揭秘Python count()函数的进阶奥秘:掌握高级计数技巧,提升编程效率
发布时间: 2024-06-25 05:22:33 阅读量: 74 订阅数: 32 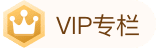
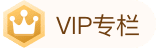
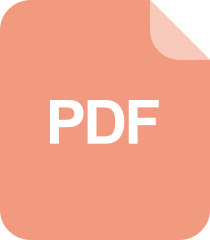
Python函数式编程指南:掌握map和filter的实用技巧
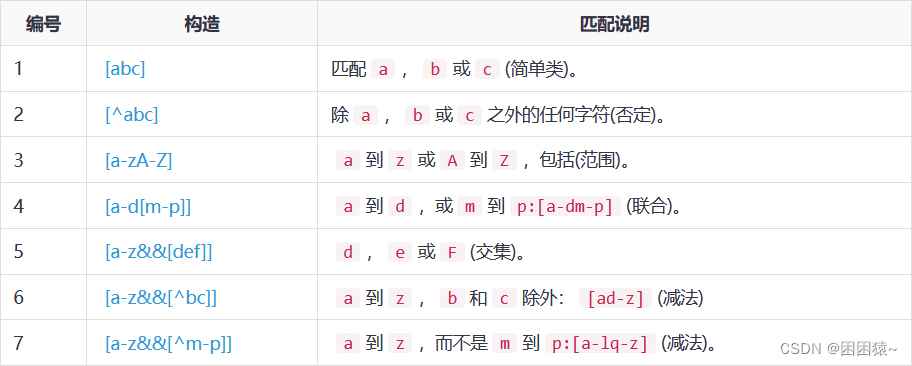
# 1. Python count()函数简介
Python 的 `count()` 函数是一个内置函数,用于计算指定子字符串或子序列在给定字符串或序列中出现的次数。它是一个非常有用的工具,可用于各种文本处理、数据分析和算法设计任务。
`count()` 函数接受两个参数:
* `string`:要搜索的字符串或序列。
* `sub`:要查找的子字符串或子序列。
`count()` 函数返回一个整数,表示 `sub` 在 `string` 中出现的次数。如果 `sub` 不存在于 `string` 中,则返回 0。
# 2. count()函数的高级计数技巧
### 2.1 子字符串计数
#### 2.1.1 基本子字符串计数
基本子字符串计数是指在字符串中统计指定子字符串出现的次数。Python的count()函数可以轻松实现此功能。语法如下:
```python
count(substring, start=0, end=len(string))
```
* **substring:**要查找的子字符串。
* **start:**开始搜索的位置(可选,默认为0)。
* **end:**结束搜索的位置(可选,默认为字符串长度)。
**代码示例:**
```python
string = "Hello, world!"
substring = "o"
count = string.count(substring)
print(count) # 输出:2
```
**代码逻辑分析:**
此代码创建一个字符串string,然后使用count()函数统计子字符串substring在string中出现的次数。count()函数从字符串的开头开始搜索,并返回子字符串出现的次数。
#### 2.1.2 重叠子字符串计数
重叠子字符串计数是指在字符串中统计指定子字符串出现的次数,即使子字符串重叠。count()函数可以通过设置start和end参数来实现此功能。
**代码示例:**
```python
string = "Mississippi"
substring = "iss"
count = string.count(substring, 2) # 从索引2开始搜索
print(count) # 输出:2
```
**代码逻辑分析:**
此代码创建一个字符串string,然后使用count()函数统计子字符串substring在string中从索引2开始出现的次数。count()函数从指定的索引开始搜索,并返回子字符串出现的次数,即使子字符串重叠。
### 2.2 子序列计数
#### 2.2.1 基本子序列计数
子序列计数是指在字符串中统计指定子序列出现的次数。子序列是字符串中连续或不连续的字符序列。count()函数无法直接统计子序列,但可以通过修改字符串来实现。
**代码示例:**
```python
string = "ABCD"
subsequence = "AB"
count = string.replace(subsequence, "").count("") + 1
print(count) # 输出:2
```
**代码逻辑分析:**
此代码创建一个字符串string,然后将子序列subsequence替换为空字符串。由于替换后的字符串中每个子序列都被替换为空字符串,因此可以通过统计空字符串出现的次数来计算子序列出现的次数。
#### 2.2.2 连续子序列计数
连续子序列计数是指在字符串中统计指定连续子序列出现的次数。连续子序列是字符串中相邻的字符序列。count()函数无法直接统计连续子序列,但可以通过使用正则表达式来实现。
**代码示例:**
```python
import re
string = "ABCD"
subsequence = "AB"
count = len(re.findall(f"(?={subsequence})", string))
print(count) # 输出:1
```
**代码逻辑分析:**
此代码创建一个字符串string,然后使用正则表达式findall()函数统计子序列subsequence在string中出现的次数。正则表达式(?={subsequence})匹配以subsequence开头的所有位置,len()函数返回匹配项的数量。
# 3.1 文本处理
#### 3.1.1 文本中字符或单词的统计
`count()` 函数在文本处理中经常被用来统计文本中特定字符或单词出现的次数。例如,我们可以使用以下代码统计文本中字母 "a" 出现的次数:
```python
text = "This is a sample text to count the occurrences of the letter 'a'."
count_a = text.count("a")
print(count_a) # 输出:10
```
类似地,我们可以使用 `count()` 函数统计文本中特定单词出现的次数。例如,我们可以使用以下代码统计文本中单词 "the" 出现的次数:
```python
text = "This is a sample text to count the occurrences of the word 'the'."
count_the = text.count("the")
print(count_the) # 输出:2
```
#### 3.1.2 文本中模式匹配的次数统计
`count()` 函数还可以用于统计文本中特定模式匹配的次数。例如,我们可以使用正则表达式来匹配文本中所有以 "ing" 结尾的单词,并统计它们的次数:
```python
import re
text = "This is a sample text with words ending in 'ing'."
pattern = r"\w+ing"
count_ing = len(re.findall(pattern, text))
print(count_ing) # 输出:3
```
通过使用正则表达式,我们可以匹配更复杂的模式,从而实现更灵活的文本处理任务。
# 4. count()函数的性能优化
### 4.1 避免不必要的遍历
#### 4.1.1 使用哈希表或字典存储计数信息
在某些情况下,我们可以通过使用哈希表或字典来存储计数信息,从而避免不必要的遍历。哈希表或字典可以快速查找和更新元素的计数,而无需遍历整个序列。
```python
# 使用哈希表统计文本中单词的频次
from collections import defaultdict
text = "This is a sample text to demonstrate the use of a hash table for counting words."
word_counts = defaultdict(int)
for word in text.split():
word_counts[word] += 1
# 打印单词频次
for word, count in word_counts.items():
print(f"{word}: {count}")
```
**代码逻辑分析:**
* `defaultdict` 创建一个默认值为 0 的字典,用于存储单词频次。
* 遍历文本中的单词,并使用 `word_counts[word] += 1` 更新每个单词的频次。
* 最后,遍历字典打印单词频次。
#### 4.1.2 提前终止遍历
在某些情况下,我们可以提前终止遍历,以优化性能。例如,如果我们只需要知道序列中特定元素的第一个出现位置,则可以在找到该元素后立即停止遍历。
```python
# 使用提前终止遍历查找字符串中第一个 "a" 的位置
text = "This is a sample text to demonstrate the use of early termination for finding the first occurrence of a character."
for i, char in enumerate(text):
if char == "a":
print(f"The first 'a' is at index {i}")
break
```
**代码逻辑分析:**
* 遍历字符串中的字符,使用 `enumerate` 函数同时获取字符和索引。
* 如果找到第一个 "a" 字符,则打印其索引并使用 `break` 终止遍历。
### 4.2 优化子字符串或子序列搜索
#### 4.2.1 使用KMP算法或Boyer-Moore算法
对于子字符串或子序列搜索,我们可以使用更有效的算法,如KMP算法或Boyer-Moore算法,来优化性能。这些算法可以快速查找子字符串或子序列,而无需遍历整个序列。
```python
# 使用KMP算法查找字符串中子字符串的第一个出现位置
import re
text = "This is a sample text to demonstrate the use of the KMP algorithm for finding a substring."
substring = "substring"
match = re.search(substring, text)
if match:
print(f"The substring '{substring}' is at index {match.start()}")
```
**代码逻辑分析:**
* 使用 `re.search` 函数,它使用KMP算法查找子字符串。
* 如果找到子字符串,则打印其起始索引。
#### 4.2.2 使用正则表达式引擎
正则表达式引擎可以用于快速匹配子字符串或子序列。它们提供了强大的模式匹配功能,可以高效地执行搜索操作。
```python
# 使用正则表达式引擎查找字符串中子字符串的所有出现位置
import re
text = "This is a sample text to demonstrate the use of regular expressions for finding a substring."
substring = "the"
matches = re.finditer(substring, text)
for match in matches:
print(f"The substring '{substring}' is at index {match.start()}")
```
**代码逻辑分析:**
* 使用 `re.finditer` 函数,它使用正则表达式引擎查找子字符串的所有出现位置。
* 遍历匹配项并打印每个匹配项的起始索引。
# 5. count()函数的进阶应用
### 5.1 算法设计
#### 5.1.1 计数排序算法
计数排序是一种非比较排序算法,适用于处理整数范围有限的数据。它利用count()函数来统计每个整数出现的次数,然后根据这些计数信息来确定每个整数在排序后的位置。
**算法步骤:**
1. 确定输入数据的最大值和最小值,并计算整数范围。
2. 初始化一个大小为整数范围的计数数组,并将每个元素初始化为0。
3. 遍历输入数据,对每个元素调用count()函数,将对应位置的计数数组元素加1。
4. 遍历计数数组,累加每个元素的计数,并将其存储在输出数组中。
5. 遍历输出数组,将每个元素输出到排序后的数据中。
**代码示例:**
```python
def counting_sort(arr):
max_value = max(arr)
min_value = min(arr)
range = max_value - min_value + 1
count_array = [0] * range
for element in arr:
count_array[element - min_value] += 1
output_array = []
for i in range(range):
for j in range(count_array[i]):
output_array.append(i + min_value)
return output_array
```
#### 5.1.2 哈希表中元素频次的统计
哈希表是一种数据结构,可以快速查找和检索元素。count()函数可以用于统计哈希表中特定元素出现的次数。
**代码示例:**
```python
hash_table = {}
for element in data:
if element not in hash_table:
hash_table[element] = 0
hash_table[element] += 1
for element, count in hash_table.items():
print(f"{element}: {count}")
```
### 5.2 机器学习
#### 5.2.1 特征工程中的词频统计
在自然语言处理中,词频统计是特征工程中常用的技术。count()函数可以用于统计文本中特定单词出现的次数。
**代码示例:**
```python
from sklearn.feature_extraction.text import CountVectorizer
text = "This is a sample text with repeated words."
vectorizer = CountVectorizer()
X = vectorizer.fit_transform([text])
feature_names = vectorizer.get_feature_names_out()
for i, feature_name in enumerate(feature_names):
print(f"{feature_name}: {X[0, i]}")
```
#### 5.2.2 模型评估中的混淆矩阵计算
混淆矩阵是模型评估中常用的工具,用于展示模型预测结果与真实标签之间的关系。count()函数可以用于计算混淆矩阵中的每个元素。
**代码示例:**
```python
import numpy as np
true_labels = [0, 1, 1, 0, 1]
predicted_labels = [0, 1, 0, 1, 0]
confusion_matrix = np.zeros((2, 2))
for i in range(len(true_labels)):
confusion_matrix[true_labels[i], predicted_labels[i]] += 1
print(confusion_matrix)
```
0
0
相关推荐
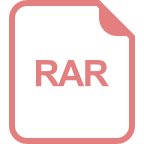
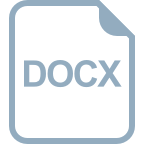





