面试官最爱:JavaScript数据结构与算法面试题的15个深度解析
发布时间: 2024-09-10 13:27:16 阅读量: 143 订阅数: 65 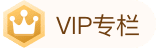
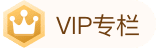
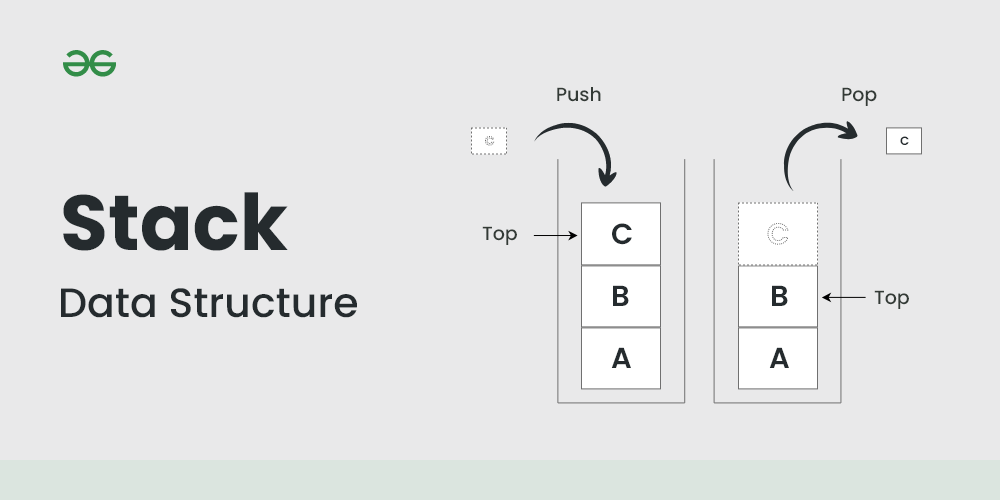
# 1. 数据结构与算法概述
数据结构与算法是计算机科学的基石,它们是实现高效程序设计的核心。本章将概述数据结构与算法的基本概念,提供一个理解它们的基础框架,并预览后续章节内容。
## 数据结构简介
数据结构是计算机存储、组织数据的方式。它能决定数据的访问效率和数据操作的复杂度。常见的数据结构包括数组、链表、栈、队列、树、图、哈希表等。它们各有适用的场景和特定的操作方法。
## 算法的定义和重要性
算法是对特定问题求解步骤的一种描述,它规定了解决问题的具体操作流程。算法的好坏直接影响到程序的运行效率和资源消耗。在面试中,算法能力往往是衡量一个程序员的重要标准。
## 数据结构与算法的关系
数据结构与算法密不可分。数据结构提供了算法操作的物理基础,而算法则决定了数据结构中数据的处理方式。理解它们之间的关系对于设计出高效的程序至关重要。
接下来的章节将深入探讨 JavaScript 中的数据结构和算法技巧,并结合实际案例分析如何将这些知识应用于实战中。
# 2. JavaScript中的基础数据结构
## 2.1 常见数据结构简介
### 2.1.1 数组与字符串
数组是JavaScript中最基本的数据结构之一,它是一种线性表结构,可以存储任意类型的数据。在JavaScript中,数组通过方括号`[]`来定义,并支持动态扩容。
字符串是数组的一个特殊形式,它只包含字符,并且通常用于文本数据处理。JavaScript中的字符串是不可变的,这意味着一旦字符串被创建,它所包含的字符就不能被更改。
```javascript
let arr = [1, 'hello', true]; // 定义一个包含不同类型元素的数组
let str = 'Hello, World!'; // 定义一个字符串
// 数组的操作示例
console.log(arr[0]); // 输出数组的第一个元素:1
// 字符串的操作示例
console.log(str.length); // 输出字符串的长度:13
console.log(str.slice(7)); // 输出从第8个字符开始到末尾的子字符串:"World!"
```
### 2.1.2 链表
链表是一种比数组更灵活的数据结构,它由一系列节点组成,每个节点都存储了数据部分和一个或多个指向其他节点的引用。在JavaScript中,链表的节点可以通过对象来实现。
```javascript
class ListNode {
constructor(value) {
this.value = value;
this.next = null;
}
}
let head = new ListNode(1);
head.next = new ListNode(2);
head.next.next = new ListNode(3);
// 遍历链表打印所有元素
let current = head;
while (current !== null) {
console.log(current.value);
current = current.next;
}
```
### 2.1.1和2.1.2小节的比较表格
| 数据结构 | 访问速度 | 动态扩容 | 内存使用 | 应用场景 |
|-------|-------|-------|-------|-------|
| 数组 | O(1) | 可以 | 较高 | 需要快速随机访问数据 |
| 字符串 | O(1) | 不支持 | 较低 | 处理文本数据,如搜索、替换 |
| 链表 | O(n) | 可以 | 适中 | 需要频繁插入或删除操作 |
## 2.2 高级数据结构分析
### 2.2.1 栈与队列
栈是一种后进先出(LIFO)的数据结构,它有两个主要操作:`push`(压栈)和`pop`(出栈)。在JavaScript中,可以使用数组来模拟栈的行为。
队列是一种先进先出(FIFO)的数据结构,它支持两个操作:`enqueue`(入队)和`dequeue`(出队)。同样地,队列的行为也可以使用数组来模拟。
```javascript
// 栈的实现
let stack = [];
stack.push(1);
stack.push(2);
console.log(stack.pop()); // 输出:2
// 队列的实现
let queue = [];
queue.push(1);
queue.push(2);
console.log(queue.shift()); // 输出:1
```
### 2.2.2 树与二叉树
树是一种非线性的数据结构,它由节点组成,节点之间通过引用连接。在JavaScript中,树通常通过嵌套对象来实现。
二叉树是一种特殊的树,它的每个节点最多有两个子节点,通常称为左孩子和右孩子。二叉树的遍历可以是前序、中序或后序。
```javascript
class TreeNode {
constructor(value) {
this.value = value;
this.left = null;
this.right = null;
}
}
let root = new TreeNode(1);
root.left = new TreeNode(2);
root.right = new TreeNode(3);
// 前序遍历二叉树
function preorder(node) {
if (node !== null) {
console.log(node.value);
preorder(node.left);
preorder(node.right);
}
}
preorder(root); // 输出:1 2 3
```
## 2.3 散列结构的应用
### 2.3.1 哈希表的概念与实现
哈希表是一种根据关键码的值而直接进行访问的数据结构。它通过哈希函数将关键码映射到表中的位置,以加快查找速
0
0
相关推荐
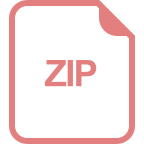
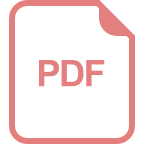
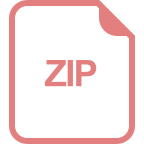
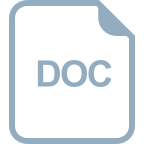
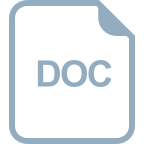
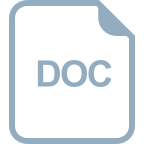
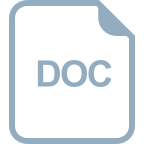
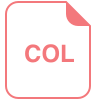
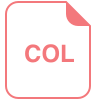