【系统设计面试攻略】:Python算法面试中的高级挑战
发布时间: 2024-09-01 04:48:19 阅读量: 586 订阅数: 89 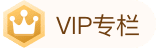
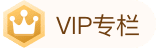
.png)
# 1. Python算法面试概述
在这一章节中,我们将对Python算法面试的基本概念和重要性进行概述,帮助读者建立起对算法面试的初步理解。首先,了解算法面试是软件开发领域中评估候选人逻辑思维能力、编程技巧和问题解决能力的重要环节。其次,分析为何Python在算法面试中备受欢迎,探讨其简洁性、强大的库支持和广泛的应用场景。最后,概述本章节将涵盖的主要内容和学习目标,使读者能够有目的地深入学习后续章节,系统掌握Python算法面试所需的知识和技巧。
# 2. Python基础知识与数据结构
在现代IT行业中,Python已经成为了一门不可或缺的编程语言。它简洁易读,适用于多种编程范式,从脚本编写到复杂系统开发,Python都有其独特的表现。本章将深入探讨Python的核心特性以及数据结构的应用。
## 2.1 Python语言核心特性
Python的设计哲学强调代码的可读性和简洁的语法(尤其是使用空格缩进来定义代码块,而不是使用大括号或关键字)。这样的设计让Python易于学习,也易于阅读。接下来,我们将重点介绍Python的内置数据类型、控制流语句和函数定义。
### 2.1.1 内置数据类型和操作
Python的内置数据类型丰富多样,包括数值类型、序列类型(列表、元组、字符串)、映射类型(字典)、集合类型等。每种类型都有其特定的操作和用途。
```python
# 数值类型示例
a = 10 # 整型
b = 3.14 # 浮点型
c = 1+2j # 复数型
# 序列类型示例
list_example = [1, 2, 3] # 列表
tuple_example = (1, 2, 3) # 元组
string_example = 'Python' # 字符串
# 映射类型示例
dict_example = {'a': 1, 'b': 2, 'c': 3} # 字典
# 集合类型示例
set_example = {1, 2, 3} # 集合
```
Python中的数值类型可以进行算术运算,序列类型可以使用索引和切片操作,映射类型可以使用键访问数据,而集合类型则用于进行数学上的集合运算。Python的这些操作都是构建在强大内置方法上的。
### 2.1.2 控制流语句与函数定义
控制流语句是编程中的基础,Python提供了`if`、`elif`、`else`用于条件判断,以及`for`和`while`循环语句来实现重复执行代码块。函数是组织代码的另一个重要工具,它允许开发者将一段代码封装起来,以便重复使用。
```python
# 函数定义示例
def add(a, b):
return a + b
# 函数使用示例
result = add(3, 4)
print(result) # 输出: 7
```
在Python中,函数使用`def`关键字定义,可以接受参数并返回结果。Python还支持默认参数、可变参数和关键字参数,这让函数更加灵活。
## 2.2 高级数据结构应用
Python不仅提供了基本的数据结构,还支持一些高级的数据结构。这些数据结构通常对于解决复杂问题提供了更高效的方法。本小节中,我们将探讨列表、字典的高级用法,以及栈、队列、树和图的操作。
### 2.2.1 列表和字典的高级用法
列表(List)是一种有序的集合,可以随时添加和删除其中的元素。字典(Dictionary)是一种通过键来存储值的映射类型数据结构。
```python
# 列表推导式示例
squares = [x**2 for x in range(10)]
print(squares) # 输出: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
# 字典推导式示例
squares_dict = {x: x**2 for x in range(10)}
print(squares_dict) # 输出: {0: 0, 1: 1, 2: 4, ...}
```
列表推导式和字典推导式是Python中的高级特性,它们提供了一种简洁的方式来创建列表和字典。此外,字典的`get`方法、`update`方法等提供了更多的灵活性。
### 2.2.2 栈、队列、树和图的操作
除了列表和字典,Python还提供了标准库,比如`collections`模块中的`deque`,可以用于高效的栈和队列操作。树和图通常用于复杂的算法和数据结构,比如用于表示组织结构或网络拓扑结构。
```python
from collections import deque
# 栈操作示例
stack = deque()
stack.append('a')
stack.append('b')
print(stack.pop()) # 输出: b
print(stack) # 输出: deque(['a'])
# 队列操作示例
queue = deque()
queue.append('a')
queue.append('b')
print(queue.popleft()) # 输出: a
print(queue) # 输出: deque(['b'])
```
### 2.2.3 哈希表和字符串处理技巧
哈希表通常在字典类型中隐式使用,但在某些情况下,我们可能需要使用更接近底层的哈希表。Python提供了`hashlib`库来处理哈希算法。字符串处理在Python中非常强大,通过一系列内置方法可以完成各种复杂的文本处理。
```python
import hashlib
# 哈希函数应用示例
message = 'Hello, world!'
hash_object = hashlib.sha256(message.encode())
print(hash_object.hexdigest()) # 输出: sha256哈希值字符串
# 字符串处理示例
text = "Python 3.8"
# 分割字符串
parts = text.split(' ')
print(parts) # 输出: ['Python', '3.8']
# 替换字符串
text = text.replace(' ', '-')
print(text) # 输出: Python-3.8
# 查找子字符串出现的位置
pos = text.index('3')
print(pos) # 输出: 8
```
在上面的示例中,我们演示了如何使用哈希库对字符串进行哈希处理,以及如何利用字符串处理的内置方法进行分割、替换和查找操作。
在本章节中,我们初步探索了Python的基础知识和数据结构的应用,为后续章节中的复杂算法逻辑和系统设计面试打下了坚实的基础。在下一章节中,我们将继续深入探讨复杂算法逻辑和设计模式的应用,帮助读者更好地理解Python在解决实际问题中的强大功能。
# 3. 复杂算法逻辑与设计模式
## 3.1 算法逻辑分析
### 3.1.1 排序和搜索算法深入理解
在数据处理和分析中,排序和搜索是基础且至关重要的算法逻辑。它们不仅用于单个数据集的处理,而且在算法优化和系统设计中发挥着重要作用。深入理解这些算法能够帮助我们编写更有效率的代码。
排序算法中,快速排序、归并排序和堆排序是三种高效的选择,它们的时间复杂度为O(n log n)。快速排序通过分区操作快速将数组分为较小和较大的两个部分,然后递归地排序两个部分。归并排序则是将数组分成两半,对每一半递归地应用归并排序,最后将排序好的两半合并在一起。堆排序使用了二叉堆这种数据结构,通过构建最大堆或最小堆,来实现元素的有序排列。
```python
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
# 示例使用
array = [3, 6, 8, 10, 1, 2, 1]
print(quicksort(array))
```
在搜索算法方面,二分搜索是最经典的搜索技术,它通过将数组分成两半来查找目标值,适用于有序数组。二分搜索的时间复杂度为O(log n),显著快于线性搜索的O(n)。
```python
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
```
0
0
相关推荐
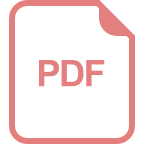
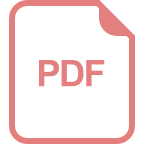
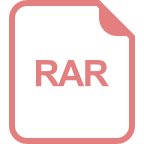
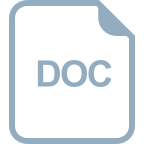
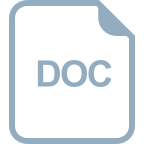
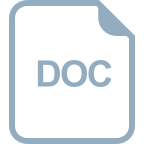
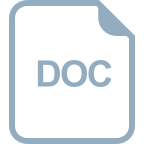
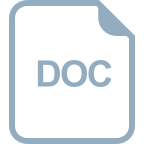
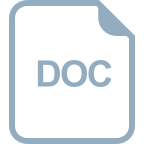