【iOS内存优化技巧】:算法与数据结构的双重效能
发布时间: 2024-09-10 00:08:12 阅读量: 23 订阅数: 26 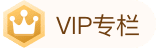
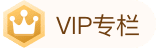
.png)
# 1. iOS内存优化的重要性与挑战
## 1.1 为何内存优化至关重要
在iOS开发中,良好的内存管理对于保持应用性能、稳定性和响应性至关重要。资源受限的移动设备对内存的使用效率提出了更高的要求,内存优化可以帮助避免应用崩溃和卡顿,同时提升用户体验。随着应用功能的丰富和复杂性的增加,内存优化的挑战也在相应增大。
## 1.2 内存优化面临的挑战
内存优化过程中可能会遇到多方面的挑战。例如,内存泄漏问题难以追踪和修复,尤其是在复杂的系统中;高效利用内存同时要保证代码的清晰和维护性;以及各种新硬件和操作系统更新带来的内存管理策略变化,都对开发者提出了更高的要求。理解并克服这些挑战,是提升应用品质的关键。
## 1.3 内存优化的长期价值
良好的内存管理不仅能够提高当前应用的性能,还有助于应用的长期可持续发展。随着用户设备的多样性,应用需要在不同配置的设备上提供良好的体验,这需要开发者在设计和编码阶段就考虑内存优化。长远来看,有效的内存管理能够减少服务器负载,降低维护成本,甚至有可能延长应用的生命周期。
# 2. 内存优化基础理论
### 2.1 内存管理的基本概念
#### 2.1.1 内存泄漏的成因与识别
内存泄漏是指程序中已分配的内存由于某些原因未被释放而造成的资源浪费。在iOS开发中,内存泄漏是导致应用性能下降和崩溃的主要原因之一。识别内存泄漏通常需要借助专业的工具,如Xcode内置的Instruments。内存泄漏的常见成因包括但不限于循环引用、未正确释放对象、使用僵尸对象等。
循环引用是由于两个或多个对象相互保持对方的强引用,使得即使它们不再需要,也无法被释放。使用弱引用(weak reference)是解决循环引用问题的有效方法。而未正确释放对象通常发生在手动内存管理的代码中,错误地使用`alloc`、`retain`、`release`或`autorelease`等方法。
```objective-c
// 示例:内存泄漏的场景
// 假设有一个类A和B,相互持有对方的强引用
@interface A : NSObject
@property (strong) B *b;
@end
@interface B : NSObject
@property (strong) A *a;
@end
// 在这种情况下,即使没有其他地方引用A或B,它们也不会被释放
```
通过Xcode的Instruments工具中的Allocations功能,开发者可以捕获这些泄漏,并采取措施解决。
#### 2.1.2 引用计数机制详解
在Objective-C中,引用计数机制用于追踪对象的拥有者数量。每当一个新的拥有者引用对象时,对象的引用计数就会增加;当拥有者放弃引用时,引用计数减少。当引用计数为零时,对象会被自动释放。
Objective-C的对象使用引用计数作为主要的内存管理机制,有如下几个关键点:
- 当创建一个新对象时,它会有一个初始引用计数为1。
- 当一个对象被另一个对象通过strong属性引用时,它的引用计数会增加。
- 当一个对象不再被使用,并且被释放时,它的引用计数会减少。
- 当对象的引用计数降至0时,系统会自动调用对象的`dealloc`方法来释放该对象。
```objective-c
// 示例:引用计数操作
MyObject *myObject = [[MyObject alloc] init]; // 引用计数1
myObject = nil; // 引用计数减少1,现在计数为0,对象被释放
```
### 2.2 数据结构对内存效率的影响
#### 2.2.1 常用数据结构的内存开销对比
在选择数据结构时,内存开销是一个重要的考量因素。不同数据结构的内存占用大小不同,例如,数组、字典、链表、树和图等。数组通过连续内存空间存储数据,其内存分配相对简单,但在插入或删除元素时可能会导致内存拷贝。字典通常需要额外的空间来存储键值对应的索引,链表则通过节点间指针连接,每个节点都需要额外的内存来存储指针信息。树和图作为复杂的数据结构,在实际应用中会根据其具体实现的不同而有不同的内存占用。
下面是一个简单的表格,对比了几种基本数据结构的内存开销情况:
| 数据结构 | 内存开销描述 | 适用场景 |
|----------|--------------|----------|
| 数组 | 连续内存存储,插入删除可能引发内存拷贝 | 数据量固定且访问频繁 |
| 字典 | 需要额外空间存储键值索引 | 快速查找和键值存储 |
| 链表 | 每个节点包含数据和指针,额外内存 | 插入删除操作频繁,数据量不固定 |
| 树 | 非平衡树可能造成偏斜,内存使用不均匀 | 需要快速排序和查找的场景 |
| 图 | 节点和边均需存储,内存占用相对较大 | 表示复杂关系和网络结构 |
#### 2.2.2 选择合适数据结构的策略
在编程实践中,选择最合适的数据结构对于内存优化至关重要。选择策略应该基于以下几个考虑:
1. 数据访问模式:如果需要频繁地通过键值访问数据,字典可能是最佳选择;如果数据量固定且顺序重要,则数组可能更为合适。
2. 插入与删除频率:在插入和删除操作频繁的场景下,链表或平衡树可能更加适合。
3. 内存占用:在内存有限的情况下,应尽量选择内存占用小的数据结构。
4. 算法复杂度:不同的数据结构在查找、排序和插入等操作中表现不同,应根据实际需求选取。
以下是一个决策树的Mermaid流程图,用于指导开发者根据使用场景选择合适的数据结构:
```mermaid
graph TD
A[开始选择数据结构] --> B[数据量是否固定?]
B -- 是 --> C[是否需要频繁的查找操作?]
B -- 否 --> D[链表]
C -- 是 --> E[字典]
C -- 否 --> F[数组]
E --> G[结束选择]
F --> G
D --> G
```
### 2.3 编码实践中的内存优化原则
#### 2.3.1 避免重复创建和释放内存
在编写代码时,应尽量减少不必要的对象创建和释放操作。每次创建对象都会消耗内存并带来性能开销,尤其是在循环或者频繁调用的代码块中。一种常见的做法是预先分配一定量的对象,并在使用后进行重置。这种方法可以减少内存分配的次数,降低内存碎片化的风险,提高应用的运行效率。
以下代码块展示了如何在循环中避免重复创建对象:
```objective-c
// 创建一个可重用的对象池
NSMutableArray *objectPool = [[NSMutableArray alloc] init];
// 在循环中使用对象池来获取对象
for (int i = 0; i < 1000; i++) {
MyObject *myObject = [objectPool lastObject];
if (!myObject) {
myObject = [[MyObject alloc] init];
}
// 使用对象进行操作...
[objectPool addObject:myObject];
}
// 对象使用完毕后,重置对象池
[objectPool removeAllObjects];
```
#### 2.3.2 实现对象的重用与池化
对象池化是一种通过重用已存在的对象来减少内存分配和回收开销的优化策略。它可以显著提高性能,特别是在需要创建大量临时对象的场景下。实现对象池通常需要一个容器来存储未使用的对象,并提供方法来获取或释放对象。正确实现对象池化需要考虑线程安全性、对象生命周期管理和池的大小等因素。
```objective-c
// 对象池化类的简单实现示例
@interface MyObjectPool : NSObject
- (MyObject *)popObject; // 从池中获取对象
- (void)pushObject:(MyObject *)object; // 将对象回收到池中
@end
@implementation MyObjectPool
{
NSMutableArray *pool;
}
- (instancetype)init {
if (self = [super init]) {
pool = [[NSMutableArray alloc] init];
}
return self;
}
- (MyObject *)popObject {
MyObject *obj = [pool lastObject];
[pool removeLastObject];
```
0
0
相关推荐
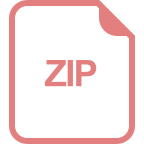
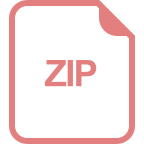
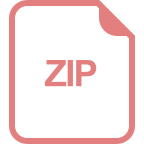





