【内存管理秘籍】:Python面试中的性能优化关键
发布时间: 2024-09-01 04:56:16 阅读量: 191 订阅数: 89 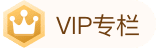
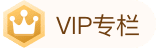
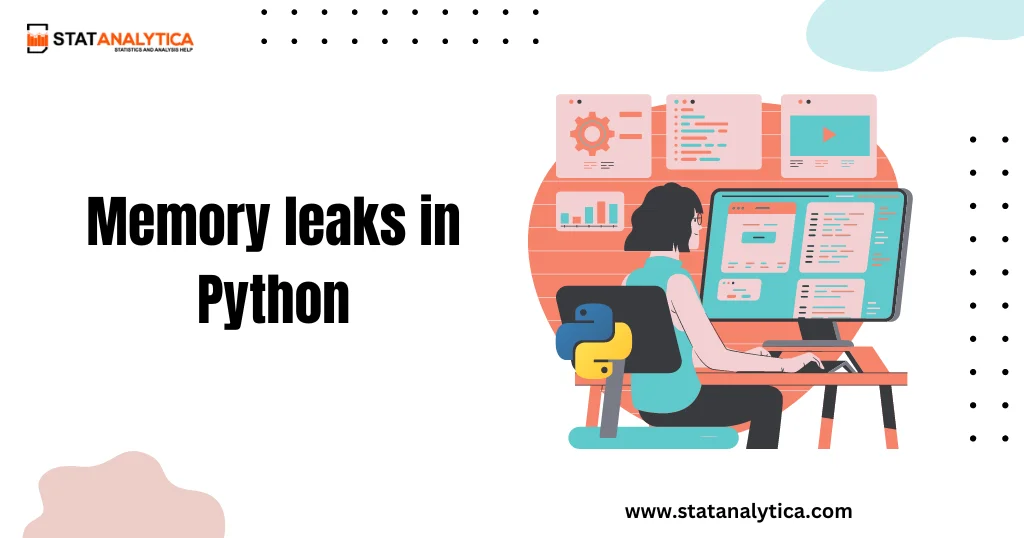
# 1. 内存管理基础与Python内存模型
## 1.1 内存管理基本概念
内存管理是编程语言的基本组成部分,负责分配、回收和管理内存资源,确保程序在有限的内存资源中高效运行。在理解内存管理时,首先要了解内存分配、内存回收以及内存访问权限这三个核心概念。程序运行时,操作系统通常将内存空间划分成多个区域,包括堆(Heap)、栈(Stack)等,不同类型的数据分配在不同的内存区域中。
## 1.2 Python内存模型概述
Python采用自动内存管理机制,其中垃圾回收机制是其核心。Python通过引用计数机制和分代垃圾回收机制来管理内存。引用计数用于跟踪对象的引用次数,当引用次数为零时,对象成为垃圾回收的候选者。分代回收则是基于对象生命周期的假设,将对象分为不同的代,并对年轻的代进行更频繁的垃圾回收,以优化性能。
## 1.3 Python的内存分配与优化
Python内存分配主要由Python内存分配器和C库(如malloc)完成。程序员可以通过多种方式优化内存使用,包括合理使用数据结构、减少全局变量的使用、优化循环逻辑等。此外,一些第三方库如Pympler可以帮助开发者监控内存分配和释放,进一步对程序的内存使用进行优化。在下一章中,我们将详细探讨内存泄漏的问题,以及如何在Python中检测和预防内存泄漏。
# 2. 内存泄漏的理论与案例分析
在第一章中,我们学习了内存管理的基础知识和Python内存模型的基本概念。这为理解内存泄漏奠定了基础。本章将深入探讨内存泄漏的理论知识和案例分析,旨在帮助读者识别、分析和预防内存泄漏问题。
## 2.1 内存泄漏的概念与原因
### 2.1.1 什么是内存泄漏
内存泄漏(Memory Leak)是指程序在申请内存后,未能在使用完毕后释放,导致该内存无法被再次使用,最终可能导致系统资源耗尽的现象。内存泄漏的问题通常不会立即显现,但随着时间的积累,它将影响程序的性能,甚至导致程序崩溃。
在高级语言中,如Python,内存泄漏可能不如低级语言(如C/C++)中常见,因为Python具有垃圾回收机制。但即便如此,不当的编程习惯仍然可能引起内存泄漏。
### 2.1.2 内存泄漏的常见原因
内存泄漏的原因多种多样,但大多数情况下可以归纳为以下几点:
- **循环引用:**在面向对象编程中,当对象彼此引用形成闭环,且没有任何引用指向该闭环时,这部分内存将无法被垃圾回收器识别,从而造成泄漏。
- **全局变量:**过多的使用全局变量可能会导致内存泄漏。因为全局变量的生命周期贯穿整个程序运行期,如果它们被误用,或者无意中存储了不再需要的大型数据,将导致资源不能释放。
- **第三方库或扩展:**使用第三方库或C扩展时,如果开发者未能正确管理内存,也可能导致内存泄漏。
## 2.2 Python内存泄漏的典型案例
### 2.2.1 案例一:循环引用导致的内存泄漏
在Python中,循环引用是内存泄漏的一个常见原因。考虑以下代码:
```python
class Node:
def __init__(self, name):
self.name = name
self.children = []
a = Node('a')
b = Node('b')
a.children.append(b)
b.children.append(a) # a和b形成循环引用
```
在这个例子中,对象`a`和`b`相互引用,形成一个无法被Python垃圾回收机制清理的环。当运行此代码后,即使我们删除了`a`和`b`的本地引用,这两个节点对象也不会被回收,因为它们互为对方的子节点列表的一部分。
### 2.2.2 案例二:全局变量缓存不当
全局变量的不当使用,如大量数据的临时缓存,也可能导致内存泄漏。例如:
```python
cache = {}
def expensive_operation(arg):
if arg not in cache:
cache[arg] = perform_expensive_computation(arg)
return cache[arg]
```
如果`perform_expensive_computation`函数返回了大量数据,而这些数据随后没有被清空或更新,它们将持续占用内存。
### 2.2.3 案例三:C扩展模块的内存管理失误
当Python与C扩展模块交互时,如果C模块没有正确管理内存,就可能导致内存泄漏。这种情况较难检测,因为需要对C代码有深入了解。
## 2.3 检测和定位Python内存泄漏
### 2.3.1 使用内存分析工具
检测和定位内存泄漏的有效方法之一是使用内存分析工具。在Python中,常用的工具包括`memory_profiler`和`objgraph`。
```bash
pip install memory_profiler
```
使用`memory_profiler`时,可以通过以下方式来检测内存泄漏:
```python
import time
from memory_profiler import memory_usage
def run():
a = [i for i in range(10000)]
if __name__ == '__main__':
m1 = memory_usage((run, ()))
time.sleep(10) # 给予程序足够时间运行,以检测潜在泄漏
m2 = memory_usage((run, ()))
print(f"Memory usage: {max(m2) - min(m1)} MB")
```
### 2.3.2 内存泄漏分析技术
内存泄漏分析技术包括以下两种基本策略:
- **比较差异:**通过比较不同时间点的内存使用情况差异来定位泄漏。
- **引用跟踪:**跟踪对象引用,寻找无法访问(无引用)但仍被占用的内存区域。
### 2.3.3 内存泄漏的预防措施
预防措施包括:
- **避免不必要的全局变量。**
- **使用弱引用(weakref)管理缓存。**
- **及时释放不再使用的资源,比如文件和网络连接。**
- **利用垃圾回收器的调试工具进行定期检查。**
通过本章内容,我们深入了解了内存泄漏的概念、原因以及检测与预防方法。在下一章,我们将继续探讨Python中的垃圾回收机制,了解如何优化内存回收过程,以及如何编写更加高效的内存使用代码。
# 3. Python中的垃圾回收机制
在讨论Python内存管理的过程中,垃圾回收机制扮演着至关重要的角色。Python自动管理内存,它通过垃圾回收机制来清理程序中不再使用的对象。这些机制减轻了程序员手动管理内存的负担,同时避免了内存泄漏等问题。
## 3.1 垃圾回收基础
### 3.1.1 引用计数机制
Python使用引用计数作为其垃圾回收的基础。每个对象都会维护一个计数器,当新的引用指向该对象时,计数器增加,当引用被删除或指向新的对象时,计数器减少。当引用计数降至零时,对象会被认为是垃圾,因为它不再被任何部分所使用。
引用计数的实现相对简单,但它的主要局限性是无法检测循环引用的情况。当两个或多个对象相互引用且不被程序的其它部分引用时,它们的引用计数不会降为零,因此它们会继续占用内存,尽管程序中再也没有对它们的引用。
```python
import sys
a = []
b = [a]
a.append(b) # a和b相互引用,引用计数不会为零
```
在这个例子中,`a` 和 `b` 都有至少两个引用(自身和对方),因此它们的引用计数至少为二,即使程序的其他部分不再需要它们,它们也不会被回收。
### 3.1.2 分代回收机制
为了解决引用计数的局限性,Python还实现了分代垃圾回收机制。这个机制将对象分为不同的代(generation),对象在创建后先被放置在年轻代(generation 0),如果它在一次垃圾回收中幸存下来,则会被移动到老年代
0
0
相关推荐
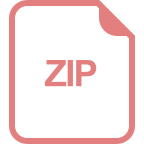
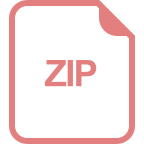
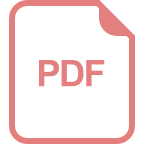
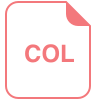
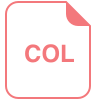
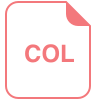
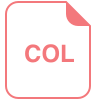
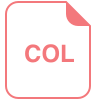
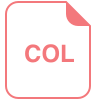