或长子兄弟链存储结构(任选一种),利用python尝试编程实现:创建一棵树设计一个算法求高度设计一个算法实现树的层次遍历设计两个算法实现先根遍历和后根遍历并测试
时间: 2023-06-08 19:06:05 浏览: 55
非常感谢您的提问!以下是对您的问题的回答:
对于创建一棵树的问题,以长子兄弟链存储结构为例,可以定义一个树结点类来表示每个结点,该类应该包含结点值、长子结点和右兄弟结点等属性。下面是一个示例的树结点类的代码:
```python
class TreeNode:
def __init__(self, value):
self.value = value
self.first_child = None
self.right_sibling = None
```
接着,可以根据给定的树的形状,依次创建每个结点,并将它们以长子兄弟链的方式连接起来。下面是一个示例的树构造函数:
```python
def build_tree():
# 创建根结点
root = TreeNode(1)
# 创建第一层子结点
root.first_child = TreeNode(2)
root.first_child.right_sibling = TreeNode(3)
# 创建第二层子结点
root.first_child.first_child = TreeNode(4)
root.first_child.first_child.right_sibling = TreeNode(5)
root.first_child.right_sibling.first_child = TreeNode(6)
# 返回根结点
return root
```
接下来,可以设计一个递归函数来求树的高度(深度):
```python
def get_height(root):
if root is None:
return 0
else:
height = 1
p = root.first_child
while p is not None:
height = max(height, get_height(p) + 1)
p = p.right_sibling
return height
```
对于树的层次遍历,可以用队列来辅助实现。以打印每个结点的值为例,可以进行如下的层次遍历:
```python
from queue import Queue
def level_order_traversal(root):
q = Queue()
q.put(root)
while not q.empty():
p = q.get()
print(p.value)
p = p.first_child
while p is not None:
q.put(p)
p = p.right_sibling
```
最后,对于先根遍历和后根遍历,可以分别设计递归函数来实现。以下是示例代码:
```python
def pre_order_traversal(root):
if root is not None:
print(root.value)
pre_order_traversal(root.first_child)
pre_order_traversal(root.right_sibling)
def post_order_traversal(root):
if root is not None:
post_order_traversal(root.first_child)
post_order_traversal(root.right_sibling)
print(root.value)
```
为了测试以上函数的正确性,可以对build_tree()函数返回的树进行如下的测试:
```python
if __name__ == '__main__':
tree = build_tree()
print(get_height(tree)) # 输出:3
level_order_traversal(tree) # 输出:1 2 3 4 5 6
pre_order_traversal(tree) # 输出:1 2 4 5 3 6
post_order_traversal(tree) # 输出:4 5 2 6 3 1
```
以上就是对于您的问题的解答。希望我的回答能够帮助到您!
相关推荐
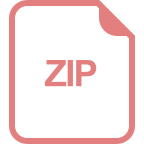
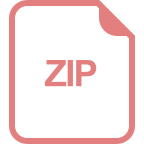














