揭秘Vue项目性能优化秘籍:10个提升用户体验的实战策略
发布时间: 2024-07-21 07:56:26 阅读量: 65 订阅数: 29 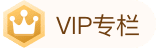
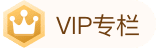
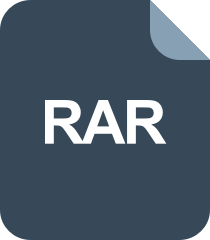
Vue.js性能优化:懒加载实战指南
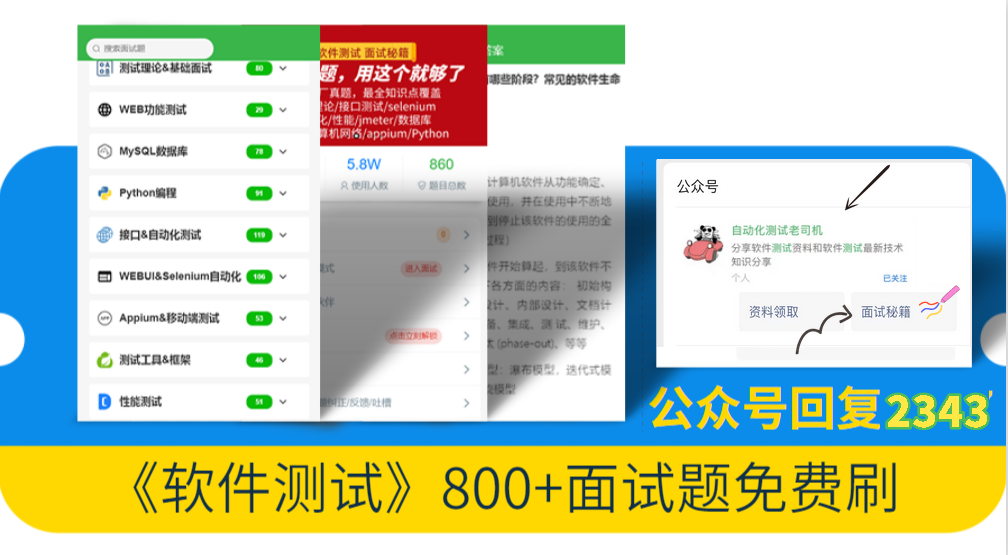
# 1. Vue项目性能优化概述
**1.1 Vue项目性能优化的重要性**
在当今竞争激烈的网络环境中,网站和应用程序的性能至关重要。性能良好的Vue项目可以提高用户满意度、减少跳出率并提高转化率。
**1.2 Vue性能优化的目标**
Vue性能优化旨在通过以下方式提升应用程序的性能:
* 减少不必要的渲染
* 优化数据操作
* 优化网络请求
* 优化组件性能
* 优化路由性能
# 2. Vue项目性能优化理论基础
### 2.1 Vue响应式系统原理
Vue.js的核心是其响应式系统,它允许应用程序在数据发生变化时自动更新UI。响应式系统通过使用代理对象来跟踪数据对象中的属性,当属性值发生变化时,代理对象会触发更新过程。
**代理对象**
Vue.js使用`Object.defineProperty()`方法创建代理对象。代理对象具有与原始数据对象相同的属性,但当属性值发生变化时,代理对象会触发更新过程。
**更新过程**
当代理对象中的属性值发生变化时,Vue.js会触发更新过程。更新过程包括以下步骤:
1. **更新视图:**Vue.js会更新使用该属性的视图。
2. **触发生命周期钩子:**Vue.js会触发`updated`生命周期钩子。
3. **通知组件:**Vue.js会通知组件,以便组件可以更新其状态。
### 2.2 虚拟DOM与真实DOM差异
Vue.js使用虚拟DOM来优化渲染性能。虚拟DOM是一个轻量级的DOM表示,它存储在内存中。当数据发生变化时,Vue.js会比较虚拟DOM和真实DOM,并仅更新发生变化的部分。
**虚拟DOM的优点**
使用虚拟DOM有以下优点:
- **更快的渲染:**虚拟DOM比真实DOM轻量级,因此渲染速度更快。
- **更少的内存使用:**虚拟DOM仅存储DOM的必要部分,因此使用更少的内存。
- **更稳定的应用程序:**虚拟DOM有助于防止应用程序崩溃,因为只有发生变化的部分才会被更新。
### 2.3 性能瓶颈分析与定位
识别和定位性能瓶颈对于优化Vue.js应用程序至关重要。以下是一些常见的性能瓶颈:
- **不必要的渲染:**频繁的渲染会导致性能问题。
- **数据操作不当:**不当的数据操作,例如频繁的数组操作,会导致性能下降。
- **网络请求:**网络请求是性能瓶颈的常见原因。
- **组件性能:**组件性能不佳会导致应用程序变慢。
- **路由性能:**路由性能不佳会导致页面加载缓慢。
可以使用以下工具分析和定位性能瓶颈:
- **Chrome DevTools:**Chrome DevTools提供了一系列工具,用于分析和定位性能问题。
- **Vue Devtools:**Vue Devtools是一个浏览器扩展,提供专门用于Vue.js应用程序的性能分析工具。
# 3.1 减少不必要的渲染
在 Vue 应用中,渲染是性能瓶颈的主要来源之一。不必要的渲染会导致性能下降,因为它需要消耗额外的计算资源和时间。因此,减少不必要的渲染对于提高 Vue 应用的性能至关重要。
#### 3.1.1 使用 v-if 和 v-show
`v-if` 和 `v-show` 是 Vue 提供的两个指令,用于控制元素的渲染。`v-if` 根据表达式的真假值来决定是否渲染元素,而 `v-show` 根据表达式的真假值来控制元素的显示和隐藏。
```vue
<template>
<div>
<p v-if="show">显示</p>
<p v-show="show">隐藏</p>
</div>
</template>
<script>
export default {
data() {
return {
show: true
}
}
}
</script>
```
在上面的示例中,当 `show` 数据为 `true` 时,`v-if` 和 `v-show` 都会渲染 `<p>` 元素。但是,当 `show` 数据为 `false` 时,`v-if` 会移除 `<p>` 元素,而 `v-show` 只是隐藏 `<p>` 元素。
使用 `v-if` 和 `v-show` 的主要区别在于:
* **v-if:**当表达式为 `false` 时,它会移除元素及其所有子元素。这对于删除不需要的元素很有用,因为它可以释放内存并减少渲染时间。
* **v-show:**当表达式为 `false` 时,它只是隐藏元素,但不会移除它。这对于隐藏元素但仍希望保留其状态很有用,例如表单输入或模态窗口。
#### 3.1.2 使用 keep-alive
`keep-alive` 是 Vue 提供的一个组件,用于缓存组件状态。当一个组件被包裹在 `keep-alive` 中时,它的状态将在组件切换时被保留,即使组件被销毁和重新创建。
```vue
<template>
<keep-alive>
<my-component></my-component>
</keep-alive>
</template>
<script>
export default {
components: {
MyComponent
}
}
</script>
```
在上面的示例中,`MyComponent` 组件被包裹在 `keep-alive` 中。当 `MyComponent` 被销毁和重新创建时,它的状态将被保留,这可以减少组件重新渲染的开销。
使用 `keep-alive` 的主要优点是:
* **减少渲染时间:**通过缓存组件状态,`keep-alive` 可以减少组件重新渲染的时间,从而提高性能。
* **保持组件状态:**`keep-alive` 可以保持组件状态,即使组件被销毁和重新创建。这对于需要保留用户输入或其他状态的组件很有用。
但是,使用 `keep-alive` 也有一些缺点:
* **增加内存使用:**`keep-alive` 会缓存组件状态,这可能会增加内存使用。
* **潜在的性能问题:**如果缓存的组件状态很大,则可能会导致性能问题。
# 4. Vue项目性能优化进阶技巧
### 4.1 使用性能分析工具
#### 4.1.1 Chrome DevTools
Chrome DevTools是Chrome浏览器内置的一款强大的性能分析工具,它提供了丰富的功能,帮助开发人员分析和优化Web应用程序的性能。
**功能:**
- **Performance面板:**记录和分析应用程序的性能指标,如加载时间、渲染时间、内存使用情况等。
- **Network面板:**分析网络请求,包括请求类型、响应时间、传输大小等。
- **Memory面板:**监控应用程序的内存使用情况,识别内存泄漏和优化内存管理。
**使用指南:**
1. 打开Chrome DevTools(按F12或右键单击并选择“检查”)。
2. 转到“Performance”面板。
3. 单击“Record”按钮开始记录应用程序的性能。
4. 执行应用程序中需要分析的操作。
5. 单击“Stop”按钮停止记录。
6. 分析记录的数据,找出性能瓶颈。
#### 4.1.2 Vue Devtools
Vue Devtools是专门为Vue.js应用程序设计的性能分析工具,它提供了针对Vue.js特定功能的优化建议。
**功能:**
- **组件树:**可视化组件树,显示组件的层级关系和数据流。
- **性能面板:**分析组件的渲染时间、内存使用情况和网络请求。
- **事件时间线:**记录和分析应用程序中的事件,包括组件生命周期钩子、事件处理程序和异步操作。
**使用指南:**
1. 安装Vue Devtools扩展程序(https://chrome.google.com/webstore/detail/vuejs-devtools/nhdogjmejiglipccpnnnanhbledajbpd)。
2. 打开Vue Devtools(按Ctrl+Shift+I或右键单击并选择“检查”)。
3. 转到“Vue”选项卡。
4. 分析组件树和性能数据,找出性能瓶颈。
### 4.2 优化组件性能
#### 4.2.1 使用scoped样式
Scoped样式可以将CSS样式限制在特定的组件内,防止样式污染和提高性能。
**示例:**
```html
<template>
<div>
<p>{{ message }}</p>
</div>
</template>
<style scoped>
p {
color: red;
}
</style>
```
**优点:**
- 提高性能,因为浏览器不需要解析和应用不必要的样式。
- 提高代码可维护性,因为样式与组件紧密耦合。
#### 4.2.2 使用函数式组件
函数式组件是仅包含render函数的组件,它们没有状态和生命周期钩子。函数式组件比类组件更轻量级,可以提高性能。
**示例:**
```javascript
const MyComponent = {
render(h) {
return h('p', { attrs: { id: 'my-component' } }, 'Hello World!');
}
};
```
**优点:**
- 提高性能,因为函数式组件没有状态和生命周期钩子。
- 提高代码可重用性,因为函数式组件可以轻松地组合和重用。
### 4.3 优化路由性能
#### 4.3.1 使用懒加载
懒加载可以延迟加载非关键组件,直到需要时才加载,从而减少初始加载时间。
**示例:**
```javascript
const router = new VueRouter({
routes: [
{
path: '/my-component',
component: () => import('./MyComponent.vue')
}
]
});
```
**优点:**
- 减少初始加载时间,因为非关键组件不会在加载时加载。
- 提高性能,因为只有在需要时才加载组件。
#### 4.3.2 使用路由缓存
路由缓存可以将已加载的组件缓存起来,以避免在再次访问时重新加载,从而提高性能。
**示例:**
```javascript
const router = new VueRouter({
routes: [
{
path: '/my-component',
component: MyComponent,
meta: {
keepAlive: true
}
}
]
});
```
**优点:**
- 提高性能,因为已加载的组件不会在再次访问时重新加载。
- 提高用户体验,因为页面切换更加流畅。
# 5. Vue项目性能优化最佳实践
### 5.1 遵循性能优化原则
在进行Vue项目性能优化时,遵循以下原则至关重要:
- **渐进式优化:**不要一次性尝试实现所有优化,而应逐步进行,并根据实际效果进行调整。
- **衡量优化效果:**使用性能分析工具或其他指标来衡量优化措施的效果,并根据结果进行调整。
- **避免过度优化:**优化应针对实际性能瓶颈,避免过度优化导致代码复杂度增加或维护成本提高。
- **遵循社区最佳实践:**参考Vue官方文档、社区博客和论坛,了解最新的最佳实践和优化技巧。
### 5.2 持续监控和改进
性能优化是一个持续的过程,需要持续监控和改进:
- **定期性能测试:**定期使用性能分析工具对应用程序进行测试,识别潜在的性能问题。
- **收集用户反馈:**收集用户反馈,了解应用程序在实际使用中的性能表现。
- **持续改进:**根据测试结果和用户反馈,不断改进优化措施,提升应用程序性能。
### 5.3 社区资源和工具推荐
Vue社区提供了丰富的资源和工具,助力性能优化:
- **Vue Devtools:**Chrome扩展,提供对Vue应用程序的深入洞察,包括性能分析。
- **Performance API:**Vue提供了一系列API,用于衡量和改进应用程序性能,例如`Vue.config.performance`和`Vue.perf.start()`。
- **社区论坛和博客:**Vue社区论坛和博客经常分享性能优化技巧和最佳实践。
- **第三方库:**有许多第三方库可以帮助优化Vue应用程序,例如`vuex-persist`(用于持久化Vuex状态)和`vue-router-lazy-load`(用于路由懒加载)。
0
0
相关推荐
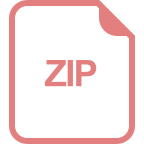
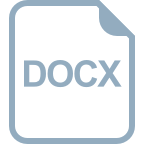
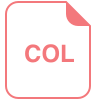
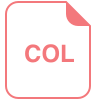
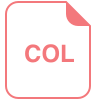
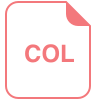
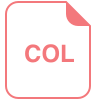
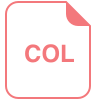