.2 队列的存储结构
#### 2.2.1 数组实现的 BlockingQueue
数组实现的BlockingQueue是最常见的,如ArrayBlockingQueue。这种实现方式在初始化时需要指定数组的大小,生产者和消费者通过循环数组进行元素的添加和移除。由于数组的容量是固定的,它提供了一种有界的队列,可以防止内存溢出问题。
ArrayBlockingQueue的数组结构为:
```java
private final Object[] items;
```
其中,`items`数组存储队列中的元素。对于非公平锁版本,生产者和消费者直接竞争锁,而在公平锁版本中,会优先让等待时间最长的线程获取锁。
**代码示例:**
```java
import java.util.concurrent.ArrayBlockingQueue;
public class ArrayBlockingQueueExample {
public static void main(String[] args) {
ArrayBlockingQueue<Integer> queue = new ArrayBlockingQueue<>(10);
for (int i = 0; i < 5; i++) {
try {
queue.put(i); // 添加元素到队列
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
for (int i = 0; i < 5; i++) {
try {
System.out.println(queue.take()); // 从队列取出元素
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
}
```
在这个示例中,创建了一个容量为10的ArrayBlockingQueue实例,然后添加了5个元素。通过`put`和`take`方法,元素被添加到队列和从队列中移除。
#### 2.2.2 链表实现的 BlockingQueue
链表实现的BlockingQueue,如LinkedBlockingQueue,是一种使用链表作为其存储结构的阻塞队列。这种队列的容量不需要在初始化时指定,因为链表的大小是动态的。它提供了无界队列,但也可以通过构造函数指定容量来创建有界队列。
LinkedBlockingQueue的内部结构为:
```java
private static class Node<E> {
E item;
Node<E> next;
Node(E x) { item = x; }
}
private transient Node<E> head;
private transient Node<E> last;
private final ReentrantLock takeLock = new ReentrantLock();
private final Condition notEmpty = takeLock.newCondition();
private final ReentrantLock putLock = new ReentrantLock();
private final Condition notFull = putLock.newCondition();
```
这里`head`和`last`指向队列的头部和尾部,`takeLock`和`putLock`分别控制队列的取出和添加操作,以及它们各自的条件变量`notEmpty`和`notFull`。
**代码示例:**
```java
import java.util.concurrent.LinkedBlockingQueue;
public class LinkedBlockingQueueExample {
public static void main(String[] args) {
LinkedBlockingQueue<Integer> queue = new LinkedBlockingQueue<>();
for (int i = 0; i < 5; i++) {
try {
queue.put(i); // 添加元素到队列
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
for (int i = 0; i < 5; i++) {
try {
System.out.println(queue.take()); // 从队列取出元素
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
}
```
在这个示例中,创建了一个默认容量的LinkedBlockingQueue实例,然后添加了5个元素。通过`put`和`take`方法,元素被添加到队列和从队列中移除。
### 2.3 线程协调的等待/通知模式
#### 2.3.1 wait/notify/notifyAll 的用法和原理
`wait()`、`notify()`和`notifyAll()`是Java Object类提供的用于多线程之间协调的方法。`wait()`方法使得当前线程释放对象锁,并进入等待状态,直到其他线程调用同一个对象的`notify()`或`notifyAll()`方法。当有线程调用了`notify()`方法时,被阻塞在`wait()`上的线程将有机会被唤醒,但具体哪个线程被唤醒是不确定的。如果调用了`notifyAll()`,所有等待这个对象锁的线程都会被唤醒。
**代码示例:**
```java
synchronized (object) {
while (!condition) {
object.wait();
}
// 处理业务逻辑
}
```
在这
0
0
相关推荐
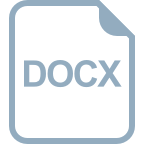
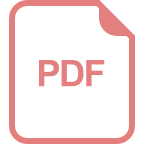
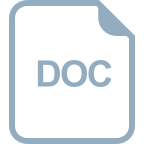
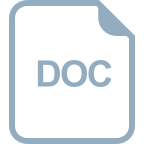
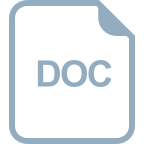
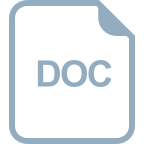
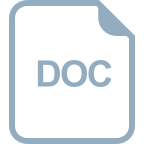
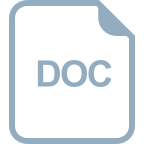