对象唯一性与一致性:线程安全单例模式,并发编程中的守护神
发布时间: 2024-08-26 12:24:07 阅读量: 59 订阅数: 32 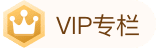
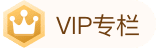
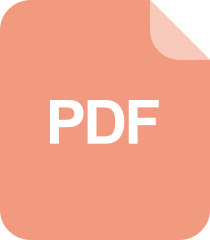
C++线程安全的单例模式:深入解析与实践
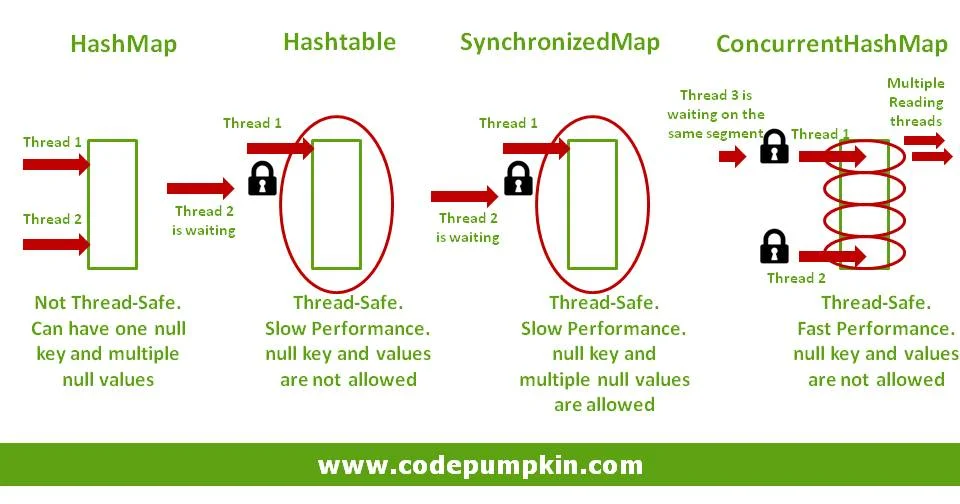
# 1. 线程安全单例模式:概念与理论基础**
单例模式是一种设计模式,它确保一个类只有一个实例,并提供一个全局访问点。它广泛应用于各种场景,例如数据库连接池、日志记录框架和容器管理。
线程安全单例模式保证在多线程环境中只有一个实例,防止并发访问导致数据不一致或程序崩溃。实现线程安全的方法包括同步锁和无锁实现(CAS)。同步锁通过互斥锁机制确保同一时间只有一个线程访问单例实例,而无锁实现使用原子操作(如 CAS)在不使用锁的情况下实现线程安全。
# 2. 单例模式的实现技巧
### 2.1 惰性初始化与延迟加载
惰性初始化是一种延迟加载技术,它推迟对象的创建,直到真正需要时才创建。这可以节省内存和启动时间,尤其是在对象很少被使用的情况下。
#### 2.1.1 双重检查锁定
双重检查锁定是一种常见的惰性初始化技术。它使用两个检查来确保对象只被创建一次:
```java
public class Singleton {
private volatile static Singleton instance;
private Singleton() { }
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
```
**逻辑分析:**
* 第一次检查在同步块外部进行,以避免不必要的同步开销。
* 如果对象不存在,则进入同步块。
* 在同步块内进行第二次检查,以确保对象在进入同步块后没有被创建。
* 如果对象仍然不存在,则创建对象并将其赋值给 `instance`。
**参数说明:**
* `instance`:单例对象的引用。
* `synchronized (Singleton.class)`:使用类的类对象作为锁,确保同步只发生在该类上。
#### 2.1.2 静态内部类
静态内部类是一种惰性初始化的替代方法。它使用一个静态内部类来延迟对象的创建:
```java
public class Singleton {
private static class SingletonHolder {
private static final Singleton instance = new Singleton();
}
private Singleton() { }
public static Singleton getInstance() {
return SingletonHolder.instance;
}
}
```
**逻辑分析:**
* 静态内部类 `SingletonHolder` 在类加载时被创建。
* `instance` 字段在静态内部类中被初始化,确保它只被创建一次。
* `getInstance()` 方法直接返回 `SingletonHolder.instance`,无需任何同步。
**参数说明:**
* `SingletonHolder`:静态内部类,持有单例对象的引用。
* `instance`:单例对象的引用。
# 3.1 数据库连接池
#### 3.1.1 连接池的原理和实现
数据库连接池是一种管理数据库连接的机制,它通过预先创建和维护一个连接池来提高数据库访问的效率。当应用程序需要访问数据库时,它可以从连接池中获取一个可用连接,使用完毕后将其归还给连接池。
连接池的实现通常涉及以下步骤:
1. **初始化连接池:**创建连接池时,需要指定连接池的大小(最大连接数和最小连接数)以及连接池中连接的配置参数(如数据库URL、用户名、密码等)。
2. **创建连接:**当应用程序需要访问数据库时,它会从连接池中获取一个可用连接。如果连接池中没有可用连接,则会根据需要创建新的连接。
3. **使用连接:**应用程序使用连接执行数据库操作。
4. **归还连接:**使用完毕后,应用程序将连接归还给连接池。连接池会将连接标记为可用,以便其他应用程序可以重用它。
####
0
0
相关推荐
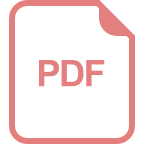
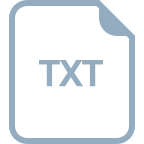
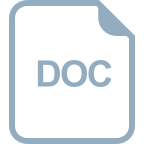
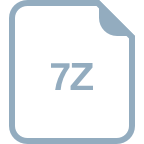
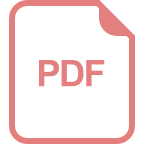
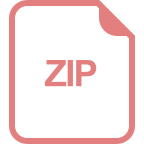
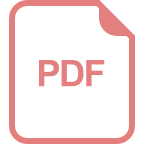
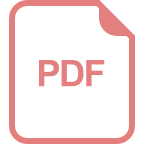