使用TypeScript编写可测试的代码
发布时间: 2023-12-20 04:08:53 阅读量: 12 订阅数: 14 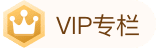
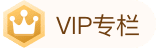
# 第一章:TypeScript简介
## 1.1 什么是TypeScript
## 1.2 TypeScript的优势和特点
## 1.3 TypeScript与JavaScript的关系
## 第二章:编写可测试的代码概述
### 第三章:TypeScript基础
在本章中,我们将介绍TypeScript的基本语法和数据类型,深入了解TypeScript的面向对象编程特性,并讨论如何在项目中集成TypeScript。
#### 3.1 TypeScript的基本语法和数据类型
TypeScript是JavaScript的超集,因此它继承了JavaScript的基本语法和数据类型。除此之外,TypeScript还引入了一些新的概念,比如类型注解和类型推断,让开发者能够更好地定义变量和函数的类型。
```typescript
// 基本数据类型
let isDone: boolean = false;
let num: number = 123;
let str: string = 'Hello, TypeScript';
// 数组类型
let arr: number[] = [1, 2, 3];
let arr2: Array<number> = [4, 5, 6];
// 元组类型
let x: [string, number];
x = ['TypeScript', 2021];
// 枚举类型
enum Color {Red, Green, Blue};
let c: Color = Color.Green;
// Any类型
let notSure: any = 4;
notSure = 'maybe a string instead';
// Void类型
function warnUser(): void {
console.log("This is a warning message");
}
// Null和Undefined类型
let u: undefined = undefined;
let n: null = null;
```
#### 3.2 TypeScript的面向对象编程特性
TypeScript支持传统的面向对象编程特性,包括类(Class)、接口(Interface)、继承(Inheritance)、多态(Polymorphism)等。我们可以使用这些特性来构建更加模块化和可维护的代码。
```typescript
// 类和继承
class Animal {
name: string;
constructor(theName: string) {
this.name = theName;
}
move(distanceInMeters: number = 0) {
console.log(`${this.name} moved ${distanceInMeters}m.`);
}
}
class Snake extends Animal {
constructor(name: string) { super(name); }
move(distanceInMeters = 5) {
console.log("Slithering...");
super.move(distanceInMeters);
}
}
// 接口
interface Shape {
name: string;
numberOfSides: number;
}
function draw(shape: Shape) {
console.log(`Drawing a ${shape.name} with ${shape.numberOfSides} sides`);
}
```
#### 3.3 如何在项目中集成TypeScript
要在项目中使用TypeScript,我们需要安装TypeScript编译器,并且将TypeScript代码编译成JavaScript代码。同时,我们可以利用配置文件`tsconfig.json`来指定编译选项和项目结构。
```bash
# 使用npm安装TypeScript编译器
npm install -g typescript
# 创建tsconfig.json配置文件
tsc --init
```
以上是对TypeScrip
0
0
相关推荐
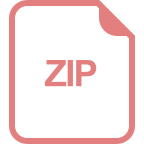
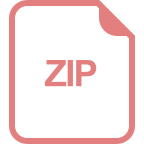
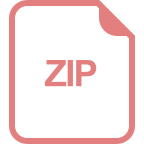
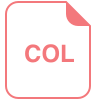
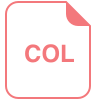
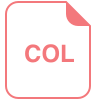
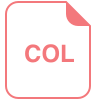

