揭秘OpenCV图像旋转:原理、算法和实战应用
发布时间: 2024-08-12 14:29:37 阅读量: 17 订阅数: 14 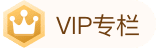
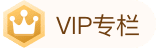
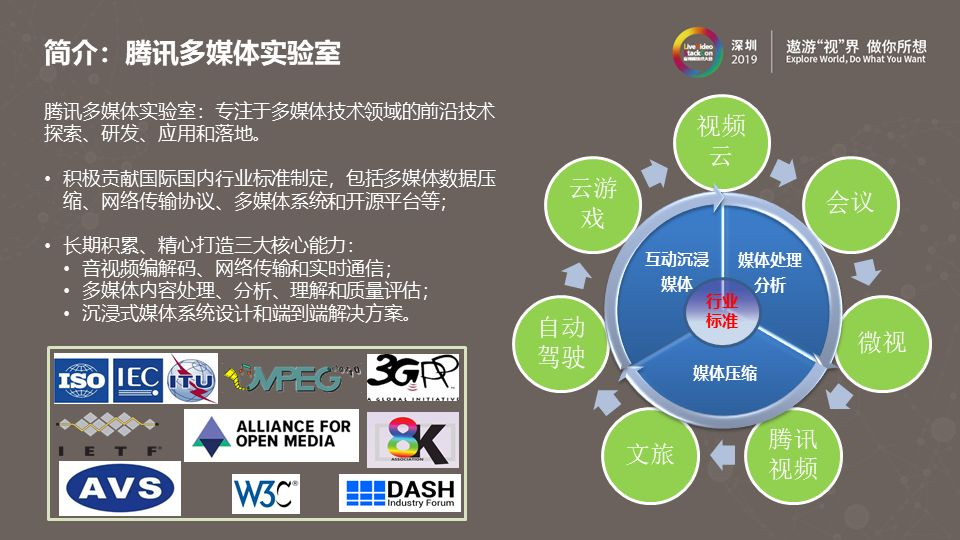
# 1. 图像旋转的基本原理**
图像旋转是一种图像处理技术,它将图像围绕一个固定点旋转一定角度。图像旋转在许多应用中都有着广泛的用途,例如图像矫正、图像增强和虚拟现实。
图像旋转的基本原理是基于仿射变换和透视变换。仿射变换是一种线性变换,它保持平行线的平行性。透视变换是一种非线性变换,它可以模拟三维场景中的透视效果。
# 2. 图像旋转算法
图像旋转是一种图像变换技术,用于将图像绕一个中心点旋转一定角度。图像旋转算法有多种,每种算法都有其独特的优点和缺点。
### 2.1 仿射变换
仿射变换是一种几何变换,它可以将一个平面上的点变换到另一个平面上。仿射变换矩阵是一个 3x3 矩阵,它定义了变换的旋转、平移和缩放参数。
#### 2.1.1 仿射变换矩阵
仿射变换矩阵通常表示为:
```
[a, b, tx]
[c, d, ty]
[0, 0, 1]
```
其中:
* `a` 和 `d` 定义了旋转角度和缩放因子。
* `b` 和 `c` 定义了平移量。
* `tx` 和 `ty` 定义了旋转中心。
#### 2.1.2 仿射变换的应用
仿射变换广泛应用于图像旋转,因为它可以同时进行旋转、平移和缩放。在 OpenCV 中,可以使用 `getRotationMatrix2D()` 函数来生成仿射变换矩阵,并使用 `warpAffine()` 函数来应用仿射变换。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 生成仿射变换矩阵
rotation_matrix = cv2.getRotationMatrix2D((image.shape[1] // 2, image.shape[0] // 2), 45, 1)
# 应用仿射变换
rotated_image = cv2.warpAffine(image, rotation_matrix, (image.shape[1], image.shape[0]))
# 显示旋转后的图像
cv2.imshow('Rotated Image', rotated_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 2.2 透视变换
透视变换是一种更复杂的几何变换,它可以将一个平面上的点变换到另一个平面上,并引入透视效果。透视变换矩阵是一个 3x3 矩阵,它定义了变换的旋转、平移、缩放和透视参数。
#### 2.2.1 透视变换矩阵
透视变换矩阵通常表示为:
```
[a1, a2, a3]
[b1, b2, b3]
[c1, c2, c3]
```
其中:
* `a1`, `a2`, `a3`, `b1`, `b2`, `b3` 定义了旋转、平移和缩放参数。
* `c1`, `c2`, `c3` 定义了透视参数。
#### 2.2.2 透视变换的应用
透视变换广泛应用于图像旋转,因为它可以引入透视效果,从而产生更逼真的旋转效果。在 OpenCV 中,可以使用 `getPerspectiveTransform()` 函数来生成透视变换矩阵,并使用 `warpPerspective()` 函数来应用透视变换。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 生成透视变换矩阵
perspective_matrix = cv2.getPerspectiveTransform(np.array([[0, 0], [image.shape[1], 0], [0, image.shape[0]]]), np.array([[0, 0], [image.shape[1], 0], [0, image.shape[0]]]))
# 应用透视变换
rotated_image = cv2.warpPerspective(image, perspective_matrix, (image.shape[1], image.shape[0]))
# 显示旋转后的图像
cv2.imshow('Rotated Image', rotated_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
# 3.1 使用仿射变换旋转图像
仿射变换是一种几何变换,它可以将图像中的点从一个位置移动到另一个位置,同时保持其形状和大小。在图像旋转中,仿射变换可以用来将图像绕一个中心点旋转指定角度。
#### 3.1.1 getRotationMatrix2D()函数
OpenCV提供了`getRotationMatrix2D()`函数来生成仿射变换矩阵。该函数需要三个参数:
- `center`:旋转中心点坐标(x, y)
- `angle`:旋转角度(以弧度为单位)
- `scale`:缩放因子(可选,默认为1)
```python
import cv2
# 旋转中心点
center = (100, 100)
# 旋转角度(弧度)
angle = np.pi / 6
# 生成仿射变换矩阵
rotation_matrix = cv2.getRotationMatrix2D(center, angle, 1)
```
#### 3.1.2 warpAffine()函数
`warpAffine()`函数使用仿射变换矩阵将图像进行变形。该函数需要三个参数:
- `image`:输入图像
- `rotation_matrix`:仿射变换矩阵
- `dsize`:输出图像的大小
```python
# 输入图像
image = cv2.imread('image.jpg')
# 应用仿射变换
rotated_image = cv2.warpAffine(image, rotation_matrix, (image.shape[1], image.shape[0]))
```
### 3.2 使用透视变换旋转图像
透视变换是一种更通用的几何变换,它可以将图像中的点从一个位置移动到另一个位置,同时改变其形状和大小。在图像旋转中,透视变换可以用来将图像绕一个消失点旋转指定角度。
#### 3.2.1 getPerspectiveTransform()函数
OpenCV提供了`getPerspectiveTransform()`函数来生成透视变换矩阵。该函数需要四个点作为参数,这些点定义了变换前后的对应关系。
```python
import cv2
# 变换前后的对应点
src_points = np.array([[0, 0], [100, 0], [0, 100], [100, 100]])
dst_points = np.array([[50, 50], [150, 50], [50, 150], [150, 150]])
# 生成透视变换矩阵
perspective_matrix = cv2.getPerspectiveTransform(src_points, dst_points)
```
#### 3.2.2 warpPerspective()函数
`warpPerspective()`函数使用透视变换矩阵将图像进行变形。该函数需要三个参数:
- `image`:输入图像
- `perspective_matrix`:透视变换矩阵
- `dsize`:输出图像的大小
```python
# 输入图像
image = cv2.imread('image.jpg')
# 应用透视变换
rotated_image = cv2.warpPerspective(image, perspective_matrix, (image.shape[1], image.shape[0]))
```
# 4. 图像旋转在实际应用中的案例
图像旋转在实际应用中有着广泛的应用,从图像矫正到图像增强,它可以帮助我们改善图像质量、增强视觉效果。
### 4.1 图像矫正
图像矫正是指将倾斜或扭曲的图像恢复到正常状态。图像旋转在图像矫正中扮演着至关重要的角色。
#### 4.1.1 透视矫正
透视矫正用于校正由于相机角度或透视变形而导致的图像扭曲。透视变换矩阵可以用来计算图像中各点的变换,从而将扭曲的图像恢复到正常状态。
```python
import cv2
# 读入扭曲图像
image = cv2.imread('distorted_image.jpg')
# 获取透视变换矩阵
pts1 = np.float32([[0, 0], [image.shape[1], 0], [0, image.shape[0]], [image.shape[1], image.shape[0]]])
pts2 = np.float32([[0, 0], [image.shape[1], 0], [0, image.shape[0]], [image.shape[1], image.shape[0]]])
matrix = cv2.getPerspectiveTransform(pts1, pts2)
# 应用透视变换
corrected_image = cv2.warpPerspective(image, matrix, (image.shape[1], image.shape[0]))
# 显示矫正后的图像
cv2.imshow('Corrected Image', corrected_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
#### 4.1.2 仿射矫正
仿射矫正用于校正图像的旋转、平移、缩放和倾斜。仿射变换矩阵可以用来计算图像中各点的变换,从而将扭曲的图像恢复到正常状态。
```python
import cv2
# 读入扭曲图像
image = cv2.imread('distorted_image.jpg')
# 获取仿射变换矩阵
pts1 = np.float32([[0, 0], [image.shape[1], 0], [0, image.shape[0]]])
pts2 = np.float32([[100, 100], [image.shape[1] - 100, 100], [100, image.shape[0] - 100]])
matrix = cv2.getAffineTransform(pts1, pts2)
# 应用仿射变换
corrected_image = cv2.warpAffine(image, matrix, (image.shape[1], image.shape[0]))
# 显示矫正后的图像
cv2.imshow('Corrected Image', corrected_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 4.2 图像增强
图像增强是指通过各种技术改善图像的视觉效果和质量。图像旋转在图像增强中也发挥着重要作用。
#### 4.2.1 图像旋转增强
图像旋转增强是指通过旋转图像来增强图像的视觉效果。例如,通过旋转图像可以将垂直的文本校正为水平的文本,或者将倾斜的图像校正为水平或垂直的图像。
```python
import cv2
# 读入图像
image = cv2.imread('image.jpg')
# 旋转图像
rotated_image = cv2.rotate(image, cv2.ROTATE_90_CLOCKWISE)
# 显示旋转后的图像
cv2.imshow('Rotated Image', rotated_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
#### 4.2.2 图像旋转去噪
图像旋转去噪是指通过旋转图像来去除图像中的噪声。例如,通过旋转图像可以将图像中的条纹噪声或椒盐噪声去除。
```python
import cv2
import numpy as np
# 读入图像
image = cv2.imread('noisy_image.jpg')
# 将图像转换为灰度图像
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 旋转图像
rotated_image = cv2.rotate(gray_image, cv2.ROTATE_90_CLOCKWISE)
# 应用中值滤波
denoised_image = cv2.medianBlur(rotated_image, 3)
# 将图像旋转回原来的方向
denoised_image = cv2.rotate(denoised_image, cv2.ROTATE_90_COUNTERCLOCKWISE)
# 显示去噪后的图像
cv2.imshow('Denoised Image', denoised_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
# 5. 图像旋转的优化技巧
### 5.1 选择合适的算法
#### 仿射变换与透视变换的比较
仿射变换和透视变换是图像旋转中常用的两种算法。它们在应用场景和效果上存在差异。
| 特征 | 仿射变换 | 透视变换 |
|---|---|---|
| 变换类型 | 线性变换 | 投影变换 |
| 保留特性 | 平行线平行 | 平行线不平行 |
| 适用场景 | 旋转、平移、缩放 | 透视矫正、3D场景建模 |
一般情况下,如果图像中没有明显的透视失真,可以使用仿射变换进行旋转。如果图像中存在透视失真,需要使用透视变换才能获得良好的效果。
### 5.2 优化旋转参数
#### 旋转角度的优化
旋转角度的优化主要涉及两个方面:
1. **角度精度:**旋转角度的精度直接影响旋转效果。对于需要精确旋转的场景,需要使用高精度的旋转算法。
2. **角度范围:**旋转角度的范围需要根据实际应用场景确定。对于需要进行大角度旋转的场景,需要使用支持大角度旋转的算法。
#### 旋转中心的优化
旋转中心的选择对旋转效果也有影响。一般情况下,旋转中心选择在图像的中心点。但是,在某些特殊场景下,可以根据实际需求选择不同的旋转中心。
例如,在图像矫正中,旋转中心可以位于图像的畸变点。这样可以使图像矫正后的效果更加准确。
# 6. 图像旋转的未来发展趋势
### 6.1 深度学习在图像旋转中的应用
深度学习,特别是卷积神经网络(CNN),在图像处理领域取得了显著的成功。CNN 可以自动学习图像中的特征,并将其用于各种任务,包括图像分类、目标检测和图像旋转。
基于 CNN 的图像旋转方法通常涉及以下步骤:
1. **训练 CNN 模型:**使用包含旋转图像的大型数据集训练 CNN 模型,以学习图像旋转的特征。
2. **预测旋转角度:**将新图像输入训练好的 CNN 模型,以预测其旋转角度。
3. **应用旋转变换:**使用预测的旋转角度将图像旋转到所需的方向。
### 6.2 图像旋转在虚拟现实和增强现实中的应用
虚拟现实(VR)和增强现实(AR)技术正在迅速发展,图像旋转在这些领域中发挥着至关重要的作用。
#### 6.2.1 360度图像旋转
在 VR 中,用户可以体验 360 度的沉浸式环境。为了创建这些体验,需要将图像旋转到不同的方向,以匹配用户的头部运动。
#### 6.2.2 增强现实中的图像旋转
在 AR 中,虚拟对象被叠加到现实世界中。为了使这些对象看起来真实,需要将它们旋转到与现实世界相匹配的角度。
0
0
相关推荐
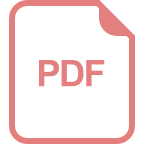
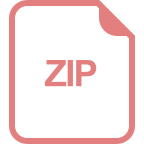
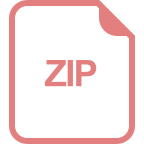
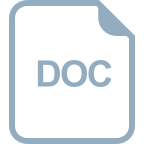
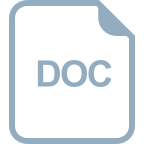
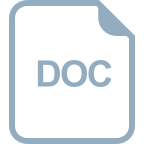
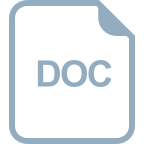
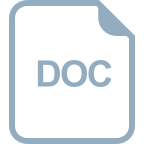
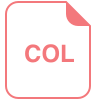