python使用线程监控某个方法执行超时
时间: 2023-09-06 22:08:50 浏览: 52
可以使用Python中的`threading`模块来实现线程监控某个方法执行超时。具体的实现步骤如下:
1. 定义一个新的线程类,该类需要继承自`threading.Thread`;
2. 在该线程类的`run`方法中调用需要监控超时的方法;
3. 在该线程类中定义一个`stop`方法,用于停止线程执行;
4. 在该线程类的构造函数中设置一个计时器,用于监控方法的执行时间;
5. 在主线程中创建一个新的线程对象,并启动该线程;
6. 在主线程中使用`join`方法等待子线程执行完毕,或者在子线程执行超时后调用`stop`方法停止子线程的执行。
以下是一个简单的示例代码:
```python
import threading
class MonitorThread(threading.Thread):
def __init__(self, target_func, timeout):
super().__init__()
self.target_func = target_func
self.timeout = timeout
self.is_timeout = False
def run(self):
timer = threading.Timer(self.timeout, self.stop)
timer.start()
self.target_func()
timer.cancel()
def stop(self):
self.is_timeout = True
def target_func():
# 需要监控超时的方法
pass
monitor_thread = MonitorThread(target_func, 10) # 监控10秒超时
monitor_thread.start()
monitor_thread.join() # 等待子线程执行完毕
if monitor_thread.is_timeout:
# 子线程执行超时
pass
else:
# 子线程执行正常
pass
```
相关推荐
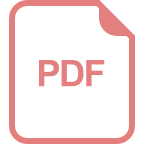
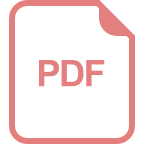
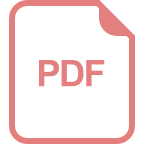
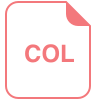
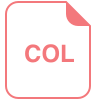
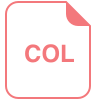
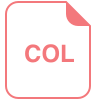










