计算机视觉实战指南:图像识别、目标检测与图像分割,3步掌握核心技术
发布时间: 2024-08-26 04:19:17 阅读量: 33 订阅数: 25 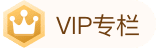
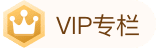
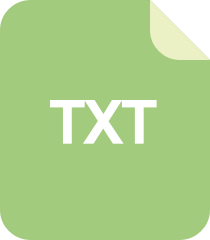
人工智能AI进阶-人工智能课件-计算机视觉与图像处理
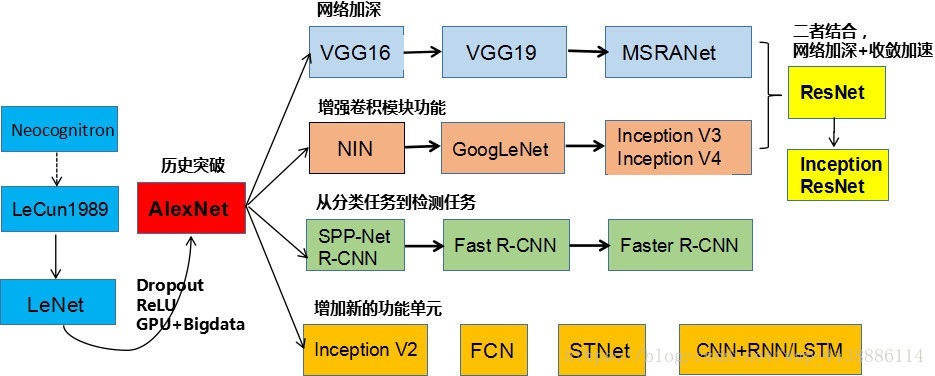
# 1. 计算机视觉基础
计算机视觉是人工智能的一个分支,它使计算机能够“理解”图像和视频。它涉及从图像和视频中提取有意义的信息,并将其用于各种任务,如图像识别、目标检测和图像分割。
计算机视觉的基础是图像处理,它涉及对图像进行一系列操作,如去噪、增强和转换,以提取有用的信息。图像处理技术包括直方图均衡、边缘检测和图像分割。
计算机视觉算法利用图像处理技术从图像中提取特征。这些特征可以是颜色、纹理、形状或其他可用于区分不同对象或场景的属性。通过分析这些特征,计算机视觉算法可以执行各种任务,如识别图像中的对象、检测图像中的目标并分割图像中的不同区域。
# 2. 图像识别
### 2.1 图像识别算法
图像识别算法是计算机视觉中用于识别图像中对象的算法。这些算法可以分为传统算法和深度学习算法。
#### 2.1.1 传统图像识别算法
传统图像识别算法使用手工设计的特征提取器和分类器来识别图像中的对象。这些特征提取器通常基于图像的形状、纹理和颜色。分类器然后使用这些特征来将图像分类为不同的类。
**优点:**
* 计算成本低
* 对图像噪声和失真具有鲁棒性
**缺点:**
* 难以处理复杂图像
* 需要针对特定任务进行手动调整
#### 2.1.2 深度学习图像识别算法
深度学习图像识别算法使用卷积神经网络(CNN)来识别图像中的对象。CNN 是一种神经网络,它可以自动从图像中学习特征。这些特征然后被用于对图像进行分类。
**优点:**
* 可以处理复杂图像
* 可以自动学习特征
* 在图像识别任务上取得了最先进的性能
**缺点:**
* 计算成本高
* 需要大量训练数据
### 2.2 图像识别应用
图像识别技术在各种应用中都有广泛的应用,包括:
#### 2.2.1 人脸识别
人脸识别系统使用图像识别算法来识别图像中的人脸。这些系统可以用于安全、访问控制和执法等应用。
#### 2.2.2 物体识别
物体识别系统使用图像识别算法来识别图像中的物体。这些系统可以用于零售、制造和医疗保健等应用。
#### 2.2.3 场景识别
场景识别系统使用图像识别算法来识别图像中的场景。这些系统可以用于自动驾驶、机器人和虚拟现实等应用。
**代码示例:**
```python
import cv2
# 加载图像
image = cv2.imread("image.jpg")
# 转换图像为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 使用 OpenCV 的 Haar 级联分类器检测人脸
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + "haarcascade_frontalface_default.xml")
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
# 在图像上绘制人脸边界框
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 显示图像
cv2.imshow("Faces detected", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码逻辑分析:**
* `cv2.imread()` 函数加载图像并将其存储在 `image` 变量中。
* `cv2.cvtColor()` 函数将图像转换为灰度图像,因为 Haar 级联分类器需要灰度图像。
* `cv2.CascadeClassifier()` 函数加载 Haar 级联分类器,用于检测人脸。
* `face_cascade.detectMultiScale()` 函数使用 Haar 级联分类器检测图像中的人脸。
* 循环遍历检测到的人脸,并在图像上绘制边界框。
* `cv2.imshow()` 函数显示图像。
* `cv2.waitKey()` 函数等待用户按任意键。
* `cv2.destroyAllWindows()` 函数关闭所有 OpenCV 窗口。
# 3. 目标检测
### 3.1 目标检测算法
目标检测旨在定位图像或视频中感兴趣的对象。其核心算法分为三大类:
**3.1.1 滑动窗口算法**
滑动窗口算法通过在图像上滑动一个预定义大小的窗口,并对每个窗口进行分类,来检测对象。优点是简单易懂,但缺点是计算量大,效率低。
```python
import cv2
# 定义滑动窗口大小
window_size = (100, 100)
# 遍历图像
for x in range(0, image.shape[1] - window_size[0]):
for y in range(0, image.shape[0] - window_size[1]):
# 获取滑动窗口内的图像区域
window = image[y:y+window_size[1], x:x+window_size[0]]
# 对滑动窗口内的图像进行分类
label = classifier.predict(window)
# 如果分类结果为目标,则标记目标位置
if label == "target":
cv2.rectangle(image, (x, y), (x+window_size[0], y+window_size[1]), (0, 255, 0), 2)
```
**3.1.2 区域生成算法**
区域生成算法通过生成一组候选区域,然后对这些区域进行分类,来检测对象。优点是速度较快,但缺点是生成的候选区域数量较多,导致计算量仍然较大。
```python
import cv2
import numpy as np
# 生成候选区域
candidate_regions = generate_candidate_regions(image)
# 对候选区域进行分类
labels = classifier.predict(candidate_regions)
# 筛选出目标候选区域
target_regions = [region for region, label in zip(candidate_regions, labels) if label == "target"]
# 标记目标位置
for region in target_regions:
cv2.rectangle(image, region[0], region[1], (0, 255, 0), 2)
```
**3.1.3 单次射击算法**
单次射击算法将目标检测问题转化为回归问题,直接预测目标的边界框坐标。优点是速度极快,但缺点是需要大量训练数据,且对目标形状和大小敏感。
```python
import cv2
import tensorflow as tf
# 加载预训练模型
model = tf.keras.models.load_model("ssd_mobilenet_v2_coco.h5")
# 对图像进行预处理
image = cv2.resize(image, (300, 300))
image = image / 255.0
# 预测目标边界框坐标
predictions = model.predict(np.expand_dims(image, axis=0))
# 解析预测结果
for prediction in predictions[0]:
if prediction[2] > 0.5:
cv2.rectangle(image, (int(prediction[3] * image.shape[1]), int(prediction[4] * image.shape[0])),
(int(prediction[5] * image.shape[1]), int(prediction[6] * image.shape[0])), (0, 255, 0), 2)
```
### 3.2 目标检测应用
目标检测技术广泛应用于各种领域,包括:
**3.2.1 行人检测**
行人检测是目标检测的一个重要应用,用于检测图像或视频中的人。它在安防监控、自动驾驶等领域有广泛应用。
**3.2.2 车辆检测**
车辆检测是目标检测的另一个重要应用,用于检测图像或视频中的车辆。它在交通管理、自动驾驶等领域有广泛应用。
**3.2.3 目标跟踪**
目标跟踪是目标检测的延伸,用于跟踪图像或视频中移动的目标。它在安防监控、自动驾驶等领域有广泛应用。
# 4. 图像分割
### 4.1 图像分割算法
图像分割是将图像分解为多个不同区域的过程,每个区域代表图像中的一个不同对象或区域。图像分割算法有多种,可根据不同的原则进行分类。
#### 4.1.1 基于阈值的分割
基于阈值的分割是一种简单的图像分割算法,它将图像中的像素分为两类:前景和背景。算法首先选择一个阈值,然后将所有像素值大于阈值的像素分类为前景,而所有像素值小于阈值的像素分类为背景。
**代码块:**
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 选择阈值
threshold = 127
# 基于阈值分割
segmented_image = cv2.threshold(gray, threshold, 255, cv2.THRESH_BINARY)[1]
# 显示分割后的图像
cv2.imshow('Segmented Image', segmented_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.imread('image.jpg')`:读取图像文件。
* `cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)`:将图像转换为灰度图像。
* `threshold = 127`:选择阈值。
* `cv2.threshold(gray, threshold, 255, cv2.THRESH_BINARY)[1]`:基于阈值进行分割,将大于阈值的像素设置为 255(白色),小于阈值的像素设置为 0(黑色)。
* `cv2.imshow('Segmented Image', segmented_image)`:显示分割后的图像。
#### 4.1.2 基于区域的分割
基于区域的分割算法将图像中的像素分组为具有相似特性的区域。这些算法通常使用图像的直方图或纹理信息来确定区域边界。
**代码块:**
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 基于区域的分割
segmented_image = cv2.watershed(gray, None)
# 显示分割后的图像
cv2.imshow('Segmented Image', segmented_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.watershed(gray, None)`:基于区域的分割,使用 Watershed 算法。
* `None`:指定没有预先定义的标记。
#### 4.1.3 基于边缘的分割
基于边缘的分割算法检测图像中的边缘,然后使用边缘信息来分割图像。这些算法通常使用梯度或拉普拉斯算子来检测边缘。
**代码块:**
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 基于边缘的分割
edges = cv2.Canny(gray, 100, 200)
# 显示分割后的图像
cv2.imshow('Segmented Image', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.Canny(gray, 100, 200)`:基于边缘的分割,使用 Canny 算法。
* `100` 和 `200`:Canny 算法的两个阈值,用于检测弱边缘和强边缘。
# 5. 计算机视觉实践**
**5.1 图像识别实践**
**5.1.1 OpenCV人脸识别**
**步骤:**
1. **导入必要的库:**
```python
import cv2
import numpy as np
```
2. **加载人脸检测模型:**
```python
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
```
3. **读取图像:**
```python
image = cv2.imread('image.jpg')
```
4. **灰度化图像:**
```python
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
```
5. **人脸检测:**
```python
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
```
* 参数说明:
* `gray`:灰度化图像
* `1.1`:缩放因子
* `4`:最小邻域大小
6. **绘制人脸框:**
```python
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (0, 255, 0), 2)
```
7. **显示结果:**
```python
cv2.imshow('Detected Faces', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**5.1.2 TensorFlow物体识别**
**步骤:**
1. **安装TensorFlow:**
```
pip install tensorflow
```
2. **导入必要的库:**
```python
import tensorflow as tf
from tensorflow.keras.preprocessing.image import load_img, img_to_array
```
3. **加载预训练模型:**
```python
model = tf.keras.models.load_model('object_detection_model.h5')
```
4. **读取图像:**
```python
image = load_img('image.jpg', target_size=(224, 224))
```
5. **预处理图像:**
```python
image = img_to_array(image)
image = np.expand_dims(image, axis=0)
```
6. **预测物体:**
```python
predictions = model.predict(image)
```
7. **获取物体标签和置信度:**
```python
class_names = ['apple', 'banana', 'orange']
for i in range(len(predictions)):
print(f"Class: {class_names[i]}, Confidence: {predictions[i][0]}")
```
# 6.1 计算机视觉的发展趋势
计算机视觉领域正在快速发展,以下是一些关键的发展趋势:
- **深度学习的持续进步:**深度学习算法在图像识别、目标检测和图像分割等计算机视觉任务中取得了显著的成功。随着计算能力的不断提升,深度学习模型将变得更加强大和复杂。
- **边缘计算的兴起:**边缘计算将计算任务从云端转移到设备端,从而减少延迟并提高效率。这将使计算机视觉应用在实时和资源受限的环境中得到广泛应用。
- **自动化机器学习(AutoML):**AutoML工具简化了计算机视觉模型的开发和部署,使非专业人员也能轻松构建和使用计算机视觉解决方案。
- **跨模态人工智能:**计算机视觉正在与其他人工智能领域,如自然语言处理和语音识别相结合,创造出更强大的多模态人工智能系统。
- **增强现实和虚拟现实:**计算机视觉在增强现实和虚拟现实应用中发挥着至关重要的作用,为用户提供身临其境的体验。
## 6.2 计算机视觉的应用前景
计算机视觉技术具有广泛的应用前景,包括:
- **安防和监控:**人脸识别、目标检测和行为分析等计算机视觉技术在安防和监控系统中得到广泛应用。
- **医疗保健:**计算机视觉用于医学图像分析、疾病诊断和手术辅助,提高医疗保健的效率和准确性。
- **零售和电子商务:**物体识别、图像搜索和虚拟试衣等计算机视觉技术为零售和电子商务行业带来了新的购物体验。
- **工业自动化:**计算机视觉用于缺陷检测、机器人引导和质量控制,提高工业生产的效率和安全性。
- **自动驾驶:**计算机视觉是自动驾驶汽车的关键技术,用于环境感知、物体识别和路径规划。
0
0
相关推荐
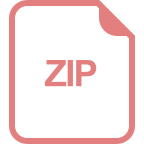
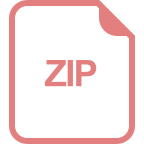
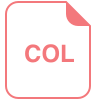
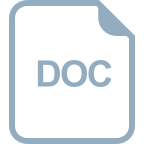
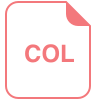
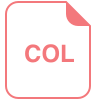
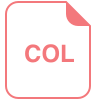
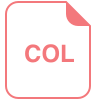