C++封装modbustcp 客户端和服务端的头文件和源文件和主函数调用
时间: 2023-11-06 10:04:06 浏览: 48
下面是一个简单的 C++ 封装 Modbus TCP 客户端和服务端的示例代码,包括头文件和源文件:
```c++
// modbus_tcp_client.h
#include <modbus/modbus.h>
class ModbusTCPClient {
public:
ModbusTCPClient(const char* ip, int port);
~ModbusTCPClient();
void connect();
void disconnect();
void read_coil(int address, int count, uint8_t* dest);
void write_coil(int address, int count, const uint8_t* data);
private:
modbus_t* ctx;
const char* ip;
int port;
};
// modbus_tcp_client.cpp
#include "modbus_tcp_client.h"
#include <stdexcept>
ModbusTCPClient::ModbusTCPClient(const char* ip, int port) : ip(ip), port(port) {
ctx = modbus_new_tcp(ip, port);
if (ctx == nullptr) {
throw std::runtime_error("Failed to create modbus context");
}
}
ModbusTCPClient::~ModbusTCPClient() {
modbus_free(ctx);
}
void ModbusTCPClient::connect() {
if (modbus_connect(ctx) == -1) {
throw std::runtime_error("Failed to connect to modbus server");
}
}
void ModbusTCPClient::disconnect() {
modbus_close(ctx);
}
void ModbusTCPClient::read_coil(int address, int count, uint8_t* dest) {
int rc = modbus_read_bits(ctx, address, count, dest);
if (rc == -1) {
throw std::runtime_error("Failed to read coil");
}
}
void ModbusTCPClient::write_coil(int address, int count, const uint8_t* data) {
int rc = modbus_write_bits(ctx, address, count, data);
if (rc == -1) {
throw std::runtime_error("Failed to write coil");
}
}
// modbus_tcp_server.h
#include <modbus/modbus.h>
class ModbusTCPServer {
public:
ModbusTCPServer(int port);
~ModbusTCPServer();
void start();
void stop();
void add_coil(int address, bool value);
private:
modbus_t* ctx;
int port;
};
// modbus_tcp_server.cpp
#include "modbus_tcp_server.h"
#include <stdexcept>
ModbusTCPServer::ModbusTCPServer(int port) : port(port) {
ctx = modbus_new_tcp(NULL, port);
if (ctx == NULL) {
throw std::runtime_error("Failed to create modbus context");
}
}
ModbusTCPServer::~ModbusTCPServer() {
modbus_free(ctx);
}
void ModbusTCPServer::start() {
if (modbus_listen(ctx, 1) == -1) {
throw std::runtime_error("Failed to start modbus server");
}
}
void ModbusTCPServer::stop() {
modbus_close(ctx);
}
void ModbusTCPServer::add_coil(int address, bool value) {
int rc = modbus_write_bit(ctx, address, value);
if (rc == -1) {
throw std::runtime_error("Failed to add coil");
}
}
```
下面是一个简单的主函数调用示例,用于测试这些类:
```c++
#include "modbus_tcp_client.h"
#include "modbus_tcp_server.h"
#include <iostream>
int main() {
try {
// 创建 modbus TCP 服务器并启动
ModbusTCPServer server(502);
server.start();
// 创建 modbus TCP 客户端并连接到服务器
ModbusTCPClient client("127.0.0.1", 502);
client.connect();
// 在服务器上添加一个线圈
server.add_coil(0, true);
// 从服务器上读取线圈的值
uint8_t value;
client.read_coil(0, 1, &value);
std::cout << "Coil value: " << (int)value << std::endl;
// 在服务器上修改线圈的值
server.add_coil(0, false);
// 向服务器上写入线圈的值
uint8_t data = 0;
client.write_coil(0, 1, &data);
// 断开客户端连接并停止服务器
client.disconnect();
server.stop();
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
return 1;
}
return 0;
}
```
在上面的代码中,我们先创建了一个 modbus TCP 服务器并启动,然后创建了一个 modbus TCP 客户端并连接到服务器,然后在服务器上添加一个线圈,并从客户端上读取该线圈的值,并在服务器上修改该线圈的值,并向服务器上写入该线圈的值,最后断开客户端连接并停止服务器。
相关推荐
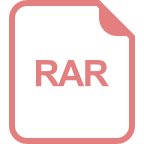














