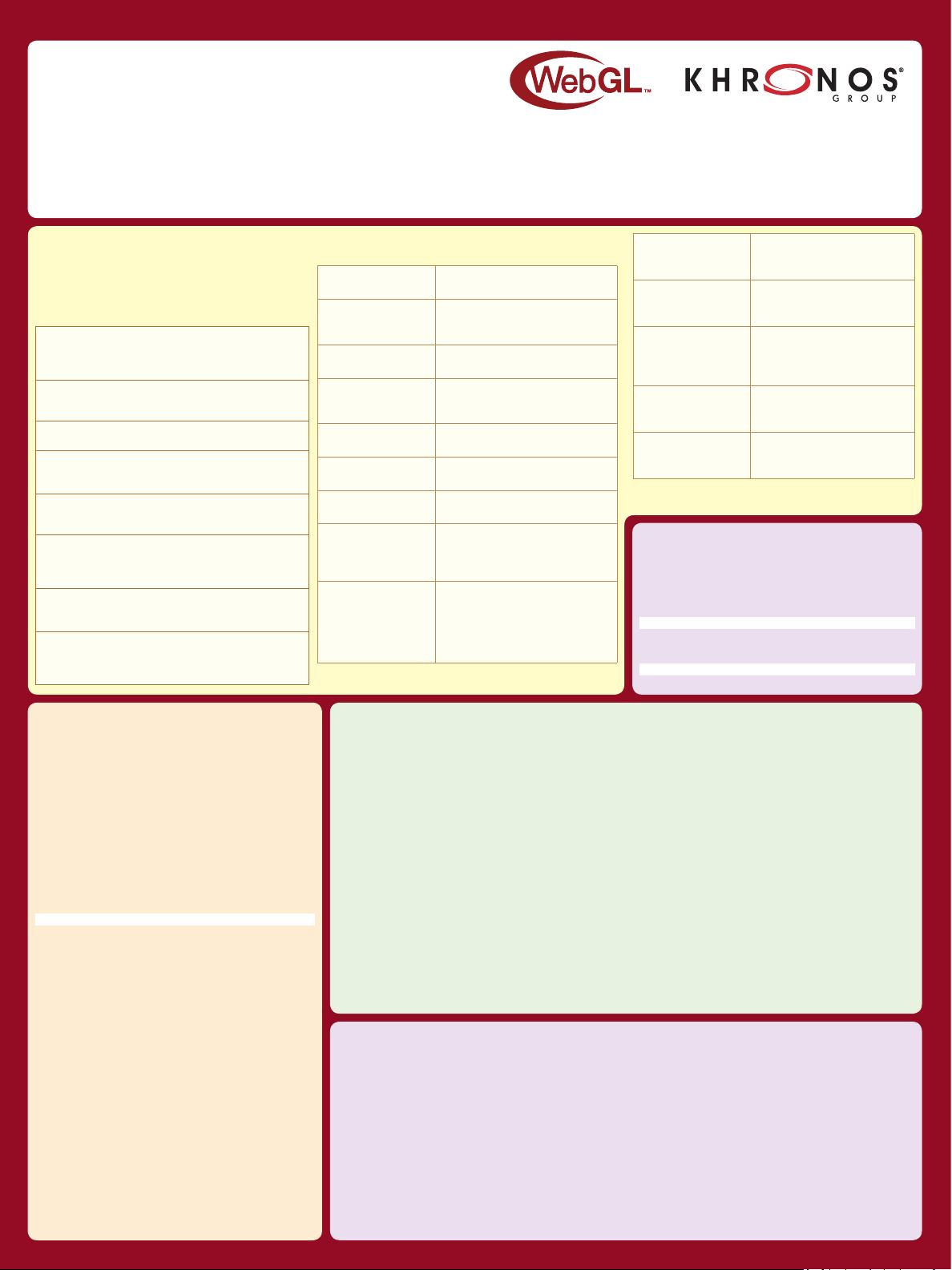
www.khronos.org/webgl©2019 The Khronos® Group Inc - Rev. 0619
WebGL 2.0 API Quick Reference Guide Page 1
ArrayBuer and Typed Arrays
[5.13]
Data is transferred to WebGL using ArrayBuer and views.
Buers represent unstructured binary data, which can be
modied using one or more typed array views. Consult the
ECMAScript specicaon for more details on Typed Arrays.
Buers
ArrayBuer(ulong byteLength);
byteLength: read-only, length of view in bytes.
Creates a new buer. To modify the data, create one or more
views referencing it.
Views
In the following, ViewType may be Int8Array, Int16Array,
Int32Array, Uint8Array, Uint16Array, Uint32Array, Float32Array.
ViewType(ulong length);
Creates a view and a new underlying buer.
length: Read-only, number of elements in this view.
ViewType(ViewType other);
Creates new underlying buer and copies other array.
ViewType(type[] other);
Creates new underlying buer and copies other array.
ViewType(ArrayBuer buer, [oponal] ulong byteOset,
[oponal] ulong length);
Create a new view of given buer, starng at oponal byte
oset, extending for oponal length elements.
buer: Read-only, buer backing this view
byteOset: Read-only, byte oset of view start in buer
length: Read-only, number of elements in this view
Other Properes
byteLength: Read-only, length of view in bytes.
const ulong BYTES_PER_ELEMENT: element size in bytes.
Methods
view[i] = get/set element i
s
et(ViewType
other
[, ulong
oset
]);
se
t(type[] other
[,
ulong oset
]
);
Replace elements in this view with those from other, starng
at oponal oset.
ViewType subArray(long begin
[,
long end
]
);
Return a subset of this view, referencing the same underlying
buer.
WebGLQuery [3.2]
Created by createQuery, made acve by
beginQuery, concluded by endQuery,
destroyed by deleteQuery
WebGLSampler [3.3]
Created by createSampler, bound
by bindSampler, destroyed by
deleteSampler
WebGLSync [3.4]
Created by fenceSync, blocked on by
clientWaitSync, waited on internal GL
by waitSync, queried by getSynciv,
destroyed by deleteSync
WebGLTransformFeedback
[3.5]
Created by createTransformFeedback,
bound by bindTransformFeedback,
destroyed by deleteTransformFeedback
WebGLVertexArrayObject
[3.6]
Created by createVertexArray,
bound by bindVertexArray,
destroyed by deleteVertexArray
WebGL
TM
is an immediate-mode 3D rendering API from The Khronos® Group
designed for the web. It is derived from OpenGL® ES 3.0, and provides similar
rendering funconality, but in an HTML context. WebGL 2 is not enrely
backwards compable with WebGL 1. Exisng error-free content wrien against
the core WebGL 1 specicaon without extensions will oen run in WebGL 2
without modicaon, but this is not always the case.
The WebGL 2 specicaon shows dierences from the WebGL 1 specicaon.
Both WebGL specicaons are available at khronos.org/webgl. Unless otherwise
specied, the behavior of each method is dened by the OpenGL ES 3.0
specicaon. The OpenGL ES specicaon is at khr.io/glesregistry.
• [n.n.n] refers to secons in the WebGL 1.0 specicaon.
• [n.n.n] refers to secons in the WebGL 2.0 specicaon.
• Content in blue is newly added with WebGL 2.0.
• Content in purple or marked with
•
has no corresponding OpenGL ES 3.0 funcon.
Per-Fragment Operaons [5.14.3]
void blendColor(clampf red, clampf green, clampf blue,
clampf alpha);
void blendEquaon(enum mode);
mode: See modeRGB for blendEquaonSeparate
void blendEquaonSeparate(enum modeRGB,
enum modeAlpha);
modeRGB, and modeAlpha: FUNC_ADD, FUNC_SUBTRACT,
FUNC_REVERSE_SUBTRACT
void blendFunc(enum sfactor, enum dfactor);
sfactor: Same as for dfactor, plus SRC_ALPHA_SATURATE
dfactor: ZERO, ONE, [ONE_MINUS_]SRC_COLOR,
[ONE_MINUS_]DST_COLOR, [ONE_MINUS_]SRC_ALPHA,
[ONE_MINUS_]DST_ALPHA, [ONE_MINUS_]CONSTANT_COLOR,
[ONE_MINUS_]CONSTANT_ALPHA
sfactor and dfactor may not both reference constant color
void blendFuncSeparate(enum srcRGB, enum dstRGB,
enum srcAlpha, enum dstAlpha);
srcRGB, srcAlpha: See sfactor for blendFunc
dstRGB, dstAlpha: See dfactor for blendFunc
void depthFunc(enum func);
func: NEVER, ALWAYS, LESS, [NOT]EQUAL, {GE, LE}QUAL, GREATER
void sampleCoverage(oat value, bool invert);
void stencilFunc(enum func, int ref, uint mask);
func: NEVER, ALWAYS, LESS, LEQUAL, [NOT]EQUAL, GREATER,
GEQUAL
void stencilFuncSeparate(enum face, enum func, int ref,
uint mask);
face: FRONT, BACK, FRONT_AND_BACK
func: NEVER, ALWAYS, LESS, LEQUAL, [NOT]EQUAL, GREATER,
GEQUAL
void stencilOp(enum fail, enum zfail, enum zpass);
fail, zfail, and zpass: KEEP, ZERO, REPLACE, INCR, DECR, INVERT,
INCR_WRAP, DECR_WRAP
void stencilOpSeparate(enum face, enum fail, enum zfail,
enum zpass);
face: FRONT, BACK, FRONT_AND_BACK
fail, zfail, and zpass: See fail, zfail, and zpass for stencilOp
WebGL Context Creaon [2.1]
To use WebGL, the author must obtain a WebGL rendering
context for a given HTMLCanvasElement. This context
manages the OpenGL state and renders to the drawing buer.
[canvas].getContext(
"webgl", WebGLContextAributes? oponalAribs)
Returns a WebGL 1.0 rendering context
[canvas].getContext(
"webgl2", WebGLContextAributes? oponalAribs)
Returns a WebGL 2.0 rendering context
Interfaces
WebGLContextAributes
[5.2]
This interface contains requested drawing surface aributes
and is passed as the second parameter to getContext. Some
of these are oponal requests and may be ignored by an
implementaon.
alpha Default: true
If true, requests a drawing buer with an alpha channel for the
purposes of performing OpenGL desnaon alpha operaons
and composing with the page.
depth Default: true
If true, requests drawing buer with a depth buer of at least
16 bits. Must obey.
stencil Default: false
If true, requests a stencil buer of at least 8 bits. Must obey.
analias Default: true
If true, requests drawing buer with analiasing using its choice
of technique (mulsample/supersample) and quality. Must obey.
premulpliedAlpha Default: true
If true, requests drawing buer which contains colors with
premulplied alpha. (Ignored if alpha is false.)
preserveDrawingBuer Default: false
If true, requests that contents of the drawing buer remain
in between frames, at potenal performance cost. May have
signicant performance implicaons on some hardware.
preferLowPowerToHighPerformance Default: false
Provides a hint suggesng that implementaon create a context
that opmizes for power consumpon over performance.
failIfMajorPerformanceCaveat Default: false
If true, context creaon will fail if the performance of the
created WebGL context would be dramacally lower than that
of a nave applicaon making equivalent OpenGL calls.
Buer Objects
[5.14.5] [3.7.3]
Once bound, buers may not be rebound with a dierent target.
void bindBuer(enum target, WebGLBuer? buer);
target: ARRAY_BUFFER, ELEMENT_ARRAY_BUFFER,
PIXEL_[UN]PACK_BUFFER, COPY_{READ, WRITE}_BUFFER,
TRANSFORM_FEEDBACK_BUFFER, UNIFORM_BUFFER
typedef (ArrayBuer or ArrayBuerView) BuerDataSource
void buerData(enum target, long size, enum usage);
target: See target for bindBuer
usage: STREAM_{DRAW, READ, COPY}, STATIC_{DRAW, READ, COPY},
DYNAMIC_{DRAW, READ, COPY}
void buerData(enum target, ArrayBuerView srcData,
enum usage, uint srcOset[, uint length=0]);
target and usage: Same as for buerData above
void buerData(enum target, BuerDataSource data,
enum usage);
target and usage: Same as for buerData above
void buerSubData(enum target, long oset,
BuerDataSource data);
target: See target for bindBuer
void buerSubData(enum target, intptr dstByteOset,
ArrayBuerView srcData, uint srcOset[, uint length=0]);
target: See target for bindBuer
void copyBuerSubData(enum readTarget, enum writeTarget,
intptr readOset, intptr writeOset, sizeiptr size);
•
void getBuerSubData(enum target, intptr srcByteOset,
ArrayBuerView dstBuer[, uint dstOset=0[,
uint length=0]]);
WebGLObject [5.3]
This is the parent interface for all WebGL resource objects:
WebGLBuer [5.4]
Created by createBuer, bound by bindBuer,
destroyed by deleteBuer
WebGLFramebuer [5.5]
Created by createFramebuer, bound
by bindFramebuer, destroyed by
deleteFramebuer
WebGLProgram [5.6]
Created by createProgram, used by
useProgram, destroyed by deleteProgram
WebGLRenderbuer [5.7]
Created by createRenderbuer, bound
by bindRenderbuer, destroyed by
deleteRenderbuer
WebGLShader [5.8]
Created by createShader, aached to program
by aachShader, destroyed by deleteShader
WebGLTexture [5.9]
Created by createTexture, bound by
bindTexture, destroyed by deleteTexture
WebGLUniformLocaon
[5.10]
Locaon of a uniform variable in a shader
program.
WebGLAcveInfo [5.11] Informaon returned from calls to
getAcveArib and getAcveUniform. The
read-only aributes are:
int size enum type DOMstring name
WebGLShaderPrecision-
Format [5.12]
Informaon returned from calls to
getShaderPrecisionFormat.
The read-only aributes are:
int rangeMin
int rangeMax
int precision