动态数组实战指南:从概念到工程应用
发布时间: 2024-08-25 16:05:55 阅读量: 39 订阅数: 33 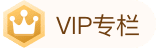
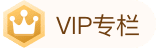
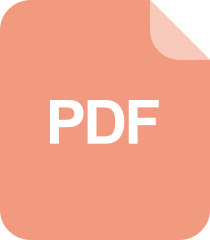
Python科学计算之NumPy与SciPy实战指南
# 1. 动态数组基础**
动态数组是一种数据结构,它可以根据需要动态地调整其大小。与传统数组不同,动态数组不需要预先分配一个固定大小的内存空间,而是可以根据需要自动扩展或缩小。
动态数组的底层通常由一个连续的内存块或链表实现。连续内存块提供了快速访问,而链表则提供了更灵活的插入和删除操作。动态数组通过管理指向底层内存的指针来实现动态大小调整,从而可以高效地添加或删除元素。
# 2. 动态数组的实现**
**2.1 数组的底层数据结构**
动态数组的底层数据结构主要有两种:连续内存块和链表。
**2.1.1 连续内存块**
连续内存块是最常见的动态数组实现方式。它将数组元素存储在连续的内存空间中,每个元素占用固定的内存空间。这种方式访问元素速度快,但扩容和缩容操作比较复杂。
**2.1.2 链表**
链表是一种非连续的动态数组实现方式。它将数组元素存储在不同的内存空间中,每个元素通过指针连接到下一个元素。这种方式扩容和缩容操作简单,但访问元素速度较慢。
**2.2 数组的扩容和缩容**
**2.2.1 扩容策略**
当动态数组达到容量时,需要进行扩容操作。常见的扩容策略有:
- **倍增扩容:**将数组容量扩大到原来的两倍。
- **固定扩容:**将数组容量扩大到一个固定的值。
- **自定义扩容:**根据实际需要自定义扩容策略。
**2.2.2 缩容策略**
当动态数组中元素数量减少时,可以进行缩容操作以释放内存空间。常见的缩容策略有:
- **倍减缩容:**将数组容量缩小到原来的二分之一。
- **固定缩容:**将数组容量缩小到一个固定的值。
- **不缩容:**不进行缩容操作,保持数组容量不变。
# 3. 动态数组的应用
### 3.1 栈和队列
栈和队列是两种基本的数据结构,它们广泛应用于各种计算机程序中。动态数组可以轻松实现栈和队列,从而简化了它们的实现。
#### 3.1.1 栈的实现
栈是一种后进先出(LIFO)的数据结构。它遵循以下规则:
- **入栈(push):**将元素添加到栈顶。
- **出栈(pop):**从栈顶移除元素。
使用动态数组实现栈非常简单,我们可以将动态数组视为栈的底层存储结构。
```cpp
class Stack {
private:
DynamicArray<int> arr;
public:
void push(int value) {
arr.add(value);
}
int pop() {
return arr.removeLast();
}
int peek() {
return arr.get(arr.size() - 1);
}
bool isEmpty() {
return arr.isEmpty();
}
};
```
**代码逻辑分析:**
- `push` 方法将元素添加到动态数组的末尾,模拟入栈操作。
- `pop` 方法从动态数组的末尾移除元素,模拟出栈操作。
- `peek` 方法返回动态数组末尾的元素,表示栈顶元素。
- `isEmpty` 方法检查动态数组是否为空,表示栈是否为空。
#### 3.1.2 队列的实现
队列是一种先进先出(FIFO)的数据结构。它遵循以下规则:
- **入队(enqueue):**将元素添加到队列尾部。
- **出队(dequeue):**从队列头部移除元素。
使用动态数组实现队列也同样简单,我们可以将动态数组视为队列的底层存储结构。
```cpp
class Queue {
private:
DynamicArray<int> arr;
public:
void enqueue(int value) {
arr.add(value);
}
int dequeue() {
return arr.removeFirst();
}
int peek() {
return arr.get(0);
}
bool isEmpty() {
return arr.isEmpty();
}
};
```
**代码逻辑分析:**
- `enqueue` 方法将元素添加到动态数组的末尾,模拟入队操作。
- `dequeue` 方法从动态数组的头部移除元素,模拟出队操作。
- `peek` 方法返回动态数组头部的元素,表示队列头元素。
- `isEmpty` 方法检查动态数组是否为空,表示队列是否为空。
### 3.2 哈希表
哈希表是一种高效的数据结构,用于快速查找和检索数据。它将键映射到值,并使用哈希函数将键转换为存储位置。
#### 3.2.1 哈希函数的设计
哈希函数是哈希表中至关重要的组件。它将键转换为一个唯一的哈希值,用于确定键在哈希表中的位置。一个好的哈希函数应满足以下条件:
- **均匀分布:**哈希值应均匀分布在哈希表的整个范围内。
- **快速计算:**哈希函数应快速计算,以避免性能瓶颈。
- **抗碰撞:**哈希函数应尽量避免产生哈希碰撞,即两个不同的键映射到同一个哈希值。
#### 3.2.2 冲突处理
哈希碰撞是不可避免的,因此哈希表需要提供冲突处理机制。常见的冲突处理方法包括:
- **线性探测:**从哈希值开始,依次探测哈希表中的位置,直到找到一个空位置或已存在的键。
- **二次探测:**使用二次探测函数,从哈希值开始,以特定步长探测哈希表中的位置。
- **链地址法:**将具有相同哈希值的键存储在链表中,并使用链表来解决冲突。
# 4.1 二叉树
### 4.1.1 二叉树的表示
**定义:**二叉树是一种非线性数据结构,它由一个根节点和一组子节点组成。每个子节点最多有两个子节点,称为左子节点和右子节点。
**表示方式:**二叉树可以通过以下方式表示:
- **递归表示:**使用一个递归数据结构,其中每个节点包含一个值和两个指向其子节点的指针。
- **数组表示:**使用一个数组来存储二叉树的节点,其中数组的索引对应于节点在树中的位置。
- **链表表示:**使用一个链表来存储二叉树的节点,其中每个节点包含一个值和两个指向其子节点的指针。
**数组表示示例:**
```java
int[] arr = {1, 2, 3, 4, 5, 6, 7};
```
在这个数组中,索引为 0 的元素是根节点,索引为 1 和 2 的元素分别是左子节点和右子节点。以此类推,索引为 3 和 4 的元素是左子树的左子节点和右子节点,索引为 5 和 6 的元素是右子树的左子节点和右子节点。
### 4.1.2 二叉树的遍历
**遍历:**遍历二叉树是指访问树中的所有节点。有三种常见的遍历方式:
- **前序遍历:**根节点 -> 左子树 -> 右子树
- **中序遍历:**左子树 -> 根节点 -> 右子树
- **后序遍历:**左子树 -> 右子树 -> 根节点
**代码示例(前序遍历):**
```java
public static void preOrder(Node root) {
if (root == null) {
return;
}
System.out.println(root.value);
preOrder(root.left);
preOrder(root.right);
}
```
**逻辑分析:**
* 函数 `preOrder` 采用递归的方式遍历二叉树。
* 如果根节点为空,则返回。
* 否则,打印根节点的值。
* 递归调用 `preOrder` 函数遍历左子树。
* 递归调用 `preOrder` 函数遍历右子树。
**参数说明:**
* `root`:要遍历的二叉树的根节点。
# 5. 动态数组的工程实践
### 5.1 性能优化
#### 5.1.1 内存分配优化
**问题:**
动态数组在扩容时需要重新分配内存,频繁的内存分配会带来性能开销。
**优化策略:**
* **使用内存池:**预先分配一定大小的内存池,在需要分配内存时直接从内存池中获取,避免频繁的系统内存分配。
* **按需分配:**根据实际需要逐步分配内存,而不是一次性分配大量内存。
* **提前预留空间:**在扩容时,预留一定的空间,避免频繁的扩容操作。
#### 5.1.2 时间复杂度优化
**问题:**
动态数组的某些操作,如插入、删除元素,时间复杂度为 O(n)。
**优化策略:**
* **使用链表:**对于需要频繁插入和删除元素的场景,使用链表可以将时间复杂度降低到 O(1)。
* **使用平衡树:**对于需要频繁查找和更新元素的场景,使用平衡树可以将时间复杂度降低到 O(log n)。
* **分块管理:**将数组划分为多个块,每个块包含一定数量的元素。在进行插入或删除操作时,只操作当前块,避免遍历整个数组。
### 5.2 异常处理
#### 5.2.1 内存不足异常
**问题:**
当系统内存不足时,动态数组的扩容操作可能会失败。
**处理策略:**
* **捕获异常:**在扩容操作中捕获内存不足异常,并进行相应的处理。
* **使用内存池:**通过使用内存池,可以减少内存分配的频率,从而降低内存不足异常发生的概率。
* **限制数组大小:**设置动态数组的最大大小,避免分配过大的数组。
#### 5.2.2 数组越界异常
**问题:**
当访问数组元素时,如果索引超出数组范围,会引发数组越界异常。
**处理策略:**
* **边界检查:**在访问数组元素之前,进行边界检查,确保索引在数组范围内。
* **使用哨兵值:**在数组末尾添加一个哨兵值,当访问到哨兵值时,表示已超出数组范围。
* **使用异常处理:**捕获数组越界异常,并进行相应的处理,如返回错误信息或终止程序。
### 代码示例
**内存分配优化:使用内存池**
```c++
class MemoryPool {
public:
MemoryPool(size_t size) {
buffer_ = new char[size];
free_head_ = buffer_;
free_size_ = size;
}
~MemoryPool() {
delete[] buffer_;
}
void* allocate(size_t size) {
if (size > free_size_) {
return nullptr;
}
void* ptr = free_head_;
free_head_ += size;
free_size_ -= size;
return ptr;
}
private:
char* buffer_;
char* free_head_;
size_t free_size_;
};
int main() {
MemoryPool pool(1024);
int* arr = (int*)pool.allocate(sizeof(int) * 100);
// ...
}
```
**时间复杂度优化:使用链表**
```c++
struct Node {
int data;
Node* next;
};
class LinkedList {
public:
LinkedList() {
head_ = nullptr;
tail_ = nullptr;
}
void insert(int data) {
Node* new_node = new Node{data, nullptr};
if (head_ == nullptr) {
head_ = new_node;
tail_ = new_node;
} else {
tail_->next = new_node;
tail_ = new_node;
}
}
int get(int index) {
Node* curr = head_;
for (int i = 0; i < index; i++) {
curr = curr->next;
}
return curr->data;
}
void remove(int index) {
Node* curr = head_;
Node* prev = nullptr;
for (int i = 0; i < index; i++) {
prev = curr;
curr = curr->next;
}
if (prev == nullptr) {
head_ = curr->next;
} else {
prev->next = curr->next;
}
delete curr;
}
private:
Node* head_;
Node* tail_;
};
int main() {
LinkedList list;
list.insert(1);
list.insert(2);
list.insert(3);
// ...
}
```
# 6.1 并行数组
### 6.1.1 并行数组的原理
并行数组是一种数据结构,它允许在多个处理器或线程上并行处理数据。与传统数组不同,并行数组将数据元素分布在多个处理器或线程的内存中,从而实现并行计算。
并行数组的实现通常基于共享内存模型,其中所有处理器或线程都可以访问同一块内存。每个处理器或线程负责处理分配给它的数据元素,并通过同步机制协调它们的访问。
### 6.1.2 并行数组的应用
并行数组在需要大量数据处理的应用中特别有用,例如:
- **科学计算:**并行数组可以用于并行化科学计算中的矩阵运算、求解偏微分方程等任务。
- **图像处理:**并行数组可以用于并行化图像处理中的图像滤波、图像增强等任务。
- **机器学习:**并行数组可以用于并行化机器学习中的训练模型、预测等任务。
### 代码示例
以下是一个并行数组的简单示例,它使用 OpenMP 库在多个线程上并行计算数组元素的和:
```cpp
#include <omp.h>
#include <stdio.h>
int main() {
// 创建一个并行数组
int arr[100000];
for (int i = 0; i < 100000; i++) {
arr[i] = i;
}
// 使用 OpenMP 并行化数组元素的求和
int sum = 0;
#pragma omp parallel for reduction(+:sum)
for (int i = 0; i < 100000; i++) {
sum += arr[i];
}
// 打印结果
printf("并行数组元素的和:%d\n", sum);
return 0;
}
```
在上面的示例中,`#pragma omp parallel for reduction(+:sum)` 指令将循环并行化,并使用 `reduction` 子句将每个线程的局部和累加到 `sum` 变量中。
0
0
相关推荐







