本文是一份关于Linux 2.6.24内核调度器的复习笔记,由Kevin.Liu于2012年10月20日至2012年10月27日整理。笔记旨在帮助读者回顾和理解调度器的工作原理,而非原创内容,主要依据Wolfgang Mauerer的《深入理解Linux内核架构》以及相关博客、网站和论文。笔记重点涵盖了以下几个方面: 1. 调度器功能结构: - 运行队列(RunQueue):这是存储待调度进程的核心数据结构,每个CPU都有一个独立的运行队列。 - 核心调度器:包括主调度器和周期性调度器,主调度器负责选择下一个运行的进程,周期性调度器处理定时任务。 - 调度器类:组织和管理不同类型的调度策略,如实时进程和普通进程。 2. 进程排序与优先级: - 实时进程:这些进程有严格的优先级和执行时间限制,调度器会确保它们得到及时处理。 - 普通进程: - 模型建立:通过比较进程的优先级、执行时间片等参数,确定其在队列中的位置。 - 抽象模型总结:介绍了调度算法,如完全公平调度(CFS),它平衡了公平性和响应速度。 - 真实模型概述:解释了调度器如何在实际操作中根据这些模型进行进程调度。 3. 注意事项: - 笔记中的“队列”并非指抽象数据结构中的queue,而是指进程按照特定顺序排列的逻辑结构。 - 作者提醒读者可能存在谬误,鼓励读者质疑并指出错误,以避免误导他人。 - 文章允许自由转载,但要求在更新错误时同步更新,以防止错误传播。 通过这篇笔记,学习者可以深入了解Linux 2.6.24内核调度器的工作原理,特别是对于理解进程优先级控制和调度策略有极大帮助。同时,它也强调了理解和验证信息的重要性,鼓励读者在学习过程中保持批判性思维。
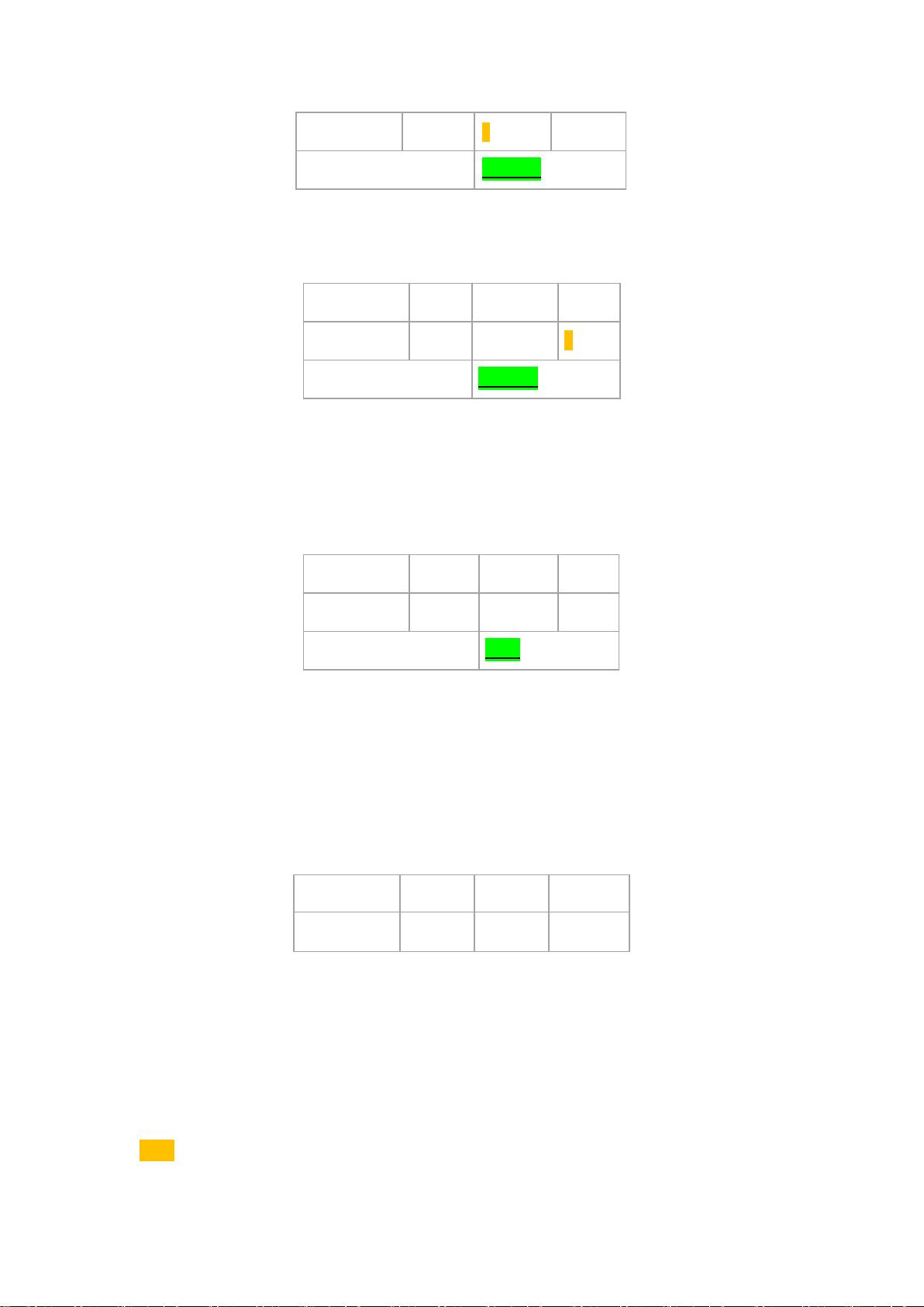
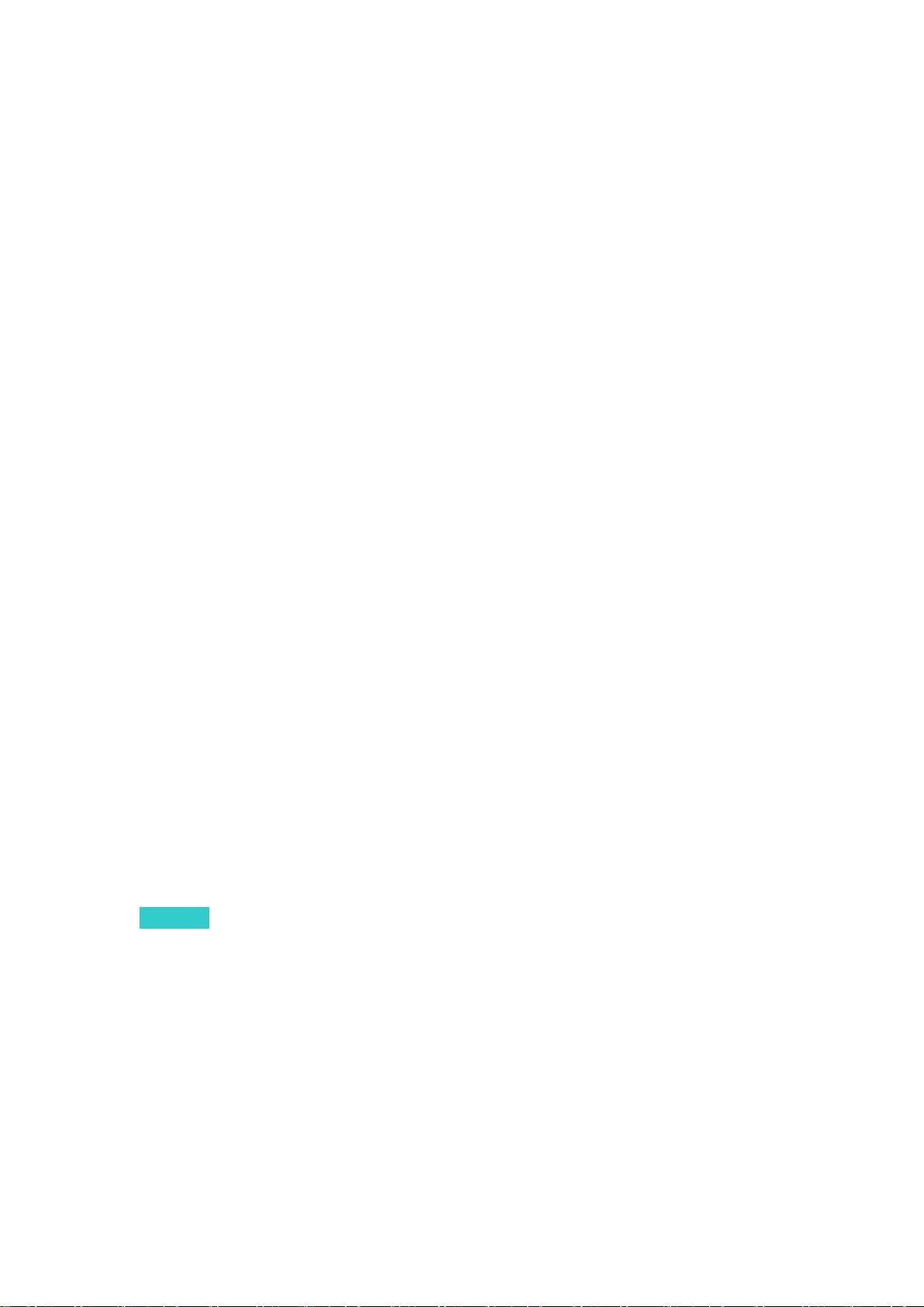
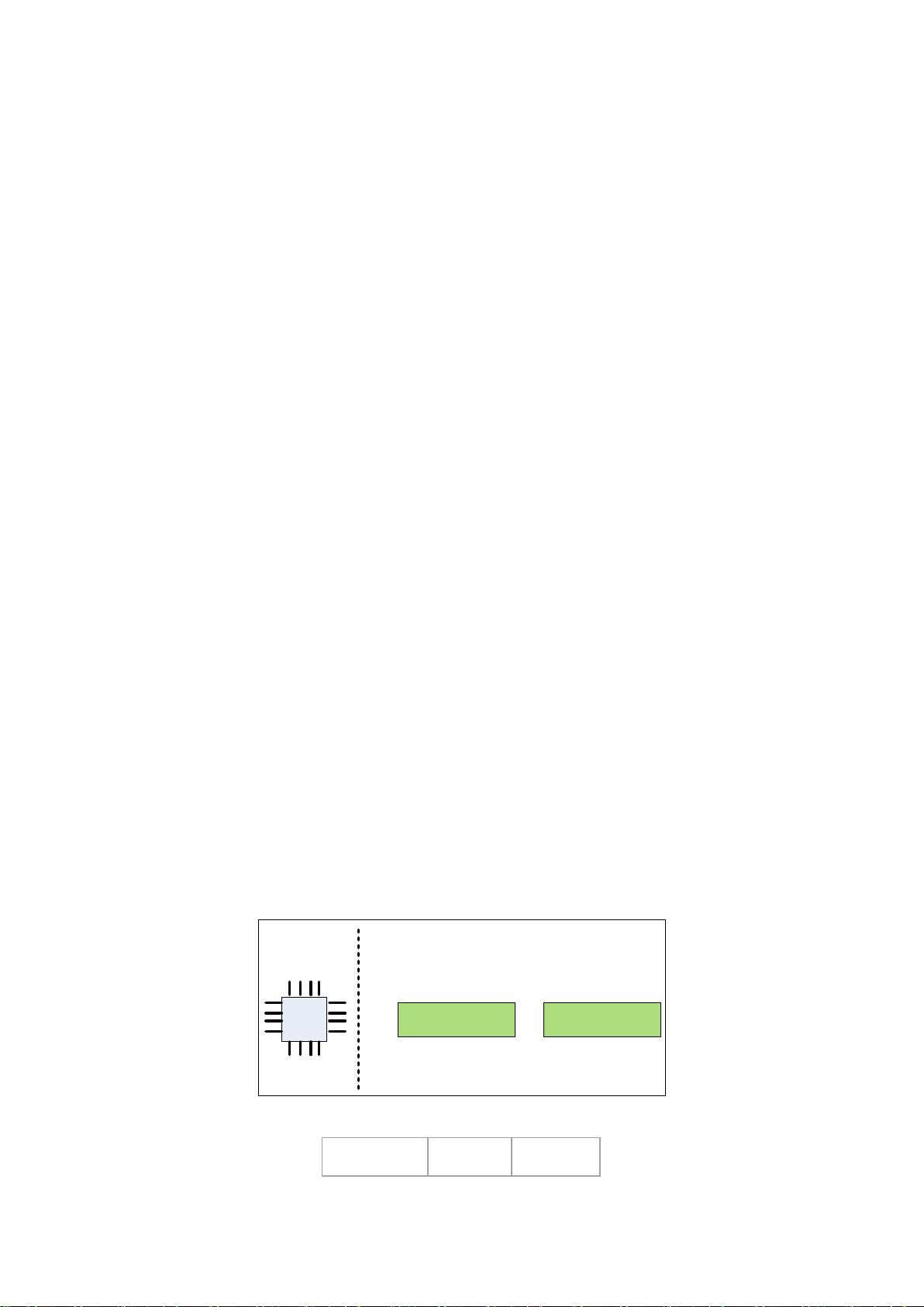
剩余59页未读,继续阅读
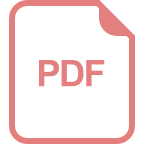
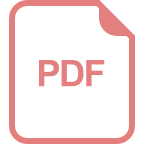



















- 粉丝: 17
- 资源: 7
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- Vue实现iOS原生Picker组件:详细解析与实现思路
- Arduino蓝牙小车:参数调试与功能控制
- 百度Java面试精华:200页精选资源涵盖核心知识点
- Swift使用CoreData填坑指南:CoreData在Swift 3.0的变化
- 微距离无线充电器创新设计及其实验探索
- MTK Android平台开发全攻略:44步详解流程
- RecyclerView全面解析:替代ListView的新选择
- Android开发:自动适配中英文键盘解决方案
- Android调用WebService接口教程
- Android开发:BitmapUtil图片处理全解析与实例
- Android多线程断点续传实现详解
- PCA算法在人脸识别会议签到系统中的应用
- EventBus 3.0:Android事件总线详解与实战应用
- Android FileUtil:全面解析文件操作实用技巧与实例
- RecyclerView添加头部和尾部实战教程
- Android实现微博滑动固定顶部栏实战与优化

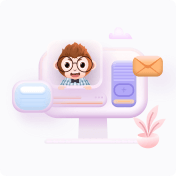
