递归阶乘的递归到非递归转换:代码实践与效率分析
发布时间: 2024-09-13 05:05:19 阅读量: 21 订阅数: 45 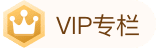
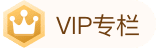
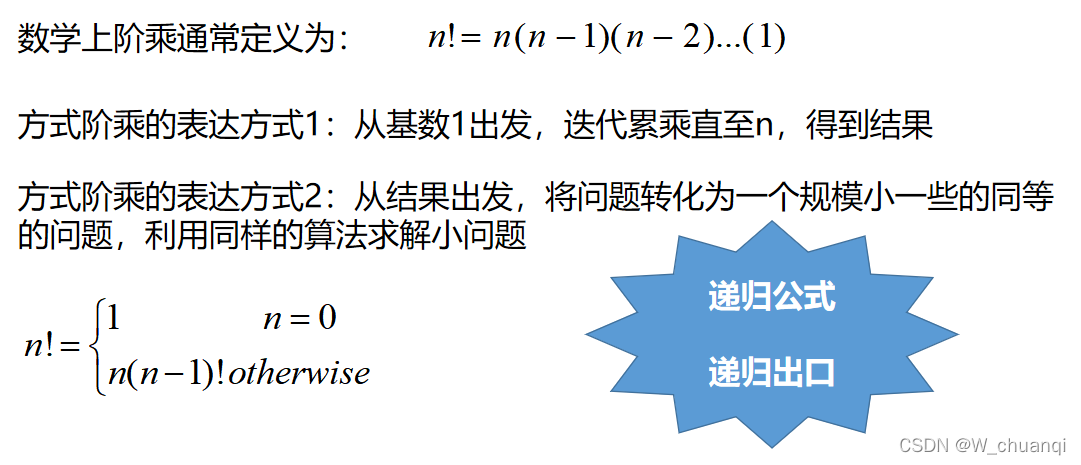
# 1. 递归阶乘的理论基础
## 1.1 递归概念解析
递归是一种编程技巧,它允许函数调用自身来解决问题。这一技术在处理具有自相似结构的问题时,例如树和图的遍历,或者数学上的阶乘计算中非常有用。理解递归的关键在于认识到它总是有一个明确的终止条件,也称为基准情况。
## 1.2 阶乘函数的定义
阶乘函数是一个典型的递归函数,对于非负整数n,其阶乘n!定义为从n乘到1的所有正整数的乘积。数学上可以表示为:n! = n * (n-1) * (n-2) * ... * 1。当n=0时,根据约定0! = 1。
## 1.3 递归思想在阶乘中的应用
通过递归思想,阶乘函数可以简单地定义为一个递归函数:f(n) = n * f(n-1),其中n是大于0的整数,而f(0) = 1。递归实现阶乘的关键在于理解递归函数如何将问题分解为更小的子问题,并最终达到基准情况。
```python
def factorial_recursive(n):
if n == 0:
return 1
else:
return n * factorial_recursive(n-1)
```
以上代码展示了如何用Python实现阶乘的递归函数。在实际使用时,用户只需提供一个整数参数n,函数便会返回其阶乘结果。
# 2. 递归算法的实现与优化
## 2.1 递归算法的基本原理
递归算法是一种通过函数自己调用自己来解决问题的编程技术。它是计算机科学中非常强大的算法设计手段,尤其适用于那些可以分解为多个子问题的场景。
### 2.1.1 递归定义与结构
递归的定义非常简单,即一个函数直接或间接调用自身。通常,一个递归函数包含两个主要部分:基本情况(Base Case)和递归步骤(Recursive Step)。基本情况通常是一个简单的问题实例,可以直接给出答案,而不需要进一步的递归调用。递归步骤则通过函数自身调用解决一个较小的问题实例,直到到达基本情况为止。
```mermaid
graph TD
A[Start] -->|Call Function| B[Check Base Case]
B --Yes--> C[Return Base Case Value]
B --No--> D[Calculate Subproblem]
D --> E[Call Function with Subproblem]
E --> B
```
### 2.1.2 递归函数的设计要点
设计递归函数时,需要确保每一步递归调用都能朝向基本情况前进,否则会形成无限递归,最终导致栈溢出错误。此外,递归函数应该被设计得尽可能简洁,以便于理解与维护。递归函数的设计也需考虑到效率,避免不必要的重复计算。
## 2.2 递归阶乘的代码实践
### 2.2.1 传统递归阶乘的实现
阶乘是递归应用的典型例子。一个数的阶乘表示所有小于或等于它的正整数的乘积。以下是使用递归实现阶乘函数的示例代码:
```python
def factorial(n):
# 基本情况
if n == 0:
return 1
# 递归步骤
else:
return n * factorial(n - 1)
print(factorial(5)) # 输出 120
```
这段代码首先检查基本情况(`n == 0`),此时函数返回1。否则,它会递归调用自身,每次将`n`减少1,直到`n`为0。每一层递归都等待其子递归计算完毕后,计算当前层的结果,并将结果传递回上一层,直至最终返回最终结果。
### 2.2.2 递归深度问题的分析
在实现递归算法时,一个需要特别关注的问题是递归深度。每个递归调用都会消耗一定的内存来保存当前状态,这个状态包括局部变量、参数以及返回地址等信息。当递归层次过深时,可能会达到调用栈的限制,导致`RecursionError`。
在Python中,可以使用`sys`模块的`setrecursionlimit`函数来设置最大递归深度。但需要注意的是,修改这个限制并不推荐,因为递归深度过深意味着会有较大的内存开销和运行时间。
```python
import sys
sys.setrecursionlimit(3000) # 设置递归深度为3000
```
## 2.3 递归算法的时间复杂度分析
### 2.3.1 递归调用栈的内存消耗
递归函数的每次调用都会增加调用栈的深度,从而增加内存的使用量。因为每次函数调用都需要在调用栈上保存状态,递归函数可能在栈上保存大量函数调用的信息,尤其在深度递归中,这一问题更为显著。
### 2.3.2 递归与非递归的时间对比
递归算法通常具有简单的逻辑,但是每次递归调用都会产生额外的开销。而非递归(迭代)算法一般在空间效率上更优,因为它们避免了额外的栈空间使用。
为了直观对比,可以观察阶乘函数的两种实现方式:递归和迭代。
递归实现:
```python
def recursive_factorial(n):
if n == 0:
return 1
else:
return n * recursive_factorial(n - 1)
```
迭代实现:
```python
def it
```
0
0
相关推荐
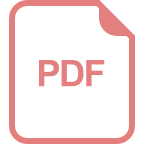
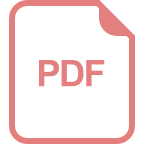
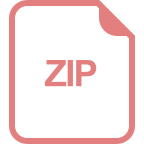





