【iOS UI布局优化】:数据结构与算法的应用平衡术
发布时间: 2024-09-10 00:05:22 阅读量: 318 订阅数: 28 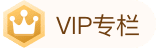
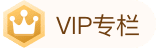
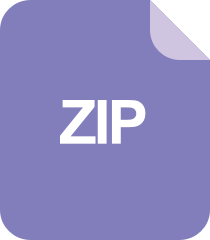
IOS应用源码之BabyDateCounterPro.zip
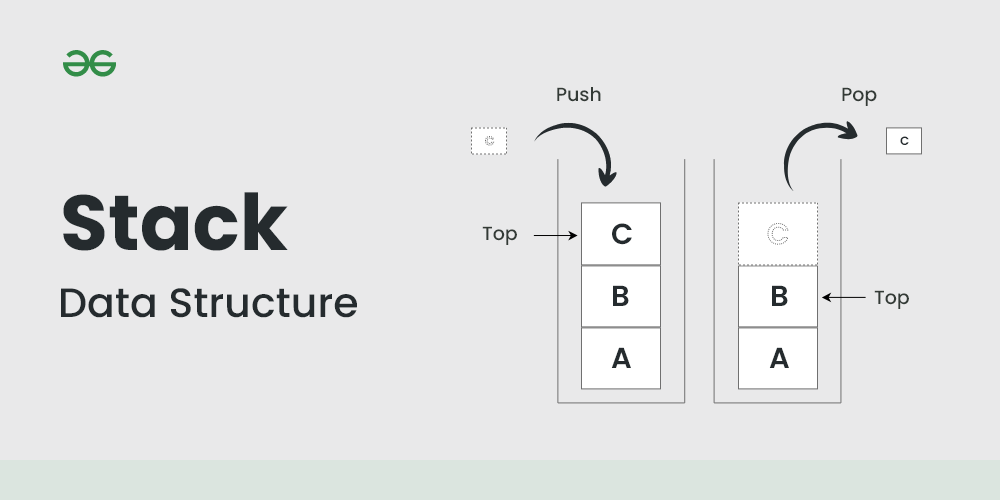
# 1. iOS UI布局优化概述
## 1.1 UI布局的重要性
在移动应用开发中,用户界面(UI)的布局对于用户体验至关重要。良好的布局可以提供流畅的交互和视觉效果,而不良的布局可能导致应用反应迟缓甚至崩溃。随着应用界面越来越复杂,优化UI布局成为了提高性能和用户满意度的关键任务。
## 1.2 UI布局优化的目标
优化UI布局的核心目标是减少渲染时间和提高响应速度。这涉及到降低CPU和GPU的负担,减少内存使用,并优化布局结构以避免布局重排和重绘。良好的布局优化能够显著提升应用的性能指标,如帧率(FPS)和应用启动时间。
## 1.3 优化的基本原则
布局优化通常遵循几个基本原则:最小化布局层级、避免过度绘制、使用合适的布局容器以及在适当的时候使用自动布局(Constraints)。开发者需要对布局进行精确控制,同时避免过多的布局约束导致的性能开销。
在接下来的章节中,我们将详细讨论如何应用数据结构和算法来优化UI布局,以及如何利用性能分析工具来诊断和解决性能问题。通过实战案例分析,我们将探究在实际开发中如何将理论应用到实践中。
# 2. 数据结构在UI布局中的应用
## 核心数据结构介绍
### 栈、队列和链表在布局中的作用
在iOS UI布局中,数据结构的选择对性能有着直接影响。栈(Stack)、队列(Queue)和链表(LinkedList)等基础数据结构在视图层次管理和布局计算中扮演着重要角色。
栈是一种后进先出(LIFO)的数据结构,非常适合用于管理视图层级关系,比如在处理视图的添加和移除时,可以通过栈来维持视图的层级状态。当一个视图需要被添加到屏幕上时,它被压入栈中,当视图被移除时,则从栈中弹出。这种后进先出的特性保证了视图层级的准确和高效管理。
队列是一种先进先出(FIFO)的数据结构,在处理UI元素的顺序操作时非常有用。例如,当需要对一系列视图进行动画处理时,队列可以帮助开发者维护一个准确的动画执行顺序。每个视图或动画任务入队列,按照先进先出的顺序被处理,确保了动画的流畅和同步。
链表则是一种可以用来存储视图对象序列的数据结构,链表的每个节点包含了数据本身以及指向下一个节点的引用。在视图的列表滚动显示场景中,链表的动态添加和删除操作比数组更加高效,因为数组在动态变化时可能需要移动大量元素,而链表则不需要。这一点对于优化长列表的滚动性能至关重要。
下面是一个链表数据结构在Swift中的简单实现:
```swift
class ListNode {
var value: UIView
var next: ListNode?
init(_ value: UIView) {
self.value = value
self.next = nil
}
}
class LinkedList {
var head: ListNode?
func append(_ value: UIView) {
let newNode = ListNode(value)
if let currentHead = head {
var currentNode = currentHead
while let nextNode = currentNode.next {
currentNode = nextNode
}
currentNode.next = newNode
} else {
head = newNode
}
}
func iterateViews() {
var currentNode = head
while let node = currentNode {
// 这里可以对node.value进行操作,例如更新UI等
currentNode = node.next
}
}
}
```
链表节点类`ListNode`包含了一个视图对象和一个指向下一个节点的引用。链表类`LinkedList`提供了添加视图到链表和迭代链表视图的方法。在处理视图列表时,使用链表可以显著提高插入和删除操作的性能。
### 树结构在视图层次管理中的应用
树形结构在iOS UI布局中同样扮演着关键的角色,其中最重要的是视图层次树。在视图层次树中,每个节点代表一个视图对象,节点之间的父子关系代表了视图之间的层次关系。在iOS开发中,开发者几乎每天都会和树结构打交道,如通过`view.subviews`获取子视图列表,或者使用`addSubview`方法将子视图添加到父视图中。
树结构之所以适合用于管理视图层次,是因为它的层次分明和易于扩展的特性。视图的层次管理在iOS中是递归进行的,每个视图都可能包含自己的子视图,子视图又可以包含更多的子视图,形成了一棵嵌套的视图层次树。
除了视图层次树,另外一个与树结构紧密相关的例子是元素的布局约束。在Auto Layout中,约束之间往往存在复杂的依赖关系,这种关系可以抽象为一个有向无环图(DAG),而DAG本质上就是一种树结构。
在视图层次树中,节点的添加和移除操作是核心操作之一。当开发者将一个视图添加为另一个视图的子视图时,实际上是将新的节点作为父节点的子节点添加到树结构中。树结构提供了有效的遍历算法,如深度优先搜索(DFS)和广度优先搜索(BFS),这些算法在查找视图、更新视图层次或应用布局约束时非常有用。
下面是一个简单的视图层次树的Swift实现示例:
```swift
class ViewNode {
var view: UIView
var children: [ViewNode] = []
init(_ view: UIView) {
self.view = view
}
func addChild(_ child: ViewNode) {
children.append(child)
}
func printHierarchy(level: Int = 0) {
let indent = String(repeating: " ", count: level * 2)
print(indent + view.description)
for child in children {
child.printHierarchy(level: level + 1)
}
}
}
```
在这个`ViewNode`类中,每个节点代表一个视图对象,并且包含一个子视图数组。`addChild`方法允许开发者将子节点添加到当前节点中,而`printHierarchy`方法则用于打印树的结构,便于开发者查看视图之间的层级关系。
利用树形结构可以有效地管理视图层级关系,同时对视图进行递归的布局计算和更新。树形结构的管理与遍历,是理解iOS UI布局优化不可或缺的一部分。通过深入理解树形结构在视图层次管理中的应用,开发者可以更好地优化布局性能,并解决复杂的布局问题。
# 3. 性能分析工具与优化策略
## 3.1 使用性能分析工具
### 3.1.1 Xcode内置的性能分析工具介绍
Xcode作为Apple官方提供的集成开发环境,其中内置了多个性能分析工具,可以帮助开发者找到应用中的性能瓶颈并进行优化。其中最著名的工具之一是Instruments,它是Xcode的重要组成部分,它提供了一系列性能监控模板,可以用来监控CPU使用率、内存分配、网络活动、文件系统活动等
0
0
相关推荐
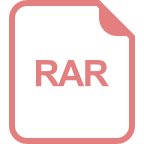
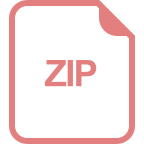
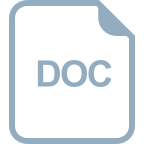
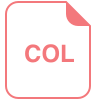
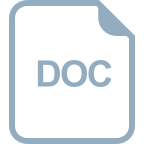
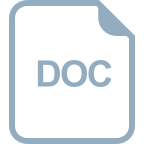
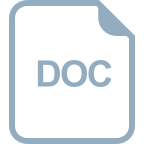
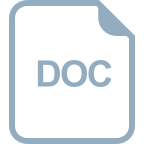