【复杂度分析速成课】:掌握算法核心,Python面试不再难
发布时间: 2024-09-01 04:36:27 阅读量: 218 订阅数: 93 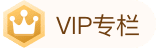
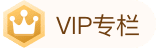
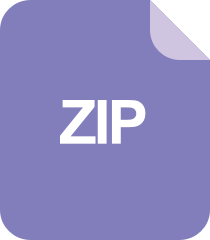
Python速成课程:Eric Matthes的Python速成课程2nd Ed之后的练习和项目
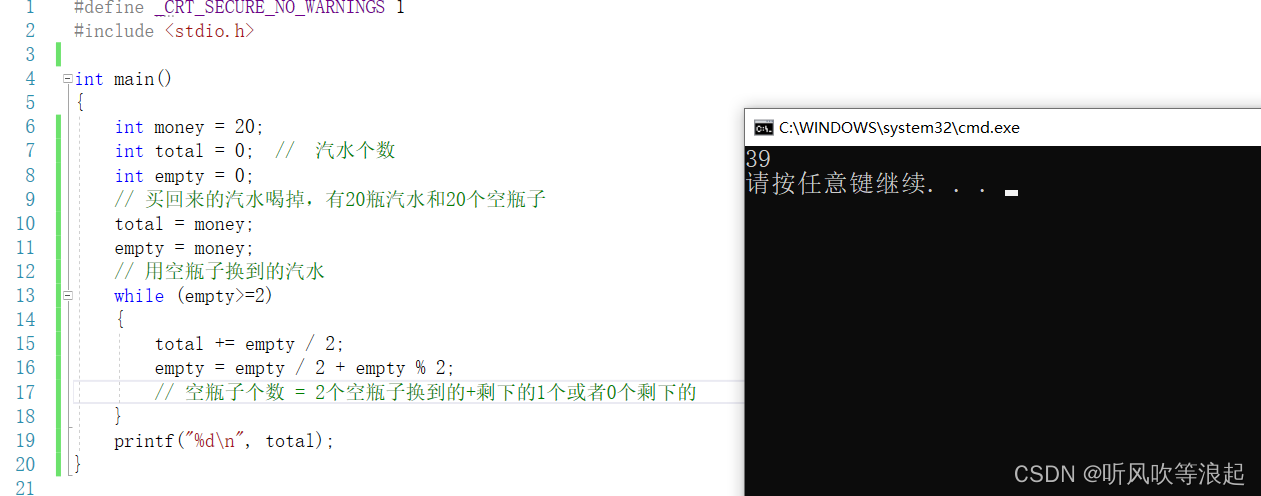
# 1. 算法与复杂度基础概念
在信息技术领域,算法是解决问题和执行任务的核心。了解算法的基本概念和复杂度分析是每个IT专业人士的必备知识。复杂度分为时间复杂度和空间复杂度,它们分别描述了算法在时间资源和空间资源上的需求。本文将带你入门复杂度基础概念,为深入学习打下坚实基础。
## 1.1 算法定义与重要性
算法是解决特定问题的一系列定义良好的计算步骤。在编程中,算法的效率直接影响软件的性能。一个高效的算法可以在有限的资源内快速解决问题,反之,则可能导致资源浪费和性能瓶颈。
## 1.2 时间与空间复杂度的概念
时间复杂度评估的是算法运行时间与输入数据规模之间的关系。通常用大O表示法来简化描述,例如O(n)表示线性时间复杂度。空间复杂度则是算法占用存储空间的量度。
## 1.3 理解大O表示法
大O表示法是一种表达算法性能的数学符号,它忽略常数和低阶项,专注于主要影响因素。比如,O(1)代表常数时间,O(n)代表线性时间,O(n^2)代表二次时间。理解大O表示法对于评估算法性能至关重要。
以上内容为本章的基础,后续章节会进一步深入探讨复杂度的各个方面,揭示算法设计与分析的秘密。
# 2. 时间复杂度的理论与实践
## 2.1 时间复杂度基本理论
### 2.1.1 大O表示法
大O表示法是描述算法性能的数学方法,用以估算算法运行时间与数据输入大小之间的关系。它关注的是随着输入规模N的增加,算法执行时间的增长率,用最坏情况下的时间复杂度来表示。例如,如果一个算法的时间复杂度是O(N),那么我们可以大致理解为算法的执行时间与输入数据的大小成正比。
大O表示法的公式通常写成`O(f(n))`的形式,其中`f(n)`是一个函数,表示随着输入数据规模`n`的增加,算法执行所需要的次数。常见的时间复杂度如O(1),O(log n),O(n),O(n log n),O(n^2)等。
### 2.1.2 时间复杂度的种类和特点
在这一部分,我们将详细介绍不同种类的时间复杂度,包括它们的特点和适用场景。
- **常数时间O(1)**: 无论输入规模如何,算法执行时间都是固定的。这种类型的算法通常不受输入数据的影响,例如访问数组中的元素。
- **对数时间O(log n)**: 通常出现在分而治之的算法中,比如二分查找。随着输入数据的增加,算法所需的执行时间以对数速率增长。
- **线性时间O(n)**: 算法的执行时间与输入数据的大小成正比。典型的线性时间算法是遍历数据结构中的元素。
- **线性对数时间O(n log n)**: 这类算法常见于最有效的排序算法,如快速排序和归并排序。随着数据规模的增加,算法的执行时间以线性对数速率增长。
- **二次时间O(n^2)**: 这种时间复杂度常见于简单的排序算法(冒泡、选择、插入排序)和嵌套循环。随着数据规模的增加,算法的执行时间以二次速率增长。
## 2.2 常见算法的时间复杂度分析
### 2.2.1 循环结构的时间复杂度
考虑一段循环代码,假设它的执行时间与循环次数成正比。若循环内的代码时间复杂度为O(1),那么整个循环结构的时间复杂度为O(n),其中n为循环次数。
```python
for i in range(n):
# 每次循环执行常数时间的操作
```
在这个例子中,每次循环的时间开销是恒定的。因此,整个循环的时间复杂度就是O(n)。
### 2.2.2 分治算法的时间复杂度
分治算法通常将问题分解为更小的子问题,递归地解决这些子问题,并将结果合并。递归函数的调用次数以及每层递归的开销会影响整个算法的时间复杂度。例如,归并排序的每层递归执行的次数都是O(n),而递归的深度为O(log n),因此总的时间复杂度是O(n log n)。
```python
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
while left and right:
if left[0] < right[0]:
result.append(left.pop(0))
else:
result.append(right.pop(0))
result.extend(left or right)
return result
```
### 2.2.3 动态规划算法的时间复杂度
动态规划算法通过将问题分解为重叠的子问题并存储这些子问题的解来避免重复计算,进而减少算法的时间复杂度。计算一个子问题的解时,动态规划通常要遍历所有相关的子问题,因此时间复杂度为O(n^2),n是子问题的数量。当子问题的依赖关系形成一个树状结构时,时间复杂度可能达到O(2^n)。
```python
def fibonacci(n):
if n <= 1:
return n
dp = [0, 1] + [0] * (n-1)
for i in range(2, n+1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# 时间复杂度分析:
# 有两个嵌套循环,外层循环n次,内层循环也为n次,因此时间复杂度是O(n^2)
```
## 2.3 时间复杂度分析实践案例
### 2.3.1 递归算法的复杂度分析
递归算法中,每个函数调用自身,因此在没有重复计算的情况下,时间复杂度是指数级的。在最佳情况下,通过使用记忆化技术,可以将时间复杂度降低到多项式级别。
以斐波那契数列为例:
```python
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
```
这个递归版本的斐波那契数列算法,其时间复杂度为O(2^n)。这是因为对于每一个函数调用,都有两个后续的函数调用。
### 2.3.2 排序算法的复杂度对比
不同的排序算法在时间复杂度上有着显著的差异。例如,冒泡排序的时间复杂度为O(n^2),而快速排序在最好情况下的时间复杂度为O(n log n)。
下面是一个表格,列出了一些常见排序算法的时间复杂度:
| 排序算法 | 最好情况 | 平均情况 | 最坏情况 | 空间复杂度 |
|----------|----------|----------|----------|------------|
| 冒泡排序 | O(n) | O(n^2) | O(n^2) | O(1) |
| 选择排序 | O(n^2) | O(n^2) | O(n^2) | O(1) |
| 插入排序 | O(n) | O(n^2) | O(n^2) | O(1) |
| 希尔排序 | O(n log n) | O(n log n) | O(n log^2 n) | O(1) |
| 归并排序 | O(n log n) | O(n log n) | O(n log n) | O(n) |
| 快速排序 | O(n log n) | O(n log n) | O(n^2) | O(log n) |
在选择排序算法时,应考虑数据的特点和要求,以平衡时间复杂度和空间复杂度。例如,若数据规模较小,冒泡排序和插入排序的常数因子较小,可能会比较快。
以上对时间复杂度的理论和实践案例的探讨,为理解算法的效率提供了一个框架,并为优化算法性能和选择合适的算法打下基础。
# 3. 空间复杂度的理论与实践
0
0
相关推荐







