无人驾驶感知与决策之匙:OpenCV图像几何变换在无人驾驶中的应用
发布时间: 2024-08-08 19:40:33 阅读量: 20 订阅数: 40 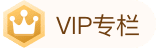
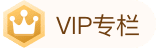
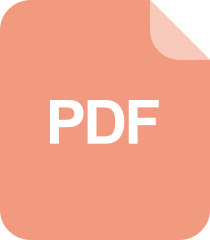
OpenCV图像几何变换之透视变换
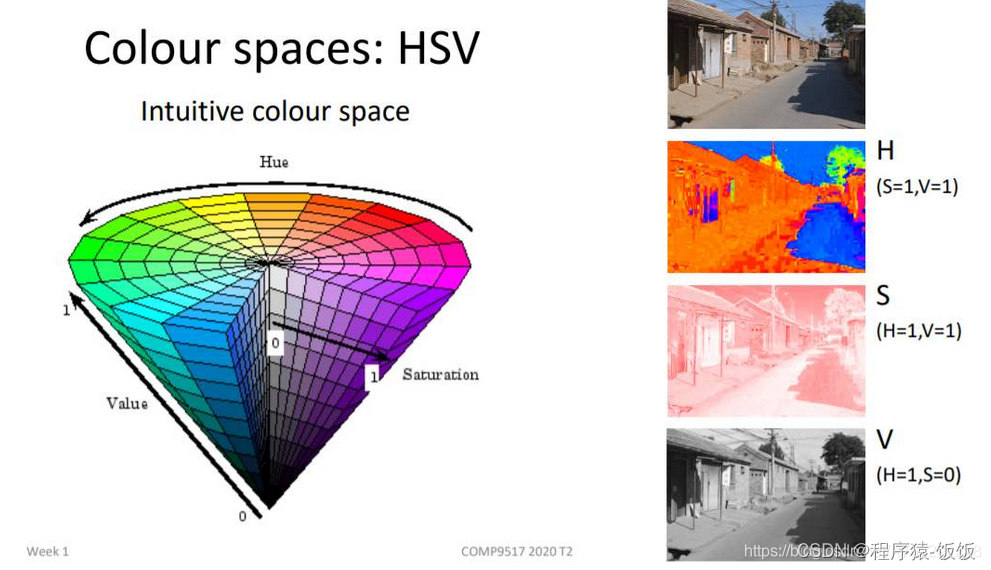
# 1. 图像几何变换基础**
图像几何变换是一种计算机视觉技术,用于对图像进行空间操作,以改变其大小、形状或透视。在无人驾驶领域,图像几何变换对于感知和决策至关重要,因为它可以帮助车辆理解其周围环境。
图像几何变换的基本类型包括平移、旋转、缩放、仿射变换和透视变换。平移变换将图像沿水平或垂直方向移动,而旋转变换将其围绕一个固定点旋转。缩放变换改变图像的大小,而仿射变换应用一个线性变换,可以同时进行平移、旋转和缩放。透视变换是一种更复杂的变换,它可以模拟三维场景的投影效果。
# 2. OpenCV图像几何变换技术**
图像几何变换是一类操作图像像素位置的技术,用于调整图像的形状、大小和透视。在无人驾驶中,图像几何变换对于感知和决策任务至关重要,因为它可以校正图像失真、提取特征并增强图像内容。
**2.1 图像平移、旋转和缩放**
平移、旋转和缩放是图像几何变换中最基本的类型。它们可以用于调整图像的位置、方向和大小。
**2.1.1 平移变换**
平移变换将图像中的所有像素沿水平或垂直方向移动相同的距离。它可以通过以下公式表示:
```python
import cv2
import numpy as np
def translate_image(image, x, y):
"""
平移图像
Args:
image: 输入图像
x: 水平平移距离
y: 垂直平移距离
Returns:
平移后的图像
"""
# 创建平移矩阵
M = np.float32([[1, 0, x], [0, 1, y]])
# 执行平移变换
translated_image = cv2.warpAffine(image, M, (image.shape[1], image.shape[0]))
return translated_image
```
**2.1.2 旋转变换**
旋转变换将图像中的所有像素围绕给定点旋转一定的角度。它可以通过以下公式表示:
```python
import cv2
import numpy as np
def rotate_image(image, angle, center=None, scale=1.0):
"""
旋转图像
Args:
image: 输入图像
angle: 旋转角度(以度为单位)
center: 旋转中心(可选,默认为图像中心)
scale: 旋转后图像的缩放因子(可选,默认为 1.0)
Returns:
旋转后的图像
"""
# 获取图像中心
if center is None:
center = (image.shape[1] // 2, image.shape[0] // 2)
# 创建旋转矩阵
M = cv2.getRotationMatrix2D(center, angle, scale)
# 执行旋转变换
rotated_image = cv2.warpAffine(image, M, (image.shape[1], image.shape[0]))
return rotated_image
```
**2.1.3 缩放变换**
缩放变换将图像中的所有像素沿水平和垂直方向缩放相同的比例因子。它可以通过以下公式表示:
```python
import cv2
import numpy as np
def scale_image(image, scale_x, scale_y):
"""
缩放图像
Args:
image: 输入图像
scale_x: 水平缩放因子
scale_y: 垂直缩放因子
Returns:
缩放后的图像
"""
# 创建缩放矩阵
M = np.float32([[scale_x, 0, 0], [0, scale_y, 0]])
# 执行缩放变换
scaled_image = cv2.warpAffine(image, M, (image.shape[1], image.shape[0]))
return scaled_image
```
**2.2 图像仿射变换和透视变换**
仿射变换和透视变换是更高级的图像几何变换类型,它们允许对图像进行更复杂的变形。
**2.2.1 仿射变换**
仿射变换将图像中的所有像素沿水平和垂直方向线性变换。它可以通过以下公式表示:
```python
import cv2
import numpy as np
def affine_transform(image, M):
"""
仿射变换图像
Args:
image: 输入图像
M: 仿射变换矩阵
Returns:
仿射变换后的图像
"""
# 执行仿射变换
affine_image = cv2.warpAffine(image, M, (image.shape[1], image.shape[0]))
return affine_image
```
**2.2.2 透视变换**
透视变换将图像中的所有像素沿三维空间中的一个平面投影到另一个平面。它可以通过以下公式表示:
```python
import cv2
import numpy as np
def perspective_transform(image, M):
"""
透视变换图像
Args:
image: 输入图像
M: 透视变换矩阵
Returns:
透视变换后的图像
"""
# 执行透视变换
perspective_image = cv2.warpPerspective(image, M, (image.shape[1], image.shape[0]))
return perspective_image
```
**表格:图像几何变换类型总结**
| 变换类型 | 描述 | 公式 |
|---|---|---|
| 平移 | 将图像沿水平或垂直方向移动 | M = [[1, 0, x], [0, 1, y]] |
| 旋转 | 将图像围绕给定点旋转 | M = cv2.getRotationMatrix2D(center, angle, scale) |
| 缩放 | 将图像沿水平和垂直方向缩放 | M = [[scale_x, 0, 0], [0, scale_y, 0]] |
| 仿射 | 将图像沿水平和垂直方向线性变换 | M = [[a, b, c], [d, e, f]] |
| 透视 | 将图像沿三维空间中的一个平面投影到另一个平面 | M = [[a, b, c, d], [e, f, g, h], [i, j, k, l]] |
**流程图:图像几何变换流程**
[流程图:图像几何变换流程](https://mermaid-js.github.io/mermaid/img/diagrams/flowchart/flowchart-simple.svg)
# 3. 无人驾驶中的图像几何变换应用
图像几何变换在无人驾驶中扮演着至关重要的角色,它能够对图像进行各种几何操作,从而帮助无人驾驶系统感知周围环境并做出决策。本章节将深入探讨图像几何变换在无人驾驶中的具体应用
0
0
相关推荐







