算法优化中的贪心算法:快速求解近似最优解的捷径
发布时间: 2024-08-25 04:54:15 阅读量: 22 订阅数: 44 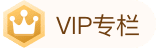
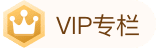
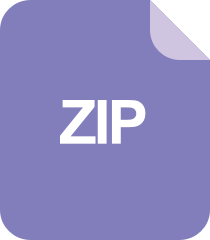
MATLAB神经网络和优化算法:3.遗传算法求解最优解最大值.zip

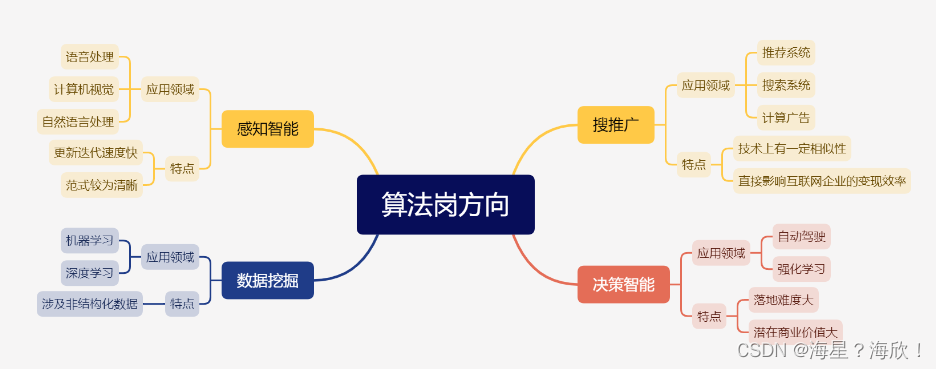
# 1. 贪心算法概述
贪心算法是一种自顶向下的启发式算法,它通过在每一步中做出局部最优选择,逐步逼近全局最优解。贪心算法的优点在于简单易懂,计算效率高,但其局限性在于,它不能保证在所有情况下都能找到全局最优解。
贪心算法适用于以下场景:
* 子问题具有独立性,即一个子问题的最优解不会影响其他子问题的最优解。
* 局部最优解可以逐步逼近全局最优解。
* 问题规模较大,难以直接求解全局最优解。
# 2. 贪心算法的理论基础
### 2.1 贪心算法的定义和原理
**定义:**
贪心算法是一种启发式算法,它在每次决策时都选择当前看来最优的局部选择,而不考虑全局最优解。
**原理:**
1. **局部最优性:**贪心算法在每次决策时都选择当前看来最优的局部选择,即局部最优解。
2. **递推性:**贪心算法通过一系列局部最优决策,逐步逼近全局最优解。
### 2.2 贪心算法的适用场景和局限性
**适用场景:**
* 问题可以分解为一系列相互独立的子问题。
* 每个子问题的最优解可以独立求解。
* 子问题的最优解组合起来可以得到全局最优解。
**局限性:**
* 贪心算法不能保证全局最优解。
* 贪心算法对输入顺序敏感,不同的输入顺序可能导致不同的结果。
* 贪心算法不适用于问题相互依赖或子问题的最优解不能独立求解的情况。
### 代码示例:
```python
def greedy_knapsack(items, capacity):
"""
0-1背包问题贪心算法
参数:
items: 物品列表,每个物品包含价值和重量
capacity: 背包容量
返回:
背包中物品的最大总价值
"""
# 按价值密度(价值/重量)降序排列物品
items.sort(key=lambda item: item["value"] / item["weight"], reverse=True)
# 初始化背包
backpack = []
total_value = 0
total_weight = 0
# 遍历物品
for item in items:
# 如果背包容量足够装下当前物品
if total_weight + item["weight"] <= capacity:
# 将当前物品放入背包
backpack.append(item)
total_value += item["value"]
total_weight += item["weight"]
else:
# 背包容量不足,跳过当前物品
break
return total_value
```
**逻辑分析:**
* 贪心算法通过按价值密度降序排列物品,每次选择价值密度最高的物品放入背包。
* 贪心算法利用了局部最优性,即每次选择价值密度最高的物品都是当前最优选择。
* 贪心算法通过递推性,逐步将局部最优决策组合起来,逼近全局最优解。
**参数说明:**
* `items`:物品列表,每个物品是一个字典,包含`value`(价值)和`weight`(重量)键值对。
* `capacity`:背包容量。
**代码解释:**
* `sort()`函数按`value` / `weight`降序排列物品,确保价值密度最高的物品排在前面。
* 循环遍历物品,如果背包容量足够装下当前物品,则将当前物品放入背包并更新背包的总价值和总重量。
* 如果背包容量不足,则跳过当前物品。
* 返回背包中物品的最大总价值。
# 3.1 背包问题中的贪心算法
#### 3.1.1 0-1背包问题
**定义:**
0-1背包问题是指在给定容量为C的背包和n件物品的情况下,每件物品有其价值v和重量w,选择若干物品放入背包,使得背包中物品的总价值最大,但不能超过背包容量。
**贪心算法:**
1. 将物品按价值密度(v/w)从大到小排序。
2. 从价值密度最大的物品开始,依次放入背包。
3. 若当前物品重量加上背包中已装物品重量超过背包容量,则跳过该物品。
4. 重复步骤2和3,直至背包装满或所有物品都已考虑。
**代码块:**
```python
def greedy_01_knapsack(items, capacity):
"""
0-1背包问题贪心算法
参数:
items: 物品列表,每个物品包含价值v和重量w
capacity: 背包
```
0
0
相关推荐
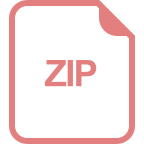
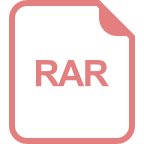
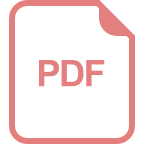
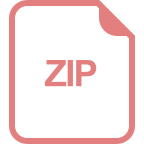
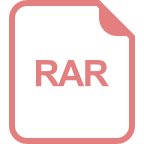
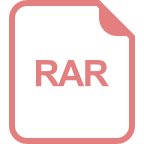
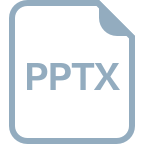