【Python线程性能优化实战】:threading监控与调试的高效方法
发布时间: 2024-10-02 09:25:31 阅读量: 40 订阅数: 24 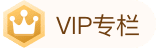
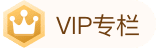
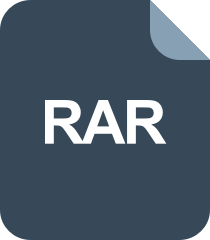
python机器人自动化实战项目

# 1. Python线程基础与多线程编程模型
## 1.1 Python线程概念与优势
Python线程是程序执行流程的一种基本形式,它允许在程序中并发执行多个任务,从而提高程序的执行效率。通过线程,可以让CPU在等待I/O操作时执行其他任务,充分利用系统资源。Python由于其全局解释器锁(GIL)的存在,在原生线程方面并不是完全的并行执行,但多线程依然在I/O密集型任务中有着显著优势。
## 1.2 Python的 threading 模块介绍
Python通过`threading`模块提供了对线程的支持。该模块允许开发者创建、运行和管理线程。`threading`模块提供了一个高级接口,使得用户可以不需要深入了解底层线程的管理细节。使用`threading.Thread`类可以方便地创建线程实例,并通过调用`start()`方法启动线程。
```python
import threading
def print_numbers():
for i in range(1, 6):
print(i)
thread = threading.Thread(target=print_numbers)
thread.start()
thread.join() # 等待线程结束
```
在这个例子中,我们定义了一个打印数字的函数`print_numbers`,然后创建了一个`Thread`对象,并将函数作为参数传递给它。通过`start()`方法启动线程,并通过`join()`方法确保主线程等待新线程结束。
## 1.3 线程的基本用法与示例
在Python中,实现多线程的一个简单示例包括定义一个任务函数,然后创建多个线程对象,每个线程都执行这个任务。需要注意的是,在多线程编程中,应当尽量减少线程之间的数据依赖,避免竞争条件和资源冲突。
```python
import threading
import time
def task(name):
print(f"Thread {name} starting.")
time.sleep(2)
print(f"Thread {name} finishing.")
threads = [threading.Thread(target=task, args=(i,)) for i in range(1, 4)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
```
在这个例子中,我们创建了三个线程,每个线程都会打印一条消息并休眠两秒。每个线程启动后,主线程会等待所有线程执行完毕。
通过本章的学习,我们已经了解了Python线程的基本概念、优势、使用`threading`模块的基本方法,以及如何创建和管理线程。在后续章节中,我们将深入探讨线程同步机制、线程性能分析工具和性能优化策略。
# 2. 线程同步机制详解
### 2.1 线程同步基础
#### 2.1.1 互斥锁(Mutex)
在多线程编程中,互斥锁(Mutex)是一种广泛使用的同步机制,用来防止多个线程同时访问同一资源,从而避免数据竞争和不一致性的问题。互斥锁有两个基本状态,即锁定和非锁定。当一个线程获得互斥锁后,其它尝试获取该锁的线程将被阻塞,直到锁被释放。
使用互斥锁时需要特别注意避免死锁的情况发生,即两个或多个线程在相互等待对方释放锁的情况下无限期地阻塞。
代码示例:
```python
import threading
lock = threading.Lock()
def func():
lock.acquire()
try:
# 临界区代码
print("Critical section")
finally:
lock.release()
# 创建线程
thread1 = threading.Thread(target=func)
thread2 = threading.Thread(target=func)
thread1.start()
thread2.start()
```
在上述代码中,`lock.acquire()` 用于尝试获取锁,如果锁当前被其他线程持有,则阻塞直到锁被释放。`lock.release()` 用于释放锁。`try...finally` 块确保无论临界区内的代码是否抛出异常,锁都能被正确释放。
#### 2.1.2 信号量(Semaphore)
信号量是一种更为通用的同步机制。与互斥锁不同,信号量可以有多个实例,并且每次允许多个线程进入临界区。信号量通常用于控制对共享资源的访问数量。
在Python中,`threading` 模块提供了 `Semaphore` 类。通过初始化一个信号量对象,并在进入临界区前调用 `acquire()` 方法,在离开临界区后调用 `release()` 方法。
代码示例:
```python
import threading
semaphore = threading.Semaphore(5) # 允许5个线程同时通过
def func():
semaphore.acquire()
try:
# 临界区代码
print("Critical section with semaphore")
finally:
semaphore.release()
# 创建线程
for i in range(10):
thread = threading.Thread(target=func)
thread.start()
```
以上示例中,信号量被初始化为5,意味着最多同时可以有5个线程进入临界区。`semaphore.acquire()` 方法在信号量计数器为0时会阻塞,直到计数器大于0。
#### 2.1.3 条件变量(Condition)
条件变量是线程同步中较为复杂的结构,它允许线程在某个条件成立之前一直挂起等待,当其他线程改变条件状态时,条件变量可以通知等待的线程。
条件变量通常与互斥锁一起使用。它提供 `wait()` 方法使线程等待,`notify()` 或 `notify_all()` 方法唤醒等待的线程。
代码示例:
```python
import threading
condition = threading.Condition()
lock = condition.lock
def wait_for_condition():
with lock:
condition.wait() # 线程进入等待状态
print("Condition met")
def signal_condition():
with lock:
print("Signaling condition")
condition.notify() # 唤醒一个等待的线程
# 创建线程
waiter = threading.Thread(target=wait_for_condition)
notifier = threading.Thread(target=signal_condition)
waiter.start()
notifier.start()
```
在上述代码中,`wait_for_condition` 线程将等待条件被满足,而 `signal_condition` 线程将通过 `notify()` 方法唤醒 `wait_for_condition` 线程。注意使用 `with` 语句来确保互斥锁的正确释放。
### 2.2 线程间通信机制
#### 2.2.1 队列(Queue)
队列(Queue)是Python中的一个线程安全的数据结构,它是线程间通信的一种简单方式。队列可以被多个生产者和消费者线程安全地访问,生产者可以向队列中添加数据,而消费者可以从队列中取出数据。
Python中的 `queue` 模块提供了线程安全的队列实现。其中 `Queue` 类是先进先出(FIFO)的队列,`LifoQueue` 是后进先出(LIFO),而 `PriorityQueue` 是优先级队列。
代码示例:
```python
import threading
import queue
q = queue.Queue(maxsize=0)
def producer():
for i in range(5):
item = f'item{i}'
print(f'Producing {item}')
q.put(item)
def consumer():
while True:
item = q.get()
print(f'Consuming {item}')
q.task_done()
# 创建生产者和消费者线程
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
consumer_thread.start()
producer_thread.join()
q.join() # 等待队列被消费完毕
```
以上代码中,生产者线程将生成数据并将其放入队列中,而消费者线程则不断从队列中取出数据进行消费。`q.join()` 方法用于等待所有项目被处理完毕。
#### 2.2.2 事件(Event)
事件(Event)是线程间通信的另一种机制,允许一个线程向其他线程发送信号,告知其发生的某些事件。事件对象有一个内部标志位,可以被置为True或False。
`threading` 模块中的 `Event` 类提供了事件的实现。线程可以调用 `wait()` 方法等待事件被设置为True,而设置事件可以使用 `set()` 方法。
代码示例:
```python
import threading
event = threading.Event()
def wait_for_event():
print("Waiting for the event to be set")
event.wait()
print("Event has occurred
```
0
0
相关推荐
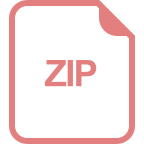
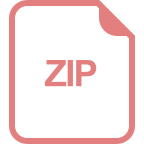





