:Solidity语言详解:解锁Sawtooth区块链智能合约的奥秘
发布时间: 2024-07-08 07:04:38 阅读量: 40 订阅数: 36 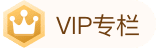
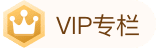
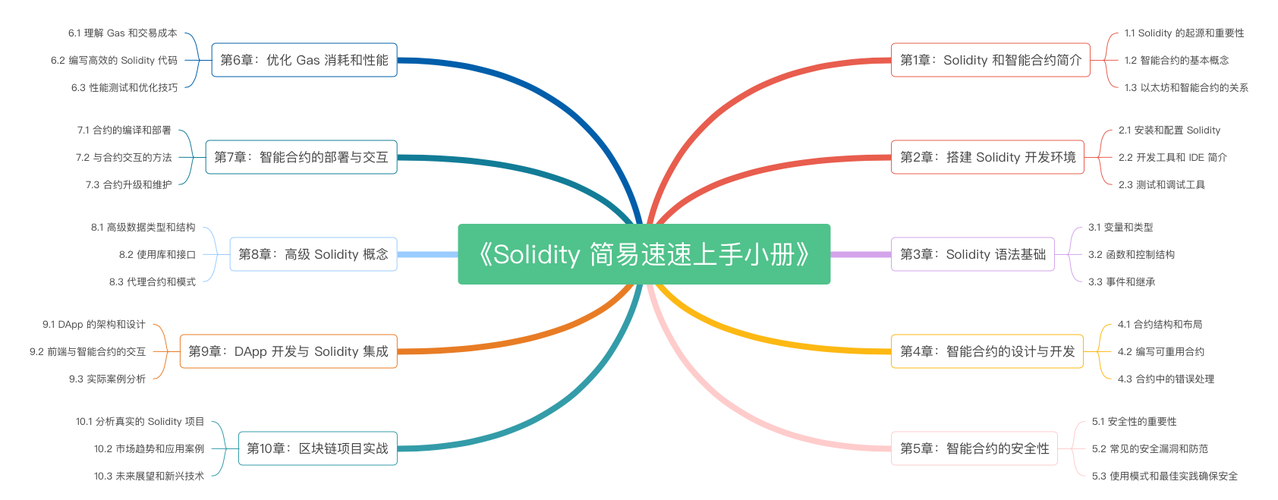
# 1. Solidity语言基础**
Solidity是一种面向合约的高级编程语言,专门设计用于编写在以太坊虚拟机(EVM)上运行的智能合约。它是一种静态类型语言,具有类似于C++和JavaScript的语法。
Solidity智能合约由一系列函数、变量和事件组成。函数定义了合约的行为,而变量存储合约的状态。事件允许合约发出事件,以便其他合约或应用程序可以监听和响应。
Solidity支持多种数据类型,包括基本类型(如整数、布尔值和字符串)和复杂类型(如数组、映射和结构)。它还提供了丰富的运算符集,用于执行算术、逻辑和比较操作。
# 2.1 智能合约的基本概念和结构
### 2.1.1 智能合约的定义和特征
智能合约是一种运行在区块链网络上的代码,它允许在没有第三方的情况下执行和强制执行合约条款。智能合约的特点包括:
- **自动化:** 智能合约自动执行预定义的合约条款,无需人工干预。
- **不可变性:** 一旦部署,智能合约就无法修改,确保合约条款的不可更改性。
- **透明度:** 智能合约的代码和执行结果都存储在区块链上,对所有参与者公开透明。
- **安全性:** 智能合约运行在分布式区块链网络上,具有很高的安全性,防止未经授权的访问和篡改。
### 2.1.2 智能合约的部署和执行
智能合约的部署涉及将合约代码编译成字节码,然后将其提交到区块链网络。字节码是一种低级机器代码,由区块链虚拟机(EVM)执行。
智能合约的执行是通过交易触发的。交易包含调用合约特定函数的数据和所需费用。EVM执行合约代码,并根据合约逻辑更新区块链状态。
```solidity
// Solidity 智能合约示例
contract SimpleStorage {
uint storedData;
function set(uint x) public {
storedData = x;
}
function get() public view returns (uint) {
return storedData;
}
}
```
**代码逻辑分析:**
- `SimpleStorage` 合约定义了一个名为 `storedData` 的状态变量,用于存储数据。
- `set` 函数允许用户将数据写入合约,并将值存储在 `storedData` 中。
- `get` 函数是一个只读函数,允许用户检索存储在 `storedData` 中的数据。
**参数说明:**
- `set` 函数接受一个 `uint` 类型参数 `x`,表示要存储的数据。
- `get` 函数不接受任何参数,并返回一个 `uint` 类型的值,表示存储的数据。
# 3. Sawtooth区块链集成
### 3.1 Sawtooth区块链概述
#### 3.1.1 Sawtooth区块链的架构和共识机制
Sawtooth区块链是一个模块化、可扩展的区块链平台,专为企业和组织设计。它采用模块化架构,允许用户根据需要定制区块链,以满足特定需求。
Sawtooth区块链使用共识机制称为PoET(证明工作时间),该机制奖励矿工根据解决密码谜题所花费的时间。PoET旨在节能,并通过要求矿工提交解决方案的哈希值而不是整个解决方案来实现这一点。
#### 3.1.2 Sawtooth区块链的智能合约模型
Sawtooth区块链支持智能合约,称为“交易处理器”。交易处理器是使用Sawtooth的智能合约语言“Seth”编写的程序。它们在Sawtooth虚拟机(SVM)上执行,该虚拟机提供隔离和安全的环境。
### 3.2 Solidity智能合约与Sawtooth区块链集成
#### 3.2.1 智能合约的部署和调用
将Solidity智能合约部署到Sawtooth区块链涉及以下步骤:
1. **编译智能合约:**使用Solidity编译器将智能合约编译为字节码。
2. **创建交易:**创建一个交易,其中包含智能合约的字节码和部署参数。
3. **提交交易:**将交易提交到Sawtooth区块链。
4. **获取智能合约地址:**一旦交易被确认,即可获取智能合约的地址。
调用智能合约涉及以下步骤:
1. **创建交易:**创建一个交易,其中包含智能合约地址和调用数据。
2. **提交交易:**将交易提交到Sawtooth区块链。
3. **获取结果:**一旦交易被确认,即可获取智能合约调用的结果。
#### 3.2.2 智能合约与交易数据的交互
Solidity智能合约可以使用Sawtooth的交易数据模型与区块链进行交互。交易数据模型允许智能合约访问交易元数据,例如发送者地址、交易ID和时间戳。
智能合约还可以使用Sawtooth的事件系统来记录和发布事件。事件可用于通知其他智能合约或应用程序有关区块链上发生的事件。
### 代码示例
以下代码示例演示了如何将Solidity智能合约部署到Sawtooth区块链:
```solidity
// Solidity智能合约
contract MyContract {
// 智能合约代码
}
```
```python
# Python脚本部署智能合约
from sawtooth_sdk.client_signing import ClientSigning
from sawtooth_sdk.protobuf.transaction_pb2 import Transaction
from sawtooth_sdk.protobuf.batch_pb2 import Batch
from sawtooth_sdk.protobuf.batch_pb2 import BatchHeader
from sawtooth_sdk.protobuf.batch_pb2 import BatchList
from sawtooth_sdk.protobuf.transaction_pb2 import TransactionHeader
# 创建客户端签名器
signer = ClientSigning()
# 编译智能合约
contract_address = signer.get_public_key().as_hex()
contract_bytecode = "0x..." # 智能合约字节码
# 创建交易
transaction = Transaction(
header=TransactionHeader(
family_name="my_contract",
family_version="1.0",
inputs=[contract_address],
outputs=[contract_address],
signer_public_key=signer.get_public_key().as_hex(),
batcher_public_key=signer.get_public_key().as_hex(),
dependencies=[],
payload_sha512=contract_bytecode,
),
payload_data=contract_bytecode,
)
# 创建批次
batch = Batch(
header=BatchHeader(
signer_public_key=signer.get_public_key().as_hex(),
transaction_ids=[transaction.header_signature],
),
transactions=[transaction],
)
# 创建批次列表
batch_list = BatchList(batches=[batch])
# 提交交易
client.send_batches(batch_list)
```
### 流程图
下图展示了将Solidity智能合约部署到Sawtooth区块链的过程:
[流程图:Solidity智能合约部署到Sawtooth区块链](https://mermaid-js.github.io/mermaid-live-editor/#/edit/eyJjb2RlIjoiZ3JhcGgKICBkYXRhc3RydWN0dXJlIGRlcGxveW1lbnQgb3ZlcnZpZXcgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAg
0
0
相关推荐
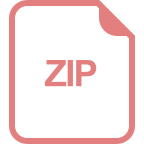






