SparkSQL简介与基本概念解析
发布时间: 2023-12-19 08:12:43 阅读量: 13 订阅数: 12 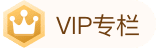
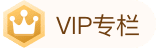
# 第一章:SparkSQL简介
## 1.1 SparkSQL概述
SparkSQL是Apache Spark生态系统中用于处理结构化数据的模块。它提供了使用SQL和DataFrame API进行交互式查询和分析的功能。SparkSQL支持从各种数据源中加载数据,并能够无缝地集成到Spark的其他组件中。
## 1.2 SparkSQL与传统SQL的对比
与传统的SQL相比,SparkSQL具有更好的扩展性和容错性。它可以处理PB级别的数据,并且能够利用Spark的并行计算能力进行高性能的数据处理和分析。
## 1.3 SparkSQL的优势和应用场景
SparkSQL的优势在于能够结合传统的SQL查询和Spark强大的分布式计算能力。它适用于需要处理大规模结构化数据的场景,比如数据仓库查询、ETL过程中的数据转换和清洗等任务。同时,由于支持SQL语法,SparkSQL也更易于被数据分析师和数据工程师所接受和使用。
### 2. 第二章:SparkSQL基本概念
2.1 数据框架与数据集
2.2 结构化数据处理
2.3 数据查询与分析
### 3. 第三章:SparkSQL执行流程
3.1 SparkSQL执行架构
3.2 Catalyst优化器和Tungsten执行引擎
3.3 查询执行过程分析
### 4. 第四章:SparkSQL基本操作
SparkSQL基本操作包括数据加载与保存、SQL查询与DataFrame操作以及数据转换与处理。在本章中,我们将详细介绍SparkSQL的基本操作,并通过代码示例演示其在实际场景中的应用。
#### 4.1 数据加载与保存
在SparkSQL中,我们可以通过不同的数据源加载数据,并将处理结果保存到指定的位置。常见的数据源包括文件系统(如HDFS)、关系型数据库(如MySQL)、NoSQL数据库(如Cassandra)等。下面是一个简单的Python示例,演示了如何加载数据并保存处理结果:
```python
# 导入必要的库
from pyspark.sql import SparkSession
# 创建SparkSession
spark = SparkSession.builder.appName("data_loading_saving").getOrCreate()
# 从CSV文件加载数据
data = spark.read.csv("hdfs://path/to/your/file.csv", header=True, inferSchema=True)
# 查看数据schema
data.printSchema()
# 对数据进行处理,这里假设对数据进行了筛选和聚合操作
processed_data = data.filter(data['age'] > 18).groupby('gender').count()
# 将处理结果保存为Parquet文件
processed_data.write.parquet("hdfs://path/to/save/processed_data.parquet")
```
#### 4.2 SQL查询与DataFrame操作
在SparkSQL中,我们可以使用SQL语句或DataFrame API进行数据查询和操作。SQL查询通常更适合熟悉SQL语法的用户,而DataFrame操作更适合程序化的数据处理流程。下面是一个简单的Java示例,演示了如何使用SQL查询和DataFrame操作对数据进行处理:
```java
// 创建SparkSession
SparkSession spark = SparkSession.builder().appName("sql_dataframe_operations").getOrCreate();
// 从关系型数据库加载数据
Dataset<Row> data = spark.read().jdbc(url, table, properties);
// 注册数据表
data.createOrReplaceTempView("people");
// 使用SQL查询
Dataset<Row> result = spark.sql("SELECT age, COUNT(*) FROM people GROUP BY age");
// 使用DataFrame操作
Dataset<Row> filteredResult = data.filter(data.col("age").gt(18)).groupBy("gender").count();
```
#### 4.3 数据转换与处理
在实际数据分析中,数据的转换与处理是至关重要的环节。SparkSQL提供了丰富的数据转换与处理功能,包括类型转换、空值处理、字符串处理、日期处理等。下面是一个简单的Python示例,演示了如何进行数据转换与处理:
```python
# 导入必要的函数
from pyspark.sql.functions import col, when
# 数据类型转换
processed_data = processed_data.withColumn("age", col("age").cast("int"))
# 处理空值
processed_data = processed_data.na.fill(0)
# 字符串处理
processed_data = processed_data.withColumn("name", when(col("name").isNull(), "Unknown").otherwise(col("name")))
# 日期处理
from pyspark.sql.functions import to_date
processed_data = processed_data.withColumn("date", to_date(col("timestamp"), "yyyy-MM-dd"))
```
### 5. 第五章:SparkSQL数据源与扩展
5.1 外部数据源与文件格式
5.2 自定义函数与UDF
5.3 SparkSQL扩展与整合
### 6. 第六章:SparkSQL性能优化
在本章中,我们将深入讨论SparkSQL的性能优化相关内容,包括查询优化与调优、分区与分桶、以及内存与磁盘存储优化。
#### 6.1 查询优化与调优
在使用SparkSQL进行数据查询时,为了提高性能和效率,我们需要考虑查询的优化和调优策略。这包括但不限于以下几个方面:
- 使用适当的数据分区和索引,以减少查询时的数据扫描范围,提高查询效率。
- 通过合理的数据统计信息收集和查询计划分析,优化查询执行路径,减少不必要的计算和数据传输。
- 合理使用缓存和持久化机制,避免重复计算和数据读取,提高数据处理性能。
以下是一个简单的示例代码,演示了如何使用SparkSQL进行查询优化:
```python
# 示例代码
from pyspark.sql import SparkSession
# 创建SparkSession
spark = SparkSession.builder.appName("query_optimization").getOrCreate()
# 读取数据
df = spark.read.format("csv").option("header", "true").load("data/sales_data.csv")
# 注册临时视图
df.createOrReplaceTempView("sales")
# 执行查询
query = """
SELECT product_category, COUNT(*) AS total_count
FROM sales
WHERE date >= '2022-01-01'
GROUP BY product_category
ORDER BY total_count DESC
"""
spark.sql(query).show()
# 停止SparkSession
spark.stop()
```
通过以上示例,我们可以使用SQL语句对数据进行筛选、聚合和排序,而后SparkSQL会根据查询执行计划对数据进行优化和处理,以提高查询效率。
#### 6.2 分区与分桶
在SparkSQL中,合理的数据分区和分桶设计对于数据处理和查询性能有着重要影响。通过对数据进行合理的分区和分桶,可以减少数据倾斜、加快数据访问速度,并且有利于并行处理和查询优化。
下面我们通过一个示例来展示如何使用SparkSQL进行数据分区和分桶操作:
```python
# 示例代码
from pyspark.sql import SparkSession
# 创建SparkSession
spark = SparkSession.builder.appName("partition_and_bucket").getOrCreate()
# 加载数据
df = spark.read.format("csv").option("header", "true").load("data/user_actions.csv")
# 对数据进行分区与分桶
df.write.format("parquet").partitionBy("date").bucketBy(10, "user_id").saveAsTable("user_actions_partitioned_bucketed")
# 停止SparkSession
spark.stop()
```
通过以上示例,我们可以将数据按照指定的列进行分区和分桶,并且将处理结果保存为表,以便后续的查询和分析。
#### 6.3 内存与磁盘存储优化
在大规模数据处理场景下,合理的内存和磁盘存储优化对于提高数据处理性能至关重要。在SparkSQL中,我们可以通过合理配置内存与磁盘存储策略,并且使用合适的数据格式和压缩算法,来优化数据的存储和读取性能。
以下是一个简单的示例代码,演示了如何在SparkSQL中使用内存与磁盘存储优化:
```python
# 示例代码
from pyspark.sql import SparkSession
# 创建SparkSession
spark = SparkSession.builder.appName("storage_optimization").getOrCreate()
# 读取数据
df = spark.read.format("parquet").load("data/user_actions_partitioned_bucketed")
# 执行查询
query = """
SELECT user_id, action_type, COUNT(*) AS total_count
FROM user_actions_partitioned_bucketed
WHERE date >= '2022-01-01'
GROUP BY user_id, action_type
ORDER BY total_count DESC
"""
spark.sql(query).show()
# 停止SparkSession
spark.stop()
```
通过以上示例,我们可以使用合适的数据格式和存储策略来优化数据的读取性能,并且通过合理的查询执行和分析操作来提高数据处理效率。
在实际的SparkSQL应用中,我们也可以根据具体的业务场景和数据特点,结合查询优化、数据分区与分桶、以及存储优化等手段,来全面提升数据处理和查询性能。
0
0
相关推荐
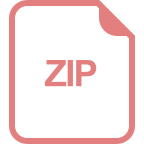





