《Linux编程实例:基础篇》是一本深入浅出的Linux编程指南,旨在帮助读者掌握在Linux/Unix环境下进行编程的关键概念和技术。本书特别关注于GNU程序,强调其标准遵循、功能强大以及跨平台性,并通过实际示例讲解编程技巧。 该书的版权信息和前言部分介绍了目标读者群,包括希望学习或提升Linux编程技能的专业人士和学生。作者承诺将通过实例引导读者理解Unix/Linux程序模型、C语言的基础及其与GNU工具的关系,以及为什么选择GNU程序的重要性。书中还强调了程序的可移植性,并推荐了一些后续阅读材料,以便进一步深化学习。 第一部分涵盖了核心概念,如第一章“简介”,介绍Linux/Unix的文件和进程模型,对比原始C语言与标准C,解释GNU程序的优势,并讨论了可移植性问题。这一章还提供了一些学习路径的建议,并总结了章节内容,同时附有实践性的练习题供读者巩固所学。 第二章“选项、参数和环境”详细解析命令行处理、选项解析函数(如`getopt()`和`getopt_long()`)的应用,以及如何利用环境变量。这有助于读者理解如何编写能够处理用户输入和配置的程序。 第三章“用户级别内存管理”探讨了Linux/Unix的地址空间概念,内存分配机制,使读者了解程序如何管理和请求内存资源。这一章同样配有实践性练习,帮助读者加深理解。 第四章“文件和文件I/O”则深入讲解Linux/Unix的I/O模型,如何处理基本的文件操作,这对于任何涉及文件操作的程序设计都是至关重要的。本章展示了如何在实际项目中应用这些概念。 《Linux Programming by Example - The Fundamentals》是一本实用且系统的教程,通过实例展示了如何运用标准库函数和工具,构建高效、可扩展的Linux程序,无论是初学者还是经验丰富的程序员都能从中受益匪浅。无论是对C语言的掌握、系统编程的理解,还是对Linux/Unix环境的熟悉,本书都是一个宝贵的学习资源。
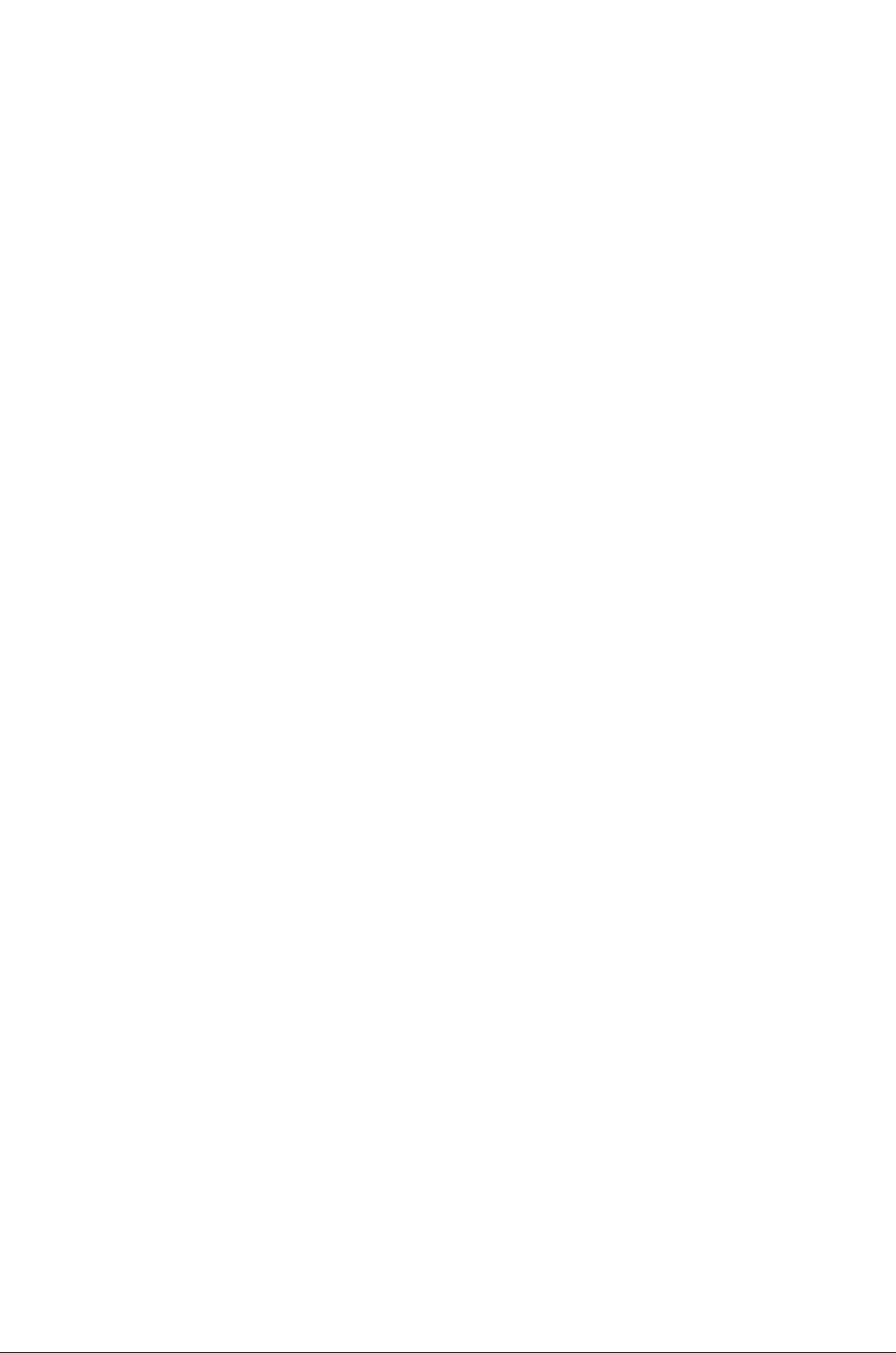
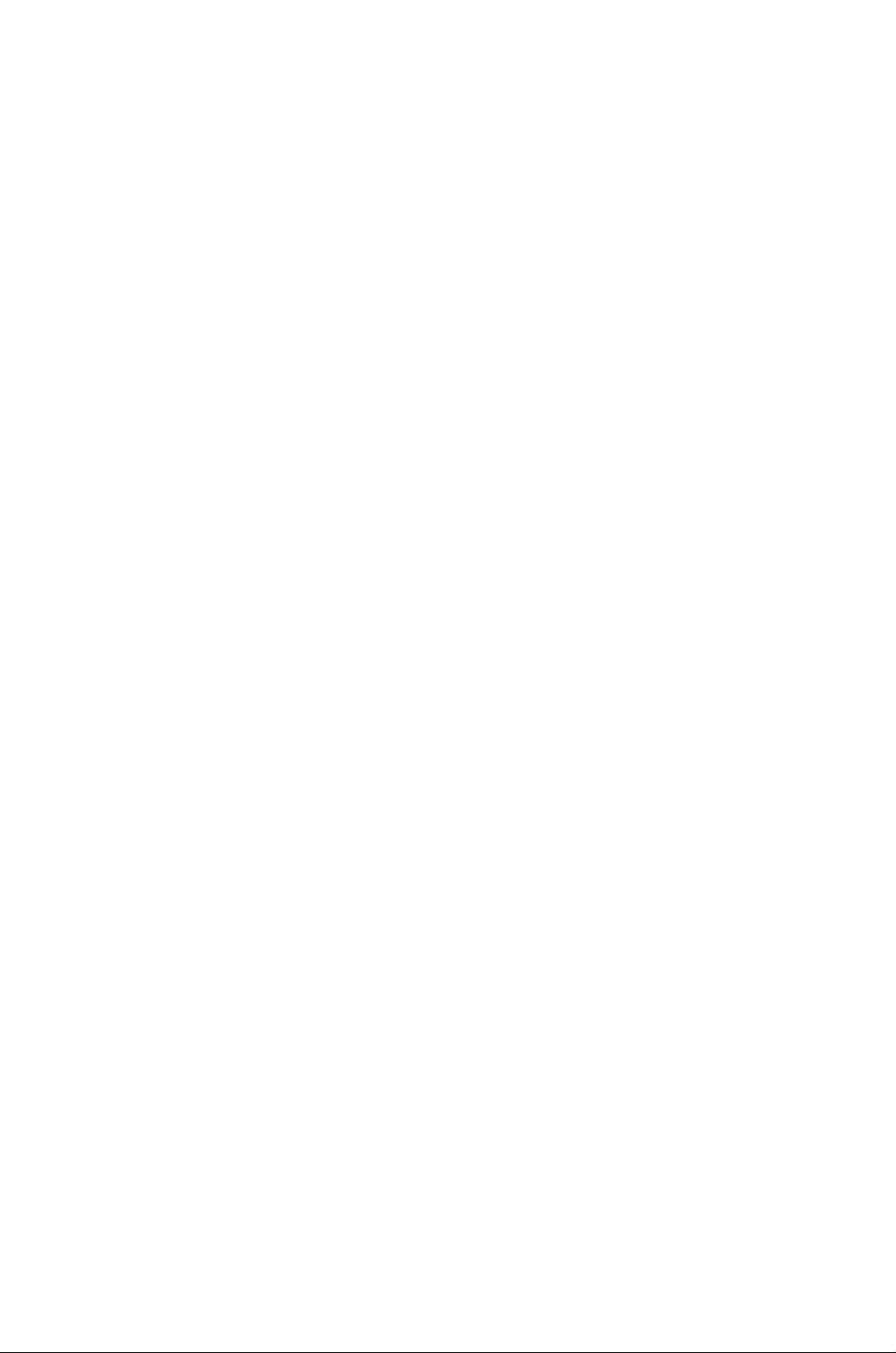
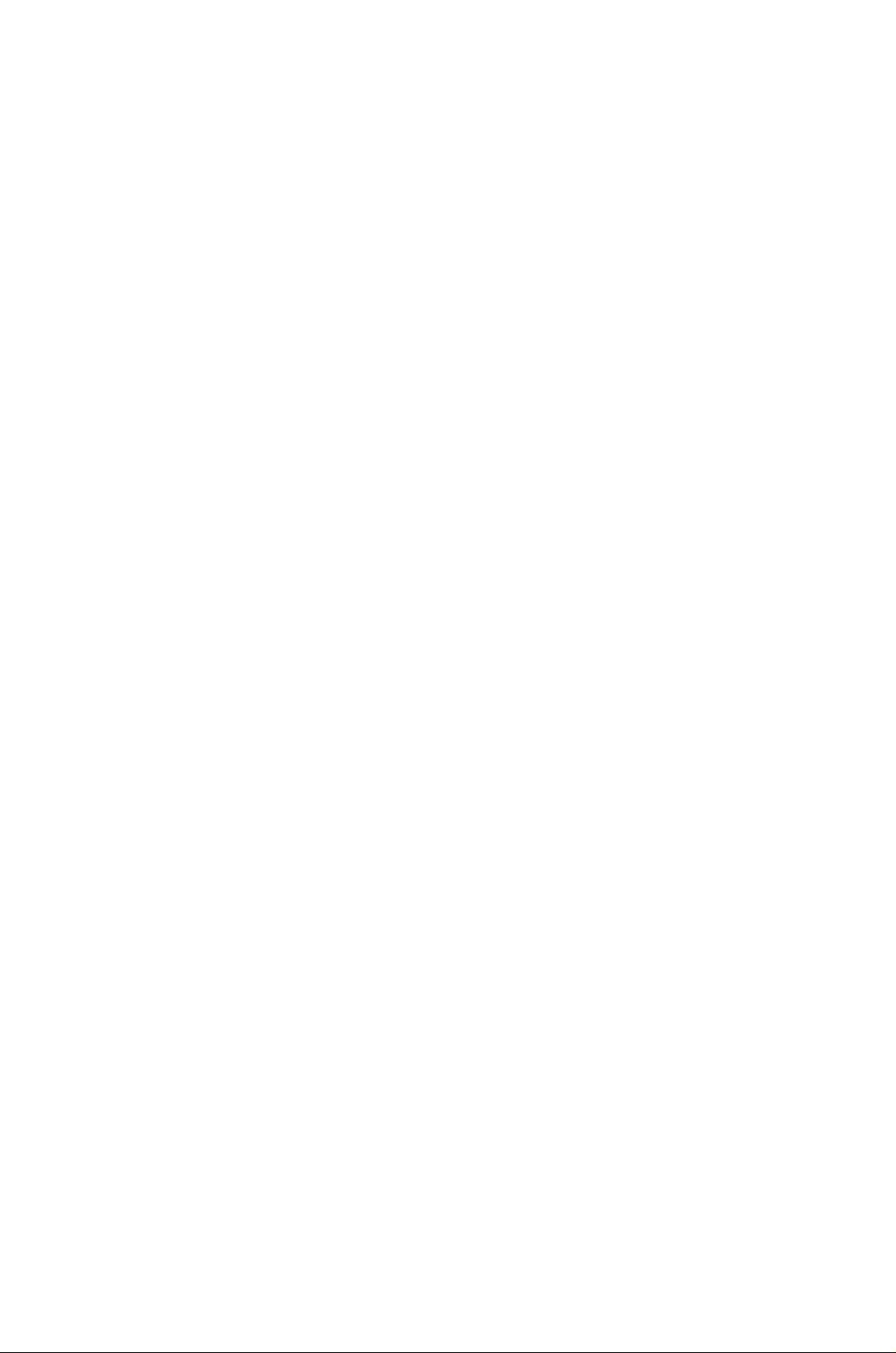
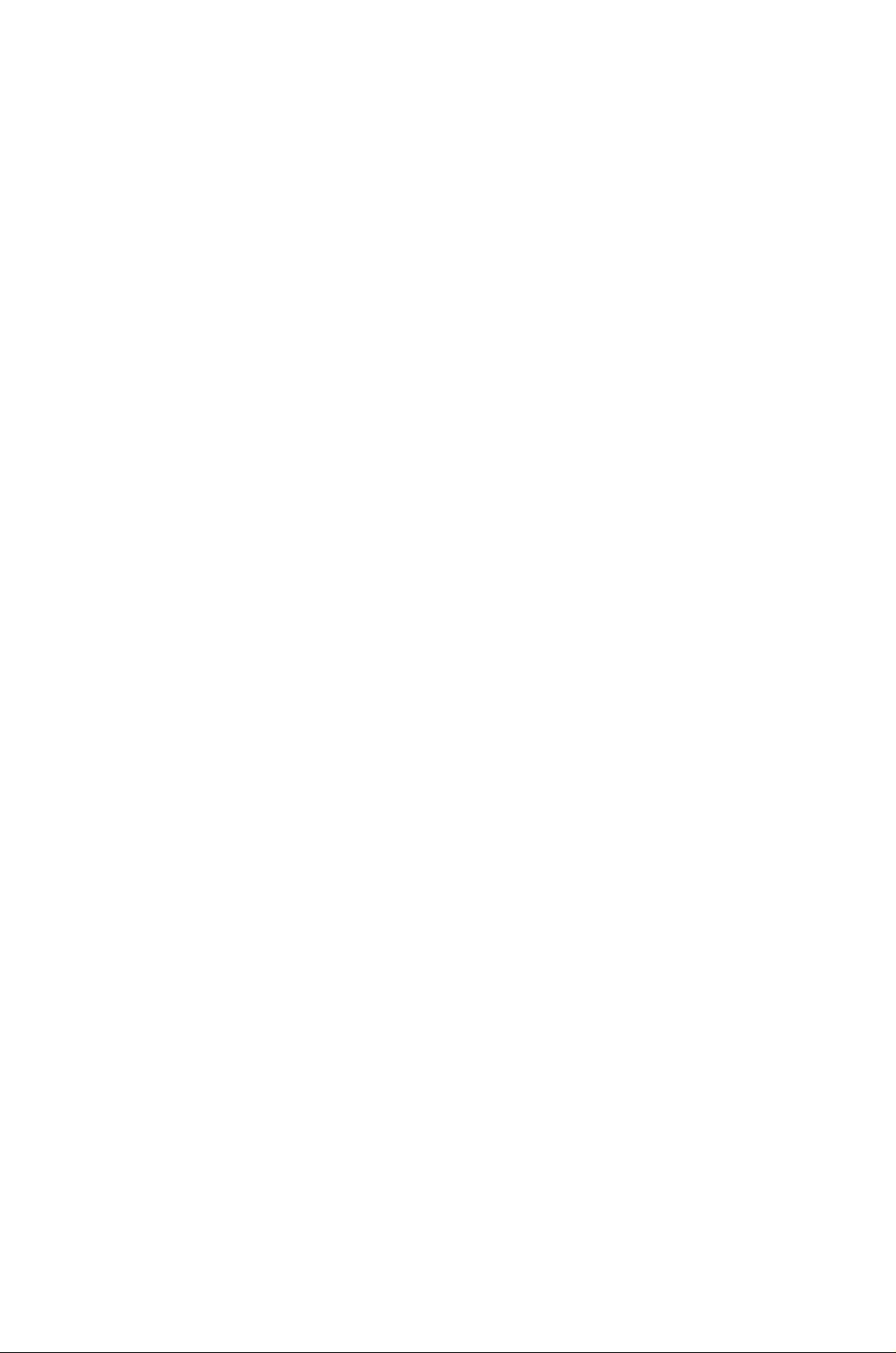
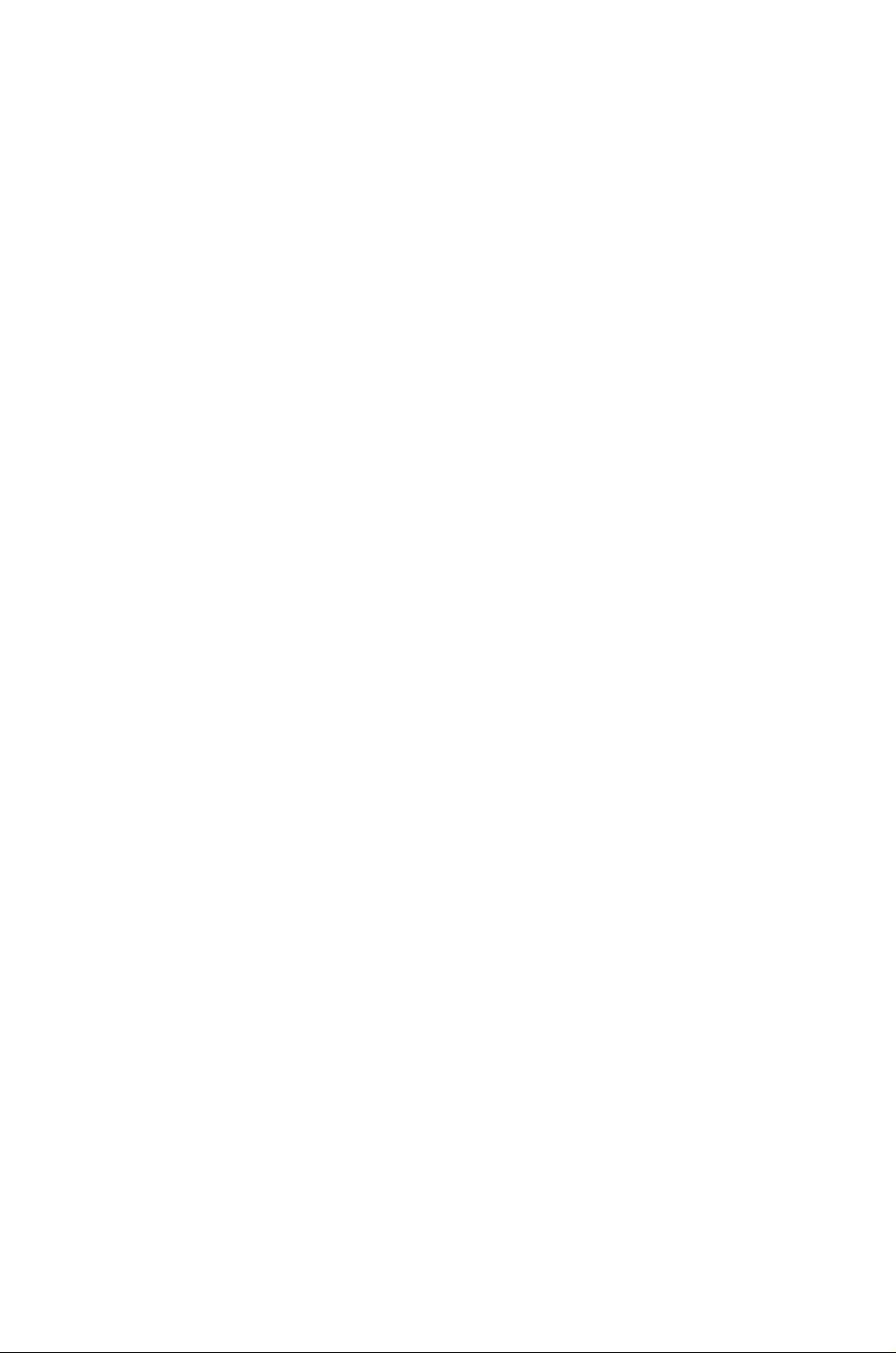
剩余591页未读,继续阅读
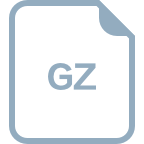

















- 粉丝: 238
- 资源: 1613
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- Vue实现iOS原生Picker组件:详细解析与实现思路
- Arduino蓝牙小车:参数调试与功能控制
- 百度Java面试精华:200页精选资源涵盖核心知识点
- Swift使用CoreData填坑指南:CoreData在Swift 3.0的变化
- 微距离无线充电器创新设计及其实验探索
- MTK Android平台开发全攻略:44步详解流程
- RecyclerView全面解析:替代ListView的新选择
- Android开发:自动适配中英文键盘解决方案
- Android调用WebService接口教程
- Android开发:BitmapUtil图片处理全解析与实例
- Android多线程断点续传实现详解
- PCA算法在人脸识别会议签到系统中的应用
- EventBus 3.0:Android事件总线详解与实战应用
- Android FileUtil:全面解析文件操作实用技巧与实例
- RecyclerView添加头部和尾部实战教程
- Android实现微博滑动固定顶部栏实战与优化

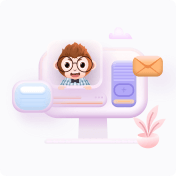
