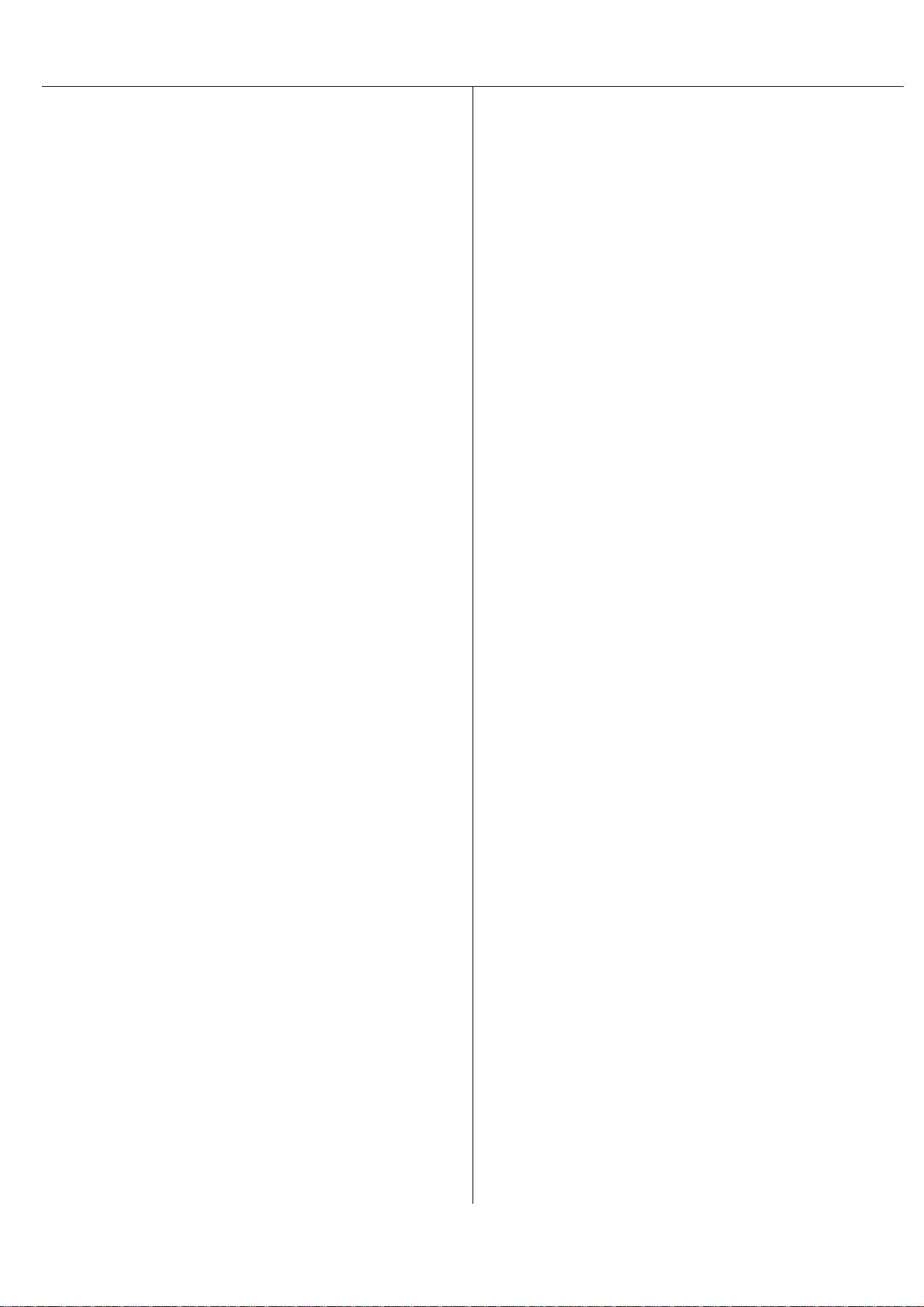
吉林大学 ACM Group
8
for (i = n - 1; i >= 0; i--) {
for (j = 0, k = -1; j < n; j++) if (0 == vis[j]) {
if (k == -1 || deg[j] > deg[k]) k = j;
}
vis[k] = 1, tag[i] = k;
for (j = 0; j<n; j++)
if (0 == vis[j] && g[k][j]) deg[j]++;
}
}
/*==================================================*\
| 稳定婚姻问题 O(n^2)
\*==================================================*/
const int N = 1001;
struct People{
bool state;
int opp, tag;
int list[N]; // man使用
int priority[N]; // woman使用, 有必要的话可以和list合并,
以节省空间
void Init(){ state = tag = 0; }
}man[N], woman[N];
struct R{
int opp; int own;
}requst[N];
int n;
void Input(void);
void Output(void);
void stableMatching(void);
int main(void){
//...
Input();
stableMatching();
Output();
//...
return 0;
}
void Input(void){
scanf("%d\n", &n);
int i, j, ch;
for( i=0; i < n; ++i ) {
man[i].Init();
for( j=0; j < n; ++j ){ //按照man的意愿递减排序
scanf("%d", &ch); man[i].list[j] = ch-1;
}
}
for( i=0; i < n; ++i ) {
woman[i].Init();
for( j=0; j < n; ++j ){ //按照woman的意愿递减排序,
但是,存储方法与man不同!!!!
scanf("%d", &ch); woman[i].priority[ch-1] = j;
}
}
}
void stableMatching(void){
int k;
for( k=0; k < n; +k ){
int i, id = 0;
for( i=0; i < n; ++i )
if( man[i].state == 0 ){
requst[id].opp =
man[i].list[ man[i].tag ];
requst[id].own = i;
man[i].tag += 1; ++id;
}
if( id == 0 ) break;
for( i=0; i < id; ++i ){
if( woman[requst[i].opp].state == 0 ){
woman[requst[i].opp].opp =
requst[i].own;
woman[requst[i].opp].state = 1;
man[requst[i].own].state = 1;
man[requst[i].own].opp =
requst[i].opp;
}
else{
if( woman[requst[i].opp].priority[ woman[requst[i].o
pp].opp ] >
woman[requst[i].opp].priority[requst[i].own] ){ //
man[ woman[requst[i].opp].opp ].state = 0;
woman[ requst[i].opp ].opp =
requst[i].own;
man[requst[i].own].state = 1;
man[requst[i].own].opp =
requst[i].opp;
}
}
}
}
}
void Output(void){
for( int i=0; i < n; ++i ) printf("%d\n", man[i].opp+1);
}
/*==================================================*\
| 拓扑排序
| INIT:edge[][]置为图的邻接矩阵;count[0…i…n-1]:顶点i的入度.
\*==================================================*/
void TopoOrder(int n){
int i, top = -1;
for( i=0; i < n; ++i )
if( count[i] == 0 ){ // 下标模拟堆栈
count[i] = top; top = i;
}
for( i=0; i < n; ++i )
if( top == -1 ) { printf("存在回路\n"); return ; }
else{
int j = top; top = count[top];
printf("%d", j);
for( int k=0; k < n; ++k )
if( edge[j][k] && (--count[k]) == 0 ){
count[k] = top; top = k;
}
}
}
/*==================================================*\
| 无向图连通分支(dfs/bfs 邻接阵)
| DFS / BFS / 并查集
\*==================================================*/
/*==================================================*\
| 有向图强连通分支(dfs/bfs 邻接阵)O(n^2)
\*==================================================*/
//返回分支数,id返回1..分支数的值
//传入图的大小n和邻接阵mat,不相邻点边权0
#define MAXN 100
void search(int n,int mat[][MAXN],int* dfn,int* low,int
now,int& cnt,int& tag,int* id,int* st,int& sp){
int i,j;
dfn[st[sp++]=now]=low[now]=++cnt;
for (i=0;i<n;i++)
if (mat[now][i]){
if (!dfn[i]){
search(n,mat,dfn,low,i,cnt,tag,id,st,sp);
if (low[i]<low[now])
low[now]=low[i];
}
else if (dfn[i]<dfn[now]){
for (j=0;j<sp&&st[j]!=i;j++);
if (j<cnt&&dfn[i]<low[now])
low[now]=dfn[i];
}
}
if (low[now]==dfn[now])
for (tag++;st[sp]!=now;id[st[--sp]]=tag);
}
int find_components(int n,int mat[][MAXN],int* id){
int ret=0,i,cnt,sp,st[MAXN],dfn[MAXN],low[MAXN];
for (i=0;i<n;dfn[i++]=0);
for (sp=cnt=i=0;i<n;i++)
if (!dfn[i])
search(n,mat,dfn,low,i,cnt,ret,id,st,sp);
return ret;
}
//有向图强连通分支,bfs邻接阵形式,O(n^2)
//返回分支数,id返回1..分支数的值
//传入图的大小n和邻接阵mat,不相邻点边权0
#define MAXN 100
int find_components(int n,int mat[][MAXN],int* id){
int ret=0,a[MAXN],b[MAXN],c[MAXN],d[MAXN],i,j,k,t;