【iOS安全实践】:数据结构与算法在算法安全中的应用
发布时间: 2024-09-09 23:54:39 阅读量: 45 订阅数: 28 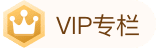
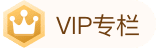
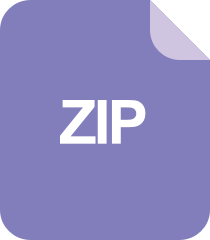
iOS算法:iOS数据结构算法
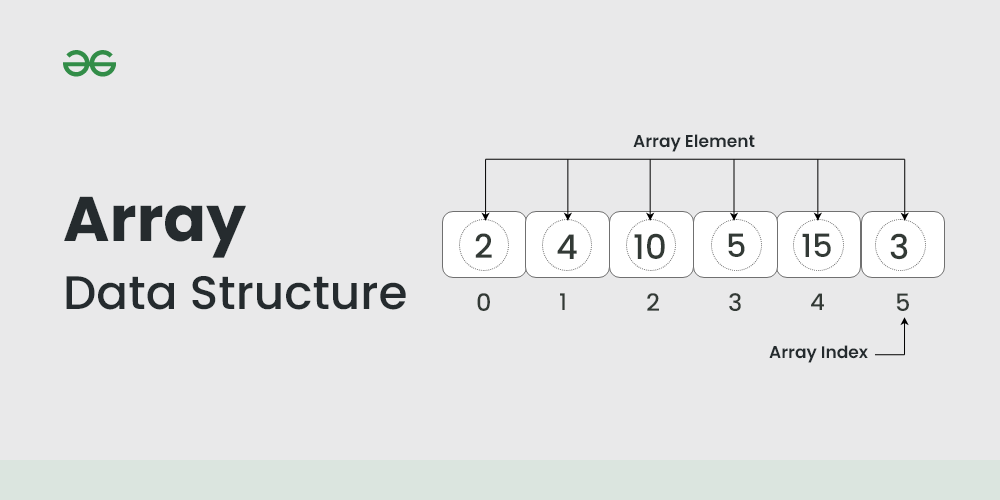
# 1. iOS安全概述与数据结构基础
## 1.1 安全性的核心地位
随着智能手机的普及,iOS设备的安全性问题也日益凸显。在移动应用中,数据泄露、恶意软件攻击等安全事件频发,这不仅损害了用户权益,也威胁到企业的品牌形象。因此,掌握iOS安全基础,构建稳固的数据结构,对于保障应用和用户数据的安全至关重要。
## 1.2 数据结构的重要性
数据结构是组织和存储数据的方式,它对应用程序的性能、内存使用和数据安全性有着直接的影响。良好的数据结构设计可以帮助开发者更高效地访问数据,提高应用运行效率,同时降低内存消耗和潜在的安全风险。在iOS开发中,合理地使用数组、字典、队列等数据结构,对于安全地管理和处理信息至关重要。
## 1.3 安全性与数据结构的融合
在iOS平台,数据结构的使用与安全性紧密相连。例如,使用加密的字典来安全地存储敏感信息,或者构建自定义的链表结构来处理复杂的业务逻辑同时保证数据的完整性。开发者不仅需要理解各种数据结构的特点和优势,还需要了解它们在不同安全场景下的应用,以确保开发出安全且高效的iOS应用。
# 2. 核心算法在iOS安全中的应用
## 2.1 哈希算法与iOS安全
### 2.1.1 哈希算法原理及分类
哈希算法是信息安全领域的基础工具,它将任意长度的输入(又称为预映射或消息)通过哈希函数转换成固定长度的输出,这一输出称为哈希值。哈希算法具有以下特性:确定性,相同的输入必然产生相同的输出;高效性,从输入计算出哈希值是快速的;不可逆性,无法从哈希值推导出原始数据;抗碰撞性,找到不同的输入使得它们具有相同的哈希值在计算上是不可行的。
哈希算法可以分为两类:一类是加密哈希函数,另一类是非加密哈希函数。加密哈希函数用于加密,它具有较强的抗碰撞性。非加密哈希函数则主要用于数据查找和检测数据完整性。iOS安全中的典型应用为MD5、SHA-1和SHA-256等。
### 2.1.2 哈希算法在iOS安全中的实践
在iOS平台,哈希算法被广泛应用于数据完整性验证、密码存储和网络通信等领域。例如,通过SHA-256算法可以对敏感数据进行加密存储,从而保护用户密码等信息安全。此外,在iOS应用中,哈希算法还可以用于验证下载的文件是否被篡改,通过比较文件的哈希值来确认文件的完整性。
```swift
import CommonCrypto
// 使用SHA-256算法进行哈希计算
func sha256(message: String) -> String {
guard let data = message.data(using: .utf8) else { return "" }
var hash = [UInt8](repeating: 0, count: Int(CC_SHA256_DIGEST_LENGTH))
data.withUnsafeBytes {
_ = CC_SHA256($0.baseAddress, UInt32(data.count), &hash)
}
return hash.map { String(format: "%02hhx", $0) }.joined()
}
// 调用示例
let message = "Hello, iOS Security!"
let messageDigest = sha256(message: message)
print("SHA-256 digest: \(messageDigest)")
```
以上代码展示了如何在Swift语言中使用`CommonCrypto`库计算一个字符串的SHA-256哈希值。Swift代码块后附有简单的逻辑分析,指出了需要导入的库、处理字符串为数据、调用底层的C函数`CC_SHA256`来执行哈希计算,并将结果格式化为十六进制字符串。
## 2.2 加密算法与iOS安全
### 2.2.1 对称与非对称加密原理
对称加密算法中,加密和解密使用同一个密钥,常用的算法包括AES、DES和RC4。这类算法加密速度快,但密钥分发存在安全隐患。与之相对的是非对称加密,使用一对密钥:一个公钥用于加密,一个私钥用于解密。公钥可以公开,私钥必须保密。RSA算法是典型的非对称加密算法。
在iOS开发中,`CommonCrypto`、`Security`框架提供了对对称和非对称加密算法的支持。开发者可以利用这些框架来实现数据的加密传输和安全存储。
### 2.2.2 加密算法在iOS安全中的应用案例
在iOS应用开发中,对称加密算法常用于本地数据加密。例如,存储用户敏感数据时,可以使用AES算法对数据进行加密,并使用设备的密钥链(Keychain)来安全地存储密钥。非对称加密则可以用于SSL/TLS协议,保证数据在传输过程中的安全。
```swift
import Security
// 使用RSA加密数据
func encrypt RSA(message: String, publicKey: SecKey) -> Data? {
let messageData = Data(message.utf8)
var encryptedData: Unmanaged<SecData>?
let status = SecKeyEncrypt(publicKey, .pkcs1, messageData as NSData, &encryptedData)
if status == errSecSuccess {
return (encryptedData?.takeRetainedValue())!
} else {
return nil
}
}
// 加密过程的示例代码
let publicKey: SecKey = ... // 获取公钥
let message = "This is a secret message."
let encryptedData = encryptRSA(message: message, publicKey: publicKey)
print("Encrypted data: \(encryptedData)")
```
上述Swift示例代码展示了如何利用`Security`框架中的`SecKey`加密数据。它展示了利用非对称加密算法RSA加密消息,并解释了关键的函数调用和其参数。代码中还展示了在实际开发中如何处理加密结果。
## 2.3 数字签名与iOS安全
### 2.3.1 数字签名的工作机制
数字签名是一种用于确认消息完整性和来源的加密手段。它确保了消息在传输过程中没有被篡改,并且可以验证发送者的身份。数字签名通常结合哈希算法和非对称加密算法实现。发送者首先计算消息的哈希值,然后用私钥进行加密,生成签名附加到消息上。接收者收到消息后,使用发送者的公钥解密签名,与自己计算的哈希值进行比较,以此验证消息的完整性和来源。
### 2.3.2 数字签名在iOS中的实现与应用
在iOS中,数字签名可以用于验证应用更新的完整性,确保下载的更新没有被第三方篡改。还可以用于iOS应用内部数据的验证,如交易信息的确认。iOS提供了API来生成和验证数字签名,例如使用`SecKey`进行密钥操作和签名过程。
```swift
// 生成数字签名的示例
import Security
func sign(message: String, privateKey: SecKey) -> Data? {
let messageData = Data(message.utf8)
var signature: Unmanaged<SecData>?
let status = SecKeySign(privateKey, .pkcs1, messageData as NSData, &signature)
if status == errSecSuccess {
return (signature?.takeRetainedValue())!
} else {
return nil
}
}
// 使用示例
let privateKey: SecKey = ... // 获取私钥
let message = "This needs to be signed."
let signature = sign(message: message, privateKey: privateKey)
print("Digital signature: \(signature)")
```
示例代码展示了数字签名生成的过程,通过使用`SecKeySign`函数和私钥进行消息签名。在实际应用中,数字签名通常会和消息以及发送者的证
0
0
相关推荐







