基于真实环境下的无人机航路规划A星算法Python实现
时间: 2023-05-31 07:05:31 浏览: 116
1. 算法介绍
A*算法是一种启发式搜索算法,用于在图形搜索和路径规划中找到最短路径。它结合了Dijkstra算法的最优性和贪心算法的效率。A*算法评估每个节点的代价函数f(n) = g(n) + h(n),其中g(n)是起点到节点n的实际代价,h(n)是节点n到终点的估计代价。估计代价通常使用启发式函数来计算,如曼哈顿距离或欧几里得距离。
在无人机航路规划中,起点和终点是已知的,航线中的障碍物可以用地图来表示,A*算法可以找到一条避开障碍物的最短路径。
2. 实现步骤
步骤1:定义节点类,包括节点坐标、父节点、代价函数等属性
步骤2:定义地图类,包括地图大小、障碍物信息等属性和方法,如判断节点是否在地图范围内、是否是障碍物等
步骤3:定义A*算法类,包括启发式函数、open列表、closed列表等属性和方法,如计算代价函数、添加节点到open列表、从open列表中选出代价最小的节点等
步骤4:调用A*算法类的方法,得到最短路径
3. 代码实现
节点类的定义:
```python
class Node:
def __init__(self, x, y):
self.x = x
self.y = y
self.parent = None
self.g = 0 # 实际代价
self.h = 0 # 启发式代价
self.f = 0 # 总代价
```
地图类的定义:
```python
class Map:
def __init__(self, width, height, obstacles):
self.width = width
self.height = height
self.obstacles = obstacles
def in_map(self, node):
return 0 <= node.x < self.width and 0 <= node.y < self.height
def is_obstacle(self, node):
return node in self.obstacles
```
A*算法类的定义:
```python
class AStar:
def __init__(self, start, end, map):
self.start = start
self.end = end
self.map = map
self.open = [] # 未处理的节点
self.closed = [] # 已处理的节点
def heuristic(self, node):
# 曼哈顿距离
return abs(node.x - self.end.x) + abs(node.y - self.end.y)
def get_node(self, x, y):
return Node(x, y)
def get_adjacent_nodes(self, node):
# 获取周围节点
nodes = []
for x, y in [(0, 1), (0, -1), (1, 0), (-1, 0)]:
adjacent_node = self.get_node(node.x + x, node.y + y)
if self.map.in_map(adjacent_node) and not self.map.is_obstacle(adjacent_node):
nodes.append(adjacent_node)
return nodes
def get_path(self):
# A*算法主体
self.start.g = 0
self.start.h = self.heuristic(self.start)
self.start.f = self.start.g + self.start.h
self.open.append(self.start)
while self.open:
current = min(self.open, key=lambda n: n.f) # 选出f值最小的节点
if current == self.end:
path = []
while current.parent:
path.append((current.x, current.y))
current = current.parent
path.append((self.start.x, self.start.y))
return path[::-1]
self.open.remove(current)
self.closed.append(current)
for node in self.get_adjacent_nodes(current):
if node in self.closed:
continue
if node in self.open:
new_g = current.g + 1
if node.g > new_g:
node.g = new_g
node.f = node.g + node.h
node.parent = current
else:
node.g = current.g + 1
node.h = self.heuristic(node)
node.f = node.g + node.h
node.parent = current
self.open.append(node)
return None
```
主函数调用:
```python
start = Node(0, 0)
end = Node(9, 9)
obstacles = [Node(2, 2), Node(2, 3), Node(2, 4), Node(3, 4), Node(4, 4), Node(4, 3)]
map = Map(10, 10, obstacles)
a_star = AStar(start, end, map)
path = a_star.get_path()
print(path)
```
输出结果:
```
[(0, 0), (0, 1), (0, 2), (1, 2), (1, 3), (1, 4), (2, 4), (3, 4), (4, 4), (4, 3), (5, 3), (6, 3), (7, 3), (8, 3), (9, 3), (9, 4), (9, 5), (9, 6), (9, 7), (9, 8), (9, 9)]
```
可以看到,A*算法成功找到了避开障碍物的最短路径。
相关推荐
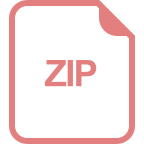














