Java压缩算法在图像处理中的应用:优化图像存储和传输,提升图像质量
发布时间: 2024-08-27 19:51:14 阅读量: 74 订阅数: 42 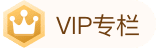
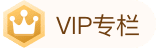
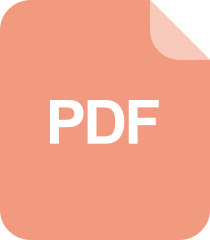
数字图像处理-Java语言算法描述
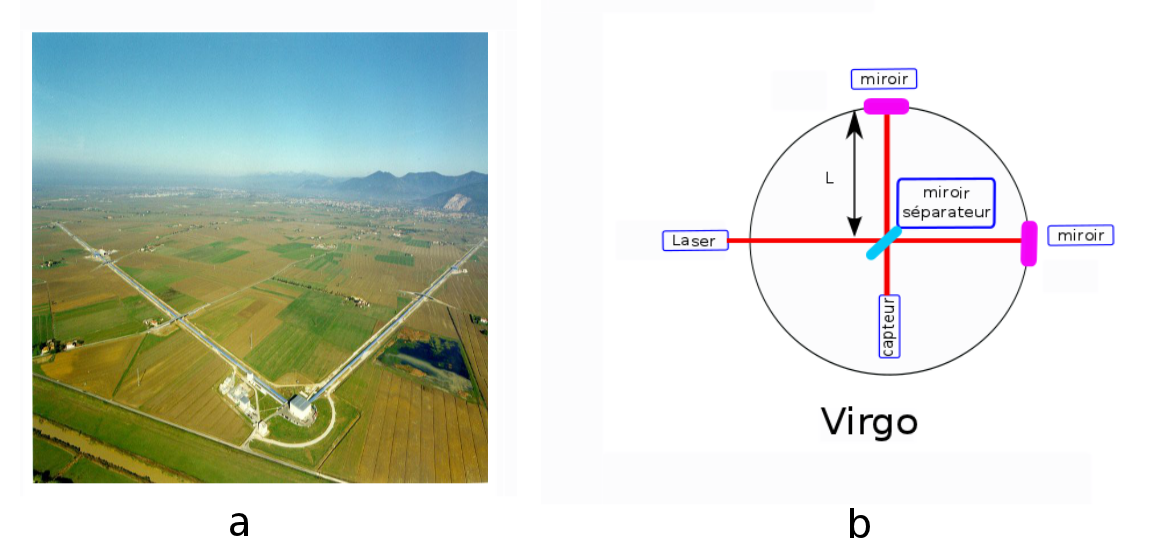
# 1. 图像压缩算法概述**
图像压缩是一种减少图像文件大小的技术,同时保持其视觉质量。它通过去除冗余信息来实现,从而降低图像文件的大小。图像压缩算法分为两大类:无损压缩和有损压缩。
无损压缩算法不丢失任何图像信息,但压缩率较低。有损压缩算法会丢失一些图像信息,但可以实现更高的压缩率。选择哪种算法取决于图像的用途和对质量的要求。
# 2. Java图像压缩算法
**2.1 无损压缩算法**
无损压缩算法在压缩过程中不会丢失任何图像信息,因此可以完美还原原始图像。常用的无损压缩算法包括:
### 2.1.1 LZW算法
LZW(Lempel-Ziv-Welch)算法是一种字典编码算法,通过将重复出现的字符序列替换为较短的代码来实现压缩。算法流程如下:
```java
// LZW压缩算法
public static byte[] compress(byte[] data) {
// 初始化字典
Map<String, Integer> dictionary = new HashMap<>();
for (int i = 0; i < 256; i++) {
dictionary.put(String.valueOf((char) i), i);
}
// 扫描输入数据,构建字典
int code = 256;
StringBuilder w = new StringBuilder();
List<Integer> compressedData = new ArrayList<>();
for (byte b : data) {
String wc = w.toString() + (char) b;
if (dictionary.containsKey(wc)) {
w = wc;
} else {
compressedData.add(dictionary.get(w));
dictionary.put(wc, code++);
w = String.valueOf((char) b);
}
}
compressedData.add(dictionary.get(w));
// 返回压缩后的数据
return toByteArray(compressedData);
}
// 将整数列表转换为字节数组
private static byte[] toByteArray(List<Integer> data) {
byte[] bytes = new byte[data.size()];
for (int i = 0; i < data.size(); i++) {
bytes[i] = (byte) data.get(i);
}
return bytes;
}
```
**逻辑分析:**
* 首先,算法初始化一个字典,其中包含所有可能的字符及其对应的代码。
* 然后,算法扫描输入数据,并逐个字符地构建字典。
* 当遇到一个重复的字符序列时,算法将其替换为字典中的代码。
* 如果字典中不存在该序列,则算法将当前序列添加到字典中,并为其分配一个新的代码。
* 算法重复此过程,直到扫描完所有输入数据。
* 最后,算法返回压缩后的数据,该数据由字典代码组成。
### 2.1.2 Huffman编码
Huffman编码是一种基于频率的压缩算法,它将出现频率较高的字符分配较短的代码,而出现频率较低的字符分配较长的代码。算法流程如下:
```java
// Huffman编码压缩算法
public static byte[] compress(byte[] data) {
// 统计字符频率
Map<Byte, Integer> frequencies = new HashMap<>();
for (byte b : data) {
frequencies.merge(b, 1, Integer::sum);
}
// 构建哈夫曼树
Node root = buildHuffmanTree(frequencies);
// 构建编码表
Map<Byte, String> codeTable = new HashMap<>();
buildCodeTable(root, "", codeTable);
// 压缩数据
StringBuilder compressedData = new StringBuilder();
for (byte b : data) {
compressedData.append(codeTable.get(b));
}
// 返回压缩后的数据
return compressedData.toString().getBytes();
}
// 构建哈夫曼树
private static Node buildHuffmanTree(Map<Byte, Integer> frequencies) {
// 创建优先级队列
PriorityQueue<Node> queue = new PriorityQueue<>((a, b) -> a.frequency - b.frequency);
// 将字符及其频率添加到队列中
for (Map.Entry<Byte, Integer> entry : frequencies.entrySet()) {
queue.add(new Node(entry.getKey(), entry.getValue()));
}
// 循环合并队列中的节点,直到只剩下一个根节点
while (queue.size() > 1) {
Node left = queue.poll();
Node right = queue.poll();
Node parent = new Node(null, left.frequency + right.frequency);
parent.left = left;
parent.right = right;
queue.add(parent);
}
// 返回根节点
return queue.poll();
}
// 构建编码表
private static void buildCodeTable(Node root, String code, Map<Byte, String> codeTable) {
if (root.left == null && root.right == null) {
codeTable.put(root.value, code);
} else {
buildCodeTable(root.left, code + "0", codeTable);
buildCodeTable(root.right, code + "1", codeTable);
}
}
// 哈夫曼树节点
private static class Node {
Byte value;
int frequency;
Node left;
Node right;
public Node(Byte value, int frequency) {
this.value = value;
this.frequency = frequency;
}
}
```
**逻辑分析:**
* 首先,算法统计输入数据中每个字符的频率。
* 然后,算法使用这些频率构建一棵哈夫曼树。
* 哈夫曼树是一个二叉树,其中出现频率较高的字符位于树的顶部,而出现频率较低的字符位于树的底部。
* 算法遍历哈夫曼树,并为每个字符分配一个编码,编码的长度与字符的频率成反比。
* 最后,算法使用这些编码压缩输入数据。
**2.2 有损压缩算法**
有损压缩算法在压缩过程中会丢失一些图像信息,从而达到更高的压缩率。常用的有损压缩算法包括:
### 2.2.1 JPEG算法
JPEG(Joint Photographic Experts Group)算法是一种基于离散余弦变换(DCT)的有损压缩算法。算
0
0
相关推荐
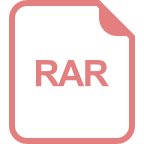
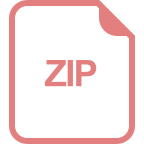
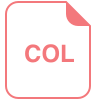
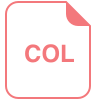
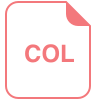
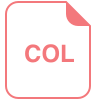
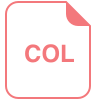
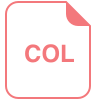