Java中的线程间通信机制
发布时间: 2024-02-21 18:23:30 阅读量: 27 订阅数: 12 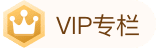
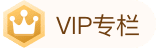
# 1. 理解Java中的多线程编程
在Java编程中,多线程是一项十分重要的技术,能够充分利用计算机的多核处理器和CPU资源,提高程序的执行效率。本章将介绍多线程编程的基本概念,Java中的线程实现方式,以及多线程编程所面临的优势和挑战。
### 多线程概念
在计算机科学中,线程是程序执行流的最小单元,一个进程可以包含多个线程。多线程让程序能够同时执行多个任务,提高了程序的运行效率。
### Java中的线程实现方式
在Java中,线程可以通过继承Thread类或实现Runnable接口来创建。另外,Java 5之后引入了Executor框架和线程池,简化了多线程编程。
### 多线程编程的优势和挑战
多线程编程可以提高程序的并发性和响应速度,但也会引入一些问题,如线程安全、死锁等。开发人员需要合理设计和管理线程,确保程序的正确性和性能。
在接下来的章节中,我们将深入探讨Java中线程间通信的机制和实现方法。
# 2. 线程间通信的基本概念
在多线程编程中,线程间通信是一个非常重要的概念。简单来说,线程间通信指的是多个线程之间通过共享的内存区域进行数据交换和协调工作。在实际开发中,线程间通信的作用至关重要,可以帮助不同线程之间协调工作顺序、共享数据、提高程序效率等。
### 什么是线程间通信
线程间通信是指在多线程编程中,多个线程之间通过协调、合作和数据交换来完成某项任务。线程通信是实现线程同步的重要手段,可以有效避免线程之间的不确定性和混乱,并确保程序的正确性和稳定性。
### 为什么需要线程间通信
在多线程编程中,不同线程通常需要协调工作、共享数据、避免竞争条件等。而线程间通信正是实现这些目标的关键。通过线程间通信,可以确保线程按照预期的顺序执行,共享数据不会出现异常,避免竞争条件带来的问题。
### Java中线程间通信的基本原则
在Java中,线程间通信的基本原则包括:
- 等待/通知机制:Java提供了wait()和notify()方法来实现线程间的等待和通知机制,可以帮助线程之间协调工作。
- 同步机制:使用同步关键字synchronized或者Lock接口来确保多个线程安全地访问共享资源,避免数据竞争和不一致性。
- 共享数据:线程间通信通常涉及共享数据,需要注意数据的一致性和线程安全性。
通过遵循这些基本原则,可以有效实现Java中线程间通信的机制,确保多线程程序的正确性和稳定性。
# 3. Java中的线程同步机制
在多线程编程中,线程同步是非常重要的概念。它可以确保多个线程按照合适的顺序访问共享资源,避免了数据的混乱和不一致。本章将介绍Java中的线程同步机制,包括同步的概念和作用,Java中的同步关键字synchronized,以及同步代码块和同步方法的具体应用。
#### 同步的概念和作用
在多线程环境下,多个线程访问共享资源可能会导致数据不一致的问题,例如同时对同一变量进行读写操作。而同步机制可以通过协调多个线程的执行顺序,来确保线程安全,避免数据竞争和冲突。
#### Java中的同步关键字synchronized
在Java中,通过使用synchronized关键字可以实现对代码块或方法的同步。当某个线程获取了对象的锁之后,其他需要这个锁的线程必须等待,直到锁被释放。
#### 同步代码块和同步方法
除了使用synchronized关键字修饰方法外,还可以使用同步代码块来实现对共享资源的同步访问。同步代码块通过指定一个对象作为锁,来保护一个代码块,只有获得了锁的线程才能执行该代码块。
以上是Java中线程同步机制的基本概念和使用方法,接下来我们将深入讨论如何利用这些机制来实现线程间的通信。
# 4. 使用wait()和notify()实现线程间通信
在Java中,线程间通信可以通过wait()和notify()方法来实现。这两个方法是Object类的方法,意味着所有Java对象都可以调用这些方法来实现线程同步。
- **wait()、notify()和notifyAll()的作用:**
- `wait()`: 让当前线程暂时释放对象锁,进入等待状态,直到其他线程调用notify()或notifyAll()方法唤醒它。
- `notify()`: 唤醒一个正在等待该对象锁的线程,如果有多个线程在等待,那么哪一个被唤醒是不确定的。
- `notifyAll()`: 唤醒所有正在等待该对象锁的线程,让它们竞争对象锁。
- **如何在Java中使用wait()和notify()方法进行线程间通信:**
在使用wait()和notify()方法时,需要注意以下几点:
1. 必须在同步方法或同步代码块中调用wait()和notify()方法。
2. 调用wait()会让当前线程释放对象锁,进入等待状态。
3. 调用notify()会唤醒一个正在等待的线程,如果有多个线程在等待,哪一个会被唤醒是不确定的。
4. 等待和唤醒的线程必须是同一个锁对象。
下面是一个简单的示例代码,演示如何使用wait()和notify()方法实现线程间通信:
```java
class Message {
private String content;
public synchronized String read() {
while (content == null) {
try {
wait(); // 当内容为空时,线程进入等待状态
} catch (InterruptedException e) {
e.printStackTrace();
}
}
String message = content;
content = null;
notify(); // 通知其他线程可以写入内容
return message;
}
public synchronized void write(String message) {
while (content != null) {
try {
wait(); // 当内容不为空时,线程进入等待状态
} catch (InterruptedException e) {
e.printStackTrace();
}
}
content = message;
notify(); // 通知其他线程可以读取内容
}
}
public class CommunicationExample {
public static void main(String[] args) {
Message message = new Message();
Thread reader = new Thread(() -> {
System.out.println("Reader is waiting to read...");
System.out.println("Message received: " + message.read());
});
Thread writer = new Thread(() -> {
System.out.println("Writer is writing the message...");
message.write("Hello, there!");
});
writer.start();
reader.start();
}
}
```
在上面的示例中,Message类表示一个消息对象,read()方法用于读取消息,write()方法用于写入消息。两个线程分别负责读取和写入消息,通过wait()和notify()方法实现线程间通信。在main方法中创建了一个Message对象,并启动了读取和写入线程,实现了简单的线程通信功能。
这就是使用wait()和notify()方法实现线程间通信的基本原理和示例代码。希望通过这个例子能更好地理解Java中线程间通信的实现方式。
# 5. 使用Lock和Condition实现线程间通信
在Java中,除了使用`synchronized`关键字外,还可以使用`Lock`接口和`Condition`接口来实现线程间通信。这种方式相比传统的`synchronized`方式更加灵活,可以更精细地控制线程的同步和互斥操作。
#### Java中的Lock接口和Condition接口介绍
- `Lock`接口是一个提供了锁功能的接口,通过`Lock`可以获取锁、释放锁以及创建`Condition`对象。常用的实现类为`ReentrantLock`。
- `Condition`接口是在使用`Lock`时,用于替代传统`synchronized`中的`wait()`和`notify()`机制的对象。每个`Condition`对象都与一个锁相关联,通过`Lock.newCondition()`方法创建。
#### 如何使用Lock和Condition实现线程间通信
下面是一个简单示例,演示如何使用`Lock`和`Condition`实现线程间通信:
```java
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class ThreadCommunicationUsingLock {
private final Lock lock = new ReentrantLock();
private final Condition condition = lock.newCondition();
private boolean isReady = false;
public void waitForSignal() throws InterruptedException {
lock.lock();
try {
while (!isReady) {
condition.await();
}
System.out.println("Received the signal!");
} finally {
lock.unlock();
}
}
public void sendSignal() {
lock.lock();
try {
isReady = true;
condition.signal();
System.out.println("Signal sent!");
} finally {
lock.unlock();
}
}
public static void main(String[] args) {
ThreadCommunicationUsingLock communication = new ThreadCommunicationUsingLock();
Thread thread1 = new Thread(() -> {
try {
communication.waitForSignal();
} catch (InterruptedException e) {
e.printStackTrace();
}
});
Thread thread2 = new Thread(() -> {
communication.sendSignal();
});
thread1.start();
thread2.start();
}
}
```
#### 与传统的synchronized方式相比的优势和适用场景
使用`Lock`和`Condition`方式相比传统`synchronized`方式的优势包括:
- 更灵活:`Lock`可以实现更细粒度的锁定,允许使用更多的同步策略。
- 更具有扩展性:`Lock`接口提供了更多高级的功能,如可轮询锁、定时锁等。
- 更安全:`Lock`接口能够避免了在`synchronized`中可能发生的死锁情况。
适用场景包括:
- 需要更细粒度控制的线程同步操作。
- 需要更多高级功能的同步操作。
- 对于某些特定场景下需要避免死锁问题的线程同步需求。
通过使用`Lock`和`Condition`实现线程间通信,我们可以更加灵活和安全地控制多线程环境下的数据共享和同步操作。
# 6. 理解线程间通信的常见问题与解决方案
在多线程编程中,线程间通信可能会引发一些常见的问题,如死锁和活锁。下面我们将分析这些问题,并探讨如何避免它们。
1. **死锁问题分析**
死锁是指两个或多个线程彼此等待对方释放所需资源而无法继续执行的情况。这种情况下,线程将永远阻塞,进程也无法终止。死锁常见于多个线程同时持有资源并且试图获取对方已持有的资源时。
下面是一个简单的死锁示例:
```java
public class DeadlockExample {
private static final Object lock1 = new Object();
private static final Object lock2 = new Object();
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
synchronized (lock1) {
System.out.println("Thread 1: Holding lock 1...");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {}
System.out.println("Thread 1: Waiting for lock 2...");
synchronized (lock2) {
System.out.println("Thread 1: Holding lock 1 and lock 2...");
}
}
});
Thread thread2 = new Thread(() -> {
synchronized (lock2) {
System.out.println("Thread 2: Holding lock 2...");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {}
System.out.println("Thread 2: Waiting for lock 1...");
synchronized (lock1) {
System.out.println("Thread 2: Holding lock 1 and lock 2...");
}
}
});
thread1.start();
thread2.start();
}
}
```
在以上代码中,两个线程分别持有lock1和lock2并试图获取对方持有的锁,从而造成死锁。
2. **避免线程通信中的常见问题**
为避免死锁和其他线程间通信中的常见问题,可以采取以下措施:
- **避免嵌套锁**:尽量减少对多个锁的依赖,减少死锁发生的可能性。
- **按序获取锁**:如果必须使用多个锁,尽量按相同的顺序获取锁,以避免不同线程获取锁的顺序不一致导致的死锁。
- **设置超时时间**:在获取锁的过程中设置超时时间,超时后释放资源,避免线程一直等待。
- **使用Lock接口**:Lock接口提供了更灵活的锁定机制,且可避免死锁等问题。
对于不同情况,还可以根据具体需求采取其他措施来避免线程通信中可能出现的问题。通过良好的设计和合理的策略,可以有效减少线程间通信引发的各类问题。
3. **示例代码与最佳实践建议**
为了更好地展示如何处理线程间通信中的常见问题,我们可以编写更加复杂的示例代码,并根据具体情况提出相应的解决方案。在实际开发中,根据具体需求和场景选择适当的线程通信方式以及避免常见问题的策略至关重要。
总的来说,理解线程间通信中可能出现的问题、学习相应的解决方案,并在实践中不断积累经验,将有助于开发者更加熟练地处理多线程编程中的挑战。
0
0
相关推荐





