Java最小公倍数算法的代码重构:面向对象与设计模式,提升代码质量
发布时间: 2024-08-27 19:13:51 阅读量: 11 订阅数: 11 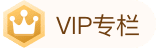
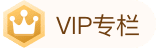
# 1. Java最小公倍数算法概述**
最小公倍数(LCM)是两个或多个整数的最小公倍数。在Java中,我们可以使用多种算法来计算LCM,包括辗转相除法和质因数分解法。
在辗转相除法中,我们不断地将较大的数除以较小的数,并将余数作为新的较小的数。当余数为0时,较大的数就是LCM。这种方法的优点是简单易懂,但对于大数可能会比较耗时。
质因数分解法是将每个整数分解为其质因数,然后将每个质因数的最高幂相乘。所得乘积就是LCM。这种方法的优点是速度快,但对于包含大量质因数的整数可能比较复杂。
# 2. 面向对象设计与代码重构
### 2.1 面向对象编程原则
面向对象编程(OOP)是一门编程范式,它将数据和方法组织成对象。OOP 原则指导着对象的设计和交互,以提高代码的可维护性、可扩展性和可重用性。
**2.1.1 封装性**
封装性是指将数据和方法封装在对象中,使其对外部代码不可见。这有助于保护数据免受意外修改,并提高代码的可维护性。
**2.1.2 继承性**
继承性允许子类继承父类的属性和方法。这有助于代码重用,并允许创建层次结构化的对象。
**2.1.3 多态性**
多态性允许子类以不同的方式实现父类的方法。这提供了代码的灵活性,并允许创建通用接口。
### 2.2 设计模式应用
设计模式是经过验证的解决方案,用于解决常见的软件设计问题。它们提供了一种重用最佳实践的方法,并提高代码的可维护性和可扩展性。
**2.2.1 工厂模式**
工厂模式创建对象而不指定其具体类。这有助于解耦代码,并允许在运行时动态创建对象。
```java
// 工厂类
public class Factory {
public static Shape getShape(String shapeType) {
if (shapeType.equals("CIRCLE")) {
return new Circle();
} else if (shapeType.equals("RECTANGLE")) {
return new Rectangle();
} else {
return null;
}
}
}
// 形状接口
public interface Shape {
void draw();
}
// 圆形类
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a circle");
}
}
// 矩形类
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a rectangle");
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
Shape circle = Factory.getShape("CIRCLE");
circle.draw();
Shape rectangle = Factory.getShape("RECTANGLE");
rectangle.draw();
}
}
```
**2.2.2 策略模式**
策略模式允许算法或行为在运行时动态切换。这提供了代码的灵活性,并允许在不同的场景中使用不同的算法。
```java
// 策略接口
public interface Strategy {
int doOperation(int num1, int num2);
}
// 加法策略
public class AdditionStrategy implements Strategy {
@Override
public int doOperation(int num1, int num2) {
return num1 + num2;
}
}
// 减法策略
public class SubtractionStrategy implements Strategy {
@Override
public int doOperation(int num1, int num2) {
return num1 - num2;
}
}
// 乘法策略
public class MultiplicationStrategy implements Strategy {
@Override
public int doOperation(int num1, int num2) {
return num1 * num2;
}
}
```
0
0
相关推荐
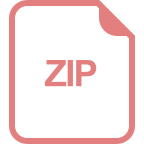
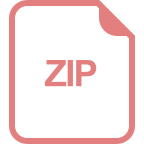
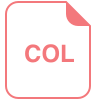
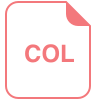
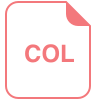
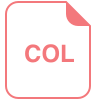

