Python count()函数在Web开发中的实战秘籍:统计网站访问量与用户行为,优化用户体验
发布时间: 2024-06-25 05:36:58 阅读量: 9 订阅数: 15 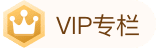
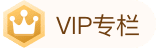
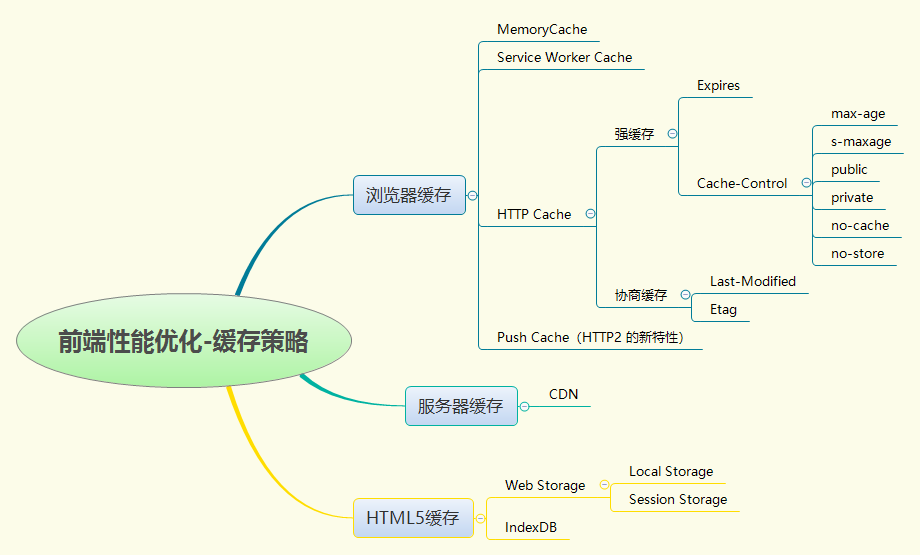
# 1. Python count()函数简介
Python 中的 `count()` 函数是一个用于统计序列中特定元素出现次数的内置函数。它接受一个序列(如列表、元组或字符串)作为参数,并返回该元素在序列中出现的次数。`count()` 函数在 Web 开发中有着广泛的应用,从统计网站访问量到分析用户行为。
`count()` 函数的语法非常简单:
```python
count(element)
```
其中 `element` 是要统计其出现次数的元素。例如,要统计列表 `[1, 2, 3, 4, 2, 5]` 中数字 2 出现的次数,可以使用以下代码:
```python
list = [1, 2, 3, 4, 2, 5]
count = list.count(2)
print(count) # 输出:2
```
# 2. count()函数在Web开发中的实战应用
### 2.1 统计网站访问量
#### 2.1.1 使用count()函数统计页面访问次数
在Web开发中,统计网站访问量是衡量网站性能和用户参与度的重要指标。Python的count()函数可以用来轻松地统计特定页面或整个网站的访问次数。
```python
from flask import Flask, request
app = Flask(__name__)
@app.route('/')
def index():
# 统计访问次数
count = request.cookies.get('count', 0)
count = int(count) + 1
# 更新访问次数
response = app.make_response('Hello, World!')
response.set_cookie('count', str(count))
return response
```
这段代码使用Flask框架创建一个简单的Web应用程序。当用户访问网站时,index()函数会检查名为"count"的cookie。如果cookie不存在,则将其设置为0。然后,它将cookie中的值转换为整数,将其加1,并将其作为新的cookie值返回给用户。
#### 2.1.2 结合其他函数分析访问趋势
除了统计访问次数外,count()函数还可以与其他函数结合使用来分析访问趋势。例如,可以结合datetime模块中的date()和time()函数来统计特定日期或时间段内的访问次数。
```python
from flask import Flask, request
from datetime import date, time
app = Flask(__name__)
@app.route('/')
def index():
# 统计访问次数
count = request.cookies.get('count', 0)
count = int(count) + 1
# 获取当前日期和时间
today = date.today()
now = time()
# 更新访问次数
response = app.make_response('Hello, World!')
response.set_cookie('count', str(count))
return response
@app.route('/stats')
def stats():
# 从数据库中获取访问次数数据
visits = db.session.query(Visit).filter(Visit.date == today).all()
# 统计特定时间段内的访问次数
start_time = time(hour=10, minute=0)
end_time = time(hour=12, minute=0)
visits_in_time_range = [visit for visit in visits if start_time <= visit.time <= end_time]
return render_template('stats.html', visits=visits, visits_in_time_range=visits_in_time_range)
```
这段代码扩展了前面的示例,它使用SQLAlchemy来存储访问次数数据。stats()函数从数据库中获取特定日期的访问
0
0
相关推荐
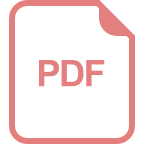
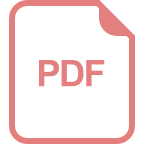
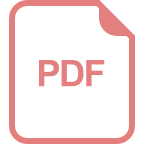





