Vue.js JSON数据处理指南:提升开发效率的5个实用技巧
发布时间: 2024-07-29 03:21:33 阅读量: 37 订阅数: 20 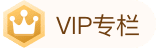
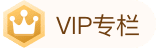
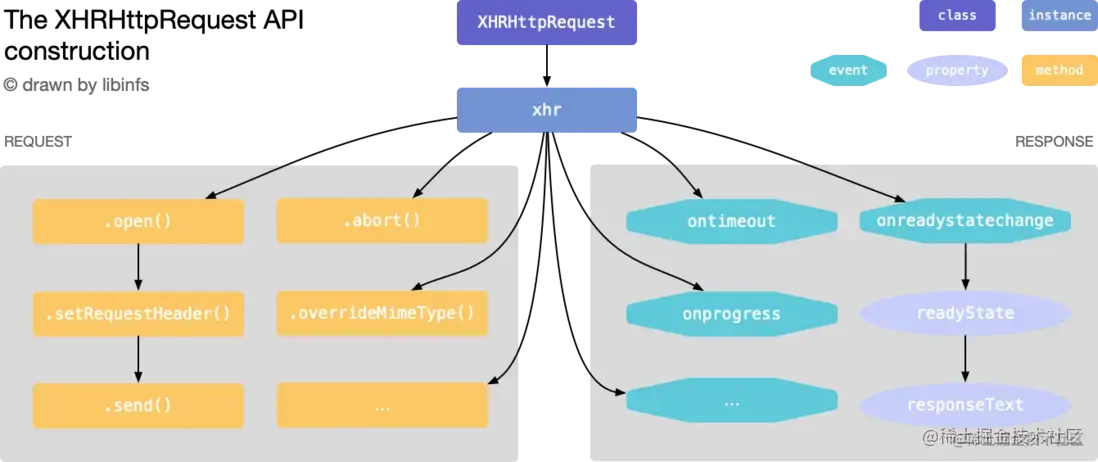
# 1. JSON 数据处理概述
JSON(JavaScript Object Notation)是一种轻量级的数据格式,用于在应用程序之间交换数据。在 Vue.js 中,JSON 数据处理是开发人员日常工作中不可或缺的一部分。
JSON 数据处理涉及到从服务器获取和处理 JSON 数据、使用 Vue.js 内置的功能进行数据转换和操作,以及管理 JSON 数据的状态。通过熟练掌握这些技巧,开发人员可以提高开发效率,并构建健壮且可维护的 Vue.js 应用程序。
# 2. Vue.js 中的 JSON 数据处理技巧
### 2.1 使用 v-model 进行双向数据绑定
v-model 是 Vue.js 中一个强大的指令,它允许在 HTML 元素和 Vue 实例的数据之间进行双向数据绑定。对于 JSON 数据,v-model 可以直接绑定到一个包含 JSON 对象的 data 属性,实现数据在组件和 HTML 之间的实时同步。
```html
<template>
<input v-model="formData.name">
</template>
<script>
export default {
data() {
return {
formData: {
name: '',
email: '',
age: ''
}
}
}
}
</script>
```
在这个示例中,`v-model` 将输入框中的值绑定到 `formData.name` 数据属性。当用户在输入框中输入内容时,`formData.name` 的值也会随之更新,反之亦然。
### 2.2 利用 computed 属性进行数据转换
computed 属性允许我们从现有的数据属性中计算出新的数据属性。对于 JSON 数据,computed 属性可以用来转换或格式化数据,以满足特定需求。
```html
<template>
<p>{{ fullName }}</p>
</template>
<script>
export default {
data() {
return {
formData: {
firstName: '',
lastName: ''
}
}
},
computed: {
fullName() {
return `${this.formData.firstName} ${this.formData.lastName}`
}
}
}
</script>
```
在这个示例中,`fullName` computed 属性从 `formData.firstName` 和 `formData.lastName` 中计算出 `fullName`。当 `formData.firstName` 或 `formData.lastName` 发生变化时,`fullName` 也会自动更新。
### 2.3 运用 methods 方法进行数据操作
methods 方法允许我们在 Vue 实例中定义可重用的函数。对于 JSON 数据,methods 方法可以用来执行各种数据操作,例如排序、过滤或验证。
```html
<template>
<button @click="sortData">排序数据</button>
</template>
<script>
export default {
data() {
return {
data: [
{ name: 'John', age: 30 },
{ name: 'Jane', age: 25 },
{ name: 'Bob', age: 40 }
]
}
},
methods: {
sortData() {
this.data.sort((a, b) => a.age - b.age)
}
}
}
</script>
```
在这个示例中,`sortData` 方法对 `data` 数组进行排序,并按年龄升序排列。当用户点击排序按钮时,`sortData` 方法就会被调用,并对数据进行排序。
# 3. JSON 数据处理的实践应用
### 3.1 从服务器获取和显示 JSON 数据
在 Vue.js 中,从服务器获取 JSON 数据是一个常见的操作。我们可以使用 `fetch` API 或第三方库(如 Axios)来发送 HTTP 请求并获取响应数据。
```javascript
// 使用 fetch API
fetch('https://example.com/api/data')
.then(response => response.json())
.then(data => {
// 使用 data
})
.catch(error => {
// 处理错误
});
```
```javascript
// 使用 Axios 库
axios.get('https://example.com/api/data')
.then(response => {
// 使用 response.data
})
.catch(error => {
// 处理错误
});
```
获取 JSON 数据后,我们可以使用 Vue.js 的响应式系统将其绑定到组件数据。
```javascript
<template>
<div>
<p>数据:{{ data }}</p>
</div>
</template>
<script>
export default {
data() {
return {
data: null
}
},
created() {
fetch('https://example.com/api/data')
.then(response => response.json())
.then(data => {
this.data = data;
})
.catch(error => {
// 处理错误
});
}
}
</script>
```
### 3.2 使用 Vuex 管理 JSON 数据状态
Vuex 是一个状态管理库,用于在 Vue.js 应用程序中管理全局状态。我们可以使用 Vuex 来管理 JSON 数据的状态,使其可以在多个组件中访问和修改。
```javascript
// store.js
import Vuex from 'vuex'
import Vue from 'vue'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
data: null
},
mutations: {
setData(state, data) {
state.data = data
}
},
actions: {
fetchData({ commit }) {
fetch('https://example.com/api/data')
.then(response => response.json())
.then(data => {
commit('setData', data)
})
.catch(error => {
// 处理错误
});
}
}
})
```
```javascript
// component.vue
<template>
<div>
<p>数据:{{ $store.state.data }}</p>
</div>
</template>
<script>
import { mapState } from 'vuex'
export default {
computed: {
...mapState(['data'])
},
created() {
this.$store.dispatch('fetchData')
}
}
</script>
```
### 3.3 通过表单提交处理 JSON 数据
在 Vue.js 中,我们可以使用表单提交来处理 JSON 数据。我们可以使用 `submit` 事件监听器来获取表单数据并将其转换为 JSON 格式。
```javascript
<template>
<form @submit.prevent="submitForm">
<input v-model="name" type="text" placeholder="姓名">
<input v-model="email" type="email" placeholder="邮箱">
<button type="submit">提交</button>
</form>
</template>
<script>
export default {
data() {
return {
name: '',
email: ''
}
},
methods: {
submitForm() {
const data = {
name: this.name,
email: this.email
}
// 将 data 转换为 JSON 格式
const json = JSON.stringify(data)
// 发送 HTTP 请求(例如使用 fetch 或 Axios)
fetch('https://example.com/api/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: json
})
.then(response => response.json())
.then(data => {
// 处理响应数据
})
.catch(error => {
// 处理错误
});
}
}
}
</script>
```
# 4. JSON 数据处理的进阶技巧
### 4.1 使用 Axios 库进行异步数据请求
**简介**
Axios 是一个用于在 Vue.js 中进行异步 HTTP 请求的流行库。它提供了简洁、易用的 API,可以轻松地发送 GET、POST、PUT 和 DELETE 请求。
**使用示例**
```javascript
import axios from 'axios';
const instance = axios.create({
baseURL: 'https://example.com/api',
timeout: 10000,
});
instance.get('/users')
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.log(error.message);
});
```
**参数说明**
| 参数 | 说明 |
|---|---|
| baseURL | 请求的基本 URL |
| timeout | 请求超时时间(毫秒) |
**代码逻辑分析**
* 创建一个 Axios 实例,设置基本 URL 和超时时间。
* 使用 `get()` 方法发送 GET 请求,并指定请求的 URL。
* 使用 `then()` 方法处理成功的响应,并打印响应数据。
* 使用 `catch()` 方法处理错误的响应,并打印错误消息。
### 4.2 使用 Lodash 库进行数据操作
**简介**
Lodash 是一个用于在 JavaScript 中进行数据操作的实用库。它提供了丰富的函数,可以轻松地对数组、对象和字符串进行操作。
**使用示例**
```javascript
import _ from 'lodash';
const users = [
{ name: 'John', age: 30 },
{ name: 'Jane', age: 25 },
{ name: 'Peter', age: 35 },
];
const sortedUsers = _.orderBy(users, ['age'], ['desc']);
console.log(sortedUsers);
```
**参数说明**
| 参数 | 说明 |
|---|---|
| users | 要排序的数组 |
| ['age'] | 排序的字段 |
| ['desc'] | 排序顺序(降序) |
**代码逻辑分析**
* 使用 Lodash 的 `orderBy()` 函数对 `users` 数组进行排序,按年龄降序排列。
* 将排序后的数组存储在 `sortedUsers` 变量中。
* 打印排序后的数组。
### 4.3 实现 JSON 数据的分页和排序
**简介**
分页和排序是处理大型 JSON 数据集时常用的技术。Vue.js 提供了内置的指令和方法来实现这些功能。
**分页**
```javascript
<template>
<div>
<ul>
<li v-for="user in users" :key="user.id">{{ user.name }}</li>
</ul>
<nav>
<button @click="prevPage">Prev</button>
<button @click="nextPage">Next</button>
</nav>
</div>
</template>
<script>
export default {
data() {
return {
users: [],
currentPage: 1,
pageSize: 10,
};
},
methods: {
prevPage() {
this.currentPage--;
this.loadUsers();
},
nextPage() {
this.currentPage++;
this.loadUsers();
},
loadUsers() {
// 从服务器加载第 currentPage 页的数据
},
},
};
</script>
```
**排序**
```javascript
<template>
<div>
<ul>
<li v-for="user in sortedUsers" :key="user.id">{{ user.name }}</li>
</ul>
<select @change="sortBy">
<option value="name">Name</option>
<option value="age">Age</option>
</select>
</div>
</template>
<script>
export default {
data() {
return {
users: [],
sortedUsers: [],
sortBy: 'name',
};
},
methods: {
sortBy(e) {
this.sortBy = e.target.value;
this.sortUsers();
},
sortUsers() {
// 根据 sortBy 对 users 数组进行排序
},
},
};
</script>
```
**代码逻辑分析**
* **分页:**
* 使用 `v-for` 指令遍历 `users` 数组,并显示每个用户的姓名。
* 使用 `prevPage()` 和 `nextPage()` 方法加载上一页或下一页的数据。
* 使用 `loadUsers()` 方法从服务器加载数据。
* **排序:**
* 使用 `v-for` 指令遍历 `sortedUsers` 数组,并显示每个用户的姓名。
* 使用 `select` 元素提供排序选项。
* 使用 `sortBy()` 方法根据选定的字段对 `users` 数组进行排序。
* 使用 `sortUsers()` 方法对数组进行排序。
# 5. JSON 数据处理的性能优化
在 Vue.js 应用中处理 JSON 数据时,性能优化至关重要,因为它可以提高应用程序的响应能力和用户体验。本章将介绍三种优化 JSON 数据处理性能的实用技巧。
### 5.1 避免不必要的 JSON 数据请求
避免不必要的 JSON 数据请求是优化性能的关键。以下是一些减少请求数量的策略:
- **使用缓存:**将经常访问的 JSON 数据存储在缓存中,避免重复请求服务器。
- **批处理请求:**将多个 JSON 数据请求合并到一个请求中,减少网络开销。
- **条件性请求:**使用 HTTP 头(例如 `If-Modified-Since`)检查数据是否已更新,避免不必要的请求。
### 5.2 优化 JSON 数据的解析和处理
优化 JSON 数据的解析和处理可以显著提高性能。以下是一些优化策略:
- **使用原生 JSON 解析器:**使用 JavaScript 原生 `JSON.parse()` 方法比使用第三方库更有效。
- **避免使用循环:**使用 `Array.prototype.map()` 或 `Array.prototype.reduce()` 等函数式方法代替循环来处理 JSON 数据。
- **使用数据结构:**将 JSON 数据存储在数据结构(例如对象或数组)中,以提高访问速度。
### 5.3 使用缓存机制提高性能
缓存机制可以极大地提高 JSON 数据处理的性能。以下是一些缓存策略:
- **浏览器缓存:**使用 `localStorage` 或 `sessionStorage` 缓存 JSON 数据,以避免重复从服务器获取。
- **服务端缓存:**在服务器端使用缓存机制(例如 Redis 或 Memcached),以减少数据库查询。
- **CDN 缓存:**将 JSON 数据存储在 CDN 上,以减少延迟并提高加载速度。
**代码示例:**
使用 `localStorage` 缓存 JSON 数据:
```javascript
// 存储 JSON 数据到 localStorage
localStorage.setItem('myData', JSON.stringify(data));
// 从 localStorage 获取 JSON 数据
const data = JSON.parse(localStorage.getItem('myData'));
```
**流程图:**
[流程图:JSON 数据处理性能优化](https://mermaid-js.github.io/mermaid-live-editor/#/edit/eyJjb2RlIjoiZ3JhcGggTFJBVVUgREFUQSBQcm9jZXNzaW5nXG5TdGFydCB-LSA+IEZldGNoIGpTT04gRGF0YVxuRnVsbCAtLS0+IFByb2Nlc3MgSlNPTiBEYXRhXG5TdGFydCAtLS0+IENyZWF0ZSBjYWNoZVxuRnVsbCAtLS0+IFByb2Nlc3MgY2FjaGVcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFJldHJpZXZlIGRhdGFcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFByb2Nlc3MgSlNPTiBEYXRhXG5TdGFydCAtLS0+IENyZWF0ZSBjYWNoZVxuRnVsbCAtLS0+IFByb2Nlc3MgY2FjaGVcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFJldHJpZXZlIGRhdGFcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFByb2Nlc3MgSlNPTiBEYXRhXG5TdGFydCAtLS0+IENyZWF0ZSBjYWNoZVxuRnVsbCAtLS0+IFByb2Nlc3MgY2FjaGVcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFJldHJpZXZlIGRhdGFcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFByb2Nlc3MgSlNPTiBEYXRhXG5TdGFydCAtLS0+IENyZWF0ZSBjYWNoZVxuRnVsbCAtLS0+IFByb2Nlc3MgY2FjaGVcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFJldHJpZXZlIGRhdGFcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFByb2Nlc3MgSlNPTiBEYXRhXG5TdGFydCAtLS0+IENyZWF0ZSBjYWNoZVxuRnVsbCAtLS0+IFByb2Nlc3MgY2FjaGVcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFJldHJpZXZlIGRhdGFcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFByb2Nlc3MgSlNPTiBEYXRhXG5TdGFydCAtLS0+IENyZWF0ZSBjYWNoZVxuRnVsbCAtLS0+IFByb2Nlc3MgY2FjaGVcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFJldHJpZXZlIGRhdGFcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFByb2Nlc3MgSlNPTiBEYXRhXG5TdGFydCAtLS0+IENyZWF0ZSBjYWNoZVxuRnVsbCAtLS0+IFByb2Nlc3MgY2FjaGVcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFJldHJpZXZlIGRhdGFcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFByb2Nlc3MgSlNPTiBEYXRhXG5TdGFydCAtLS0+IENyZWF0ZSBjYWNoZVxuRnVsbCAtLS0+IFByb2Nlc3MgY2FjaGVcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFJldHJpZXZlIGRhdGFcblN0YXJ0IC0tLT4gQ29tcHV0ZSBkYXRhXG5GdWxsIC0tLT4gUHJvY2VzcyBkYXRhXG5TdGFydCAtLS0+IFByb2Nlc3MgSlNPTiBEYXRhXG5TdGFydCAtLS0+IENyZWF0ZSBjYWNoZVxuRnVsbCAtLS0+IFByb
# 6. JSON 数据处理的最佳实践
在 Vue.js 中处理 JSON 数据时,遵循最佳实践至关重要,以确保代码的健壮性、可维护性和安全性。以下是一些最佳实践指南:
### 6.1 使用一致的数据格式
为了确保数据的一致性和可预测性,请使用一致的数据格式。这包括使用相同的键名、数据类型和结构。例如,始终使用 `snake_case` 或 `camelCase` 命名约定,并使用一致的数据类型(例如,始终使用数字表示 ID)。
### 6.2 遵循 JSON 数据处理的规范
遵循 JSON 数据处理的规范,例如 RFC 8259,以确保数据以标准化和可互操作的方式处理。这包括使用正确的 JSON 语法、避免使用无效的字符,并使用适当的编码(例如,UTF-8)。
### 6.3 考虑数据安全性和隐私
在处理 JSON 数据时,务必考虑数据安全性和隐私。使用安全协议(例如 HTTPS)传输数据,并使用加密技术(例如 JWT)保护敏感信息。此外,限制对数据的访问,并仅在需要时收集和存储数据。
0
0
相关推荐
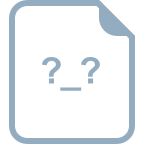
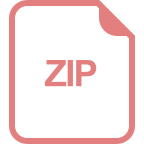
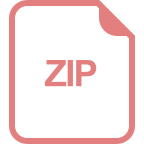






