揭秘动态数组的底层奥秘:从扩容机制到性能提升
发布时间: 2024-08-25 16:03:48 阅读量: 28 订阅数: 24 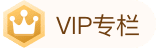
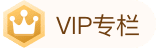
# 1. 动态数组的简介和原理
动态数组是一种可以动态调整大小的数据结构,它可以根据需要自动增加或减少容量。与传统数组不同,动态数组不需要在创建时指定固定大小,这使其非常适合处理大小未知或不断变化的数据集。
动态数组的底层实现通常基于一个连续的内存块,该内存块可以根据需要进行扩展或缩小。当需要添加或删除元素时,动态数组会自动调整内存块的大小,以容纳新的元素或释放不再需要的内存。
# 2. 动态数组的底层实现
### 2.1 扩容机制
动态数组的扩容机制是其核心实现之一,它允许数组在需要时动态地增加其容量。
#### 2.1.1 扩容策略
扩容策略决定了当数组达到其当前容量时如何增加其容量。有两种常见的扩容策略:
- **加倍扩容:** 当数组达到其当前容量时,将其容量加倍。
- **固定大小扩容:** 当数组达到其当前容量时,将其容量增加一个固定的数量,例如 10 或 20。
加倍扩容策略更简单,但可能会导致不必要的内存浪费,特别是当数组容量较大时。固定大小扩容策略更有效率,但需要更仔细地选择扩容大小。
#### 2.1.2 扩容成本
扩容操作的成本是 O(n),其中 n 是数组的当前容量。这是因为扩容需要分配一个新的内存块,并将现有元素复制到新的内存块中。
### 2.2 内存管理
动态数组的内存管理涉及分配和释放内存以满足其容量需求。
#### 2.2.1 内存分配
当创建动态数组时,需要分配内存来存储其元素。内存分配通常使用 malloc() 或 calloc() 等函数进行。
```c
int* arr = (int*) malloc(sizeof(int) * capacity);
```
其中,capacity 是数组的初始容量。
#### 2.2.2 内存释放
当动态数组不再需要时,需要释放其分配的内存。内存释放通常使用 free() 函数进行。
```c
free(arr);
```
释放内存后,数组中的元素将不再有效,并且该内存可以重新用于其他目的。
# 3. 动态数组的性能优化
### 3.1 扩容优化
动态数组在使用过程中,可能会频繁地进行扩容操作。为了提高扩容效率,可以采取以下优化措施:
#### 3.1.1 扩容阈值
扩容阈值是指动态数组在扩容时,需要扩容的最小元素个数。如果扩容的元素个数小于阈值,则不进行扩容。
```cpp
template <typename T>
class DynamicArray {
// ...
// 扩容阈值
size_t capacity_threshold;
// ...
};
```
#### 3.1.2 扩容因子
扩容因子是指动态数组在扩容时,扩容的元素个数与原有元素个数的比值。
```cpp
template <typename T>
class DynamicArray {
// ...
// 扩容因子
double capacity_factor;
// ...
};
```
### 3.2 内存管理优化
动态数组的内存管理也是影响性能的关键因素。以下介绍两种优化内存管理的方法:
#### 3.2.1 内存池
内存池是一种预先分配好固定大小的内存块,当需要分配内存时,直接从内存池中获取,避免了频繁的系统内存分配和释放操作。
```cpp
template <typename T>
class DynamicArray {
// ...
// 内存池
std::vector<T> memory_pool;
// ...
};
```
#### 3.2.2 内存对齐
内存对齐是指将数据结构中的成员变量按照特定的对齐方式进行排列,以提高内存访问效率。
```cpp
template <typename T>
class DynamicArray {
// ...
// 内存对齐
alignas(64) T* data;
// ...
};
```
# 4. 动态数组的实际应用
动态数组是一种多功能的数据结构,可用于各种实际应用中。它特别适合需要高效存储和管理大量数据的场景。本章将探讨动态数组在数据结构和算法实现中的实际应用。
### 4.1 数据结构
#### 4.1.1 队列
队列是一种遵循先进先出(FIFO)原则的数据结构。动态数组可以轻松实现队列,因为它们允许在数组的末尾添加元素(入队)和从数组的开头删除元素(出队)。
```cpp
class Queue {
private:
int front, rear, size;
int* arr;
public:
Queue(int size) {
this->size = size;
arr = new int[size];
front = rear = -1;
}
void enqueue(int data) {
if ((rear + 1) % size == front) {
cout << "Queue is full" << endl;
return;
}
else if (front == -1) {
front = rear = 0;
}
else {
rear = (rear + 1) % size;
}
arr[rear] = data;
}
int dequeue() {
if (front == -1) {
cout << "Queue is empty" << endl;
return -1;
}
int data = arr[front];
arr[front] = -1;
if (front == rear) {
front = rear = -1;
}
else {
front = (front + 1) % size;
}
return data;
}
};
```
**逻辑分析:**
* 构造函数初始化队列,分配动态数组并设置 front 和 rear 指针为 -1。
* enqueue() 函数检查队列是否已满,如果已满则返回错误。否则,它将元素添加到数组的末尾并更新 rear 指针。
* dequeue() 函数检查队列是否为空,如果为空则返回错误。否则,它将数组开头处的元素出队并更新 front 指针。
#### 4.1.2 栈
栈是一种遵循后进先出(LIFO)原则的数据结构。动态数组也可以轻松实现栈,因为它们允许在数组的末尾添加元素(压栈)和从数组的末尾删除元素(弹栈)。
```cpp
class Stack {
private:
int top, size;
int* arr;
public:
Stack(int size) {
this->size = size;
arr = new int[size];
top = -1;
}
void push(int data) {
if (top == size - 1) {
cout << "Stack is full" << endl;
return;
}
arr[++top] = data;
}
int pop() {
if (top == -1) {
cout << "Stack is empty" << endl;
return -1;
}
return arr[top--];
}
};
```
**逻辑分析:**
* 构造函数初始化栈,分配动态数组并设置 top 指针为 -1。
* push() 函数检查栈是否已满,如果已满则返回错误。否则,它将元素添加到数组的末尾并更新 top 指针。
* pop() 函数检查栈是否为空,如果为空则返回错误。否则,它将数组末尾处的元素出栈并更新 top 指针。
### 4.2 算法实现
#### 4.2.1 排序
动态数组可以用于实现各种排序算法,例如冒泡排序、选择排序和快速排序。
```cpp
// 冒泡排序
void bubbleSort(int* arr, int size) {
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
```
**逻辑分析:**
* 外层循环遍历数组中的每个元素,内层循环将相邻元素进行比较并交换。
* 通过多次迭代,将最大的元素逐个移动到数组的末尾。
#### 4.2.2 搜索
动态数组也可以用于实现各种搜索算法,例如线性搜索和二分搜索。
```cpp
// 二分搜索
int binarySearch(int* arr, int size, int key) {
int low = 0, high = size - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (arr[mid] == key) {
return mid;
}
else if (arr[mid] < key) {
low = mid + 1;
}
else {
high = mid - 1;
}
}
return -1;
}
```
**逻辑分析:**
* 将数组划分为两个子数组,比较中间元素与目标值。
* 根据比较结果,调整搜索范围,直到找到目标值或确定其不存在。
# 5.1 泛型动态数组
### 5.1.1 泛型编程
泛型编程是一种编程范式,它允许创建独立于特定数据类型的代码。泛型代码使用类型参数,这些参数在编译时被替换为实际类型。这使得泛型代码可以用于各种数据类型,而无需为每种类型编写单独的代码。
### 5.1.2 泛型动态数组的实现
泛型动态数组可以通过在动态数组的定义中使用类型参数来实现。例如,以下 C++ 代码展示了泛型动态数组的实现:
```cpp
template <typename T>
class DynamicArray {
public:
// ...
};
```
在这个例子中,`T` 是类型参数,它可以被替换为任何数据类型。这允许 `DynamicArray` 类用于存储各种数据类型,例如整数、浮点数或字符串。
泛型动态数组的优点包括:
- **代码重用:**泛型代码可以用于各种数据类型,无需为每种类型编写单独的代码。
- **类型安全:**编译器会检查类型参数的类型安全性,确保泛型代码不会产生无效的类型转换。
- **可扩展性:**泛型代码易于扩展,以支持新的数据类型。
0
0
相关推荐
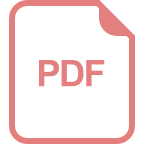
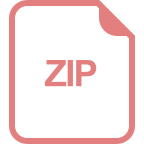
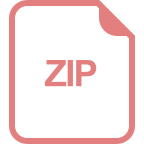
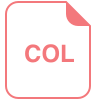
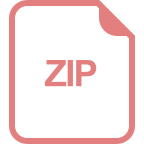
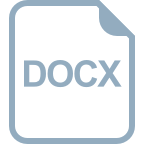
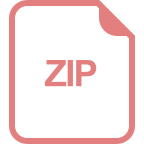
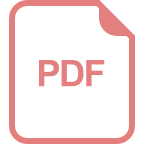